Learn how to display the current device location on a map or scene.
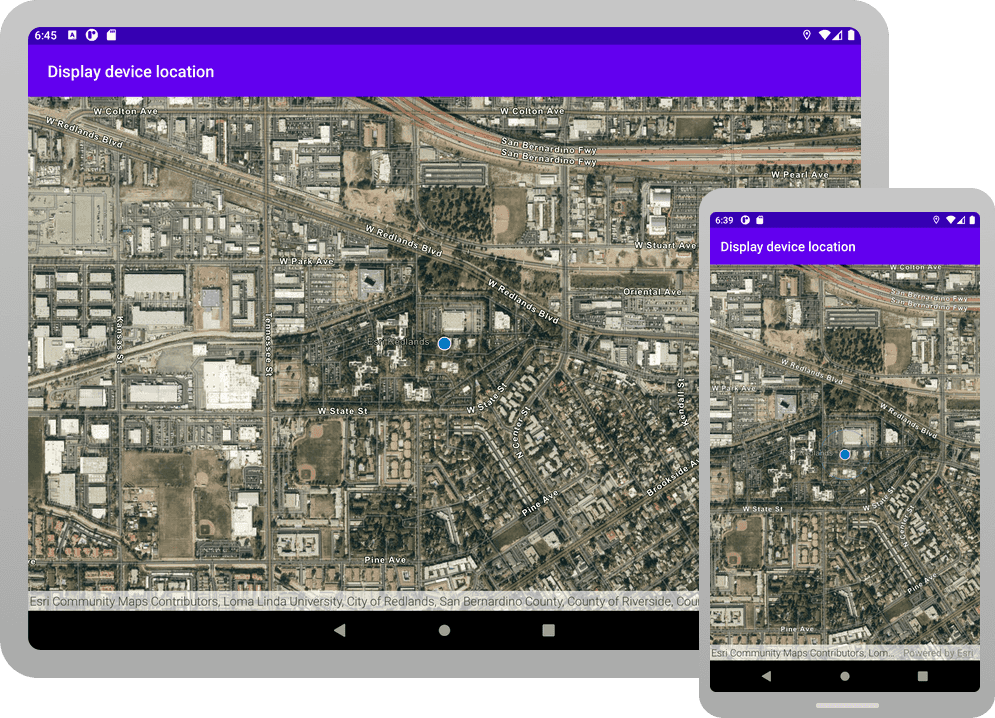
You can display the device location on a map or scene. This is important for workflows that require the user's current location, such as finding nearby businesses, navigating from the current location, or identifying and collecting geospatial information.
By default, location display uses the device's location provider. Your app can also process input from other location providers, such as an external GPS receiver or a provider that returns a simulated location. For more information, see the Show device location topic.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Display device location._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display device location</string> <string name="location_permission_denied">LocationDisplayManager cannot run because location permission was denied</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.
Change the value of
root
to "Display device location".Project.name settings.gradleUse dark colors for code blocks rootProject.name = "Display device location" include ':app'
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
method, find theApi Key For App() ArcGIS
call and paste your access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.Runtime Environment.set Api Key(" YOUR _ACCESS _TOKE N") MainActivity.ktUse dark colors for code blocks private fun setApiKeyForApp(){ ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN") }
-
Add import statements
-
Replace app-specific import statements with the imports (highlighted in yellow) needed for this tutorial.
MainActivity.ktUse dark colors for code blocks 17 18 19 20 21 22 34 35 36 37 38Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line package com.example.app import android.os.Bundle import androidx.appcompat.app.AppCompatActivity import android.content.pm.PackageManager import android.Manifest import android.widget.Toast import androidx.core.app.ActivityCompat import androidx.core.content.ContextCompat import com.esri.arcgisruntime.ArcGISRuntimeEnvironment import com.esri.arcgisruntime.mapping.ArcGISMap import com.esri.arcgisruntime.mapping.BasemapStyle import com.esri.arcgisruntime.mapping.view.MapView import com.esri.arcgisruntime.mapping.view.LocationDisplay import com.example.app.databinding.ActivityMainBinding class MainActivity : AppCompatActivity() {
Ask user for permission to display location
Set the user permissions to allow display location.
-
In Android.Manifest.xml, add permissions for coarse and fine location.
MainActivity.ktUse dark colors for code blocks Copy 9 10<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
-
In MainActivity.kt, create the
request
method.Permissions() Check whether your app already has permissions for accessing fine location and coarse location by calling
check
. If one or both permissions have not been granted, then request permission from the user by callingSelf Permission() Activity
. Otherwise, show a Toast with a non-permission error, if any, retrieved from the status changed event that is passed to the current method.Compat.request Permissions() MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private fun requestPermissions(dataSourceStatusChangedEvent: LocationDisplay.DataSourceStatusChangedEvent) { val requestCode = 2 val reqPermissions = arrayOf( Manifest.permission.ACCESS_FINE_LOCATION, Manifest.permission.ACCESS_COARSE_LOCATION ) // fine location permission val permissionCheckFineLocation = ContextCompat.checkSelfPermission(this@MainActivity, reqPermissions[0]) == PackageManager.PERMISSION_GRANTED // coarse location permission val permissionCheckCoarseLocation = ContextCompat.checkSelfPermission(this@MainActivity, reqPermissions[1]) == PackageManager.PERMISSION_GRANTED if (!(permissionCheckFineLocation && permissionCheckCoarseLocation)) { // if permissions are not already granted, request permission from the user ActivityCompat.requestPermissions(this@MainActivity, reqPermissions, requestCode) } else { // report other unknown failure types to the user - for example, location services may not // be enabled on the device. val message = String.format( "Error in DataSourceStatusChangedListener: %s", dataSourceStatusChangedEvent .source.locationDataSource.error.message ) Toast.makeText(this@MainActivity, message, Toast.LENGTH_LONG).show() } }
-
Override the
on
callback inherited from theRequest Permissions Result() Fragment
class.Activity If the grant results array indicates that location permissions were granted, then start the location display. (In this tutorial, we test only the first permission in the array, which is for fine location.)
When the
location
call inDisplay.start Async() setup
fails to start the location display, the status of the data source changes, causing the status change listener's lambda to be executed. (You will editMap() setup
and add the data source status change listener in the next section of this tutorial: Show the current location.)Map() The lambda calls this app's
request
method, which ultimately results inPermissions() on
being called. At this point, you verified location permissions, so it's time for another attempt to start location display.Request Permissions Result() MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. override fun onRequestPermissionsResult( requestCode: Int, permissions: Array<String>, grantResults: IntArray ) { super.onRequestPermissionsResult(requestCode, permissions, grantResults) // if request is cancelled, the results array is empty if (grantResults.isNotEmpty() && grantResults[0] == PackageManager.PERMISSION_GRANTED) { locationDisplay.startAsync() } }
-
If location permissions were not granted, show a Toast saying that permissions were denied.
MainActivity.ktUse dark colors for code blocks 119 120 121 122 123 124 125 126 127 128 129 137 138Add line. Add line. Add line. Add line. Add line. Add line. Add line. override fun onRequestPermissionsResult( requestCode: Int, permissions: Array<String>, grantResults: IntArray ) { super.onRequestPermissionsResult(requestCode, permissions, grantResults) // if request is cancelled, the results array is empty if (grantResults.isNotEmpty() && grantResults[0] == PackageManager.PERMISSION_GRANTED) { locationDisplay.startAsync() } else { Toast.makeText( this@MainActivity, resources.getString(R.string.location_permission_denied), Toast.LENGTH_SHORT ).show() } }
Show the current location
Each map view has its own instance of a
LocationDisplay
for showing the current location (point) of the device. The location is displayed as an overlay in the map view.
Instances of this class manage the display of device location on a map view: the symbols, animation, auto pan behavior, and so on. Location display is an overlay of the map view, and displays above everything else, including graphics overlays.
The location display does not retrieve location information, that is the job of the associated data source, which provides location updates on a regular basis. In addition to the default system location data source, you can use location providers based on external GPS devices or a simulated location source.
Each map view has its own instance of a location display and instances of location display and location data source are not shared by multiple map views. This allows you to start and stop location display independently on multiple map views without affecting each other.
-
In
setup
, delete the code that sets the map's initial viewpoint.Map MainActivity.ktUse dark colors for code blocks // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle streets val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) // set the map to be displayed in the layout's MapView mapView.map = map // set the viewpoint, Viewpoint(latitude, longitude, scale) mapView.setViewpoint(Viewpoint(34.0270, -118.8050, 72000.0)) }
-
In
setup
, add a listener that detects when the status of the location data source changes. In the listener's lambda, call the request permissions method so the user can approve location permissions.Map() MainActivity.ktUse dark colors for code blocks 82 83 84 85 86 87 88 89 98Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle Topographic val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) // set the map to be displayed in the layout's MapView mapView.map = map locationDisplay.addDataSourceStatusChangedListener { // if LocationDisplay isn't started or has an error if (!it.isStarted && it.error != null) { // check permissions to see if failure may be due to lack of permissions requestPermissions(it) } } }
-
Set a
LocationDisplay.AutoPanMode
that centers the map at the device location. Then try to start the location display.MainActivity.ktUse dark colors for code blocks 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 100 101Add line. Add line. // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle Topographic val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) // set the map to be displayed in the layout's MapView mapView.map = map locationDisplay.addDataSourceStatusChangedListener { // if LocationDisplay isn't started or has an error if (!it.isStarted && it.error != null) { // check permissions to see if failure may be due to lack of permissions requestPermissions(it) } } locationDisplay.setAutoPanMode(LocationDisplay.AutoPanMode.RECENTER) locationDisplay.startAsync() }
Run your app
-
Click Run > Run > app to run the app.
The Android Emulator should display and run your app in the Android Virtual Devcie (AVD) selected in the Android Studio toolbar:
If your app builds but no AVD displays, you need to add one. Click Tools > AVD Manager > Create Virtual Device...
You should see your current location displayed on the map. Different location symbols are used depending on the auto pan mode and whether a location is acquired. See
LocationDisplay.AutoPanMode
for details.By default, a round blue symbol is used to display the device's location. The location data source tries to get the most accurate location available but depending upon signal strength, satellite positions, and other factors, the location reported could be an approximation. A semi-transparent circle around the location symbol indicates the range of accuracy. As the device moves and location updates are received, the location symbol will be repositioned on the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: