Learn how to create and display a scene from a web scene stored in ArcGIS.
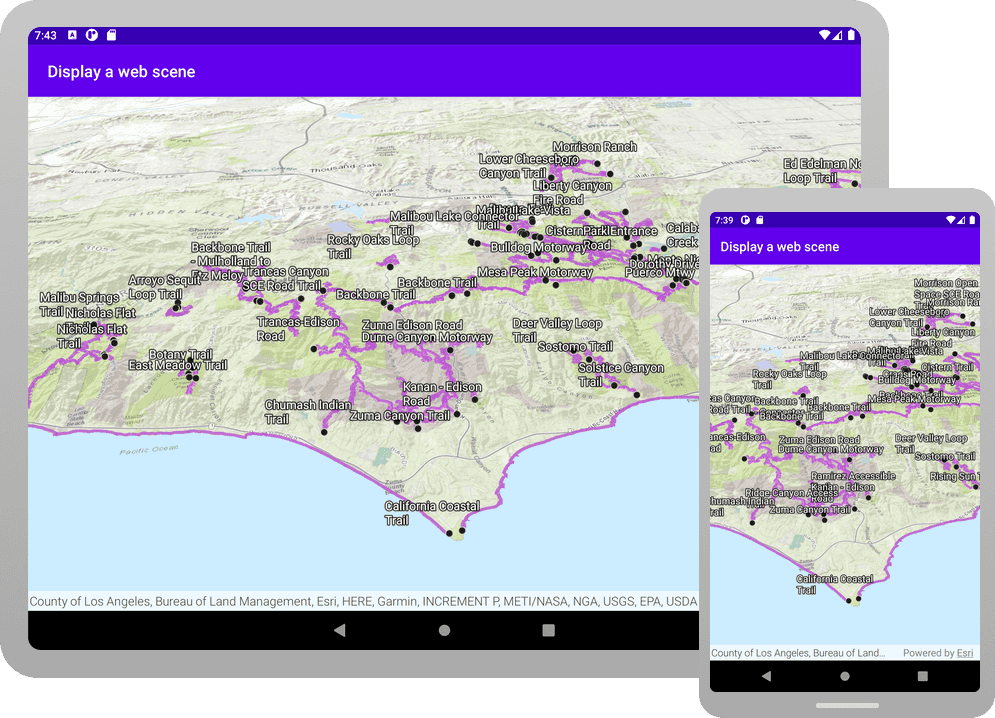
This tutorial shows you how to create and display a scene from a web scene. All web scenes are stored in ArcGIS with a unique item ID. You will access an existing web scene by item ID and display its layers. The web scene contains feature layers for the Santa Monica Mountains in California.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Create a new Android Studio project
-
To start this tutorial, complete the Display a scene tutorial. Or download and unzip the Display a scene solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Display a web scene._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display a web scene</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.
Change the value of
root
to "Display a web scene".Project.name settings.gradleUse dark colors for code blocks rootProject.name = "Display a web scene" include ':app'
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
method, find theApi Key For App() ArcGIS
call and paste your access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.Runtime Environment.set Api Key(" YOUR _ACCESS _TOKE N") MainActivity.ktUse dark colors for code blocks private fun setApiKeyForApp(){ ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN") }
-
Prepare files before coding the app
Modify the files from the Display a scene
tutorial so that you can use them in this tutorial: you will add imports, change the application title, and remove unnecessary code.
-
In the Android Studio, in the Android tool window, open app > java > com.example.app and then click MainActivity. Add the following imports, replacing those from the
Display a scene
tutorial.MainActivity.ktUse dark colors for code blocks package com.example.app import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import com.esri.arcgisruntime.ArcGISRuntimeEnvironment import com.esri.arcgisruntime.mapping.ArcGISScene import com.esri.arcgisruntime.mapping.view.SceneView import com.esri.arcgisruntime.portal.Portal import com.esri.arcgisruntime.portal.PortalItem import com.example.app.databinding.ActivityMainBinding
-
In
start()
, delete the code for base surface, surface elevation, camera, and camera viewpoint. The web scene defines these characteristics, so you don't have to set them in your app.MainActivity.ktUse dark colors for code blocks // set up your scene here. You will call this method from onCreate() private fun setupScene() { val scene = ArcGISScene(BasemapStyle.ARCGIS_IMAGERY_STANDARD) // set the scene on the scene view sceneView.scene = scene // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface(listOf(elevationSource)) // add an exaggeration factor to increase the 3D effect of the elevation. surface.elevationExaggeration = 2.5f scene.baseSurface = surface // Point(x, y, z, spatialReference) val cameraLocation = Point(-118.794, 33.909, 5330.0, SpatialReferences.getWgs84()) // Camera(location, heading, pitch, roll) val camera = Camera(cameraLocation, 355.0, 72.0, 0.0) sceneView.setViewpointCamera(camera) }
-
In
start()
, delete the code that creates anArcGIS
by passing a basemap. In this tutorial, you will pass a portal item instead.Scene MainActivity.ktUse dark colors for code blocks // set up your scene here. You will call this method from onCreate() private fun setupScene() { val scene = ArcGISScene(BasemapStyle.ARCGIS_IMAGERY_STANDARD) // set the scene on the scene view sceneView.scene = scene }
Get the web scene item ID
You can use ArcGIS tools to create and view web scenes. Use the Scene Viewer to identify the web scene item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web scene in the Scene Viewer in ArcGIS Online. This web scene displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be 579f97b2f3b94d4a8e48a5f140a6639b.
Display the web scene
You can display a web scene using the web scene's item ID. Create a scene from the web scene portal item, and display it in your app.
-
In the
setup
method, create a newScene() Portal
referencing ArcGIS Online as theportal
parameter andUrl false
for thelogin
parameter.Required MainActivity.ktUse dark colors for code blocks 73 74 75 77 78 79 80 81 82Add line. // set up your scene here. You will call this method from onCreate() private fun setupScene() { val portal = Portal("https://www.arcgis.com", false) // set the scene on the scene view sceneView.scene = scene }
-
Create a
Portal
for the web scene, by passing theItem portal
and the web scene's item ID as parameters. -
Create an
ArcGIS
using theScene portal
as the constructor parameter.Item MainActivity.ktUse dark colors for code blocks 73 74 75 76 77 80 82 83 84 85 86 87Add line. Add line. Add line. // set up your scene here. You will call this method from onCreate() private fun setupScene() { val portal = Portal("https://www.arcgis.com", false) val itemId = "579f97b2f3b94d4a8e48a5f140a6639b" val portalItem = PortalItem(portal, itemId) val scene = ArcGISScene(portalItem) // set the scene on the scene view sceneView.scene = scene }
-
Use the existing code to display the new
ArcGIS
on the scene view by setting theScene scene
property on thescene
.View MainActivity.ktUse dark colors for code blocks Copy 73 74 75 76 77 78 79 80 81 82 83 86 87// set up your scene here. You will call this method from onCreate() private fun setupScene() { val portal = Portal("https://www.arcgis.com", false) val itemId = "579f97b2f3b94d4a8e48a5f140a6639b" val portalItem = PortalItem(portal, itemId) val scene = ArcGISScene(portalItem) // set the scene on the scene view sceneView.scene = scene }
-
Click Run > Run > app to run the app.
Your app should display the scene that you viewed earlier in the Scene Viewer.
What's Next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: