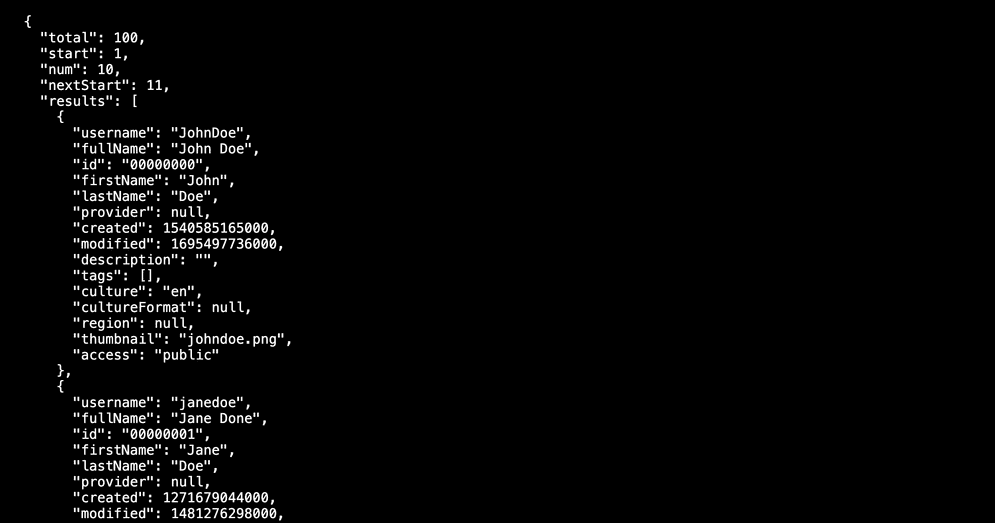
View members in your organization using an API key
An Application Programming Interface key (API key) is a long-lived access token that defines the scope and permission for granting a public-facing application access to ready-to-use services. With ArcGIS REST JS, you use the Api
class to set your API key before accessing services.
In this tutorial, you create and configure an access token to view members in your organization.
Prerequisites
An ArcGIS Location Platform, ArcGIS Online, or ArcGIS Enterprise account.
Steps
Create a new app
Create a new CodePen app with a <pre
element that is the full width and height of the browser window.
-
Go to CodePen and create a new pen for your application.
-
In CodePen > HTML, add HTML and CSS to create a page with a
<pre
element called> result
.Use the HTML to create a page with a map. Use the
map
div to display the map. Use the CSS to make the map full width and height.The
<!
tag is not required in CodePen. If you are using a different editor or running the page on a local server, be sure to add this tag to the top of your HTML page.DOCTYP E html > Use dark colors for code blocks <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width" /> <title>ArcGIS REST JS</title> <style> body { font-family: monospace; color: white; background: #000000; } pre { overflow: auto; padding: 1rem; background: #000000; } </style> </head> <body> <pre id="result"></pre> </body> </html>
Set up authentication
Create a new API key credential with the correct privileges to access the resources used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges:
- General privileges > Members > View
- Privileges:
- Copy the API key access token to your clipboard when prompted.
Set developer credentials
-
Add references to the
arcgis-rest-request
library. The library contains request/response processing, authentication helpers, and error handling.Use dark colors for code blocks <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width" /> <title>ArcGIS REST JS</title> <script src="https://unpkg.com/@esri/arcgis-rest-request@4/dist/bundled/request.umd.js"></script>
-
Create an
access
variable and set its value to your API key. Create anToken authentication
variable that will call thefrom
method to create an instance ofKey Api
.Key Manager Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken);
Search for members in the organization
-
Define a
url
variable and assign it the portal URL where the request will be sent.Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken); const url = "https://www.arcgis.com/sharing/rest/community/users" </script>
-
Use the
request
function to retrieve a list of users in your organization whose names contain the textjohn
. Provide the search parametersq
in the request options to filter the results. Then, print out the JSON result.: "john" Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken); const url = "https://www.arcgis.com/sharing/rest/community/users" arcgisRest.request(url, { authentication, params: { q: "john", // The query string to search the users against f: "json" } }).then(response => { document.getElementById("result").textContent = JSON.stringify(response, null, 2); }) </script>
Run the app
Run the app.
The result should look similar to this.What's next?
Learn how to use additional ArcGIS location services in these tutorials: