User authentication (formerly ArcGIS identity) generates a short-lived access token, authorizing your application to access location services, content, and resources on behalf of a logged in ArcGIS user.
The temporary token from user authentication is created using the OAuth 2.0 protocol. It authorizes your application to act on the user's behalf without revealing their secure password to your application. If your application will access your users' secure content in ArcGIS or if you plan to distribute your application through ArcGIS Marketplace, you must use user authentication.
ArcGIS REST JS provides helper methods for the Node.js server environment to handle authentication in your applications. In this tutorial, you use an OAuth2.0 server-enabled workflow. With server-side authentication, you can use the refresh token generated from the session and stored on your server environment to get a short-lived access token for the user.
Prerequisites
An ArcGIS Location Platform, ArcGIS Online, or ArcGIS Enterprise account.
Steps
Create OAuth 2.0 credentials
Create a new OAuth credential to register the application.
- Go to the Create OAuth credentials for user authentication tutorial to create an OAuth credential.
- Copy the Client ID and Redirect URL from your OAuth credentials item and paste them to a safe location. They will be used in a later step.
Set up a configuration file
-
In the item page of your OAuth credentials, copy your client ID. Ensure that
http//localhost
is registered as a redirect URL in the item settings.:3000/authenticate -
Generate a session secret and an encryption key. These are used to encrypt session information on the server. You can generate random, secure strings at RandomKeygen.
-
Open a code editor and create a
config.json
file. Set theCLIENT
and_ID REDIRECT
to the values you configured in your OAuth credentials. Set the_URI SESSION
and_SECRET ENCRYPTION
values to those generated in the previous step. Set the_KEY PORTAL
value to your portal URL._URL config.jsonUse dark colors for code blocks { "CLIENT_ID": "CLIENT_ID", "SESSION_SECRET": "SESSION_SECRET", "ENCRYPTION_KEY": "ENCRYPTION_KEY", "REDIRECT_URI": "http://localhost:3000/authenticate", "PORTAL_URL": "https://{machine.domain.com}/{webadaptor}/sharing/rest" }
Import modules
By using the Node.js express
server environment along with express-session
and session-file-store
, the user's session will persist on the server. The session is not stored on the client-side application and there is no permanent, persistent token stored on the user's machine.
-
In a separate
server.js
file, import theexpress
,express-session
, andsession-file-store
npm packages, as well as the ArcGIS REST JSArcGIS
class.Identity Manager server.jsUse dark colors for code blocks require("cross-fetch/polyfill"); require("isomorphic-form-data"); const express = require("express"); const session = require("express-session"); const FileStore = require("session-file-store")(session); const app = express(); const { ArcGISIdentityManager } = require("@esri/arcgis-rest-request");
-
Create an instance of
express
and acredentials
object to set theclient
,Id redirect
, andUrl portal
.server.jsUse dark colors for code blocks const express = require("express"); const session = require("express-session"); const FileStore = require("session-file-store")(session); const app = express(); const { ArcGISIdentityManager } = require("@esri/arcgis-rest-request"); const { CLIENT_ID, SESSION_SECRET, ENCRYPTION_KEY, REDIRECT_URI, PORTAL_URL } = require("./config.json"); const credentials = { clientId: CLIENT_ID, redirectUri: REDIRECT_URI, portal: PORTAL_URL // OPTIONAL - For ArcGIS Enterprise only };
Get user session
When the user is redirected to the sign in page, the authorize
method will be called to take the provided credentials and the response object. After the user is redirected back to the authenticate
page, the exchange
method will be used to exchange the code from the redirect for a token and instantiate a new user session.
-
Call the
authorize
method withcredentials
andres
(the response object) as parameters when the user gets redirected to the sign in page.server.jsUse dark colors for code blocks const credentials = { clientId: CLIENT_ID, redirectUri: REDIRECT_URI, portal: PORTAL_URL // OPTIONAL - For ArcGIS Enterprise only }; app.get("/sign-in", (req, res) => { ArcGISIdentityManager.authorize(credentials, res); });
-
Destroy
the cookie and session file when the user signs out and returns to the home page.server.jsUse dark colors for code blocks app.get("/sign-in", (req, res) => { ArcGISIdentityManager.authorize(credentials, res); }); app.get("/sign-out", (req, res) => { // invalidate the token ArcGISIdentityManager.destroy(req.session.arcgis).then(() => { // delete the cookie and stored session }); });
-
Redirect the user to the authorization page. Call the
exchange
to use the credentials and exchange the code for a token in the session.Authorization Code server.jsUse dark colors for code blocks app.get("/sign-out", (req, res) => { // invalidate the token ArcGISIdentityManager.destroy(req.session.arcgis).then(() => { // delete the cookie and stored session }); }); app.get("/authenticate", async (req, res) => { req.session.arcgis = await ArcGISIdentityManager.exchangeAuthorizationCode( credentials, req.query.code //The code from the redirect: exchange code for a token in instaniated user session. );
-
Redirect the user to the home page and display session information when the user provides credentials.
server.jsUse dark colors for code blocks }); app.get("/", (req, res) => { //Redirect to homepage. if (req.session.arcgis) { res.send(` <h1>Hi ${req.session.arcgis.username}<h1> <pre><code>${JSON.stringify(req.session.arcgis, null, 2)}</code></pre> <a href="/sign-out">Sign Out<a> `); } else { res.send(`<a href="/sign-in">Sign In<a>`); } });
Store the session
When you set up a session, express
will set a cookie on the client to keep track of the session ID
and persist the corresponding session on the server. By assigning the user
to the request, the session ID
is saved on the user's machine as a secure, encrypted file.
-
Save the sesssion on the user's machine and redirect the user to the homepage.
server.jsUse dark colors for code blocks app.get("/sign-out", (req, res) => { // invalidate the token ArcGISIdentityManager.destroy(req.session.arcgis).then(() => { // delete the cookie and stored session req.session.destroy(); }); }); app.get("/authenticate", async (req, res) => { req.session.arcgis = await ArcGISIdentityManager.exchangeAuthorizationCode( credentials, req.query.code //The code from the redirect: exchange code for a token in instaniated user session. ); res.redirect("/"); });
-
Call the
use
method ofexpress
to start implementingexpress-session
. Set thesecret
to your application'sSESSION
. This is used to encrypt the cookies and session information._SECRET express-session
supports many different kinds of compatible stores. For a list of stores, see [compatible session stores(https://www.npmjs.com/package/express-session#compatible-session-stores).server.jsUse dark colors for code blocks const { CLIENT_ID, SESSION_SECRET, ENCRYPTION_KEY, REDIRECT_URI, PORTAL_URL } = require("./config.json"); const credentials = { clientId: CLIENT_ID, redirectUri: REDIRECT_URI, portal: PORTAL_URL // OPTIONAL - For ArcGIS Enterprise only }; app.use( session({ name: "ArcGIS REST JS server authentication tutorial", secret: SESSION_SECRET, resave: false, saveUninitialized: false, cookie: { maxAge: 2592000000 // 30 days in milliseconds },
-
Create a
File
to save the sessions on your server as files., and set theStore secret
with theENCRYPTION
you configured in the_KEY config.json
file.server.jsUse dark colors for code blocks app.use( session({ name: "ArcGIS REST JS server authentication tutorial", secret: SESSION_SECRET, resave: false, saveUninitialized: false, cookie: { maxAge: 2592000000 // 30 days in milliseconds }, store: new FileStore({ ttl: 2592000000 / 1000, // 30 days in seconds retries: 1, secret: ENCRYPTION_KEY, })
-
Set the
encoder
option with thesession
to serialize the session object before it is encrypted and stored on disk. TheObj decoder
decodes thesession
using the encryption key the user specifies.Content server.jsUse dark colors for code blocks app.use( session({ name: "ArcGIS REST JS server authentication tutorial", secret: SESSION_SECRET, resave: false, saveUninitialized: false, cookie: { maxAge: 2592000000 // 30 days in milliseconds }, store: new FileStore({ ttl: 2592000000 / 1000, // 30 days in seconds retries: 1, secret: ENCRYPTION_KEY, // define how to encode our session to text for storing in the file. We can use the `serialize()` method on the session for this. encoder: (sessionObj) => { console.log({ sessionObj }); sessionObj.arcgis = sessionObj.arcgis.serialize(); return JSON.stringify(sessionObj); }, // define how to turn the text from the session data back into an object. We can use the `ArcGISIdentityManager.deserialize()` method for this. decoder: (sessionContents) => { console.log({ sessionContents }); const sessionObj = JSON.parse(sessionContents); if (sessionObj.arcgis) { sessionObj.arcgis = ArcGISIdentityManager.deserialize(sessionObj.arcgis); } return sessionObj; } })
-
Set the
decode
option to deserialize thesession
into a JavaScript object.Obj server.jsUse dark colors for code blocks // define how to encode our session to text for storing in the file. We can use the `serialize()` method on the session for this. encoder: (sessionObj) => { console.log({ sessionObj }); sessionObj.arcgis = sessionObj.arcgis.serialize(); return JSON.stringify(sessionObj); }, // define how to turn the text from the session data back into an object. We can use the `ArcGISIdentityManager.deserialize()` method for this. decoder: (sessionContents) => { console.log({ sessionContents }); const sessionObj = JSON.parse(sessionContents); if (sessionObj.arcgis) { sessionObj.arcgis = ArcGISIdentityManager.deserialize(sessionObj.arcgis); } return sessionObj; }
Run the app
-
Call the
listen
method and place aconsole.log
to allow the user to navigate tohttp
.://localhost :3000/ server.jsUse dark colors for code blocks res.redirect("/"); }); app.get("/", (req, res) => { //Redirect to homepage. if (req.session.arcgis) { res.send(` <h1>Hi ${req.session.arcgis.username}<h1> <pre><code>${JSON.stringify(req.session.arcgis, null, 2)}</code></pre> <a href="/sign-out">Sign Out<a> `); } else { res.send(`<a href="/sign-in">Sign In<a>`); } }); app.listen(3000, () => { console.log(`Visit http://localhost:3000/ to get started!`); });
When you click Sign in, you will be redirected back to your server. The session information including the client
, the refresh
, and your username
will populate the page:
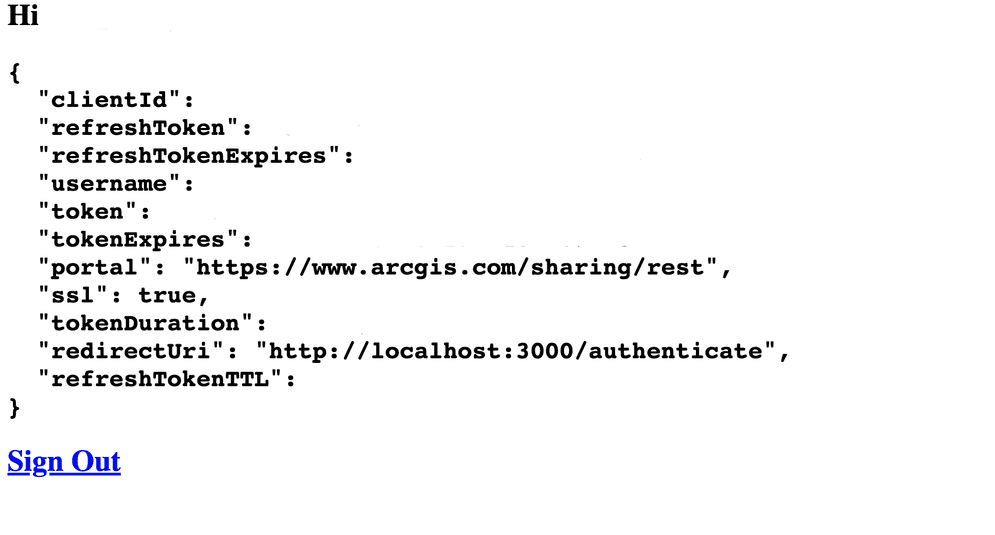
What's next?
Learn how to use additional ArcGIS location services in these tutorials: