What is Jimu UI?
The Jimu UI component library is part of ArcGIS Experience Builder that provides a set of reusable UI components for building custom widgets and experiences. It is created to contain all UI components that experiences will use. The library is built on top of technologies like React, reactstrap, and emotion-js.
Jimu UI library is organized into three main categories:
- General UI components such as buttons and icons.
- Focused components such as ColorPicker, Slider, and Switch.
- Advanced components such as FeatureLayerPicker, FieldSelector, and Filter.
You use Jimu UI components to build:
- Widgets with consistent look and feel across Experience Builder applications.
- Custom widgets without re-creating common UI elements.
- Custom widgets that require a specific theming and styling customization to match specific UI requirements.
How to use Jimu UI components in a custom widget
The general steps to use Calcite components in a custom widget are:
1. Set up your environment
You need to install ArcGIS Experience Builder Developer Edition and the necessary components.
- Download and install the current LTS version of Node.js.
- Download and install ArcGIS Experience Builder Developer Edition.
2. Create custom widget
Next, create a custom widget and set up your development environment.
- Open your preferred Integrated Development Environment (IDE), such as Visual Studio Code, and navigate to the
client
directory in the Experience Builder project file structure. - Create a new directory for your custom widget inside the
client/your-extensions/widgets
directory. - Follow the directory structure outlined in Widget implementation.
3. Add Jimu UI components
You import Jimu UI components in your custom widget by adding the following import statements at the beginning of your widget's code:
import { Button, Icon, ColorPicker } from 'jimu-ui'
After importing the Jimu UI components, you can use them in your widget's render method. For example, you can use a button
component in your widget like this:
<Button type="primary">Primary Button</Button>
To use a Color
component in your widget, you can import the component:
import { ColorPicker } from 'jimu-ui/color-picker'
Then use the Color
component in your widget's render method:
<ColorPicker></ColorPicker>
Example
The following example demonstrates how to use a Button
and Color
component in your custom widget:
import { JimuMapViewComponent, JimuMapView } from 'jimu-arcgis'
import { Button, ColorPicker } from 'jimu-ui'
export default class MyWidget extends React.PureComponent {
render() {
return (
<div>
<Button type="primary">Primary Button</Button>
<ColorPicker></ColorPicker>
</div>
);
}
}
Best practices
Consider the following key points when using Jimu UI components in your custom widget:
-
Import components correctly and always import the required Jimu UI components at the top of your widget file.
Use dark colors for code blocks Copy import { Card, CardBody, Button } from 'jimu-ui'
-
Make sure to include the JSX pragma at the top of your file to enable JSX syntax.
Use dark colors for code blocks Copy // widget.tsx: /** @jsx jsx */ // <-- make sure to include the jsx pragma import { React, AllWidgetProps, jsx } from 'jimu-core'
-
Jimu UI component library includes CSS utility classes that are consistent with those provided by Bootstrap. These utility classes allow you to apply styles to DOM elements through the
class
attribute. They simplify the process of styling by offering predefined, reusable styles for common design needs, such as spacing, typography, and alignment.Names -
You have three options to style your custom widget: Inline CSS, External CSS stylesheets, and CSS-in-JS. CSS-in-JS is the recommended method for styling custom widgets due to these advantages:
-
Scoped styles ensures styles are scoped to specific components. This avoids issues like global CSS conflicts or unintentional overrides.
-
Dynamic styling which allows you to conditionally apply styles based on a component's state or properties. For example:
Use dark colors for code blocks Copy const dynamicStyle = (isActive) => css` background-color: ${isActive ? 'green' : 'red'}; color: white; padding: 10px; `; function MyWidget({ isActive }) { return <div css={dynamicStyle(isActive)}>This widget is {isActive ? 'Active' : 'Inactive'}</div>; }
-
Code maintainability and readability by keeping styles close to the component they are applied to. This makes it easier to understand and update styles when needed.
-
-
Use the Jimu UI Storybook to explore available components, their usage, and API documentation. The Storybook provides a live playground to test components and see how they look and behave in different scenarios.
Tutorials

Create a starter widget
Learn how to build the base implementation for a widget.
ArcGIS Online
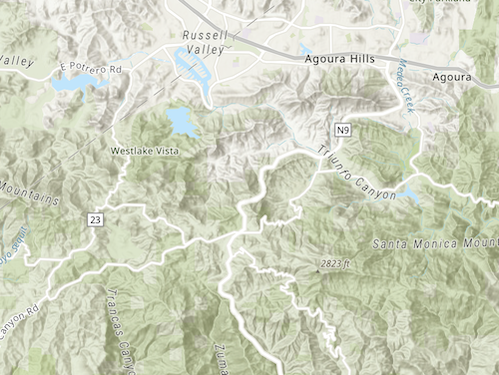
Get map coordinates
Learn how to display the latitude and longitude, scale, and zoom level of the map in a custom widget..
ArcGIS Online
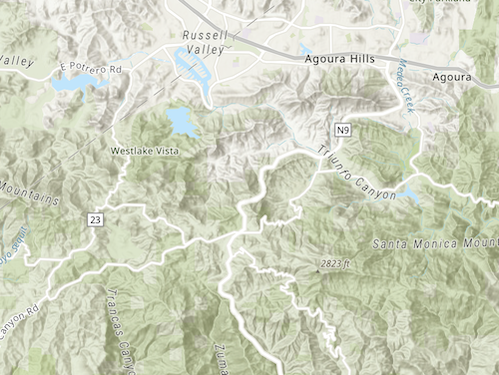
Add layers to a map
Learn how to add layers to a map from a custom widget.
ArcGIS Online
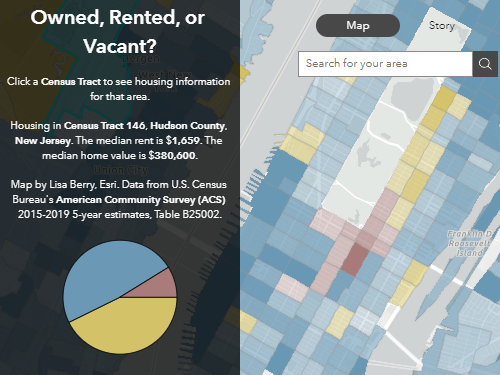
Get started with ArcGIS Experience Builder
Build an interactive web app about housing in America.
ArcGIS Online
Tools
Use tools to access your ArcGIS portal and create and manage content for your low-code applications.