1. Select an operation
The first step is to determine which request to use from the elevation service. The service supports two requests: /at-point
to find the elevation of a single point or /at-many-points
to find the elevation of multiple points.
GET https://elevation-api.arcgis.com/arcgis/rest/services/elevation-service/v1/elevation/at-point
2. Define input parameters
The next step is to provide input parameters for the operation. For the multiple points operation, you are required to provide a request body. Both single point and multiple points operations require latitude and longitude coordinates of the location(s) of interest. You can also specify the datum for the elevation values, such as above mean sea level or ground level. The most common parameters are listed in the table below.
The following parameters are used to get an elevation value of a single coordinate:
Name | Description | Examples |
---|---|---|
lon | The longitude of the specified point. | lon=-157.7079 |
lat | The latitude of the specified point. | lat=21.4040 |
relative | The datum from which to measure elevation. The supported datums are above mean sea level or above ground level. | relative (default value), relative |
3. Make a request
The final step is to make a request to the elevation service using an ArcGIS Maps SDK, scripting API, or open source library. In general, you:
- Reference the service endpoint.
- Set the coordinates.
- Set your access token.
APIs
async function getElevationData(lon, lat) {
const url =
"https://elevation-api.arcgis.com/arcgis/rest/services/elevation-service/v1/elevation/at-point";
const response = await esriRequest(url, {
query: {
lon: lon,
lat: lat,
},
responseType: "json",
});
const { x, y, z } = response.data.result.point;
arcgisMap.popup = {
visibleElements: {
collapseButton: false,
closeButton: false,
actionBar: false,
},
};
const title = `Elevation relative to mean sea level`;
const content = `
Latitude: ${y.toFixed(5)}<br>
Longitude: ${x.toFixed(5)}<br>
Elevation: ${z} m`;
addPointToMap(lon, lat);
arcgisMap.openPopup({
location: [lon, lat],
content: content,
title: title,
});
}
REST API
# You can also use wget
curl -X GET https://elevation-api.arcgis.com/arcgis/rest/services/elevation-service/v1/elevation/at-point?lon=-179.99&lat=-85.05 \
-d "f=json" \
-d "token=<YOUR_ACCESS_TOKEN>"
Additional resources
Tutorials
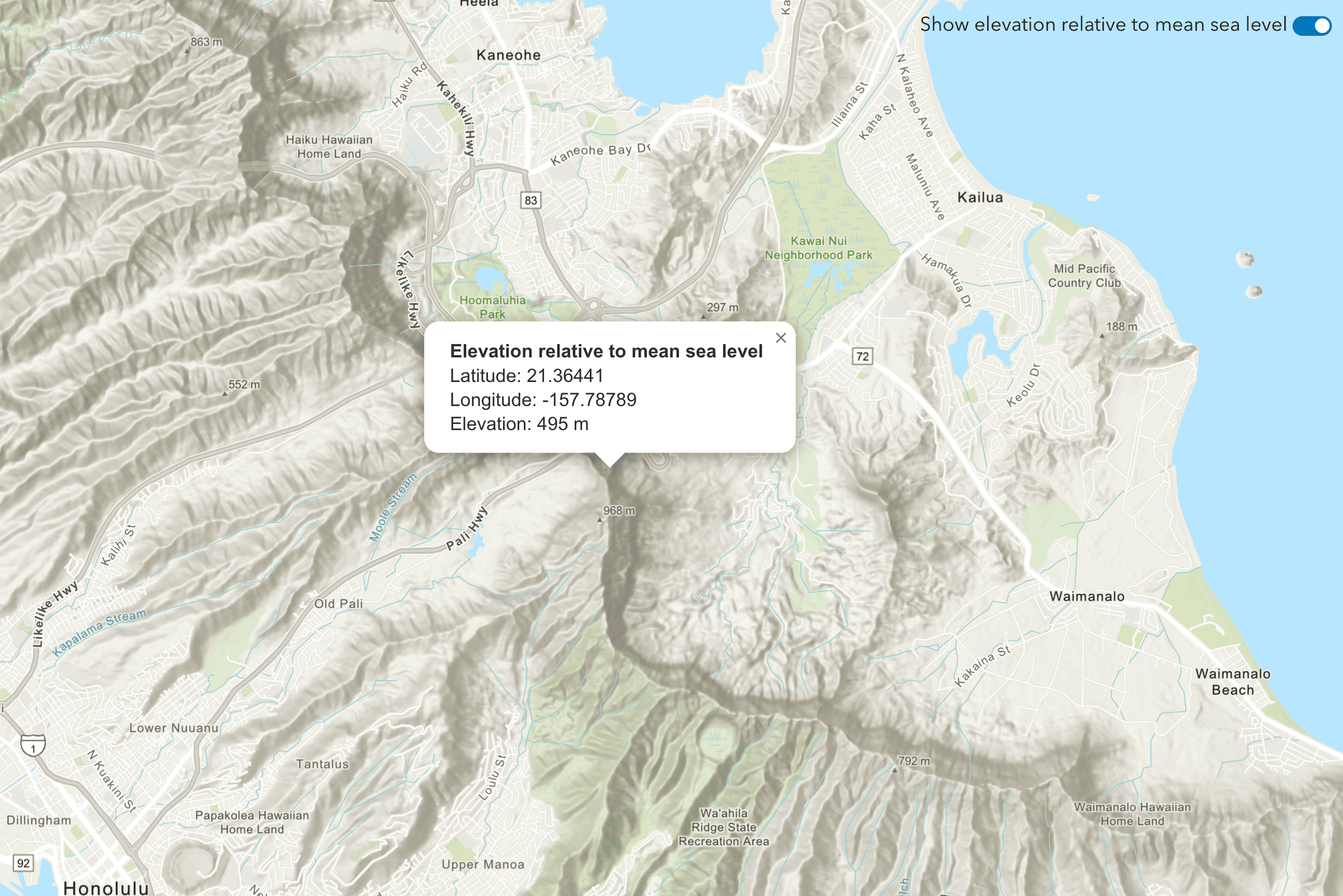
Find the elevation of a point
Find elevation value of a single location on land or water.
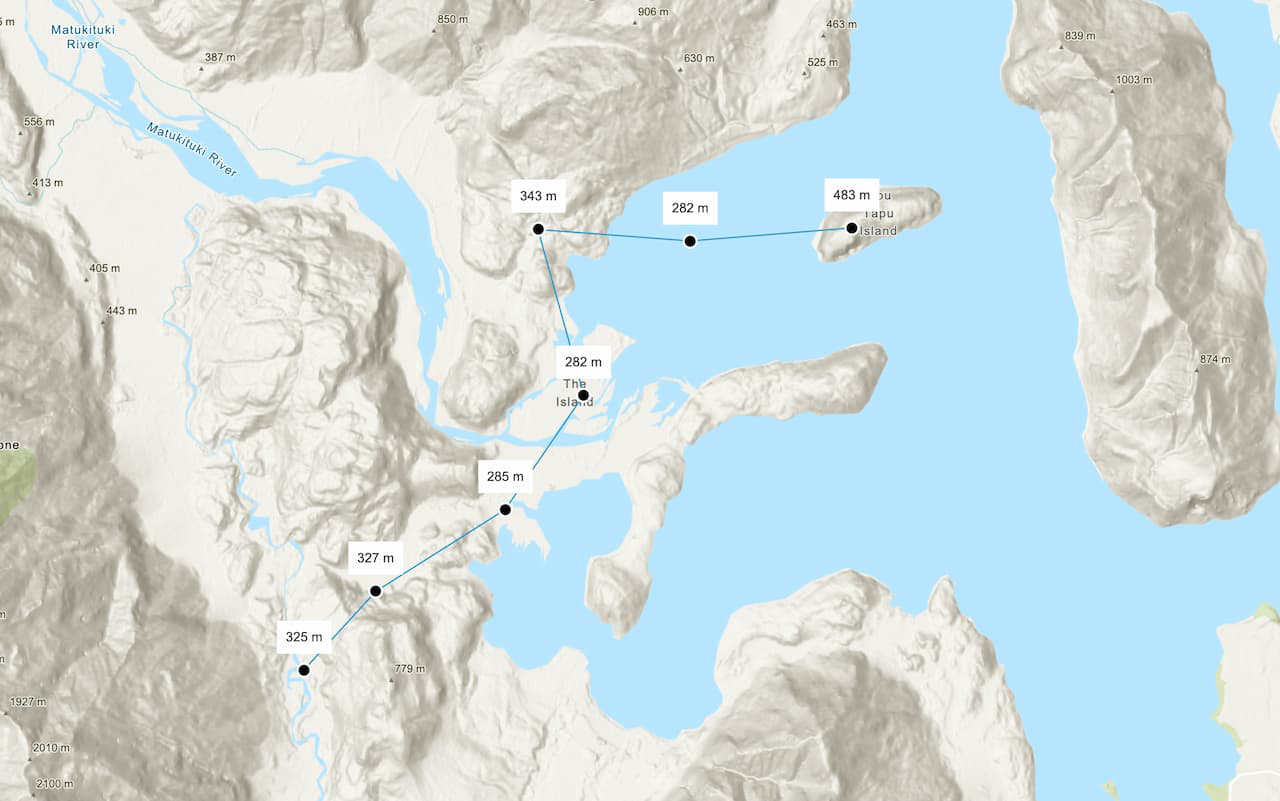
Find the elevations of multiple points
Find elevation values of multiple locations on land or water.