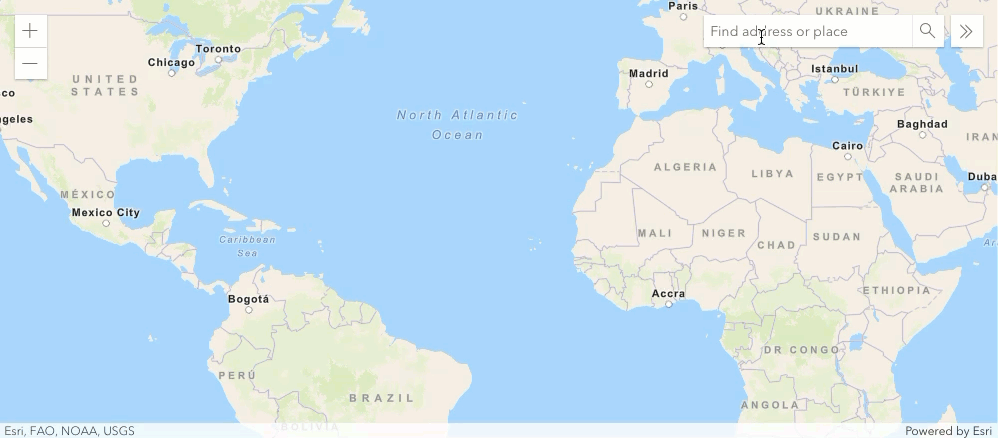
Suggestions displayed as characters using a widget and the geocoding service
What is autosuggest?
Autosuggest, also known as autocomplete, is the process of using incomplete text to search for place names and addresses. For example, you can search for "New Y", "Dodgers S", and "Cali" to get a list of suggested place names that match.
You can use autosuggest to build applications that:
- Autocomplete addresses and places as you type.
- Provide a list of suggestions for incomplete address and place names.
- Refine place names and addresses to improve geocoding accuracy.
How autosuggest works
You can get address and place name suggestions by making an HTTP request to the geocoding service suggest
operation or by using client APIs. Specify the address or place name text, and optionally, additional parameters to refine the search.
You can provide text for a partial place name or a partial address to get search results. Typically 2-3 characters are needed to start searching.
To refine the search and improve accuracy, you can provide additional information such as the location, search extent, category, and country code.
The geocoding service parses the text and uses all of the parameters provided to return a set of suggestions. Each suggestion contains text
(address or place name), i
(single distinct place or multiple with the same name or category), and magic
(search identifier) properties.
URL request
https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/suggest?text={partialText}&f=json&token=<ACCESS_TOKEN>
https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer/suggest?text={partialText}&f=json&token=<ACCESS_TOKEN>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An API key or OAuth 2.0 access token. | token=< |
Key parameters
Name | Description | Examples |
---|---|---|
text | The partial address or place name. Different formats are supported. | text=starbutext=100, main sttext=New Y |
Additional parameters: Refine the search by using parameters such as location
, search
, category
, and country
.
Code examples
Get suggestions for a string
This example finds suggestions for the string New Y
. To find the actual address and location for a suggestion, you have to pass each text
and magic
value to the geocoding service. Most APIs provide a LocatorTask to access the service.
Steps
-
Reference the geocoding service.
-
Set the text to search for.
-
Set the API key.
The response is a set of suggestions (max of 5) containing the text
and magic
properties. Use these properties to search for the complete address with a location.
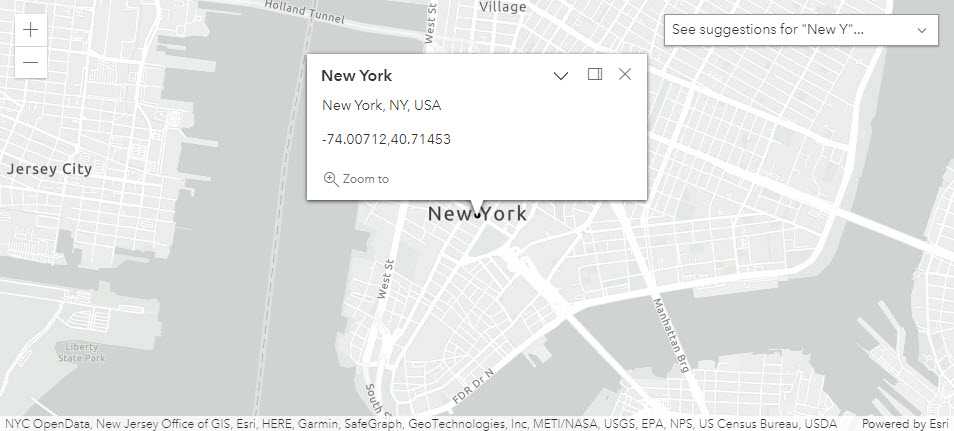
Find suggestions for the string New Y
.
APIs
geocodingServiceUrl = "https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer";
const params = {
text: "New Y", // Suggestion text
};
locator.suggestLocations(geocodingServiceUrl, params).then((response) => {
// Show a list of the suggestions
response.forEach((suggestion) => {
showSuggestion(suggestion.text, suggestion.magicKey);
});
// When a suggestion is selected, geocode to find its location
select.addEventListener("calciteSelectChange", (event) => {
const text = event.target.innerHTML;
const magicKey = event.target.value;
geocodeSuggestion(text, magicKey);
});
});
REST API
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/suggest \
-d 'f=pjson' \
-d 'text=New Y' \
-d 'token=<ACCESS_TOKEN>'
Response (JSON)
{
"suggestions": [
{
"text": "New York, NY, USA",
"magicKey": "dHA9MCNsb2M9NzUwOTc4OCNsbmc9MzMjcGw9MjcxNjU1MyNsYnM9MTQ6MjM4MTIyMzc=",
"isCollection": false
},
{
"text": "New York, USA",
"magicKey": "dHA9MCNsb2M9NzMxOTEwOCNsbmc9MzMjcGw9MjU5OTMyMyNsYnM9MTQ6MjM4MTIyMzc=",
"isCollection": false
},
{
Tutorials
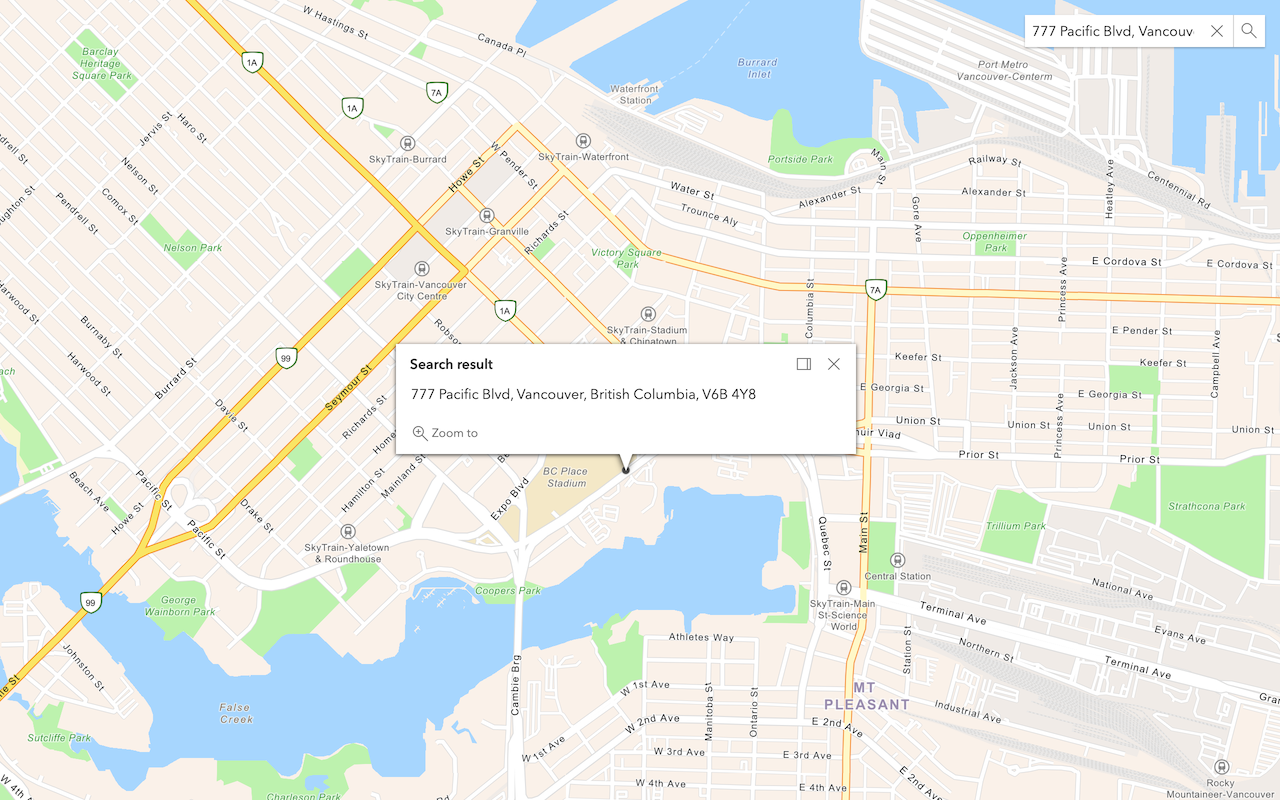
Search for an address
Convert an address or place to a location with the geocoding service.