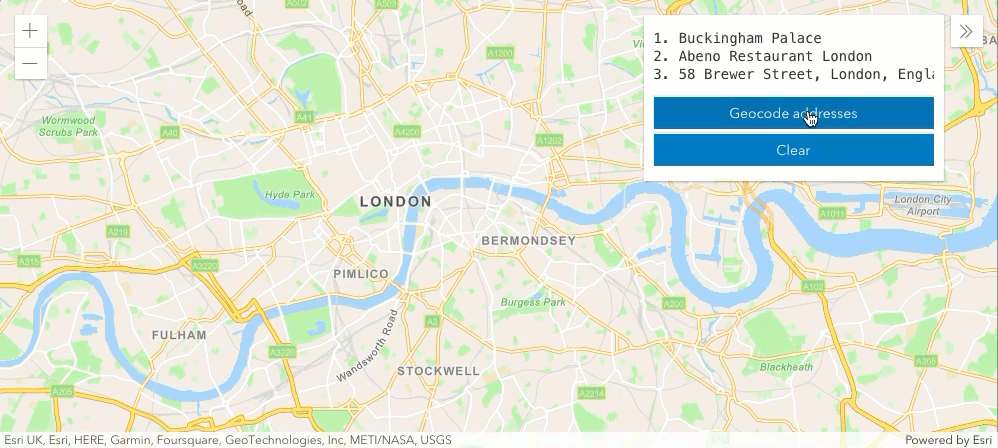
Three text strings geocoded in one transaction with the geocoding service
What is batch geocoding?
Batch geocoding, also known as bulk geocoding, is the process of converting a list of addresses or place names to a set of complete addresses with locations. The list of addresses can be formatted as a JSON structure or provided in a CSV file.
Batch geocoding is commonly used to:
- Convert a number of addresses to complete addresses.
- Find the locations for a list of addresses.
- Perform large batches of geocoding.
- Geocode addresses that can be saved for future use.
How batch geocoding works
The geocoding service parses each address and using the parameters you provide, returns a set of geocoded address locations. Each returned location contains a full address, x and y coordinates, attributes, and a score of how well it matched.
There are two ways to batch geocode addresses:
- Provide addresses as JSON input and use
geocode
.Addresses - Provide address data as file input and use
batch
.Geocode
JSON input
To geocode a list of addresses you can use the geocode
direct request. The input is a list of addresses as a JSON collection, and optionally, additional parameters to refine the output. The output is returned as a list of records with addresses.
To keep track of each input and output address, the input values should contain an objectid field to connect the input data record to the output address candidate returned (see ResultID).
JSON format
The JSON format requires a record with attributes for each address string to geocode:
{
"records": [
{
"attributes": {
"objectid": 1,
"address": "Buckingham Palace"
}
...
}
To refine the geocoded address output, provide additional parameters such as the search extent, location type, category, and source country.
File input
To geocode a file of addresses, use the batch
job request. The input can be either a CSV (.csv) file or a zipped CSV (.zip) file, along with a case-sensitive key-value list that maps the fields in your file to those required by the service. The output is returned as a file of addresses with locations, along with additional informational fields describing the geocoding results (in CSV format).
This method requires a job request with the following steps:
- Add the file of addresses to your portal.
- Submit the job request to the service.
- Monitor the status of your request.
- Access the results file URL.
- Download the result file.
File Format
The format of the input CSV file can contain address data in multiple columns or in a single column.
Address data in multiple columns:
Address,City,State,Postal
11270 SW CINDY ST,Portland,OR,97008
16360 SW ESTUARY DR,Portland,OR,97006
13895 SW MERIDIAN ST,Portland,OR,97005
...
Address data in a single column:
Addresses
"380 New York St, Redlands, CA"
"1 World Way, Los Angeles, CA"
"1200 Getty Center Drive, Los Angeles, CA
...
URL requests
Direct (geocode Addresses
)
Use the geocode
operation to batch geocode a list of addresses.
https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer/geocodeAddresses?<parameters>
Learn more about standard and enhanced endpoints in Service endpoints.
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An access token (API key or OAuth 2.0) with the required premium privilege. Go to Security and authentication to generate an access token. | token= |
Key parameters
Name | Description | Examples |
---|---|---|
addresses | A JSON object with an address or place name. Different address formats are supported. | See example |
Additional parameters: Refine the search results by using parameters such as search
, location
, category
, country
. Use lang
to return results in a specific language.
Job (batch Geocode
)
Use the batch
operation to geocode a file with a large number of addresses. The file needs to be uploaded with the add
operation before submitting the job. To see all of the steps required for the job request, go to Geocode a file of addresses (code example).
https://geocode-beta.arcgis.com/arcgis/rest/services/World/GeocodeServer/batchGeocode/beta/submitJob
Required parameters
Name | Description | Examples |
---|---|---|
token | An access token (API key or OAuth 2.0) with the required premium privilege. Go to Security and authentication to generate an access token. | token={ |
item | The item ID returned in the response from the add request. | item={"item |
field | A list of key strings mapping the fields in your address file to the input fields expected by the service. | field |
Code examples
Direct: Geocode a set of addresses
Use the geocode
direct request to find the locations for a list addresses.
Steps
- Reference the geocoding service.
- Set the addresses to be geocoded. All input addresses should include an
objectid
field. - Set the
token
parameter to your API key.
The response contains a set of geocoded addresses. The input objectid
matches the output Result
for each record. Addr
represents whether the input address resolved to a standard address or POI. score
represents how well it matched.
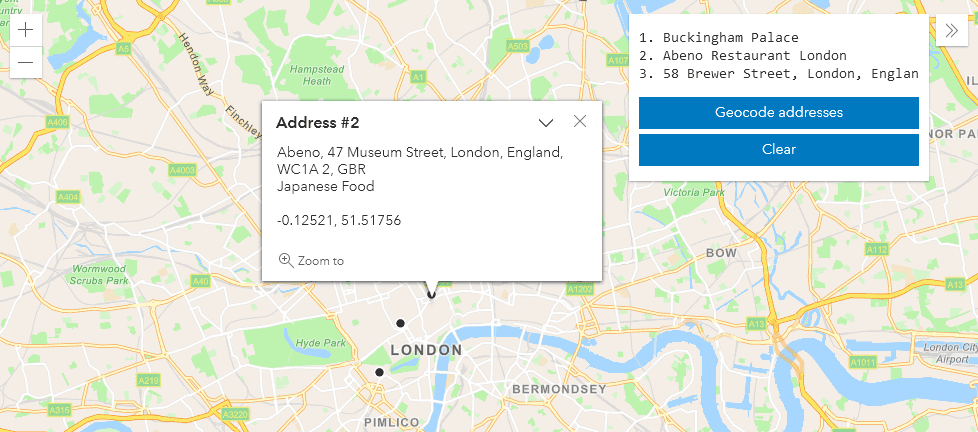
Use the geocode
operation to find the location of many addresses.
APIs
const geocodingServiceUrl =
"http://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer"
const params = {
addresses: [
{
objectid: 1,
address: "Buckingham Palace",
},
{
objectid: 2,
address: "Abeno Restaurant London",
},
{
objectid: 3,
address: "58 Brewer Street, London, England",
},
],
}
locator.addressesToLocations(geocodingServiceUrl, params).then(
results => {
if (results.length) {
results.forEach(result => {
addGraphic(result)
})
}
},
function(error) {
console.log(error)
}
)
REST API
curl https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer/geocodeAddresses \
-d 'f=pjson' \
-d 'addresses=
{
"records": [
{
"attributes": {
"objectid": 1,
"address": "Buckingham Palace"
}
},
{
"attributes": {
"objectid": 2,
"address": "Bernardis Restaurant London"
}
},
{
"attributes": {
"objectid": 3,
"address": "58 Brewer Street, London, England"
}
}
]
}' \
-d 'token=<ACCESS_TOKEN>'
Job: Geocode a file of addresses
Use the batch
job request to find the locations for a file of addresses.
Steps
- Upload a CSV(.csv) or zipped CSV (.zip) to the geocoding service.
- Submit the job request.
- Check the job status.
- Access the URL to the result file.
- Download the result file.
Input file (.csv)
Address | City | State | Postal |
---|---|---|---|
11270 SW CINDY ST | Portland | OR | 97008 |
16360 SW ESTUARY DR | Portland | OR | 97006 |
13895 SW MERIDIAN ST | Portland | OR | 97005 |
... |
Result output file (.csv)
ShapeX | ShapeY | Loc_name | Status | Score | Match_addr | LongLabel | ShortLabel | Addr_type | Place_addr | Rank | AddNum | StPreDir | StName | StType | StAddr | City | Subregion | Region | RegionAbbr | Postal | PostalExt | Country | CntryName | LangCode | Distance | X | Y | DisplayX | DisplayY | Xmin | Xmax | Ymin | Ymax | Address | City | State | Postal |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
-122.793334 | 45.465578 | World | M | 99.081803 | 11270 SW Cindy St, Beaverton, Oregon, 97008 | 11270 SW Cindy St, Beaverton, OR, 97008, USA | 11270 SW Cindy St | PointAddress | 11270 SW Cindy St, Beaverton, Oregon, 97008 | 20.000000 | 11270 | SW | Cindy | St | 11270 SW Cindy St | Beaverton | Washington County | Oregon | OR | 97008 | 5885 | USA | United States | ENG | 0.000000 | -122.793333 | 45.465758 | -122.793334 | 45.465578 | -122.794334 | -122.792334 | 45.464578 | 45.466578 | 11270 SW CINDY ST | Portland | OR | 97008 |
-122.843902 | 45.514404 | World | M | 99.081803 | 16360 SW Estuary Dr, Beaverton, Oregon, 97006 | 16360 SW Estuary Dr, Beaverton, OR, 97006, USA | 16360 SW Estuary Dr | PointAddress | 16360 SW Estuary Dr, Beaverton, Oregon, 97006 | 20.000000 | 16360 | SW | Estuary | Dr | 16360 SW Estuary Dr | Beaverton | Washington County | Oregon | OR | 97006 | 7929 | USA | United States | ENG | 0.000000 | -122.843953 | 45.513692 | -122.843902 | 45.514404 | -122.844902 | -122.842902 | 45.513404 | 45.515404 | 16360 SW ESTUARY DR | Portland | OR | 97006 |
-122.822046 | 45.502979 | World | M | 99.081803 | 13895 SW Meridian St, Beaverton, Oregon, 97005 | 13895 SW Meridian St, Beaverton, OR, 97005, USA | 13895 SW Meridian St | PointAddress | 13895 SW Meridian St, Beaverton, Oregon, 97005 | 20.000000 | 13895 | SW | Meridian | St | 13895 SW Meridian St | Beaverton | Washington County | Oregon | OR | 97005 | 2476 | USA | United States | ENG | 0.000000 | -122.822743 | 45.503274 | -122.822046 | 45.502979 | -122.823046 | -122.821046 | 45.501979 | 45.503979 | 13895 SW MERIDIAN ST | Portland | OR | 97005 |
... |
APIs
submit_url = BATCH_URL + "/submitJob"
params = {
"fieldMapping": FIELD_MAPPING,
"item": {"itemID": item.id},
"token": token,
}
if not use_get:
response = requests.post(submit_url, json=params)
else:
response = requests.get(submit_url, params=params)
json_response = response.json()
if json_response.get("error") is not None:
print(json_response)
sys.exit()
job_id = json_response["jobId"]
print("Job submitted for geocoding")
REST API
Request
POST /sharing/rest/content/users/{USER_NAME}/addItem HTTP/1.1
Host: www.arcgis.com
Content-Length: 1214
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="f"
json
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="token"
{ACCESS_TOKEN}
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="file"; filename="addresses.csv"
Content-Type: text/csv
(data)
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="title"
MyAddressFile
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="type"
CSV
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="typeKeywords"
CSV, Text, Data
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="description"
This is a csv file uploaded programmatically for use with the batchGeocode(beta) service
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="tags"
postman, batchGeocode, csv, addresses
------WebKitFormBoundary7MA4YWxkTrZu0gW--
Response (JSON)
{
"success": true,
"id": "{ITEM_ID}",
"folder": ""
}