What is place geocoding?
Place geocoding is the process of searching for addresses for businesses, administrative locations, and geographic features. You can search for the name of a place and/or your can provide categories to filter the results. For example, you can use the text Mexican
and the Food
category to search for restaurant addresses.
You can use place search to:
- Search for place addresses around the world (no search location provided).
- Search for place addresses near or within a distance of a point.
- Search for places by category such as restaurants, gas stations, or schools.
- Find and display places on a map.
How place geocoding works
You can search for a place by making an HTTPS request to the geocoding service find
operation or by using client APIs. Specify the place name, output data fields, and optionally, additional parameters to refine the search.
The more complete you can make the input place name, the more likely the geocoding service will find an exact match. For example, "Grand Canyon", "Disneyland in California", or "Starbucks at Newport Beach".
To refine the search or search for POI, you can specify a category such as coffee shop, restaurant, or gas station. See more categories in Category filtering. To refine it further, specify parameters such as the search extent, country code, and city.
The geocoding service parses the place name and uses the all of the parameters to return a set of place candidates. Most candidates contain a place name, full address, location, attributes, and a score of how well it matched.
Types of place search
There are two types of searches you can perform: Local and Global. A local search uses a location to search for places nearby or a search extent to confine the search area. A global search is an open search that typically isn't confined to an extent.
URL request
https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates?SingleLine={placeName}&location={longitude,latitude}&category={category}f=json&token=<ACCESS_TOKEN>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An API key or OAuth 2.0 access token. | token= |
Key parameters
Name | Description | Example |
---|---|---|
address | The address or place name string. Different formats are supported. | address=Disneyland address=Los Angeles Starbucks address=Seattle, Washington address=Mount Everest address=-117.155579,32.703761 |
location | Use to focus the search on a specific location. | location=-117.196,34.056 |
category | Filter places by place type values. | category=food,mexican food category=coffee shop category=gas station |
out | A list of data fields to return. | outFields=PlaceName,Place_addr, outFields=* (return all fields) |
Additional parameters: Refine the search by using parameters such as search
, neighborhood
, city
, and country
. Use lang
to return results in a specific language.
Code examples
Local search (by name)
This example uses the geocoding service to search for Starbucks
near a location in San Francisco. You can use this type of search to find the location of businesses, points of interest, or landmarks. Most APIs provide a LocatorTask to access the service.
Steps
-
Reference the geocoding service.
-
Set the place name to search for.
-
Set the location to search from.
-
Set the list of data fields to return. e.g. PlaceName,Place_addr
-
Set the API key.
The response is a set of place candidates with a place name, full address, location, score, and attributes.
APIs
function findPlaces(pt) {
const geocodingServiceUrl = "http://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer";
const params = {
address: {
address: "Starbucks"
},
location: pt, // San Francisco (-122.4194, 37.7749)
outFields: ["PlaceName","Place_addr"]
}
locator.addressToLocations(geocodingServiceUrl, params).then((results)=> {
showResults(results);
});
REST API
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates \
-d 'f=pjson' \
-d 'address=Starbucks' \
-d 'location=-122.4194,37.7749' \
-d 'outfields=PlaceName,Place_addr' \
-d 'token=YOUR_API_KEY'
Local search (by category)
This example searches for gas stations near a location by setting the Gas Station
category near a location in Paris.
To see a full list of categories, visit Category filtering.
Steps
-
Reference the geocoding service.
-
Set location to search from.
-
Set the category of places to search for.
-
Set the list of data fields to return. e.g. PlaceName,Place_addr
-
Set the API key.
The response is a set of place candidates with a place name, full address, location, score, and attributes.
APIs
function findPlaces(pt) {
const geocodingServiceUrl = "http://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer";
const params = {
categories: ["gas station"],
location: pt, // Paris (2.34602,48.85880)
outFields: ["PlaceName","Place_addr"]
}
locator.addressToLocations(geocodingServiceUrl, params).then((results)=> {
showResults(results);
});
REST API
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates \
-d 'f=pjson' \
-d 'category=gas station' \
-d 'location=2.34602,48.85880' \
-d 'outfields=PlaceName,Place_addr' \
-d 'token=<ACCESS_TOKEN>'
Global search
This example executes a global search for Grand Canyon
. No location or extent parameters are provided.
Steps
-
Reference the geocoding service.
-
Set the place name or address to search.
-
Set the list of data fields to return.
-
Set the API key.
The response is a set of candidates with an address, location, score, and many other attributes.
APIs
function findPlaces() {
const geocodingServiceUrl = "http://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer";
const params = {
address: {
address: "Grand Canyon"
},
outFields: "*"
}
locator.addressToLocations(geocodingServiceUrl,params).then((results)=> {
showResults(results);
});
REST API
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates \
-d 'f=pjson' \
-d 'address=grand canyon' \
-d 'token=<ACCESS_TOKEN>'
Tutorials
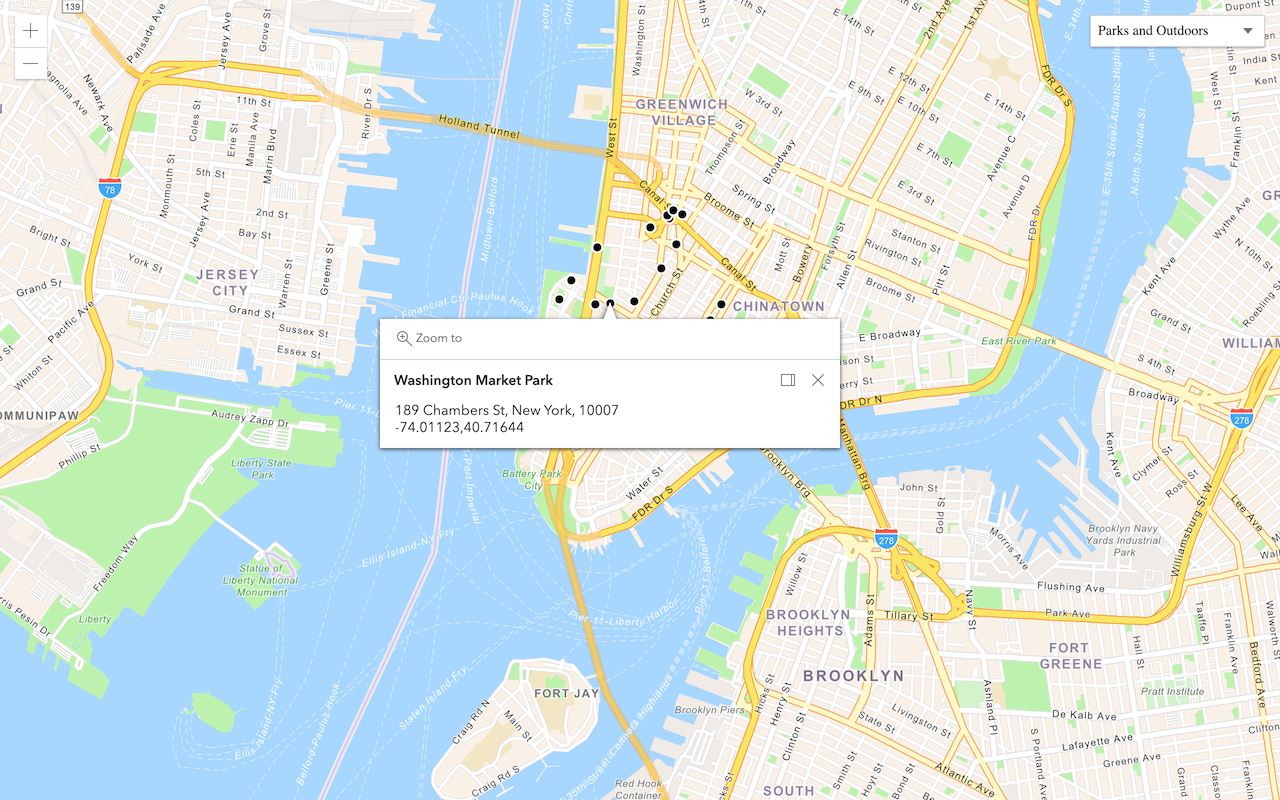
Find place addresses
Find an address, business, or place with the geocoding service.