What is a bounding box search?
A bounding box search finds places within an extent. An extent typically represents the visible area of a map.
You can use this search to:
- Find and display places within a current map extent.
- Get the latitude and longitude for places.
- Filter places using categories and/or text.
- Find place attributes including
place
,I d name
,categories
, andlocation
. - Find place IDs so you can request additional place details.
How a bounding box search works
To perform a bounding box search, you use the places service /places/within-extent
request and set the xmin
, ymin
, xmax
, and ymax
coordinates. The maximum width and height of the search extent is 20,000 meters.
The general steps are:
- Get the places service URL.
- Set the parameters:
xmin
,xmax
,ymin
,ymax
category
,Ids search
Text page
Size
- Execute the request.
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?f=json&xmin=-0.01399040222167969&ymin=51.504148054725356&xmax=0.014548301696777345&ymax=51.51579343362533&categoryIds=11000&pageSize=20&token=<ACCESS_TOKEN>
If the number of places in the search area is greater than the page
, you can start paging.
The steps to start paging are:
- Execute the initial search request (see above).
- Use the response to check
response.links
. If the value is valid, you can page. - Build the next search request:
- Use
response.links.base
andresponse.links.next
to create the URL. - The
offset
parameter is automatically added and incremented for you. - Add
token=<
to provide authentication.ACCESS_ TOKEN>
- Use
- Execute the next search request.
- Repeat the steps until
response.links
isnull
. This indicates no additional results are available.
You can use paging to request up to 200 places.
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?f=json&xmin=-0.01399040222167969&ymin=51.504148054725356&xmax=0.014548301696777345&ymax=51.51579343362533&categoryIds=11000&pageSize=20&offset=20&token=<ACCESS_TOKEN>
URL request
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/within-extent?xmin=<LONGITUDE>&ymin=<LATITUDE>&xmax=<LONGITUDE>&ymax=<LATITUDE>&token=<ACCESS_TOKEN>&f=pjson
Parameters
Name | In | Type | Required | Default value | Description |
---|---|---|---|---|---|
query |
| The minimum x coordinate, or longitude, of the search extent. This is the furthest west that will be searched. | |||
query |
| The minimum y coordinate, or latitude, of the search extent. This is the furthest south that will be searched. | |||
query |
| The maximum x coordinate, or longitude, of the search extent. This is the furthest east that will be searched. | |||
query |
| The maximum y coordinate, or latitude, of the search extent. This is the furthest north that will be searched. | |||
query |
|
| Filters places to those that match the category Ids. Places will be returned if they match any of the category Ids. If this property is not set, places will be returned regardless of their category. You can obtain information on category Ids from the
You can search up to a maximum of | ||
query |
|
| Free search text for places against names, categories etc. | ||
query |
| none | Determines whether icons are returned and the type of icon to use with a place or category. Use this parameter to define the type of icon URL for a given place or category. Place icons are available in the following formats:
The SVG and CIM symbols default to 15 x 15 pixels but can be scaled smoothly for display in larger UI elements or to emphasize these features on a map. The PNG icons are provided as 48 x 48 pixels but for map display the recommended size is 16 x 16 pixels. The default is | ||
query |
| 10 | The number of places that should be sent in the response for a single request. You can set this to any value up to If the query results in more than this page size, then the response
object will contain a Regardless of paging, the maximum number of places that can be returned
in total is The default | ||
query |
|
| Request results starting from the given offset. This parameter works with the Regardless of paging, the maximum number of places that can be returned
in total is | ||
query |
| json | The requested response format - either | ||
query |
|
| The authentication token, created from an ArcGIS Location Platform account, with the The Alternatively, you can supply a token in the request header with one of the following keys using the "Bearer" scheme:
|
Code examples
Find places by category
In this example, you use specific categories to find places within a map extent for Helsinki. Moving the map will execute a search for the new extent.
The categories are:
- Museum (16027)
- Park (16032)
- Monument (16026)
- Dog parks (16033)
- Playgrounds (16037)
Steps
- Reference the places service.
- Set the min and max x and y values for the extent and the
category
.Ids - Set the token parameter to your access token.
APIs
findPlacesWithinExtent({
xmin: 24.897,
ymin: 60.159,
xmax: 24.979,
ymax: 60.178,
categoryIds: ["16026", "10027", "16032", "16033", "16037"],
authentication,
}).then(response => {
console.log(JSON.stringify(response.results, null, 2));
});
REST API
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1//within-extent' \
-d 'xmin=24.897' \
-d 'ymin=60.159' \
-d 'xmax=24.979' \
-d 'ymax=60.178' \
-d 'categoryIds=["16026", "10027", "16032", "16033", "16037"]' \
-d 'token=<ACCESS_TOKEN>' \
-d 'f=pjson'
{
"results": [
{
"placeId": "6dcabdbdee17e4d57d64e50fd2bf5b67",
"location": {
"x": 24.92331,
"y": 60.176246
},
"categories": [
{
"categoryId": "10028",
"label": "Art Museum"
}
],
"name": "Reitzin säätiön kokoelmat"
},
{
"placeId": "04db7e0fa56592edcb70a88a0c480fab",
"location": {
"x": 24.914545,
"y": 60.159993
},
"categories": [
{
"categoryId": "16032",
"label": "Park"
}
],
"name": "Selkämerenpuisto"
},
// more results...
],
"pagination": {
"nextUrl": "https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?xmin=24.89700000&ymin=60.15900000&xmax=24.97900000&ymax=60.17800000&categoryIds=16026,10027,16032&f=pjson&offset=20&pageSize=20"
}
}
Find places by category and text
In this example, you use the category
and search
parameters to find places that are within the map extent for area in London. Moving the map will execute a search for the new extent.
This example uses the restaurants (13065) category to find and filter places.
Steps
- Reference the places service.
- Define the extent for the search and set the
category
andIds search
parameters with the keywords.Text - Set the token parameter to your access token.
APIs
findPlacesWithinExtent({
xmin: -0.107,
ymin: 51.513,
xmax: -0.148,
ymax: 51.502,
searchText: "sports",
categoryIds: ["13018", "13051", "18065"],
authentication,
}).then(response => {
console.log(JSON.stringify(response.results, null, 2));
});
REST API
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1//within-extent' \
-d 'xmin=-0.107' \
-d 'ymin=51.513' \
-d 'xmax=-0.148' \
-d 'ymax=51.502' \
-d 'searchText="sports"' \
-d 'categoryIds=["13018", "13051", "18065"]' \
-d 'token=<ACCESS_TOKEN>' \
-d 'f=pjson'
{
"results": [
{
"placeId": "22a25084338d14aa48e41a2387880c44",
"location": {
"x": -0.12833,
"y": 51.50711
},
"categories": [
{
"categoryId": "18065",
"label": "Sports Club"
}
],
"name": "News Sports"
},
{
"placeId": "c37f9d7946964078b530d2c7eeb64d2f",
"location": {
"x": -0.126172,
"y": 51.507999
},
"categories": [
{
"categoryId": "18065",
"label": "Sports Club"
}
],
"name": "Social Media"
},
// more results...
],
}
Page through results
This example searches for all places without using categories for an extent near Seattle. Moving the map will execute a search for the new extent.
If there are more results than the page
, you can page through them using the next
URL in the links
attribute. The maximum number of places that you can page through is 200
.
Steps
- Reference the places service.
- Define the extent for the search.
- Set the token parameter to your access token.
- Using ArcGIS REST JS, if
repsponse.next
is valid, use thePage next
to send the next request.Page()
APIs
const getPlaces = async () => {
let places = await arcgisRest.findPlacesWithinExtent({
xmin: -122.322,
ymin: 47.609,
xmax: -122.342,
ymax: 47.603,
authentication,
});
console.log(JSON.stringify(lastResponse.results, null, 2));
while (lastResponse.nextPage) {
try {
lastResponse = await lastResponse.nextPage();
console.log(JSON.stringify(lastResponse.results, null, 2));
} catch {
break;
}
}
};
getPlaces();
REST API
## initial request
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent' \
-d 'xmin=-122.322' \
-d 'ymin=47.609' \
-d 'xmax=-122.342' \
-d 'ymax=47.603' \
-d 'pageSize=20' \
-d 'token=<ACCESS_TOKEN>' \
-d 'f=pjson'
## next request
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent' \
-d 'xmin=-122.322' \
-d 'ymin=47.609' \
-d 'xmax=-122.342' \
-d 'ymax=47.603' \
-d 'offset=40' \
-d 'pageSize=20' \
-d 'token=<ACCESS_TOKEN>' \
-d 'f=pjson'
// initial request
{
"results":["<results array>"]
"pagination": {
"nextUrl": "https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?xmin=-122.32164860&ymin=47.60912875&xmax=-122.34233379&ymax=47.60286441&f=pjson&offset=20&pageSize=20"
}
}
// next request
{
"results":["<results array>"],
"links": {
"previousUrl": "https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?xmin=-122.32164860&ymin=47.60912875&xmax=-122.34233379&ymax=47.60286441&f=pjson&offset=0&pageSize=20",
"nextUrl": "https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/within-extent?xmin=-122.32164860&ymin=47.60912875&xmax=-122.34233379&ymax=47.60286441&f=pjson&offset=40&pageSize=20"
}
}
Tutorials
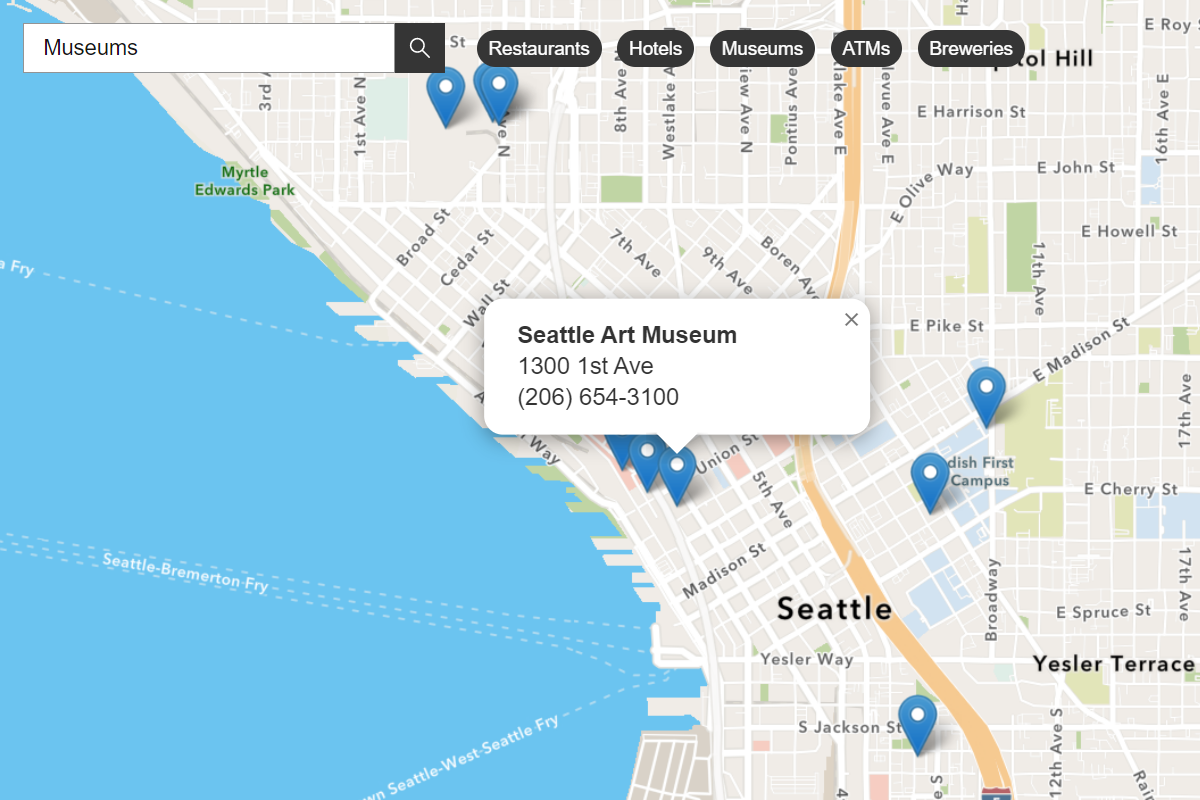
Find places in a bounding box
Perform a text-based search to find places within a bounding box.