1. Select a category
The first step is to identify one or more place categories for the types of places you are interested in. Categories are used to help filter search results so only the type of places you are interested in are returned. All categories have a name and a unique ID. To help find the appropriate category (and ID) you can use the /categories
request.
The steps to find a category are:
- Reference the places service.
- Define a filter category.
- Set the access token.
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/categories?filter=<CATEGORY>&token=<ACCESS_TOKEN>&f=pjson
APIs
import { ApiKeyManager } from "@esri/arcgis-rest-request";
import { getCategories,searchCategories,getCategory } from "@esri/arcgis-rest-places";
const apiKey = "YOUR_ACCESS_TOKEN";
const authentication = ApiKeyManager.fromKey(apiKey);
getCategories({
authentication
}).then((results)=>{
console.log(JSON.stringify(results,null,2));
});
REST API
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/categories?token=<ACCESS_TOKEN>&f=pjson'
2. Search for places
The next step is to perform a search with /near-point or /within-extent. To filter and return the best results, you should provide a list of categories and/or keywords when you search. When places are returned, they contain attributes such as name
, place
, location
, and categories
. You can use the place attributes to display them on a map or to get more details about each place.
You can perform the following types of place search with the JavaScript Maps SDK, scripting APIs, or open source libraries. The general steps are to:
- Reference the places service.
- Set the min and max
x
andy
values for theradius
orextent
, as well as thecategory
.Ids - Set the access token.
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/near-point?x=<LONGITUDE>&y=<LATITUDE>&radius=<METERS>&categoryIds=<IDs>&pageSize=<SIZE>&token=<ACCESS_TOKEN>&f=pjson
APIs
findPlacesNearPoint({
x: 24.938,
y: 60.169,
categoryIds: ["16026", "16041", "10027"],
radius: 500,
authentication,
}).then(results => {
console.log(JSON.stringify(results.places, null, 2))
})
REST API
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/near-point' \
-d 'x=24.938' \
-d 'y=60.169' \
-d 'radius=500' \
-d 'categoryIds=16026,16041,16032' \
-d 'token=<ACCESS_TOKEN>' \
-d 'f=pjson'
3. Get place details
The last step is to request additional place attributes for each place by using the /places/{place
operation.
To get place details, you need to define the following:
- Reference the places service.
- Set the place ID returned from either a nearby or bounding box search.
- Set the
requested
parameter to specify the fields you want. The request fields are grouped into Place, Address, and/or Details price groups. The number of attributes returned can vary depending on the type of place.Fields - Set the access token.
https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/<placeId>?requestedFields=<place_details>&token=<ACCESS_TOKEN>&f=pjson
APIs
arcgisRest
.getPlaceDetails({
placeId: "bd5f5dfa788b7c5f59f3bfe2cc3d9c60",
requestedFields: ["name", "categories"],
authentication,
})
.then(results => {
console.log(JSON.stringify(results, null, 2))
})
REST API
curl 'https://places-api.arcgis.com/arcgis/rest/services/places-service/v1/places/bd5f5dfa788b7c5f59f3bfe2cc3d9c60' \
-d 'requestedFields=name,categories' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>' \
Additional resources
Tutorials
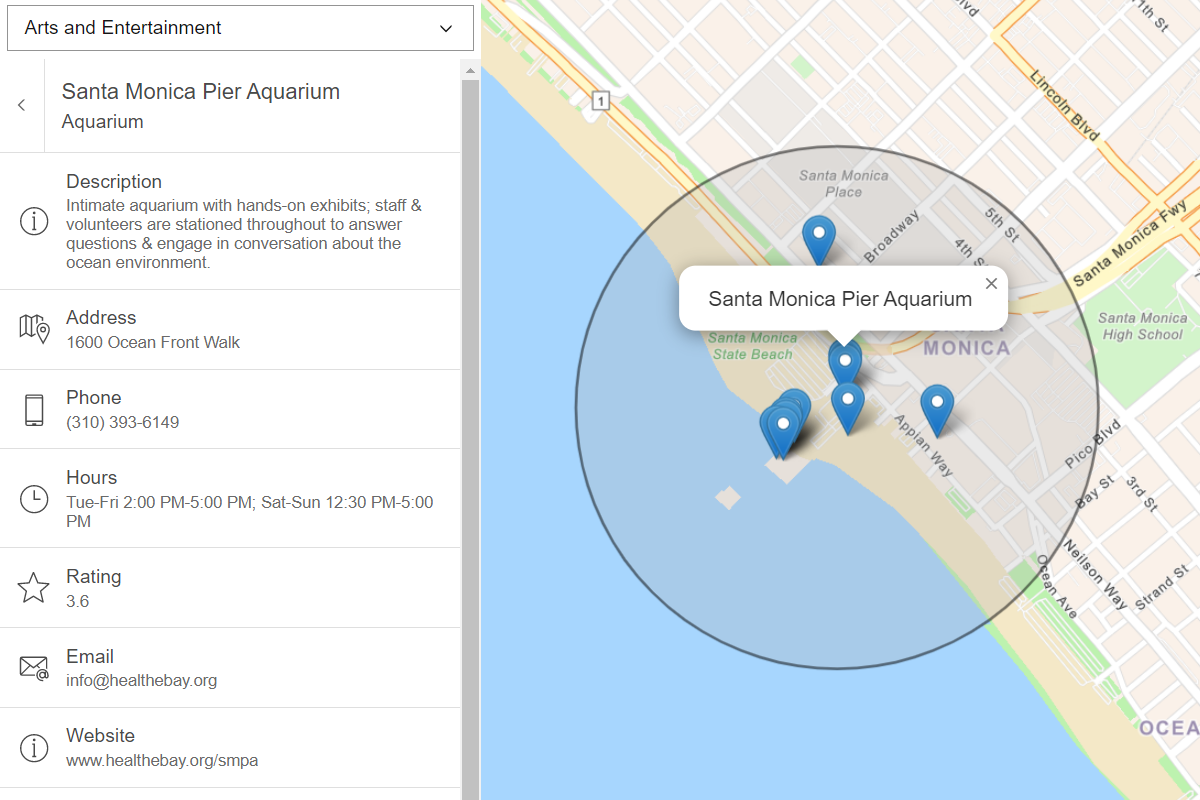
Find nearby places and details
Find points of interest near a location and get detailed information about them.
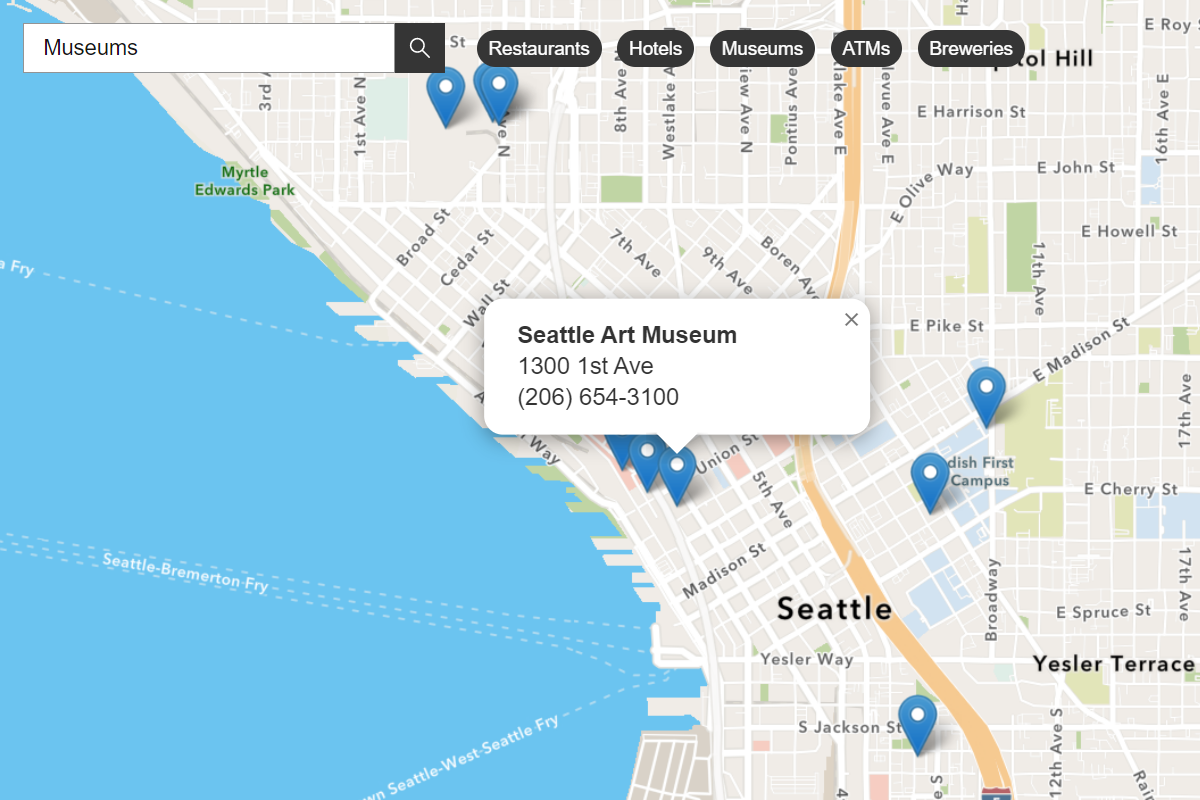
Find places in a bounding box
Perform a text-based search to find places within a bounding box.