To display vector tile layers, you need to use a client-side mapping API such as ArcGIS Maps SDKs or open source libraries. In most cases, the APIs support a class you can use to make requests to a vector tile service with a service URL. The API is responsible for requesting tiles for the visible map extent.
How to display vector tiles
The steps to display vector tiles are:
- Find the URL for the vector tile service you want to display.
- Set the service URL for the vector tile service.
- Add the layer to a map.
To access layer data, use a URL with the host, unique service ID, and path to tiles in {z}/{y}/{x}
format.
https://{host}/{organizationId}/arcgis/rest/services/{serviceName}/VectorTileServer/tile/{z}/{y}/{x}.pbf
https://{host}/{organizationId}/arcgis/rest/services/{serviceName}/VectorTileServer/tile/{z}/{y}/{x}.pbf
https://{host}/{webAdaptor}/rest/services/Hosted/{serviceName}/VectorTileServer/tile/{z}/{y}/{x}.pbf
Code examples
Get vector tile styles
To access the service styles, use a URL with the host, unique service ID, and path to root.json
.
https://{host}/{organizationId}/arcgis/rest/services/{serviceName}/VectorTileServer/resources/styles/root.json
Display a vector tile layer
To display a hosted vector tile layer, you reference the layer by its URL or ID, and then add it to a map or scene. The API communicates with the service to retrieve data for the current visible extent. ArcGIS Maps SDKs optimize data access by utilizing the service functionality and caching.
Steps
- Create a map or scene.
- Get the hosted vector tile itemID or layer URL.
- Add the layer.
esriConfig.apiKey = "YOUR_ACCESS_TOKEN"
const vectorLayer = new VectorTileLayer({
portalItem:{
id: "c4fc087b100542fe91df0b279df23ef9"
},
opacity: 0.75,
})
const map = new Map({
basemap: "arcgis-light-gray",
layers: [vectorLayer],
})
Tutorials
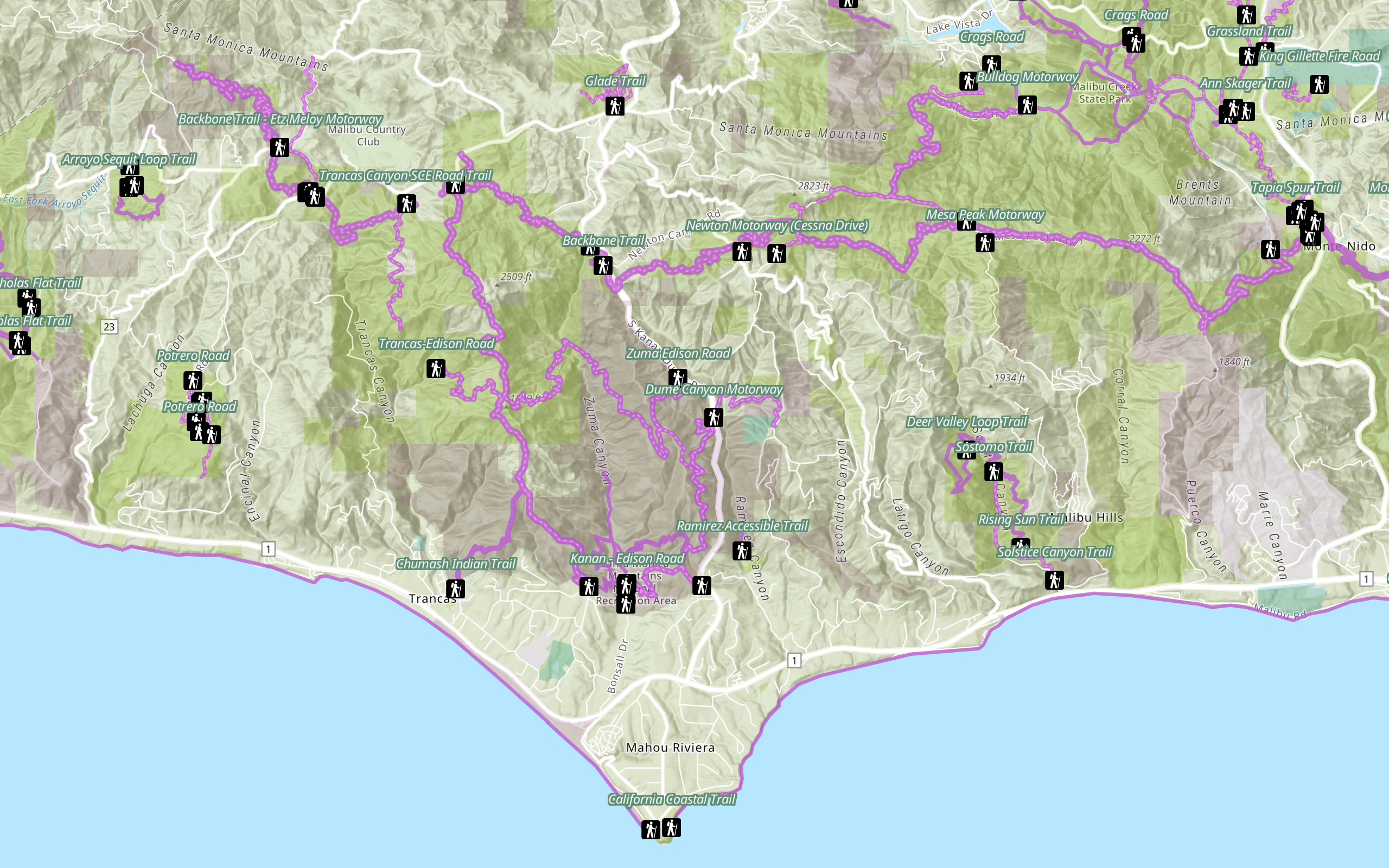
Add a feature layer
Access and display point, line, and polygon features from a feature service
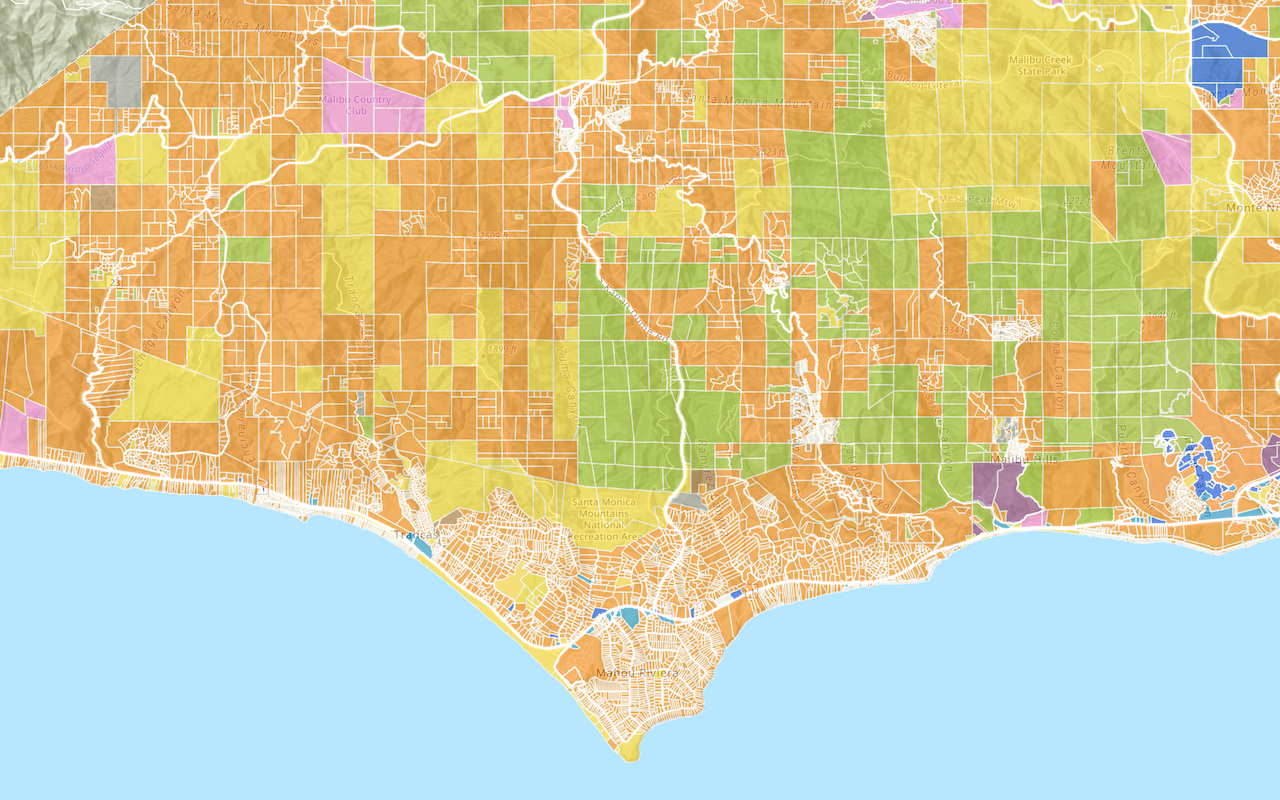
Add a vector tile layer
Access and display a vector tile layer in a map.
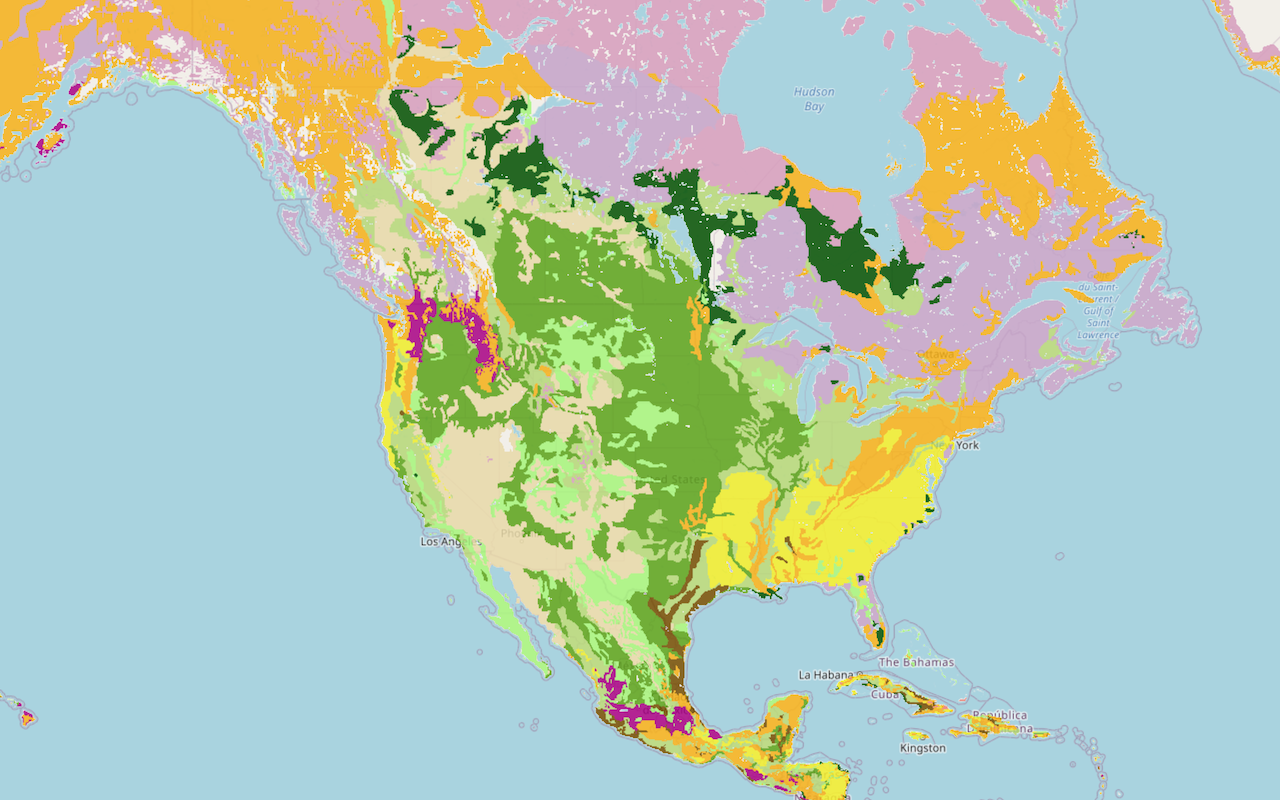
Add a map tile layer
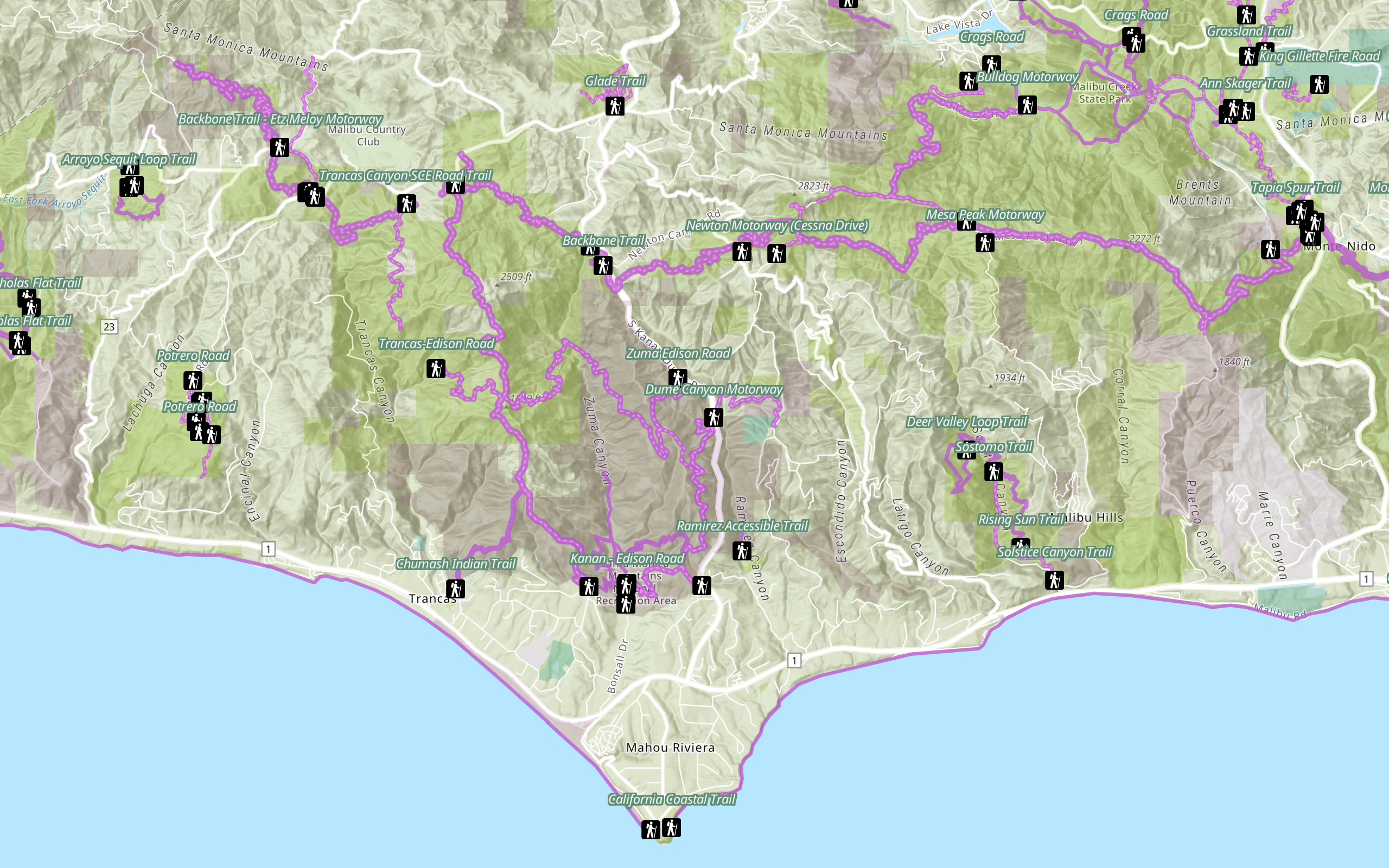
Style a feature layer
Use symbols and renderers to style feature layers.
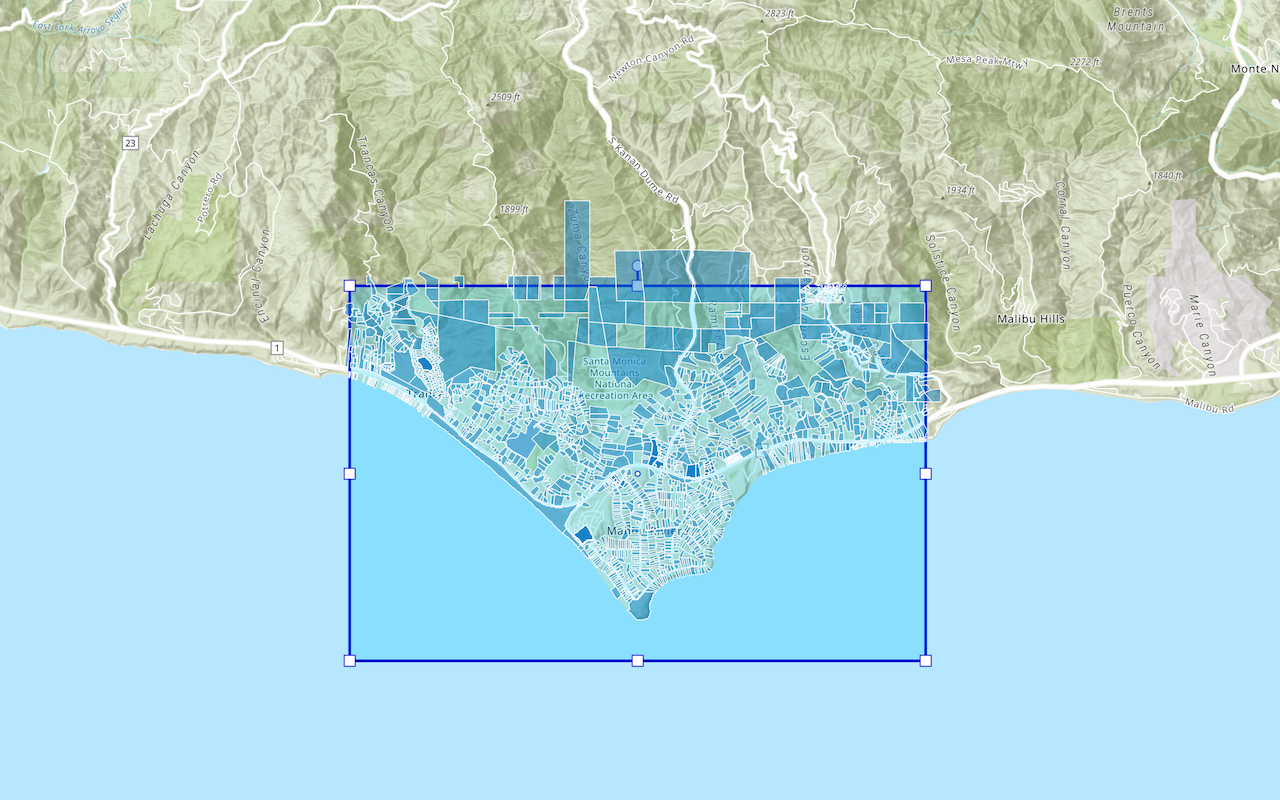
Query a feature layer (spatial)
Execute a spatial query to get features from a feature layer.
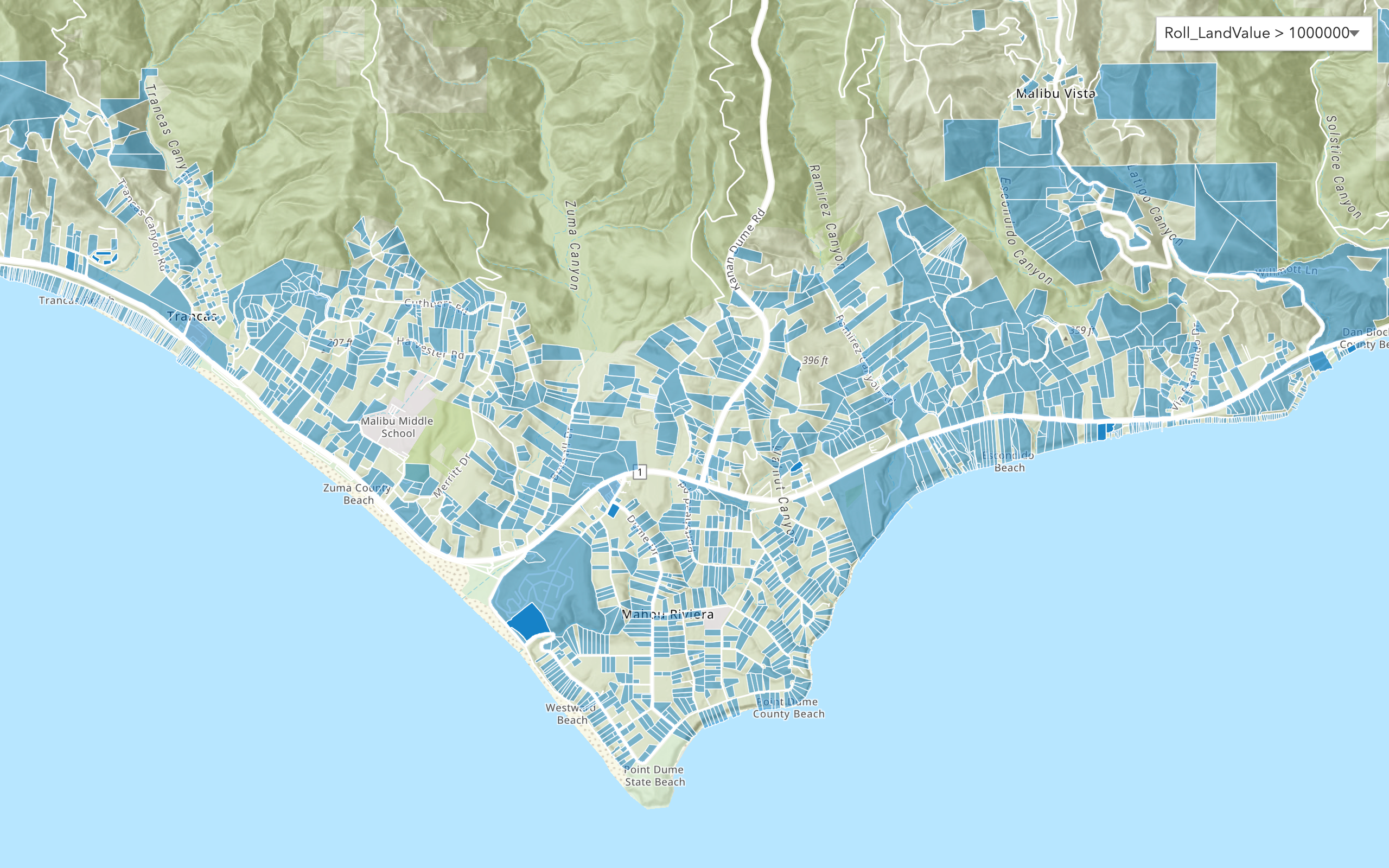
Query a feature layer (SQL)
Execute a SQL query to access polygon features from a feature layer.
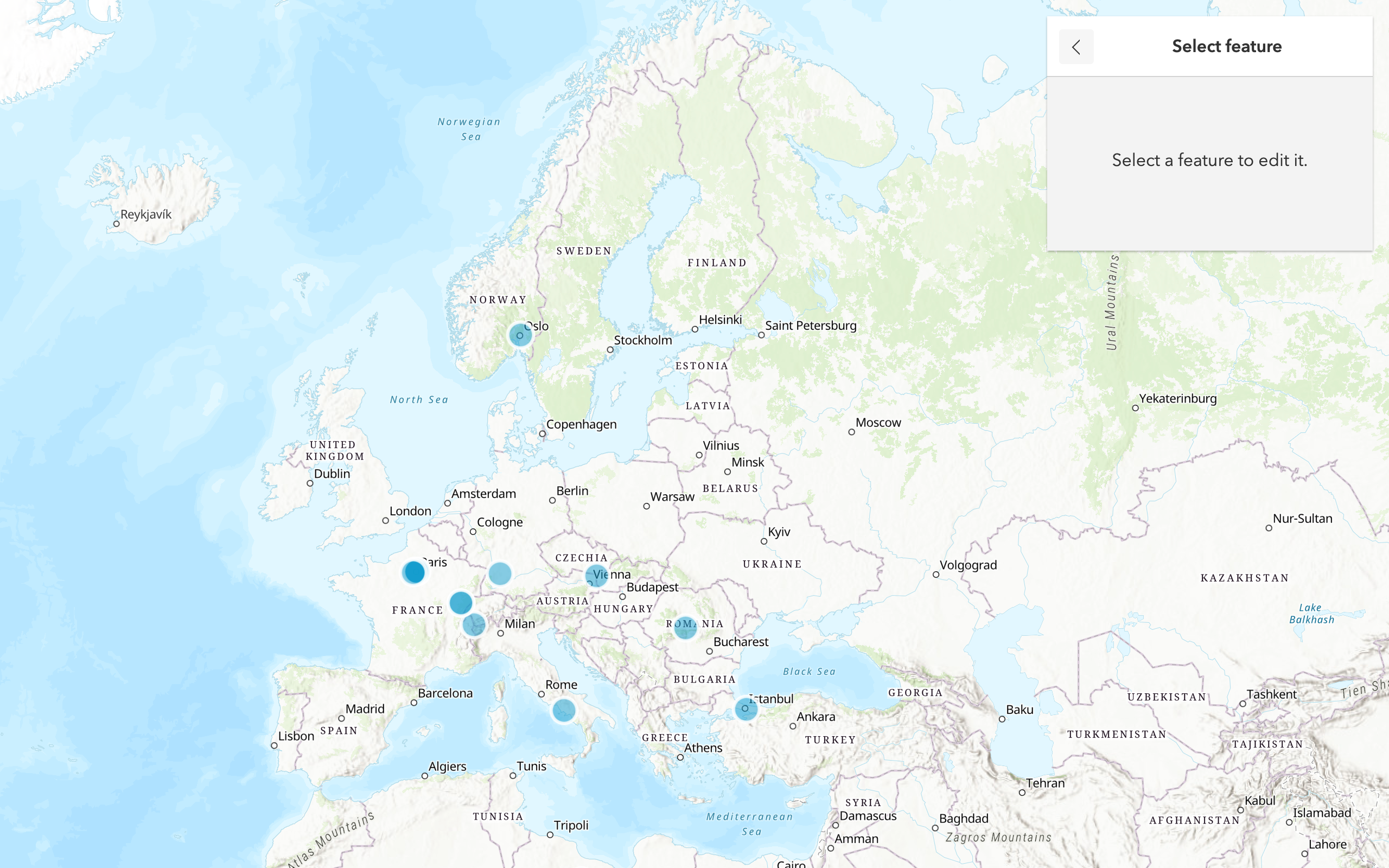
Edit feature data
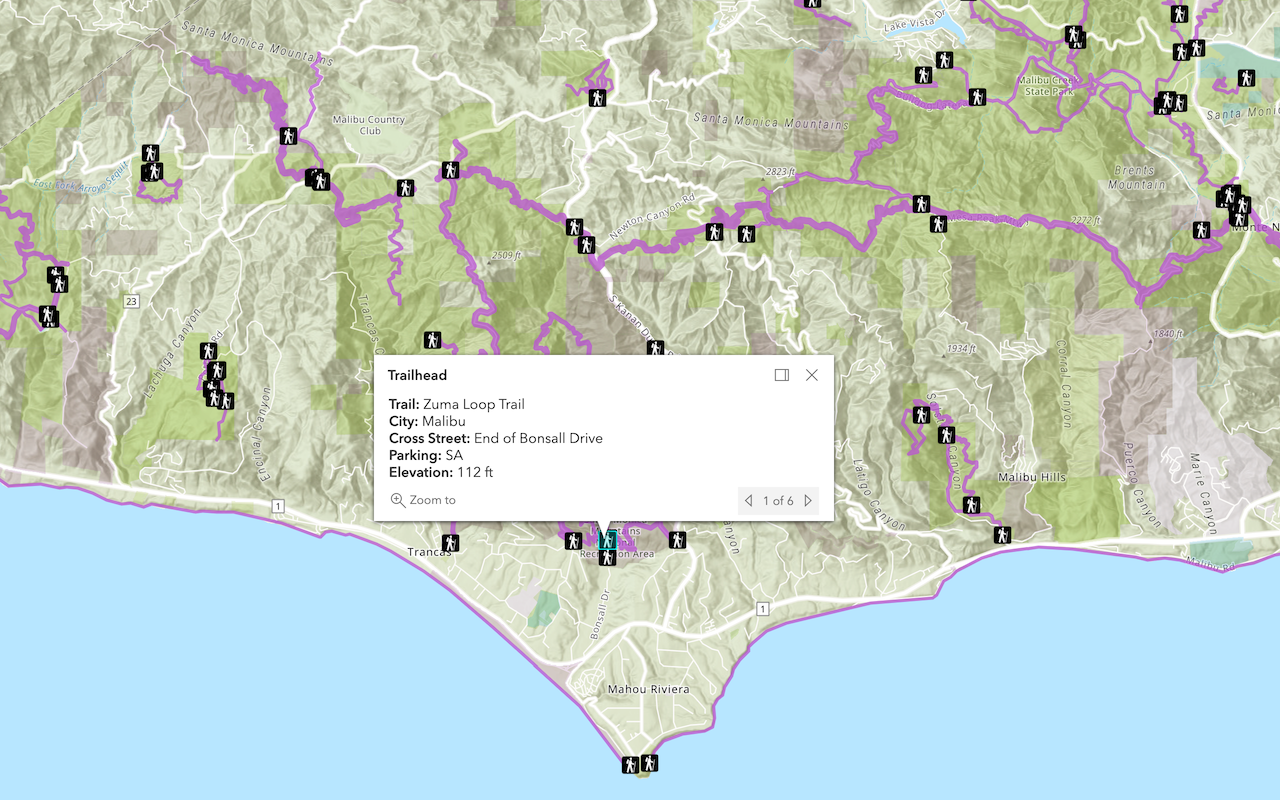
Display a popup
Format a popup to show attributes in a feature layer.
Workflows
Create a feature service for an app
Learn how to import parcel data, create and style a feature layer, and then access the features in an app.

Create a feature layer view for an editor app
Learn how to import parcel data, create and style a feature layer view, and then access the features in an editing app.

Create a vector tile service for an app
Learn how to import parcel data, style a feature layer, and then create a vector tile service for an app.

Create a map tile service for an app
Learn how to import contour data, style a feature layer, and create a map tile service for an app.

Services
Feature service
Add, update, delete, and query feature data.
Vector tile service
Store and access vector tile data.
Map tile service
Store and access map tile data.
Image service
Store and access imagery and raster data.
API support
Use data management tools or Client APIs to create, manage, and access data services. The table below outlines the level of support for each API.
- 1. Use portal class and direct REST API requests
- 2. Access via ArcGIS REST JS
- 3. Requires manually setting styles for renderers