The general steps to work with items.
1. Create an item
To create and access items, you can use interactive tools, client APIs, or the REST API.
The typical workflow is:
- Create and store an item with tools, such as ArcGIS Online and the Location Platform Dashboard.
- Manage your items with tools.
- Access items in the portal service with a client API or the REST API.
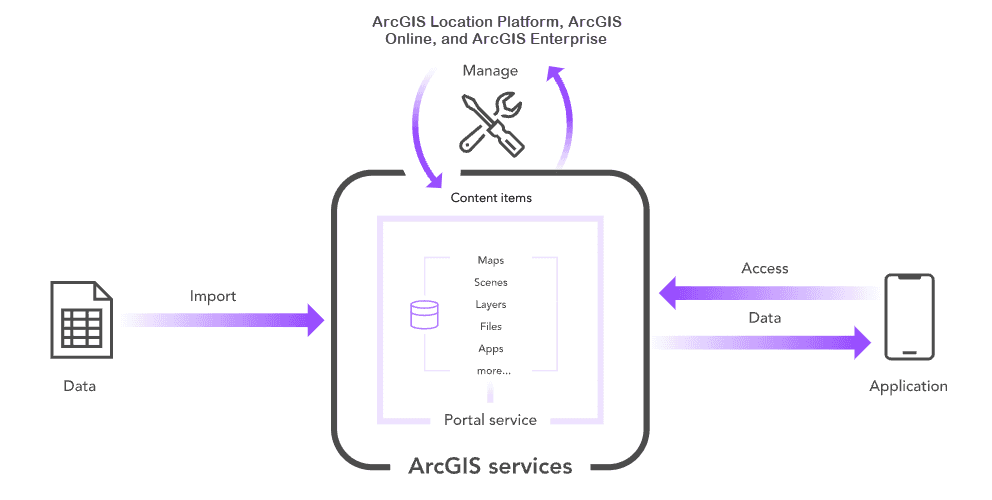
Examples
You can create and store new items in the portal by specifying the item type and other required parameters. This request requires an access token.
This example creates a web map item.
NOTE: Child data should also be provided to define the JSON for the web map itself. See Create a web map to learn how to create a web map and get the JSON.
APIs
const portal = new Portal();
portal.url = "https://www.arcgis.com/"
portal.authMode = "immediate";
// Prompts for username and password
portal.load()
.then(()=> {
const item = new PortalItem({
title: "World geography",
description: "This is a map of the world",
tags: ["map", "world", "geography"],
type: "Web Map"
});
const params = {
item: item
};
portal.user.addItem(params).then((response)=>{
console.log(response); // Item information
});
});
REST API
curl https://www.arcgis.com/sharing/rest/content/users/<USER_NAME>/addItem \
-d 'f=pjson' \
-d 'title=World geography' \
-d 'description=This is the map of the world' \
-d 'type=Web Map' \
-d 'tags=map, world, geography' \
-d 'token=<ACCESS_TOKEN>'
2. Manage an item
The easiest way to access and manage an item is to use an item page. An item page is a web page where you can access item properties through tools such as ArcGIS Online and the Location Platform Dashboard.
Item pages
Access an item page to view and manage the item properties.
Public and private URL examples:
https://www.arcgis.com/home/item.html?id=<ITEM_ID>&token=<ACCESS_TOKEN>
Share an item
To share an item with different users, set the access
property. The scope can be public (everyone), organization (your organization members only), group (private or public group), or private (only you).
You must be the item's owner or an administrator for the organization to change this property.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
item = gis.content.get("ITEM_ID")
results = item.share(everyone=True)
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/content/users/<YOUR_ORG>/items/<ITEM_ID>/share \
-d 'everyone=true' \
-d 'org=true' \
-d 'groups=<GROUP_IDs>' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
3. Access an item
To access and work with items in the portal, you can use client APIs or the REST API.
Get an item
To access an item and its properties, you can access it by item ID
. The response contains the item properties.
The public example shows how to access a public web map item by its ID. The private example shows how to access any private item that you own by ID.
APIs
const item = new PortalItem({
id: "5a5147abe878444cbac68dea9f2f64e7",
portal: "https://www.arcgis.com/sharing/rest"
});
item.load().then((response)=>{
console.log(response);
});
REST API
## Public
curl https://www.arcgis.com/sharing/rest/content/items/5a5147abe878444cbac68dea9f2f64e7 \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/content/items/<ITEM_ID> \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Get item data
Items can contain child data. The data is typically stored as text (JSON) or as a reference to services, files, or other resources.
The public example gets the child data for a public web map item. The private example gets the child data for an item that you own. The data returned is the JSON. To create your own private item, see Create a web map.
APIs
REST API
## Public
curl https://www.arcgis.com/sharing/rest/content/items/5a5147abe878444cbac68dea9f2f64e7/data \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/content/items/<ITEM_ID>/data \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Search for an item
Use a query to search for items in the portal. If many results are returned, use paging.
The public example searches for all items that contain the string, type, and owner. The private example searches for your private items that are web maps.
APIs
const portal = new Portal({
url: "https://www.arcgis.com/"
});
portal.load().then(()=>{
const query = {
query: "title: Seven Natural Wonders, type: web map, owner: esri_devlabs"
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
## Public
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"Seven Natural Wonders of the World" AND type:"web map" AND owner:"esri_devlabs"' \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"Seven Natural Wonders of the World" AND type:"web map" AND owner:"<YOUR_USER_ID>"' \
-d 'f=pjson'
-d 'token=<ACCESS_TOKEN>'
Services
API support
- 1. Limited operations, use HTTP requests.
- 2. Access via ArcGIS REST JS.
Tools
Use tools to access the portal and create and manage content for applications.
ArcGIS Enterprise
Create, manage, analyze, and share data, maps, and applications in your organization.
Portal
Create, manage, and access content and data services for applications.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, style, and explore web scenes.
Vector Tile Style Editor
Create styles for basemap and vector tile layers.
Content management tools
Create, manage, organize, and share items in a portal.
Data management tools
Import data and create hosted layers and data services. Upload and manage documents, images, and other files.
Spatial analysis tools
Perform feature and raster analysis to create new datasets with the Map Viewer.
Developer credentials tool
Create API key and OAuth 2.0 developer credentials for custom applications.
Items
Manage and share items.
ArcGIS Pro
Create, style, and explore maps and scenes.
Geoprocessing tools
Import, manage, and analyze data.