Learn how to use the portal service to create copy a hosted layer item in your portal.
Prerequisites
You need the following for this tutorial:
- Account: An ArcGIS Location Platform, ArcGIS Online, or ArcGIS Enterprise account.
- Authentication: A basic understanding of API key authentication or User authentication.
Steps
Get the portal URL
To access a portal, you need an ArcGIS account which is associated with an organization that allows you to store and manage your content.
- In a web browser, sign in to your portal with your ArcGIS account.
- Identify the portal URL from the navigation bar. The base URL should be one of the following:
- ArcGIS Location Platform:
https
://www.arcgis.com/sharing/rest - ArcGIS Online:
https
://www.arcgis.com/sharing/rest - ArcGIS Enterprise:
https
://{machine.domain.com}/{webadaptor}/rest
- ArcGIS Location Platform:
Implement authentication
You need an access token with the correct privileges for in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
-
Privileges
- Admin privileges > Content > View all
- Admin privileges > Content > Update
-
Set up environment
- In a different window, launch Postman and create a blank request.
Copy a hosted layer item
- Add the following import statement to your code.
main.py
Use dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureLayerCollection
- Establish a connection to the portal using the
GI
module.S() main.pyUse dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureLayerCollection # Replace with your actual username, password, and item ID username = 'your_username' password = 'your_password' feature_layer_item_id = 'your_feature_layer_item_id' # The item ID of the item you want to copy portal_url = 'https://www.arcgis.com' # Authenticate with ArcGIS Online gis = GIS(portal_url, username, password)
- Get the item using the
item
.ID main.pyUse dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureLayerCollection # Replace with your actual username, password, and item ID username = 'your_username' password = 'your_password' feature_layer_item_id = 'your_feature_layer_item_id' # The item ID of the item you want to copy portal_url = 'https://www.arcgis.com' # Authenticate with ArcGIS Online gis = GIS(portal_url, username, password) # Access the hosted feature layer item feature_layer_item = gis.content.get(feature_layer_item_id)
- Use the
clone
method to create a deep copy of the feature layer item._items main.pyUse dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureLayerCollection # Replace with your actual username, password, and item ID username = 'your_username' password = 'your_password' feature_layer_item_id = 'your_feature_layer_item_id' # The item ID of the item you want to copy portal_url = 'https://www.arcgis.com' # Authenticate with ArcGIS Online gis = GIS(portal_url, username, password) # Access the hosted feature layer item feature_layer_item = gis.content.get(feature_layer_item_id) # Use the `clone_items` method to create an entirely new copy of the feature layer item cloned_items = gis.content.clone_items([feature_layer_item]) # Check the result of the clone operation if cloned_items: print(f"Successfully cloned the item. New item ID: {cloned_items[0].id}") else: print("Failed to clone the item.")
View the results
If successful, your code will print an output like this to verify that the operation was successful:
Successfully cloned the item. New item ID: <new_item_id>
What's next
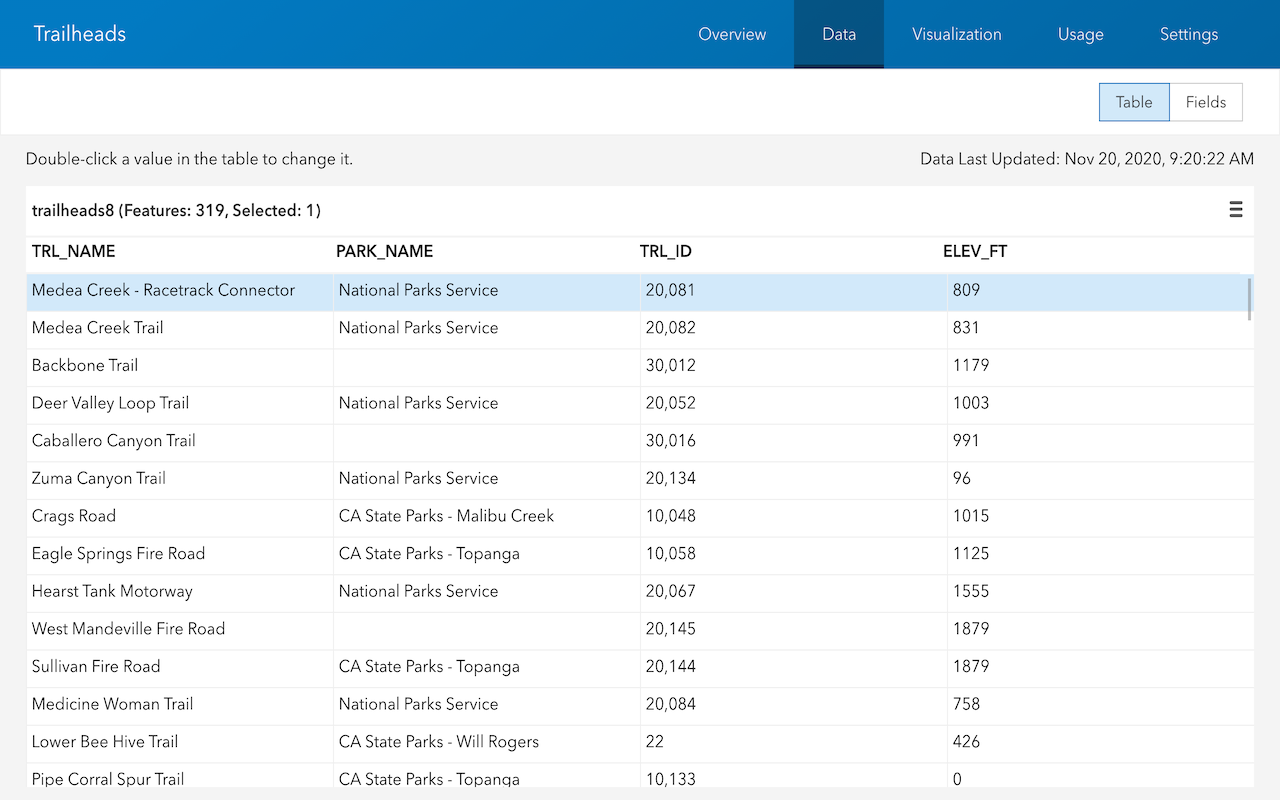
Sign in and access your portal
Use the portal service to sign in and access your portal.
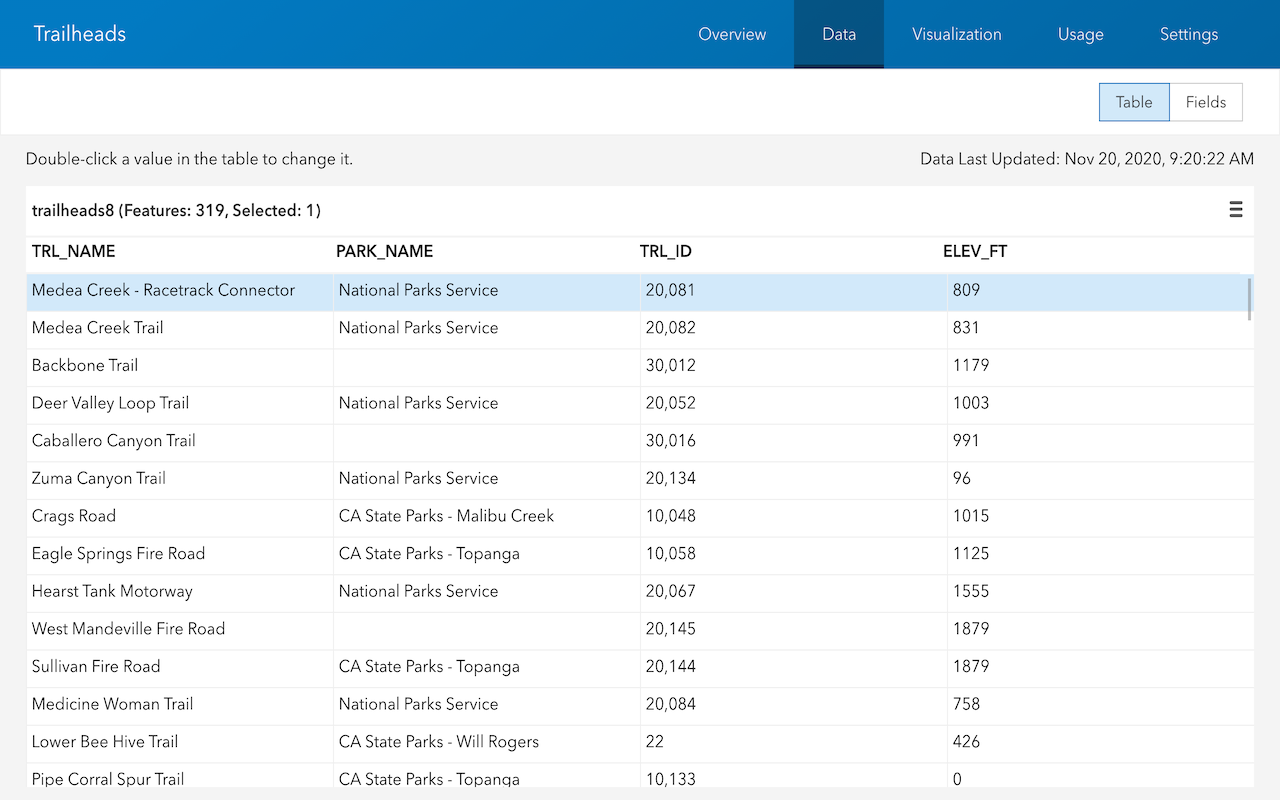
List items in your portal
Use the portal service to list items in your portal.
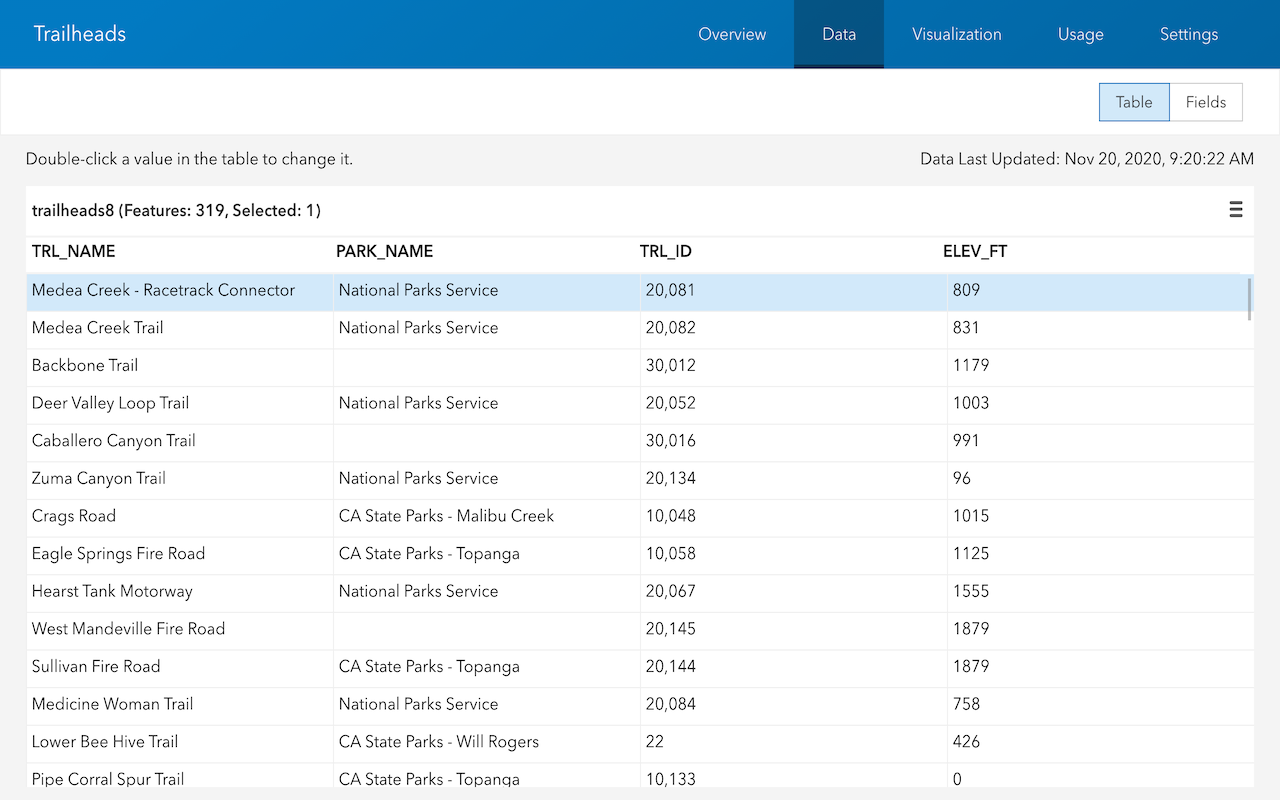
Set sharing level for an item
Use the portal service to set the sharing level for an item in your portal.