You can use the portal service to access and manage groups in an organization. A group is a container for items available to a specific set of users in the portal.
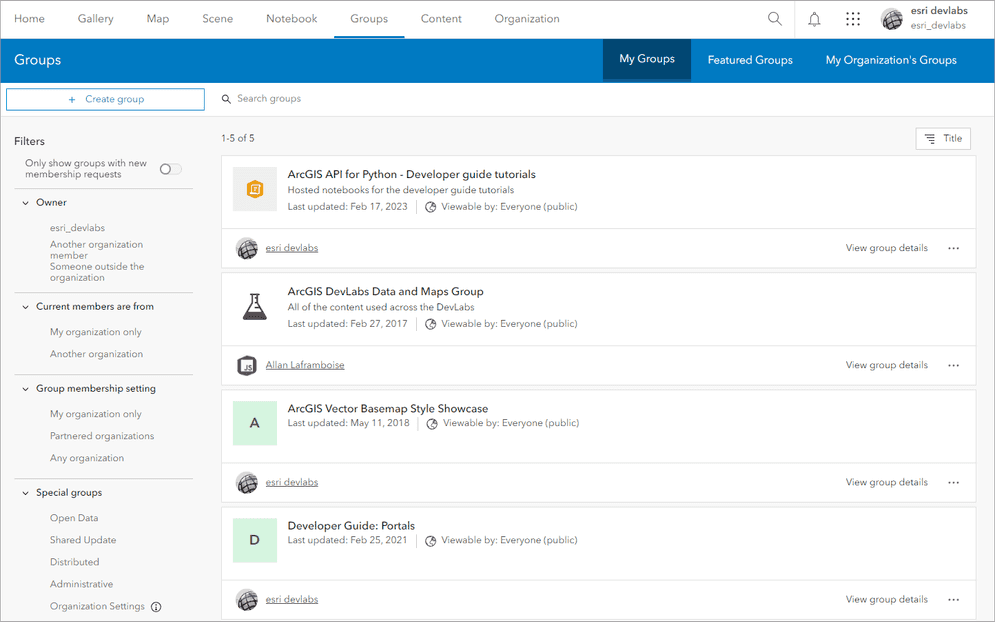
Working with groups
Groups are used to organize and share items and define which users can access and view specified content. You can create groups of hosted layers, web maps, or any other item type. Or you can create a group and add users to it, allowing them to collaborate and share items within the group.
The typical workflow for using groups is to:
- Define a group in the portal.
- Add items the group.
- Add users to the group.
- Manage the group properties.
Code examples
Search for a group
Find a one or more groups in the portal using a query.
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: "water"
};
portal.queryGroups(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/community/groups \
-d 'q=water' \
-d 'f=pjson'
Create a new group
Define a new group and how it can be accessed.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
group = gis.groups.create(title="Traffic issues", tags="traffic", access="public")
print(group)
REST API
curl https://www.arcgis.com/sharing/rest/community/createGroup \
-d 'title=Traffic issues' \
-d 'access=public' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Invite users to a group
Invite users by their user names.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
group = gis.groups.get("GROUP_ID")
results = group.invite_users(usernames=["USER_TO_INVITE"])
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/community/groups/<GROUP_ID>/invite \
-d 'users=<USER_TO_INVITE>' \
-d 'role=group member' \
-d 'expiration=20160' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Add items to a group
Add an item to group to be shared with a set of users.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
item = gis.content.get("ITEM_ID")
results = item.share(groups="GROUP_ID")
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/content/users/<USERNAME>/items/<ITEM_ID>/share \
-d 'f=json' \
-d 'groups=<GROUP_ID>' \
-d 'token=<ACCESS_TOKEN>'
Search for group content
Find items shared with a group using a query.
APIs
const portal = new Portal();
portal.url = "https://www.arcgis.com/"
portal.load()
.then(()=> {
const portalGroup = new PortalGroup({
id: "d6340748701d4ccfb18ad3c42b7feb9e",
portal: portal
});
const queryParameters = {
query: "bike"
};
portalGroup.queryItems(queryParameters).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/content/groups/d6340748701d4ccfb18ad3c42b7feb9e/search \
-d 'q=bike' \
-d 'f=pjson'
Update properties
Change the properties of a group such as the name, description, and permissions.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
group = gis.groups.get("GROUP_ID")
results = group.update("new") # new title
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/community/groups/GROUP_ID/update \
-d 'title=new' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Services
API support
- 1. Limited operations, use HTTP requests.
- 2. Access via ArcGIS REST JS.
Tools
Use tools to access the portal and create and manage content for applications.
ArcGIS Enterprise
Create, manage, analyze, and share data, maps, and applications in your organization.
Portal
Create, manage, and access content and data services for applications.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, style, and explore web scenes.
Vector Tile Style Editor
Create styles for basemap and vector tile layers.
Content management tools
Create, manage, organize, and share items in a portal.
Data management tools
Import data and create hosted layers and data services. Upload and manage documents, images, and other files.
Spatial analysis tools
Perform feature and raster analysis to create new datasets with the Map Viewer.
Developer credentials tool
Create API key and OAuth 2.0 developer credentials for custom applications.
Items
Manage and share items.
ArcGIS Pro
Create, style, and explore maps and scenes.
Geoprocessing tools
Import, manage, and analyze data.