You can use the portal service to access and manage users in an organization. A user is a stored identity that represents a registered user of the portal.
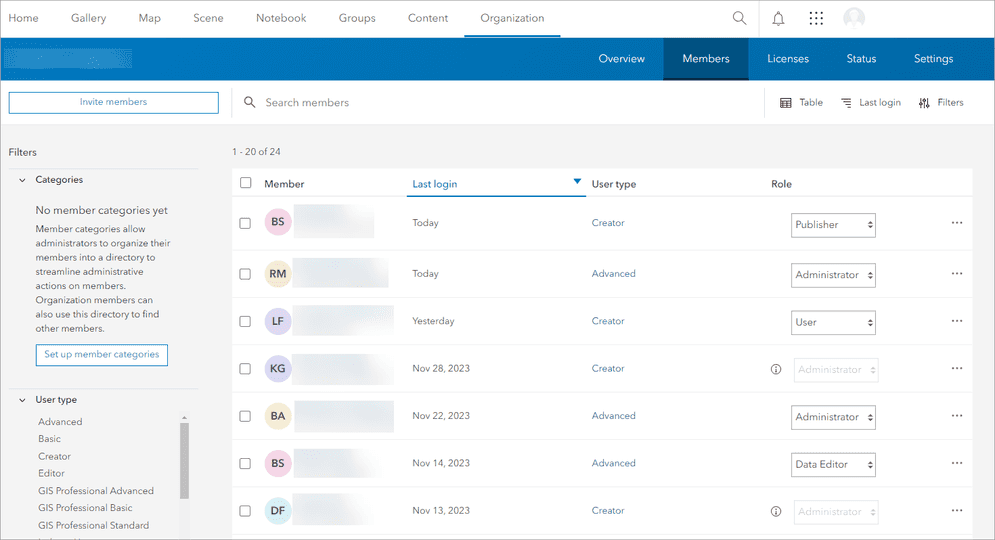
Working with users
A user is created when someone signs up for a developer account, when an account is created for a user by an administrator, or when someone joins an organization. When an account is created, an identity and a role are assigned. The role defines the set of privileges and operations that the user can perform within the organization associated with the account.
Administrators can also use data management tools and ArcGIS REST API to create and manage users. Only an administrator or the owner of a user's account can make updates to users within an organization.
The typical way to work with users is to:
- Invite users or create new users in the portal.
- Search for users.
- Assign users to groups.
- Manage user properties.
Code examples
Create a new user
Create a new user using new member default values.
Python
from arcgis.gis import GIS
gis = GIS() # Default portal: https://www.arcgis.com/
new_user = gis.users.create(username= 'new_unique_username',
password= '<strong_password>',
firstname= 'user_firstname',
lastname= 'user_lastname',
email= 'user_email@company.com',
description= 'new user using member defaults')
print(f"New user: {new_user.username}")
print(f"Role: {new_user.role}")
print(f"User type: {new_user.userLicenseTypeId}")
Search for a user
Use a query to search users by name, description, and other information.
APIs
const portal = new Portal();
portal.url = "https://www.arcgis.com/"
portal.authMode = "auto";
portal.load()
.then(()=> {
const queryParameters = {
query: "JSmith"
};
portal.queryUsers(queryParameters).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/community/users \
-d 'q=JSmith' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Get a user
Find a user with their username.
APIs
const url = "https://www.arcgis.com/sharing/rest/community/users/jsmith"
const options = {
query: {
f: "pjson"
},
responseType: "pjson"
};
esriRequest(url, options,{
}).then((response)=>{
const data = response.data
console.log(data);
})
REST API
curl https://www.arcgis.com/sharing/rest/community/users/jsmith \
-d 'f=pjson'
Update user information
Update user profile information such as their role or credits assigned.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
user = gis.users.get(gis.users.me.username)
results = user.update(description="A new description of your user account.")
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/community/users/<USERNAME>/update \
-d 'username=<USERNAME>'
-d 'description=A new description of user ' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Get invitation status
Find the status of an invitation that was sent to a user to join the portal.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
invitation = gis.users.invitations.get("INVITATION_ID")
print(invitation)
REST API
curl https://www.arcgis.com/sharing/rest/community/users/<USERNAME>/invitations/<INVITATION_ID> \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Services
API support
- 1. Limited operations, use HTTP requests.
- 2. Access via ArcGIS REST JS.
Tools
Use tools to access the portal and create and manage content for applications.
ArcGIS Enterprise
Create, manage, analyze, and share data, maps, and applications in your organization.
Portal
Create, manage, and access content and data services for applications.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, style, and explore web scenes.
Vector Tile Style Editor
Create styles for basemap and vector tile layers.
Content management tools
Create, manage, organize, and share items in a portal.
Data management tools
Import data and create hosted layers and data services. Upload and manage documents, images, and other files.
Spatial analysis tools
Perform feature and raster analysis to create new datasets with the Map Viewer.
Developer credentials tool
Create API key and OAuth 2.0 developer credentials for custom applications.
Items
Manage and share items.
ArcGIS Pro
Create, style, and explore maps and scenes.
Geoprocessing tools
Import, manage, and analyze data.