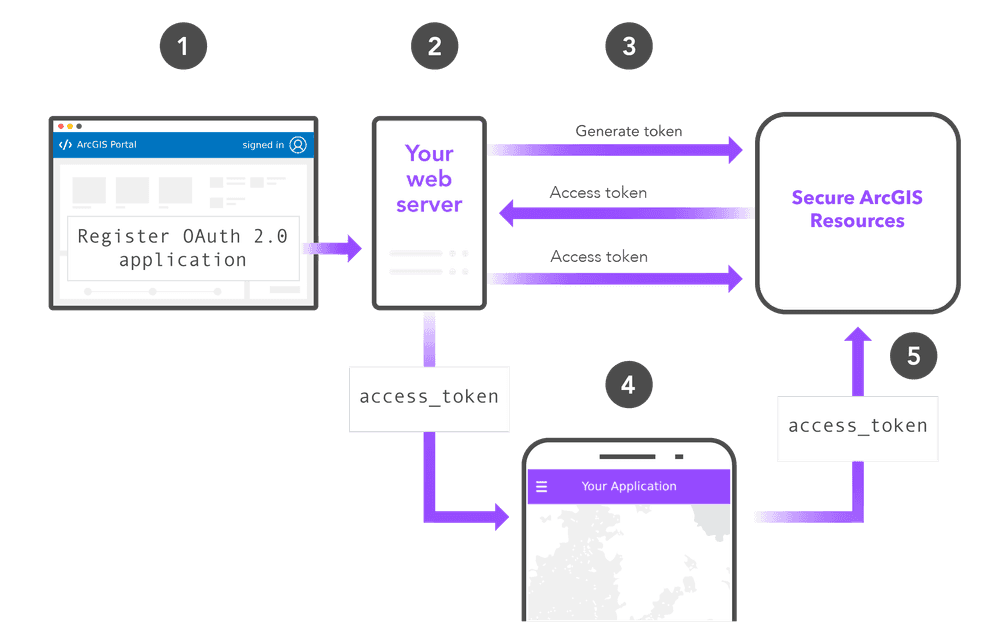
ArcGIS uses a client credentials flow to implement app authentication. In this flow, a secure server uses a client
and client
from a set of OAuth credentials to request an access token, then delivers the token to a client application.
The diagram above explains this flow using the following steps:
-
OAuth credentials are registered in the portal to obtain a
client
and_id client
._secret -
The confidential
client
and_id client
are stored in a server-side component._secret -
The server gets an access token by submitting a request to your organization's portal service
-
The server delivers the access token to the client application upon request.
-
The client application uses the access token to authorize requests to secure resources.
This flow adheres to the client
grant type defined in the OAuth 2.0 specification. The main benefit of this flow is that the server handles requesting an access token, ensuring that the confidential client
and client
values are never exposed to the client application. To read more about the client credentials protocol, go to OAuth 2.0 RFC 6749 section 4.4.
Manual implementation
The remainder of this page shows how to manually implement app authentication by making direct requests to your organization's portal service. The sample is written in JavaScript, but can be implemented in any language by making HTTP requests.
Create OAuth credentials
A set of OAuth credentials are required for app authentication. These credentials are created as an item in your organization's portal.
The steps to create OAuth credentials with an ArcGIS Location Platform account are:
-
Sign in to your ArcGIS portal.
-
Click Content > My content > New item and select Developer credentials.
-
In the Credential types menu, select OAuth credentials.
-
Add a redirect URL and click Next. This URL is required during creation, but will not be used in app authentication.
-
Set the credential privileges to determine the operations your access tokens will be authorized to perform.
-
Set the credential item access privileges to determine the items your access tokens will be authorized to access.
-
Review your selections and, when you are ready, click Generate credentials.
Configure authentication variables
-
Copy the
client
and_id client
parameters from your OAuth credentials and paste them into a new application._secret server.jsUse dark colors for code blocks const clientId = 'YOUR_CLIENT_ID'; const clientSecret = 'YOUR_CLIENT_SECRET';
Request the token endpoint
App authentication is implemented by submitting a request to the token endpoint of your ArcGIS organization.
-
Find the URL of the token endpoint for your ArcGIS organization. For ArcGIS Online and Location Platform users, the token endpoint is
https
.://www.arcgis.com/sharing/rest/oauth2/token server.jsUse dark colors for code blocks const token_endpoint = ' https://www.arcgis.com/sharing/rest/oauth2/token';
-
Submit an HTTP POST request to the endpoint. Include your
client
,_id client
, and a_secret grant
parameter set to_type 'client
._credentials' server.jsUse dark colors for code blocks const token_endpoint = ' https://www.arcgis.com/sharing/rest/oauth2/token'; const response = await fetch(token_endpoint, { method: 'POST', headers: { "Content-type":"application/x-www-form-urlencoded" }, body: new URLSearchParams({ 'grant_type':'client_credentials', 'client_id':clientId, 'client_secret':clientSecret }) })
Use the token
After obtaining the access token, you can use it to authorize requests directly from the server or alternatively deliver it to a client application. The method of implementation depends on the framework and libraries you are using.