This topic outlines the high-level steps of how to implement app authentication.
1. Create OAuth credentials
App authentication requires a set of OAuth credentials.
The steps to create OAuth credentials with an ArcGIS Location Platform account are:
-
Sign in to your ArcGIS portal.
-
Click Content > My content > New item and select Developer credentials.
-
In the Credential types menu, select OAuth credentials.
-
Add a redirect URL and click Next. This URL is required during creation, but will not be used in app authentication.
-
Set the credential privileges to determine the operations your access tokens will be authorized to perform.
-
Set the credential item access privileges to determine the items your access tokens will be authorized to access.
-
Review your selections and, when you are ready, click Generate credentials.
2. Implement a client credentials flow
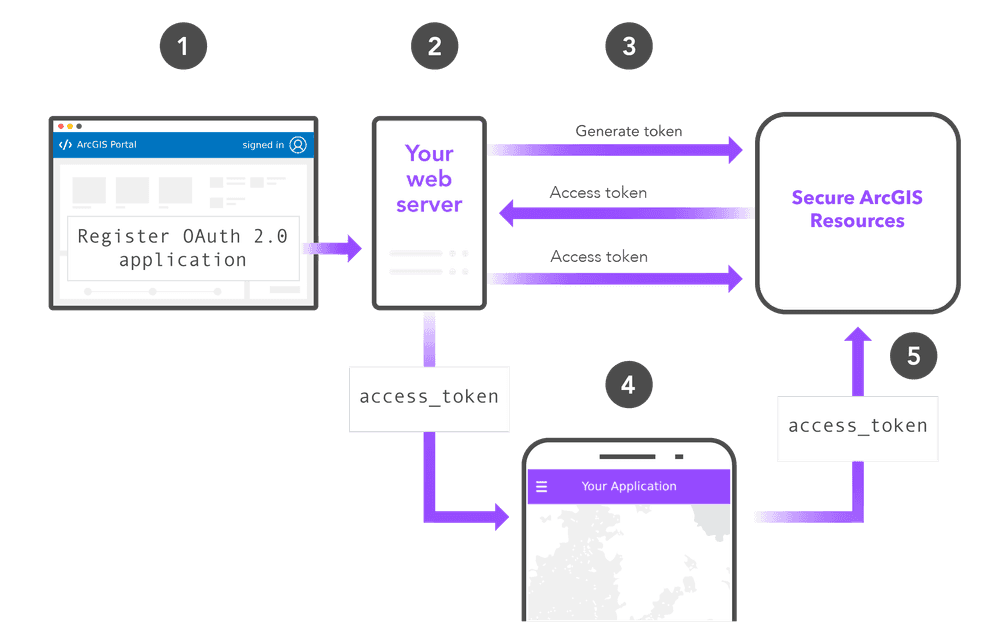
App authentication uses an OAuth 2.0 authorization flow with a grant type of client
. This involves making a request to the token endpoint with a client
and client
from OAuth credentials. The high-level steps to implement this flow are as follows:
-
Paste the
client
and_id client
from a set of OAuth credentials into your application._secret -
Submit a POST request to the token endpoint, either directly or through a helper class provided by an ArcGIS API.
-
Use the access token returned in the response. If you made the request on a server, you can now send the access token to your client application.
ArcGIS APIs
ArcGIS REST JS provides an Application
class that can be used to implement app authentication.
import { ApplicationCredentialsManager } from "@esri/arcgis-rest-request";
import { geocode } from "@esri/arcgis-rest-geocoding";
const appManager = ApplicationCredentialsManager.fromCredentials({
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET"
});
appManager.refreshToken().then((manager) => {
Server-side examples
The following examples show how to set up a web server that implements app authentication and passes the resulting access token to a client application.
3. Make a request
Implementing app authentication successfully will grant an access token to your application when it requests one. The access token will have privileges defined by the OAuth credentials used to supply the client
and client
.
ArcGIS APIs and SDKs
If you use app authentication, the access token returned from the /oauth2/token
endpoint can be used directly in requests.
The examples below show how to display a map using an access token.
esriConfig.apiKey= "YOUR_ACCESS_TOKEN";
const map = new Map({
basemap: "arcgis/topographic" // Basemap layer
});
const view = new MapView({
map: map,
center: [-118.805, 34.027],
zoom: 13, // scale: 72223.819286
container: "viewDiv",
constraints: {
snapToZoom: false
}
});
ArcGIS REST APIs
Your application can also include the access token in requests to REST APIs by setting the token
parameter.
This example shows how to geocode an address with the geocoding service.
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates \
-d "f=pjson" \
-d "address=1600 Pennsylvania Ave NW, DC" \
-d "token=<YOUR_ACCESS_TOKEN>"