This topic outlines the high-level steps of how to implement user authentication.
1. Create OAuth credentials
User authentication requires a set of OAuth credentials.
The steps to create OAuth credentials with an ArcGIS Location Platform account are:
-
Sign in to your ArcGIS portal.
-
Click Content > My content > New item and select Developer credentials.
-
In the Credential types menu, select OAuth credentials.
-
Add a redirect URL and click Next.
-
In the Privileges and Grant item access menus, click Next; these are not required for user authentication.
-
Name the credentials and click Next to review. When you are ready to create the credentials, click Create.
2. Implement an OAuth flow
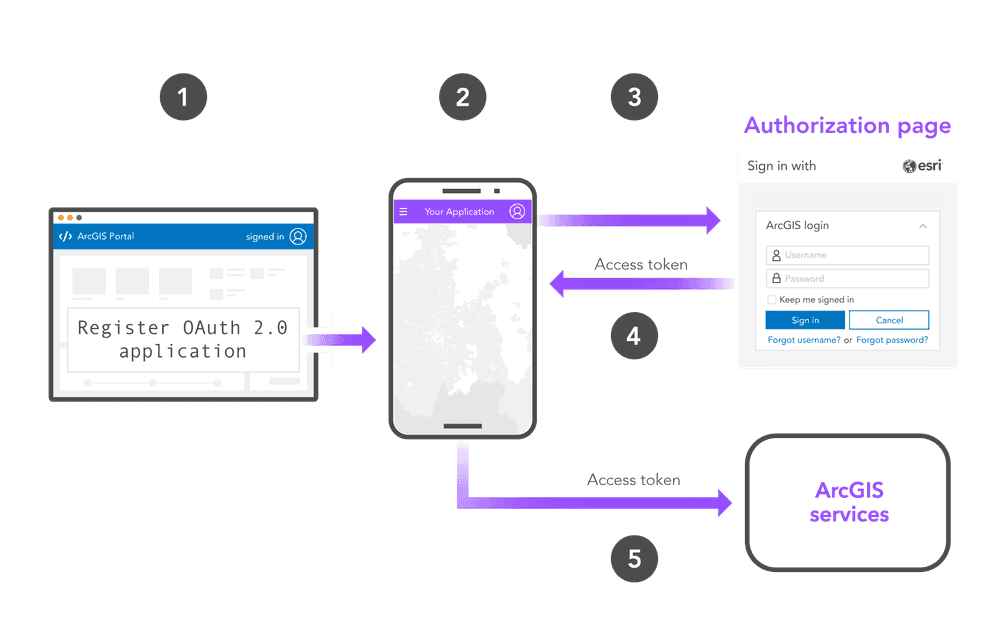
The next step is to implement a user authentication flow by adding authentication code to your application. ArcGIS supports several different user authentication flows that you can implement, most of which adhere to the OAuth 2.0 specification.
The OAuth 2.0 Authorization code flow with PKCE is the recommended flow for all client-facing applications. This is the authorization flow used by ArcGIS Maps SDKs and scripting APIs.
ArcGIS APIs and SDKs
User authentication is typically implemented using an ArcGIS Maps SDK or a scripting API such as ArcGIS REST JS or the ArcGIS API for Python. These libraries make it easy to implement user authentication using the built-in Authentication
and Identity
classes.
const info = new OAuthInfo({
appId: "YOUR_CLIENT_ID",
});
esriId.registerOAuthInfos([info]);
esriId
.checkSignInStatus(info.portalUrl + "/sharing")
.then(() => {
handleSignedIn();
})
.catch(() => {
handleSignedOut();
});
document.getElementById("sign-in").addEventListener("click", function () {
esriId.getCredential(info.portalUrl + "/sharing");
});
document.getElementById("sign-out").addEventListener("click", function () {
esriId.destroyCredentials();
window.location.reload();
});
let map;
Manual implementation
User authentication can also be implemented manually by making direct requests to the proper REST API endpoints. To do so, typically make a request to the authorization endpoint and token endpoint of your organization's portal service
This example shows how to implement an OAuth 2.0 authorization code flow with PKCE without an ArcGIS API.
const authorizationEndpoint = 'https://www.arcgis.com/sharing/rest/oauth2/authorize'+
'?client_id=' + clientId +
'&code_challenge=' + codeChallenge +
'&code_challenge_method=S256' +
'&redirect_uri=' + window.encodeURIComponent(redirectUri)+
'&response_type=code'+
'&expiration=20160';
signInButton.addEventListener('click', () => window.open(authorizationEndpoint, 'oauth-window', 'height=400,width=600,menubar=no,location=yes,resizable=yes,scrollbars=yes,status=yes'));
signOutButton.addEventListener('click', () => updateSession(false));
const oauthCallback = (authorizationCode) => {
const tokenEndpoint = 'https://www.arcgis.com/sharing/rest/oauth2/token';
fetch(tokenEndpoint, {
method:'POST',
body: JSON.stringify({
client_id:clientId,
grant_type:'authorization_code',
code:authorizationCode,
redirect_uri:redirectUri,
code_verifier:codeVerifier
}),
headers: {
"Content-type": "application/json; charset=UTF-8"
}
})
Generate token flow
The generate token flow can be used in situations where it is not possible to implement secure OAuth 2.0 flows. This flow requests an access token by making a request to the /generate
endpoint that includes an unencrypted username and password.
curl https://www.arcgis.com/sharing/rest/generateToken \
-d 'f=json' \
-d 'username=USERNAME' \
-d 'password=PASSWORD' \
-d 'referer=https://www.arcgis.com' \
-d 'client=referer'
3. Make a request
Implementing user authentication successfully will grant a unique access token to your application every time a user signs in. The access token will inherit the privileges of the signed-in user's ArcGIS account, granting it access to all of the secure resources the user's account has access to.
ArcGIS APIs and SDKs
If you use user authentication with an ArcGIS Maps SDK, the Authentication
or Identity
classes automatically handle authentication with the access token, requiring no additional action from you.
The examples below show how to display a map in apps that implement user authentication.
function handleSignedIn() {
map = new Map({
basemap: "arcgis/topographic" // basemap styles service
});
const view = new MapView({
map: map,
center: [-118.805, 34.027], // Longitude, latitude
zoom: 13, // Zoom level
container: "viewDiv" // Div element
});
}
ArcGIS REST APIs
If you implement user authentication manually, your application can include the access token in requests to REST APIs by setting the token
parameter.
This example shows how to geocode an address with the geocoding service.
curl https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer/findAddressCandidates \
-d "f=pjson" \
-d "address=1600 Pennsylvania Ave NW, DC" \
-d "token=<YOUR_ACCESS_TOKEN>"
Tutorials
Create OAuth credentials for user authentication
Sign in with user authentication
Create an application that requires users to sign in with an ArcGIS account.