What is a buffer analysis?
A buffer analysis is the process of creating an area at a distance around a point, line, or polygon feature. To execute the analysis, use the spatial analysis service and the Create
operation.
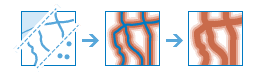
Real-world examples of this analysis include the following:
- Creating a buffer around schools.
- Creating a buffer around transit lines.
- Generating a buffer around points of interest.
After calculating a buffer area, you can use the resulting polygon for further analysis to answer questions such as: how many points of interest are within a mile of a specific location, or what buildings are within 500 feet of a school?
How to create a buffer
The general steps to create a buffer are as follows:
- Review the parameters for the
Create
operation.Buffers - Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https
:// <YOUR _ANALYSIS _SERVICE >/arcgis/rest/services/tasks/ GP Server/ Create Buffers/submit Job - Parameters:
input
: Your points dataset as a hosted feature layer or feature collection.Layer distances
orfield
: The value containing the buffer distance. Usedistances
if you want multiple distances with which to calculate buffers.output
: A string representing the name of the hosted feature layer to return with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
http://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/CreateBuffers/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token |
An access token (API key or OAuth 2.0) with the required Spatial Analysis > Feature analysis privilege. | token= |
input | The point, line, or polygon features to be buffered. | {"url" |
distances | If not using field . The value with which the buffer will be calculated. | 1 |
field | If not using distances . A field within the input containing a buffer distance. | ATTRIBUTE |
Key parameters
Name | Description | Examples |
---|---|---|
units | The unit to be used with the specified distance values. | Meters , Kilometers , Miles |
dissolve | Determines whether overlapping buffers are split, dissolved, or kept. | None , Dissolve , Split |
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
context | A bounding box or output spatial reference for the analysis. | "extent" |
Code examples
Schools (points)
This example uses the Create
operation to create areas 1000 feet around schools.
APIs
input_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/DeriveHighSchools/FeatureServer"
results = create_buffers(
input_layer=input_layer,
dissolve_type=None,
distances=[1000],
units="Feet",
ring_type="Rings",
# Outputs results as a hosted feature layer.
output_name="Schools buffer results PYTHON",
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Bike routes (lines)
This example uses the Create
operation to create areas 200 feet around bike routes.
APIs
input_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Portland%20Bike%20Routes/FeatureServer/0"
results = create_buffers(
input_layer=input_layer,
dissolve_type="Dissolve",
distances=[200],
units="Feet",
ring_type="Rings",
context={
"extent": {
"xmin": -13727534.4477,
"ymin": 5604395.5062,
"xmax": -13547856.7377,
"ymax": 5741928.7477,
"spatialReference": {"wkid": 102100},
}
},
# Outputs results as a hosted feature layer.
output_name="Create buffer results",
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Recreational parcels (polygons)
This example uses the Create
operation to create areas one mile around recreational parcels in the Santa Monica Mountains Parcels hosted feature layer.
APIs
input_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Santa_Monica_Mountains_Parcels/FeatureServer/0/query?where=UseType='Recreational'"
results = create_buffers(
input_layer=input_layer,
dissolve_type="Dissolve",
distances=[1],
units="Miles",
ring_type="Rings",
context={
"extent": {
"xmin": -13241162.787695415,
"ymin": 4029393.2145536076,
"xmax": -13208887.252502043,
"ymax": 4049992.993676435,
"spatialReference": {"wkid": 102100},
}
},
# Outputs results as a hosted feature layer.
output_name="Create buffer results",
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}