What is a find centroid analysis?
A find centroid analysis is the process of finding the representative centers of each multipoint, polyline, or polygon feature. To execute the analysis, use the spatial analysis service and the Find
operation.
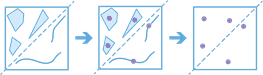
Real-world examples of this analysis include the following:
- Preparing home or spending values for analyses that require point data.
- Finding the representative centers of parcels.
- Finding the representative centers for open spaces.
How to find centroids
The general steps to find centroids are as follows:
- Review the parameters for the
Find
operation.Centroids - Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https
:// <YOUR _ANALYSIS _SERVICE >/arcgis/rest/services/tasks/ GP Server/ Find Centroids/submit Job - Parameters:
input
: Your points dataset as a hosted feature layer or feature collection.Layer point
: Whether the output point locations are calculated by the geometric center of each input feature.Location output
: A string representing the name of the hosted feature layer to return with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
http://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/FindCentroids/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token |
An access token (API key or OAuth 2.0) with the required Spatial Analysis > Feature analysis privilege. | token= |
input | The multipoint, line, or polygon features that will be used to generate centroid point features. | {"url" |
Key parameters
Name | Description | Examples |
---|---|---|
point | Output points are determined by the calculated geometric center of each input feature. If set to true the points will be contained by the bounds of the input feature. | false (default) |
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
context | A bounding box or output spatial reference for the analysis. | "extent" |
Code examples
Find centroids within square bins
This example uses the Find
operation to generate points within square bins. The input
value is the Generate tessellations in Portland hosted feature layer. The feature layer was created using the Generate
operation. To learn how to generate square bins, go to generate tessellations.
APIs
inputLayer = {
"url": "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Generate_tessellations_on_Portland_boundary/FeatureServer/0"
}
results = find_centroids(
input_layer=inputLayer,
point_location=False,
#Outputs results as a hosted feature layer.
output_name=f"Find centroids results"
)
result_features = results.layers[0].query()
print(
f"The find centroids layer has {len(result_features.features)} new records"
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Find centroids for census blocks
This example uses the Find
operation to generate points that represent census blocks. The input
value is the Census blocks in SF hosted feature layer.
APIs
inputLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Intersect_of_U_S__Census_Blocks_and_SF_Neighborhoods/FeatureServer/0"
results = find_centroids(
input_layer=inputLayer,
point_location=False,
#Outputs results as a hosted feature layer.
output_name=f"Find centroids results"
)
result_features = results.layers[0].query()
print(
f"The find centroids layer has {len(result_features.features)} new records"
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}