What is a find existing locations analysis?
A find existing location analysis is the process of selecting and copying features that satisfy one or more SQL and/or spatial expressions. To execute the analysis, use the spatial analysis service and the Find
operation.
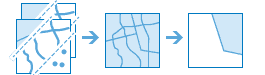
Real-world examples of this analysis include the following:
- Finding neighborhoods within a specified distance from schools or police stations.
- Selecting specific points of interest within a larger dataset.
- Finding road access that cannot be on or within view of a protected area.
How to perform a find location analysis
The general steps to performing a find existing locations analysis are as follows:
- Review the parameters for the
Find
operation.Existing Locations - Determine if you would like the results as a feature collection (dynamic) or hosted feature layer (stored).
- Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https
:// <YOUR _ANALYSIS _SERVICE >/arcgis/rest/services/tasks/ GP Server/ Find Existing Locations/submit Job - Parameters:
expressions
: The SQL or spatial relation to select a subset of the data.input
: The feature collection(s) or hosted feature layer(s) from which you want to extract features.Layers output
: A string representing the name of the hosted feature layer to return with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
http://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/FindExistingLocations/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An OAuth 2.0 access token. | token= |
input | The point, polyline, or polygon feature data that will be used to construct the query. | [{"url" |
expressions | The SQL or spatial query. | [{"operator" |
Key parameters
Name | Description | Examples |
---|---|---|
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
context | A bounding box or output spatial reference for the analysis. | "extent" |
Code examples
Find neighborhoods (spatial query)
This example uses the Find
operation to construct a spatial query that selects and returns all the neighborhoods within a half mile of a police station.
For the analysis, the input
value is the Police stations and San Francisco Neighborhoods hosted feature layers. The expression
value is: S
.
APIs
police_stations = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Police_Stations/FeatureServer/0"
neighborhoods = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/SF_Neighborhoods/FeatureServer/0"
results = find_existing_locations(
input_layers=[neighborhoods, police_stations],
expressions=[
{
"operator": "",
"layer": 0,
"selectingLayer": 1,
"spatialRel": "withinDistance",
"distance": 0.5,
"units": "Miles",
}
],
#Outputs results as a hosted service.
output_name="Find locations results"
)
result_features = results.layers[0].query()
print(
f"Find existing features returned {len(result_features.features)} new records"
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Find hotels (SQL query)
This example uses the Find
operation to construct a SQL query that selects and returns the restaurants within the OpenStreetMap-Points of interest (bèta) hosted layer.
For the analysis, the input
value is the OpenStreetMap-Points of interest (bèta) hosted layer. The expression
value is: "where"
.
To run this example you need an access token with the following privileges:
portal
:user :create Item portal
:publisher :publish Features premium
:user :spatialanalysis
To learn more about privileges, go to Security and authentication guide > Privileges
APIs
osm_points_of_interest = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/OpenStreetMap - Points of interest/FeatureServer/0"
results = find_existing_locations(
input_layers=[osm_points_of_interest],
expressions=[
{"operator": "", "layer": 0, "where": "POI-laag = 'Hotels en Pensions'"}
],
#Outputs results as a hosted feature layer.
output_name="Find locations results",
context={
"extent": {
"xmin": 568547.4926900844,
"ymin": 6816058.336549545,
"xmax": 570749.8345676091,
"ymax": 6817610.963686628,
"spatialReference": {"wkid": 102100, "latestWkid": 3857},
}
},
)
result_features = results.layers[0].query()
print(
f"Find existing features returned {len(result_features.features)} new records"
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}