Learn how to change a basemap style in a map using the Basemap styles service.
The basemap styles service provides a number of basemap layer styles such as topography, streets, outdoor, navigation, and imagery that you can use in maps.
In this tutorial, you use the layers
control to toggle between a number of different basemap styles.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
To learn about the other types of authentication available, go to Types of authentication.
Remove the basemap code
-
Remove the
basemap
andEnum vector
references contained in the .Basemap Layer Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
Create a basemap style function
Create a function that accepts a basemap style enumeration and returns the corresponding basemap style
-
Define a new
get
function that accepts a style enumeration and returns aV2 Basemap Vector
. SetBasemap Layer version
to access the styles service, and include your API key to validate the call.:2 Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; function getV2Basemap(style) { return L.esri.Vector.vectorBasemapLayer(style, { token: accessToken, version:2 }) }
Add the basemap styles
Reference the additional basemap styles you would like to use in your application.
-
Create a
basemap
object that contains the labels for each basemap layer enumeration. For each enumeration, call theLayers get
function to populateV2 Basemap basemap
.Layers Use dark colors for code blocks function getV2Basemap(style) { return L.esri.Vector.vectorBasemapLayer(style, { token: accessToken, version:2 }) } const basemapLayers = { "arcgis/outdoor": getV2Basemap("arcgis/outdoor").addTo(map), "arcgis/community": getV2Basemap("arcgis/community"), "arcgis/navigation": getV2Basemap("arcgis/navigation"), "arcgis/streets": getV2Basemap("arcgis/streets"), "arcgis/streets-relief": getV2Basemap("arcgis/streets-relief"), "arcgis/imagery": getV2Basemap("arcgis/imagery"), "arcgis/oceans": getV2Basemap("arcgis/oceans"), "arcgis/topographic": getV2Basemap("arcgis/topographic"), "arcgis/light-gray": getV2Basemap("arcgis/light-gray"), "arcgis/dark-gray": getV2Basemap("arcgis/dark-gray"), "arcgis/human-geography": getV2Basemap("arcgis/human-geography"), "arcgis/charted-territory": getV2Basemap("arcgis/charted-territory"), "arcgis/nova": getV2Basemap("arcgis/nova"), "osm/standard": getV2Basemap("osm/standard"), "osm/navigation": getV2Basemap("osm/navigation"), "osm/streets": getV2Basemap("osm/streets"), "osm/blueprint": getV2Basemap("osm/blueprint") };
-
Append
add
to theTo arcgis/outdoor
entry so that it is the default style when the application loads.Use dark colors for code blocks function getV2Basemap(style) { return L.esri.Vector.vectorBasemapLayer(style, { token: accessToken, version:2 }) } const basemapLayers = { "arcgis/outdoor": getV2Basemap("arcgis/outdoor").addTo(map), "arcgis/community": getV2Basemap("arcgis/community"), "arcgis/navigation": getV2Basemap("arcgis/navigation"), "arcgis/streets": getV2Basemap("arcgis/streets"), "arcgis/streets-relief": getV2Basemap("arcgis/streets-relief"), "arcgis/imagery": getV2Basemap("arcgis/imagery"), "arcgis/oceans": getV2Basemap("arcgis/oceans"), "arcgis/topographic": getV2Basemap("arcgis/topographic"), "arcgis/light-gray": getV2Basemap("arcgis/light-gray"), "arcgis/dark-gray": getV2Basemap("arcgis/dark-gray"), "arcgis/human-geography": getV2Basemap("arcgis/human-geography"), "arcgis/charted-territory": getV2Basemap("arcgis/charted-territory"), "arcgis/nova": getV2Basemap("arcgis/nova"), "osm/standard": getV2Basemap("osm/standard"), "osm/navigation": getV2Basemap("osm/navigation"), "osm/streets": getV2Basemap("osm/streets"), "osm/blueprint": getV2Basemap("osm/blueprint") };
-
Create a
Layers
control that referencesbasemap
and add it to your map.Layers Use dark colors for code blocks const basemapLayers = { "arcgis/outdoor": getV2Basemap("arcgis/outdoor").addTo(map), "arcgis/community": getV2Basemap("arcgis/community"), "arcgis/navigation": getV2Basemap("arcgis/navigation"), "arcgis/streets": getV2Basemap("arcgis/streets"), "arcgis/streets-relief": getV2Basemap("arcgis/streets-relief"), "arcgis/imagery": getV2Basemap("arcgis/imagery"), "arcgis/oceans": getV2Basemap("arcgis/oceans"), "arcgis/topographic": getV2Basemap("arcgis/topographic"), "arcgis/light-gray": getV2Basemap("arcgis/light-gray"), "arcgis/dark-gray": getV2Basemap("arcgis/dark-gray"), "arcgis/human-geography": getV2Basemap("arcgis/human-geography"), "arcgis/charted-territory": getV2Basemap("arcgis/charted-territory"), "arcgis/nova": getV2Basemap("arcgis/nova"), "osm/standard": getV2Basemap("osm/standard"), "osm/navigation": getV2Basemap("osm/navigation"), "osm/streets": getV2Basemap("osm/streets"), "osm/blueprint": getV2Basemap("osm/blueprint") }; L.control.layers(basemapLayers, null, { collapsed: false }).addTo(map);
Run the app
In CodePen, run your code to display the map.
Use the layers control in the top right to select and explore different basemap layer styles from the Basemap styles service.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:

Change the basemap style
Switch a basemap style in a map using the basemap styles service.

Change the place label language
Switch the language of place labels on a basemap.
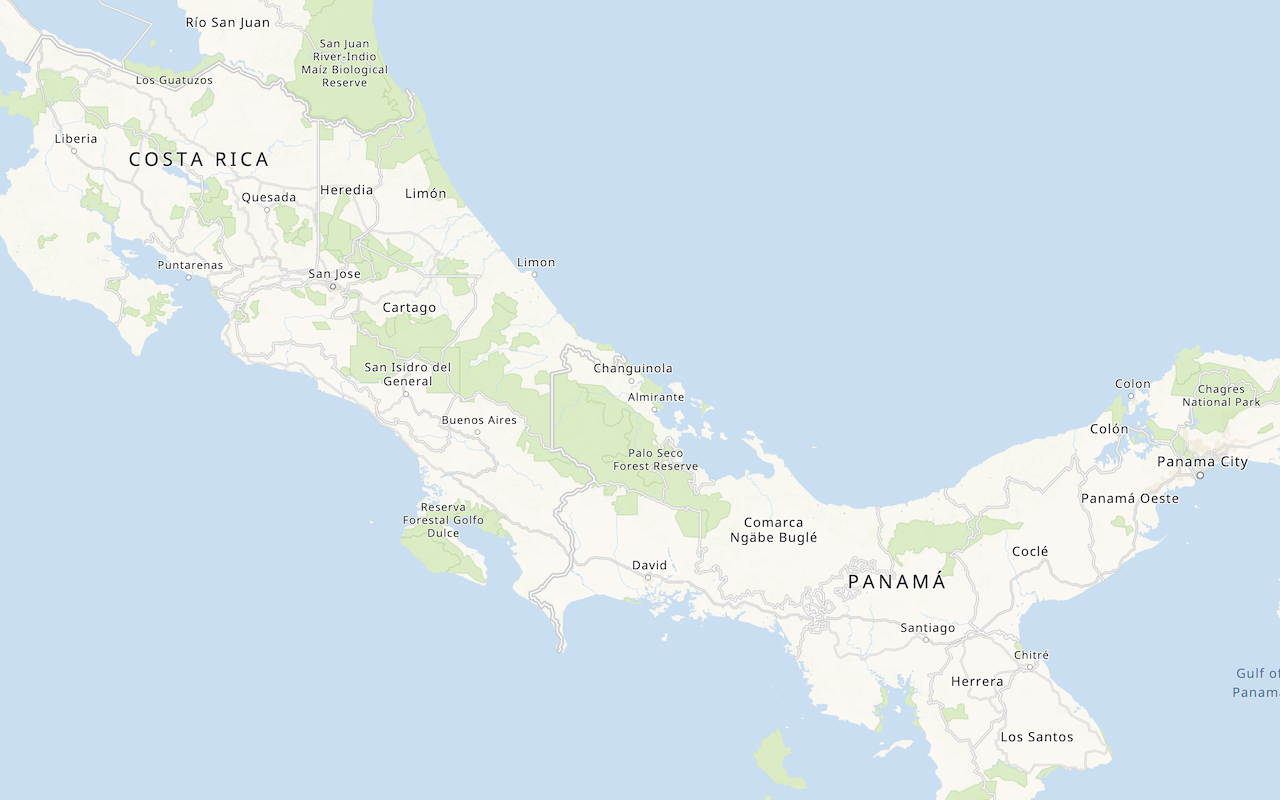
Display a custom basemap style
Add a styled vector basemap layer to a map.
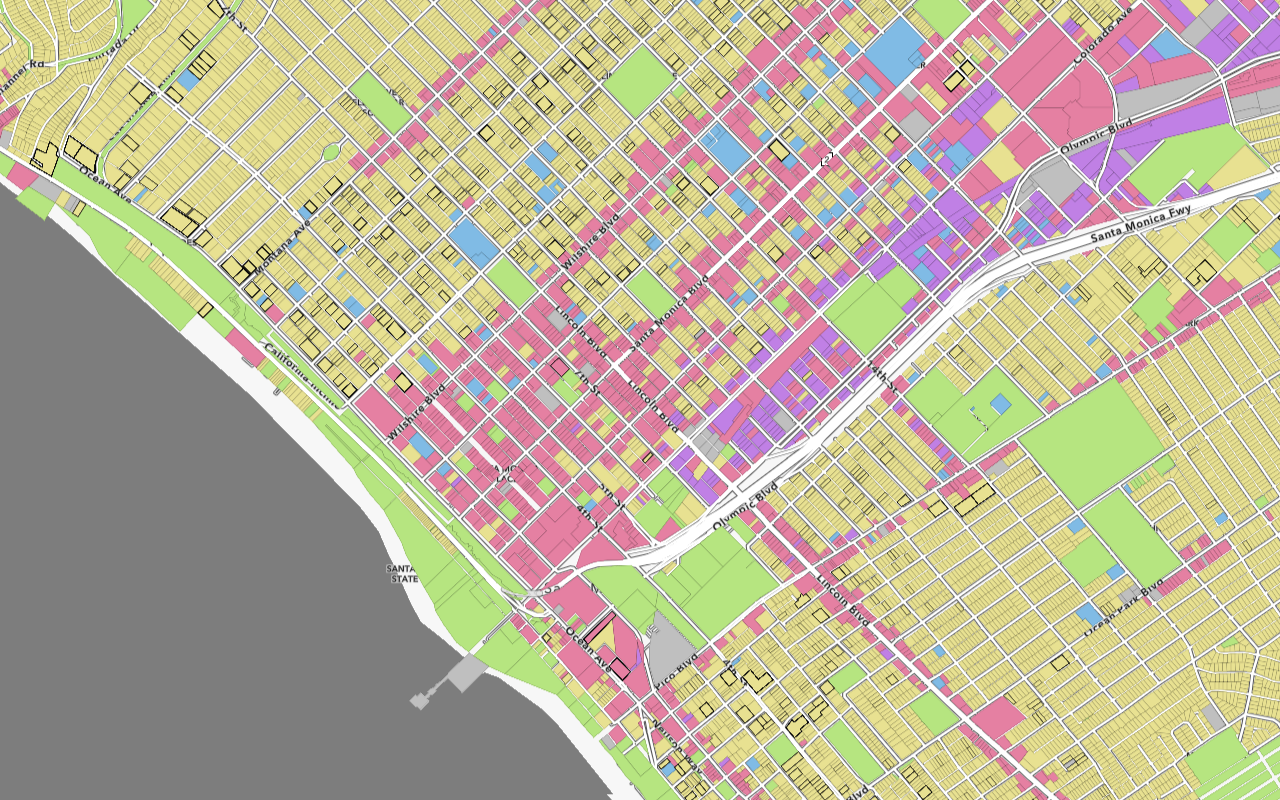
Style vector tiles
Change the fill and outline of vector tiles based on their attributes.