Learn how to use Esri Leaflet to display a map.
You can display a map with Esri Leaflet by using a vector tile basemap layer from the basemap styles service. A vector tile basemap layer contains the styles, layers, font glyphs, and icons to render the layers.
In this tutorial, you display a map of the Santa Monica Mountains using the streets basemap layer from the Basemap styles service. The code is used as the starting point for the other Esri Leaflet tutorials.
Prerequisites
An ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new app
Select a type of authentication below and follow the steps to create a new application.
Set up authentication
Create developer credentials in your portal for the type of authentication you selected.
Set developer credentials
Use the API key or OAuth developer credentials created in the previous step in your application.
Add script references
To access vector basemap layers, you need to reference the leaflet
libraries as well as the esri-leaflet
and esri-leaflet-vector
plugins.
-
In the index.html file, add the following
<link
and> <script
references if they are missing.> Use dark colors for code blocks <head> <meta charset="utf-8" /> <meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no" /> <title>Esri Leaflet Tutorials: Display a map</title> <!-- Load Leaflet from CDN --> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" /> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script> <!-- Load Esri Leaflet from CDN --> <script src="https://unpkg.com/esri-leaflet@3.0.14/dist/esri-leaflet.js"></script> <script src="https://unpkg.com/esri-leaflet-vector@4.2.7/dist/esri-leaflet-vector.js"></script>
Create a map
Use a map
to add a map to the div
element with the basemap you specify. To find the list of default basemap styles, go to Basemap styles service.
-
In the
<script
tag, create a> map
with themin
set toZoom 2
.Use dark colors for code blocks const map = L.map("map", { minZoom: 2 })
-
Call the
set
method to center the map on the coordinatesView 34.02, -118.805
and to set the zoom level to13
.Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13);
-
Create a
basemap
variable to store the basemap identifier,Enum arcgis/streets
.Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const basemapEnum = "arcgis/streets";
-
Instantiate the
vector
class and set theBasemap Layer basemap
andEnum access
before adding the layer to theToken map
.Use dark colors for code blocks const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
Run the app
Run the app.
The map should display a street basemap layer centered on Point Dume in California.What's next?
Learn how to use additional ArcGIS location services in these tutorials:
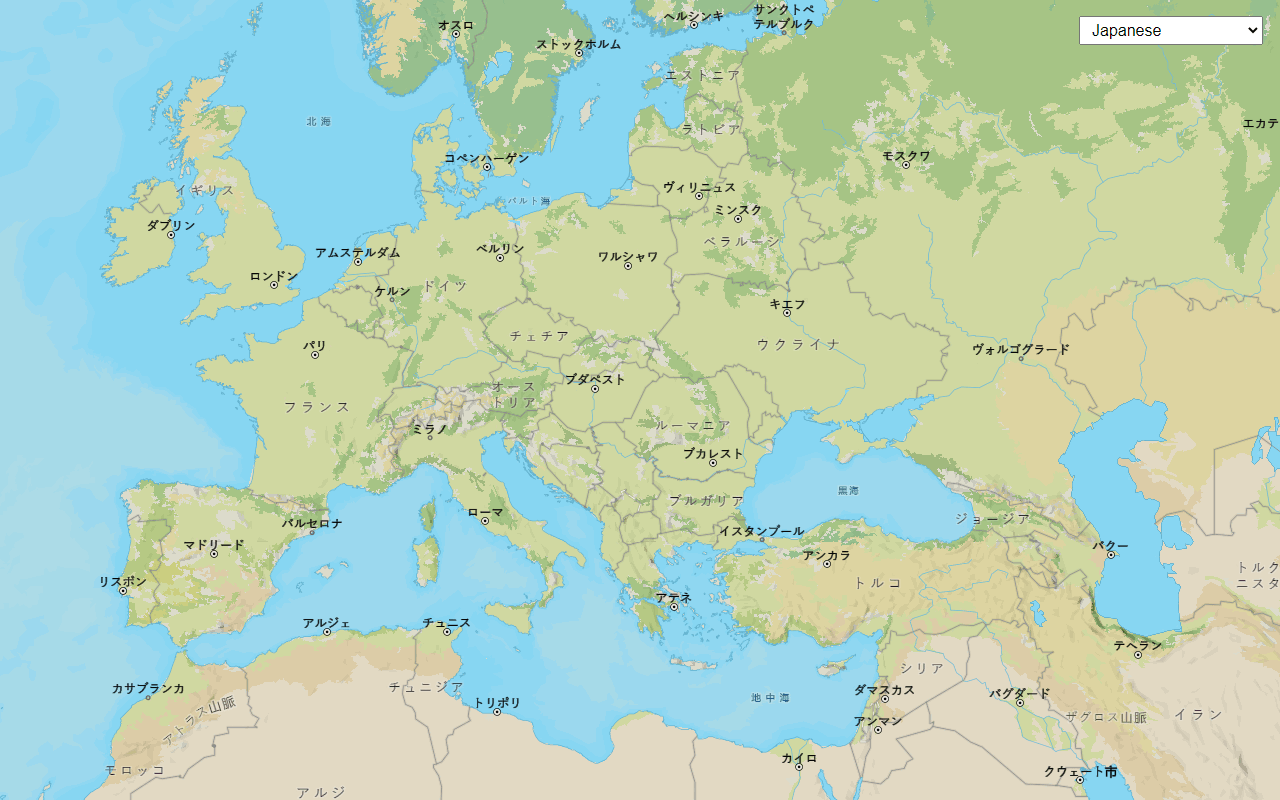
Change language labels for basemap styles
Switch the language of place labels on a basemap.
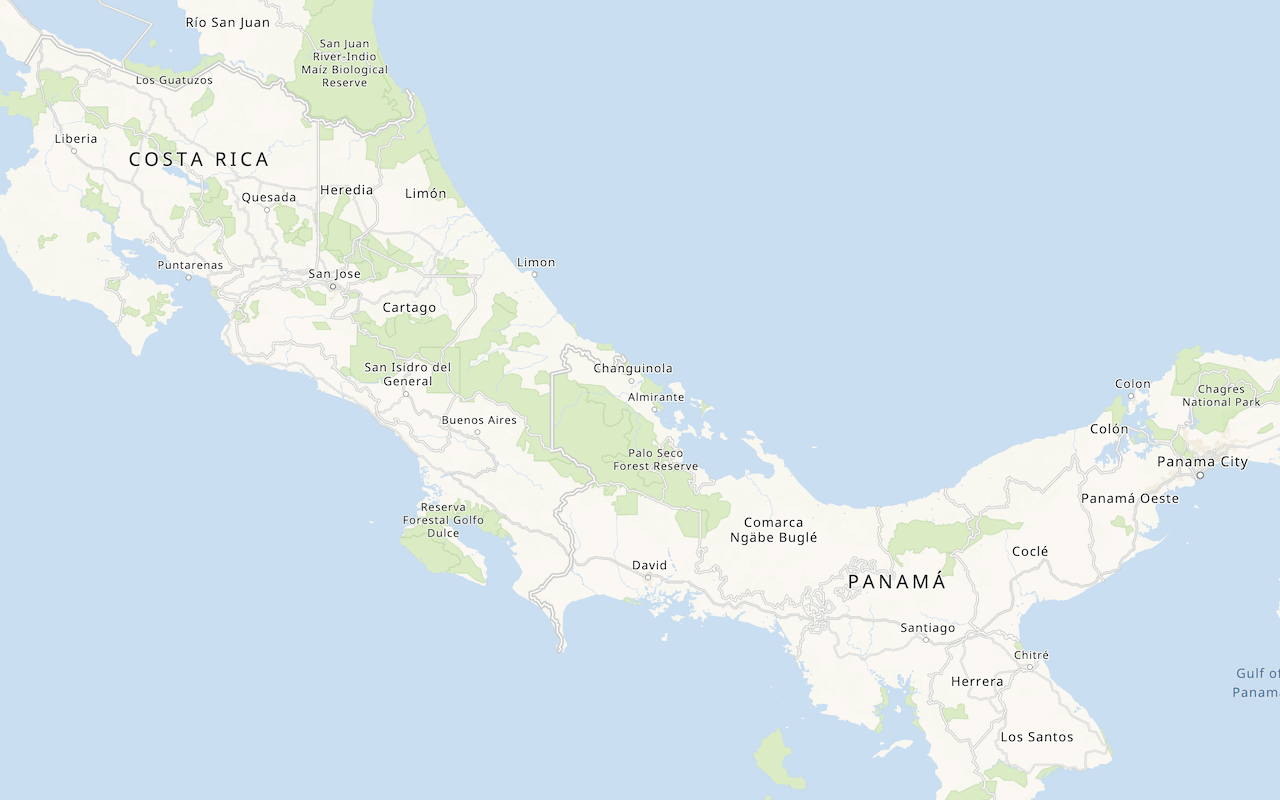
Display a custom basemap style
Add a styled vector basemap layer to a map.
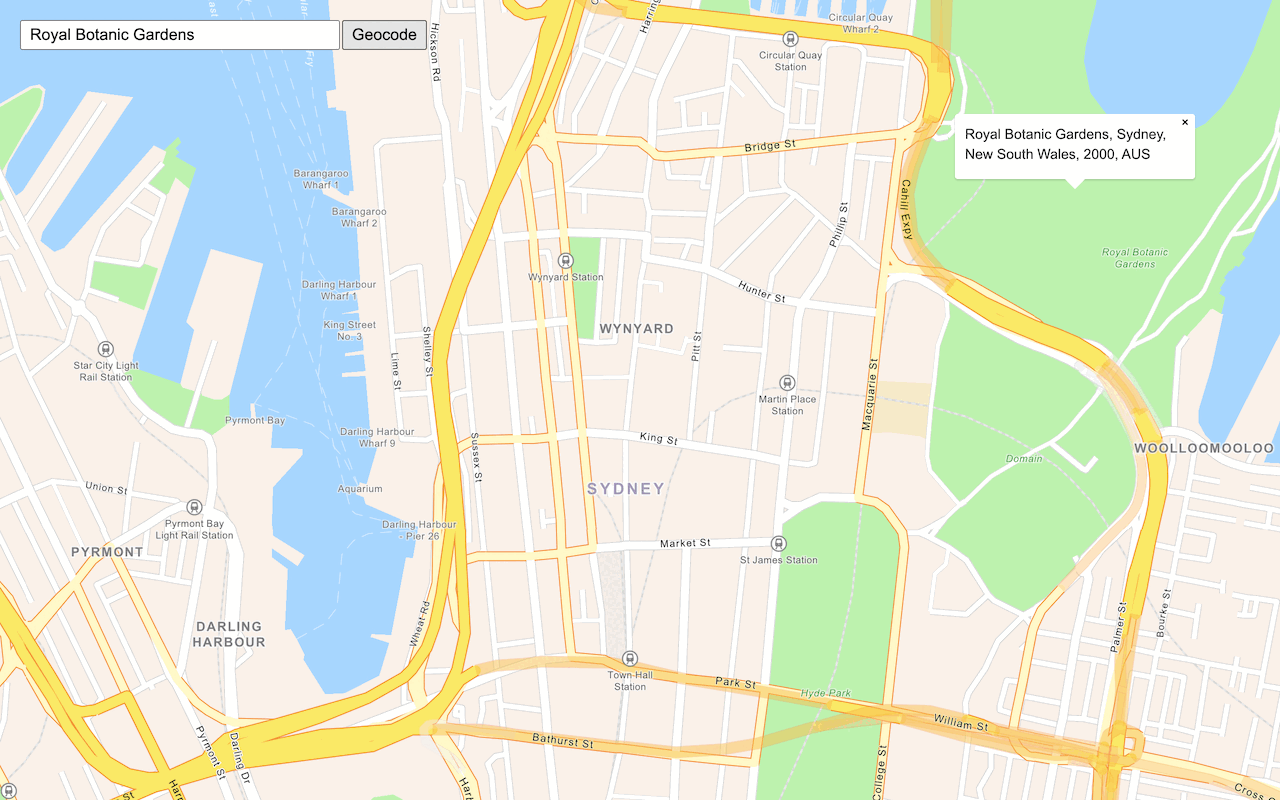
Search for an address
Find an address or place using a search box and the geocoding service.
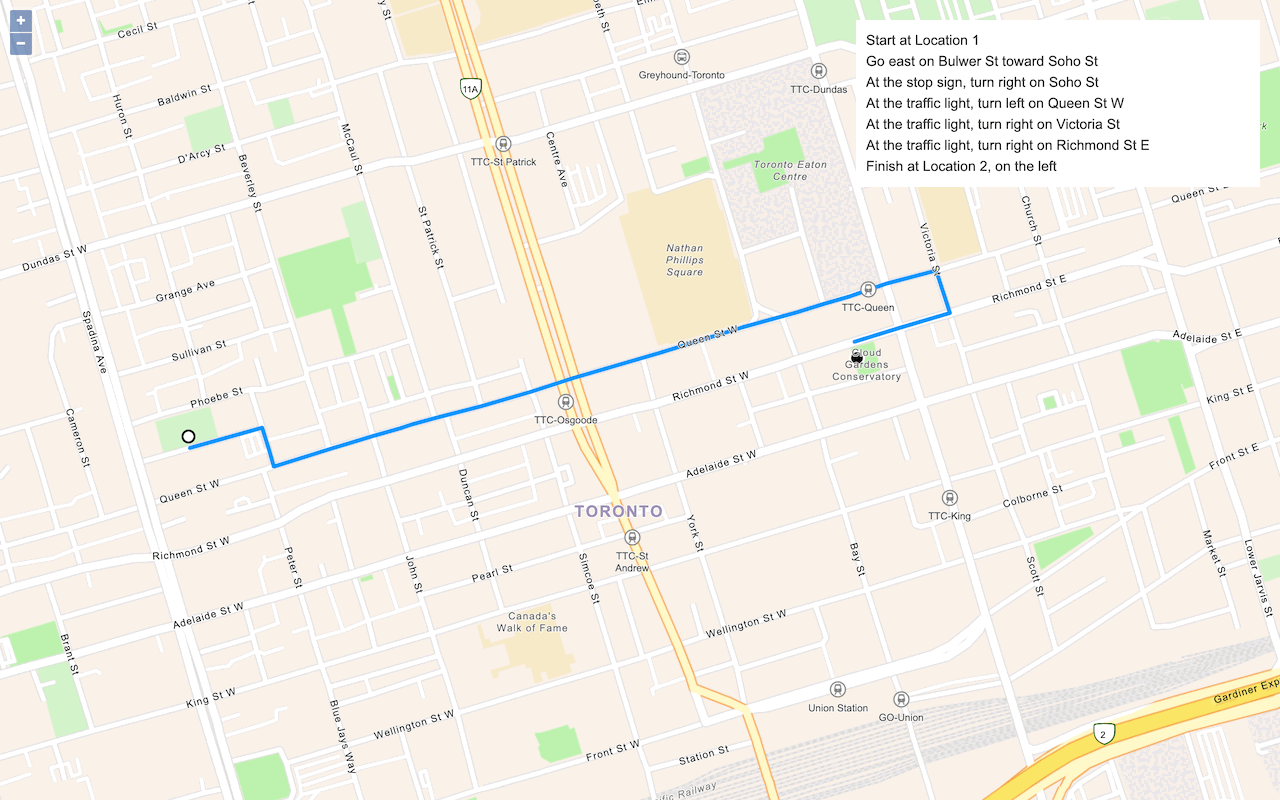
Find a route and directions
Find a route and directions with the route service.