Overlay combines two DataFrames into a single DataFrame using one of five overlay types: Intersect, Erase, Union, Identity, or Symmetric Difference.
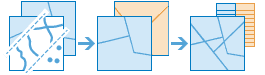
Usage notes
-
Overlay requires that two inputs are specified using the
input
and_dataframe overlay
parameters in the_dataframe run()
method. The two supported overlay types and input geometries are described in the following tables:Intersect Erase Union Identity Symmetric Difference Point and point Point and linestring Point and polygon Linestring and point Linestring and linestring Linestring and polygon Polygon and point Polygon and linestring Polygon and polygon Full supportPartial supportNo support -
The following table summarizes the overlay operations:
Overlay type Description Intersect
The geometries or portions of geometries in the overlay DataFrame that overlap the input DataFrame are returned. This is the default. Erase
The geometries or portions of geometries in the input DataFrame that do not overlap the overlay DataFrame are returned. Union
The result will contain a geometric union of the input DataFrame and overlay DataFrame. All geometries and attributes will be returned. Identity
The result will contain geometries or portions of geometries that overlap in both the input DataFrame and overlay DataFrame. Symmetric Difference
The result will contain geometries or portions of geometries of the input DataFrame and the overlay DataFrame that do not overlap. -
When applying the indentity, intersect, symmetric difference, or union overlay types, fields from both DataFrames (
input
and_dataframe overlay
) are in the result. If the inputs have the same field names, the overlay fields will be renamed_dataframe fieldname
. For example, if your_overlay input
had the fields_dataframe county
andstate
, and theoverlay
had the field_dataframe county
, your output DataFrame would have the fieldscounty
,state
, andcounty
._overlay
Limitations
Sliver results may be excluded based on the tolerance of the processing spatial reference.
Results
The tool outputs records that include the following fields in addition to the input
fields:
Field | Description |
---|---|
overlay | If your input had a geometry column, a new field named geometry is created. |
Fields from the overlay | When you use identity, intersect, symmetric difference, or union overlay type. |
Performance notes
Improve the performance of Overlay by doing one or more of the following:
-
Only analyze the records in your area of interest. You can pick the records of interest by using one of the following SQL functions:
- ST_Intersection—Clip to an area of interest represented by a polygon. This will modify your input records.
- ST_BboxIntersects—Select records that intersect an envelope.
- ST_EnvIntersects—Select records having an evelope that intersects the envelope of another geometry.
- ST_Intersects—Select records that intersect another dataset or area of intersect represented by a polygon.
Similar capabilities
Use the Spatiotemporal join tool if you want to join two DataFrames based on their spatial relationship.
The following functions complete spatial overlay operations:
- ST_Aggr_Intersection
- ST_Aggr_union
- ST_Contains
- ST_Crosses
- ST_Difference
- ST_Dwithin
- ST_Equals
- ST_Intersection
- ST_Intersects
- ST_Overlaps
- ST_SymDifference
- ST_Touches
- ST_Union
- ST_Within
Syntax
For more details, go to the GeoAnalytics Engine API reference for overlay.
Setter | Description | Required |
---|---|---|
run(input | Runs the Overlay tool using the provided DataFrames. | Yes |
set | Sets the type of overlay to be performed. The default is intersect. | No |
Examples
Run Overlay
# Log in
import geoanalytics
geoanalytics.auth(username="myusername", password="mypassword")
# Imports
from geoanalytics.tools import Overlay
from geoanalytics.sql import functions as ST
from pyspark.sql import functions as F
# Paths to development area and planned pathway datasets
development_path="https://services.arcgis.com/P3ePLMYs2RVChkJx/ArcGIS/rest/" \
"/services/DevelopmentProjectArea/FeatureServer/0"
pathway_path="https://services.arcgis.com/P3ePLMYs2RVChkJx/ArcGIS/rest/" \
"services/DevA_Pathways/FeatureServer/1"
# Create a DataFrame for the development areas and pathways
development_df=spark.read.format("feature-service").load(development_path)
pathway_df=spark.read.format("feature-service").load(pathway_path)
# Use Overlay to union the pathways with the development area.
# The result will be a DataFrame containing the development area enhanced with
# planned pathway geometries and information
overlay_result=Overlay() \
.setOverlayType(overlay_type="Union") \
.run(input_dataframe=development_df, overlay_dataframe=pathway_df)
overlay_result.filter(overlay_result["shapeType"] == 'Street') \
.select("OBJECTID", "shapeType", F.round("Z_Min", 13).alias("Z_Min"), F.round("Z_Max", 13).alias("Z_Max")) \
.sort("Z_Max", "Z_Min", ascending=False) \
.show(5)
+--------+---------+----------------+----------------+
|OBJECTID|shapeType| Z_Min| Z_Max|
+--------+---------+----------------+----------------+
| 1| Street|50.1885900000052|52.4717399999936|
| 1| Street|42.6825300000055|52.4717399999936|
| 1| Street|35.4103199999954|50.1885900000052|
| 1| Street|47.8472699999984|48.1978600000002|
| 1| Street|34.7740400000039|48.1978600000002|
+--------+---------+----------------+----------------+
only showing top 5 rows
Plot results
# Plot the resulting development and pathway areas
result_plot=overlay_result.st.plot(facecolor="lightblue",
edgecolors="navy",
figsize=(16,10),
basemap="light")
result_plot.set_title("Development area (Multnomah, Oregon) enhanced with" \
"information for planned pathways")
result_plot.set_xlabel("X (Meters)")
result_plot.set_ylabel("Y (Meters)")
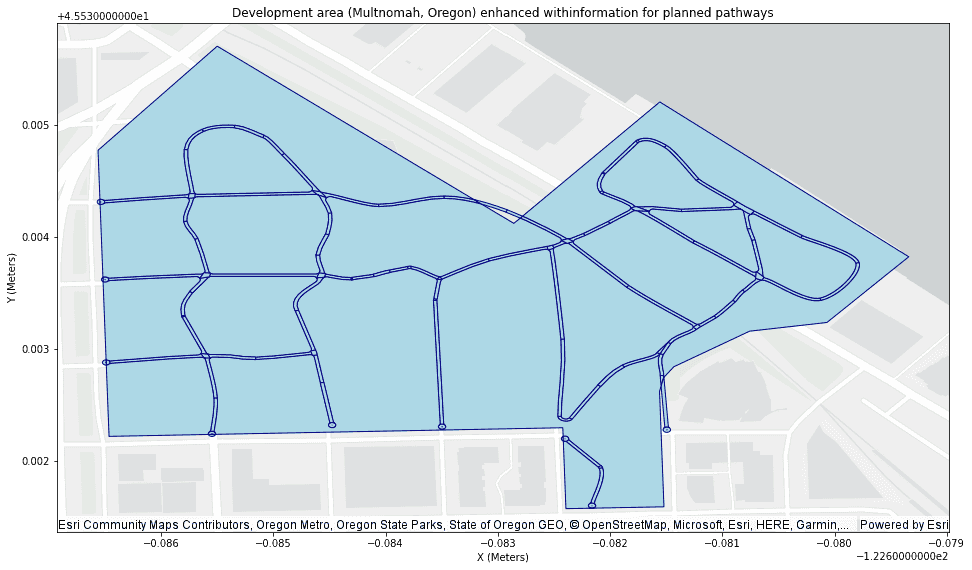
Version table
Release | Notes |
---|---|
1.0.0 | Python tool introduced |