Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials.
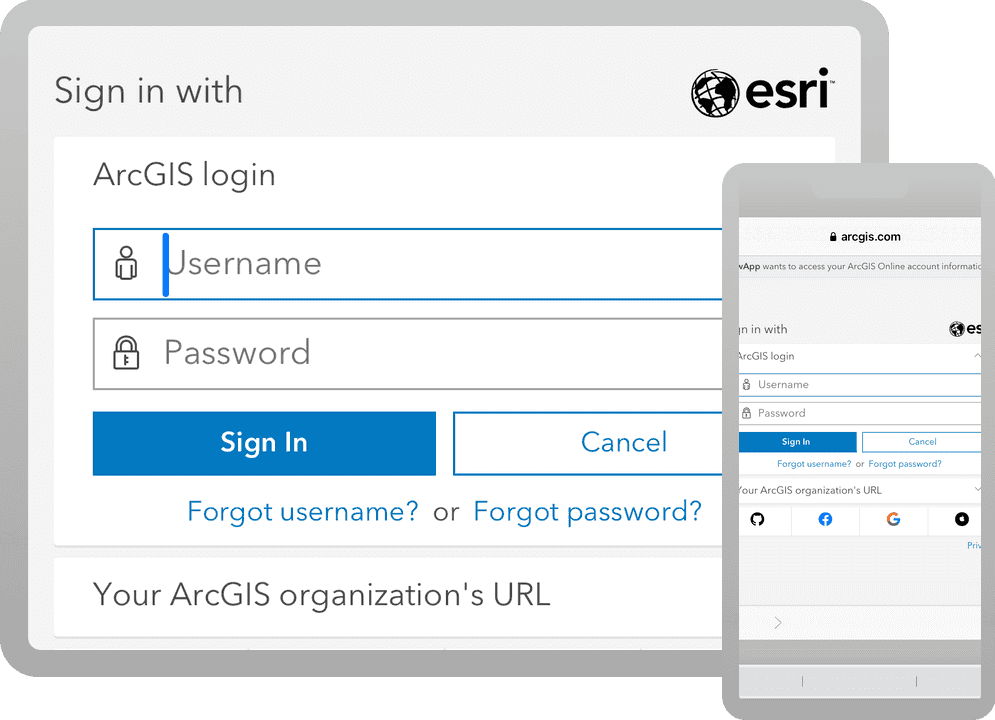
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
Steps
Create OAuth credentials
OAuth credentials are required to implement user authentication. These credentials are created as an Application item in your organization's portal.
-
Sign in to your portal.
-
Click Content > My content > New item and select Developer credentials.
-
In the Create developer credentials window, select OAuth 2.0 credentials radio button and click Next.
-
Add a Redirect URL to your OAuth credentials:
my-app
. The remaining properties, Referrer URLs, Application environment and URL, can remain with their default values. Click Next.://auth -
For Privileges, click Next. Privileges are not required for this tutorial.
-
Click Skip to move past Grant item access as it is not required for this tutorial.
-
Provide a Title of your choice. Optionally, stipulate a Folder to store your Application item, add Tags, and add a Summary. Click Next.
-
Review your settings and go back to correct any errors. When you are ready, click Create. When the application item is created,
Client ID
,Client Secret
, andTemporary Token
values will also be generated. You will be redirected to the Application item's Overview page.
Client ID
and Redirect URL
when implementing OAuth in your app's code. The Client ID
is found on the Application item's Overview page, while the Redirect URL
is found on the Settings page.The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth sign in. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode.
Remove API key
An API Key access token is not required for this app because you are implementing user authentication using OAuth 2.0 protocol.
-
Delete the code that sets your API key.
AppDelegate.swiftUse dark colors for code blocks 16 17 19 20 21 22 23 24 25 26 27 29 30 31 32 33Remove line Remove line import UIKit import ArcGIS @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { AGSArcGISRuntimeEnvironment.apiKey = "YOUR_ACCESS_TOKEN" return true } }
Set the app settings
Create a new Swift file and define constants you'll need in the app.
-
Add a new Swift file to your Xcode project named
App
. You will use this file to hold configuration constants required by your app.Configuration - In Xcode's app menu, select File > New > File.
- Select
Swift File
from theSource
sub-menu. - Name the file
App
and ensure your app's target is checked.Configuration - Click create.
-
Add the following to AppConfiguration.swift. Change
"
to the Client ID obtained from the first step above. ChangeYOU R- AP P- CLIEN T- I D" "
to match your Redirect URL entry above.YOU R- AP P- REDIREC T- UR L" AppConfiguration.swiftUse dark colors for code blocks extension String { static let clientID = "YOUR-APP-CLIENT-ID" static var redirectURL = "YOUR-APP-REDIRECT-URL" static let keychainIdentifier = "\(Bundle.main.bundleIdentifier!).keychainIdentifier" } extension URL { static let trafficLayerURL = URL(string: "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer")! }
Add layer to map
Add an operational layer to the map and test run the app.
-
Open ViewController.swift and update the existing
setup
method to add the World Traffic layer to the map.Map() The World Traffic layer is a premium service on ArcGIS Online that requires authentication for access.
ViewController.swiftUse dark colors for code blocks let trafficLayer = AGSArcGISMapImageLayer(url: .trafficLayerURL) map.operationalLayers.add(trafficLayer)
-
Press <Command+R> to run the app.
Only the basemap displays in the map. The traffic layer will not load until you use the AGS
to log in with an authorized account.
Integrate OAuth credentials into your app
Add OAuth components to your app, including adding the Redirect URL
to the app's plist
file, and setting up AGS
.
-
Configure a redirect URL scheme for your app. Right-click on info.plist file in the Project Navigator and then select Open As > / Source Code. Edit the file just after the opening top-level
<dict
tag and add the following XML:> Be sure to use your exact bundle identifier for your app.
Use the scheme part of the Redirect URL you configured in the application definition, but without the path (everything before the
://
). This is how OAuth 2.0 in iOS is able to return information about the authentication process back to your app. Note these strings must match exactly.Info.plistUse dark colors for code blocks <key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleTypeRole</key> <string>Editor</string> <key>CFBundleURLName</key> <string>com.esri.access-services-with-oauth</string> <key>CFBundleURLSchemes</key> <array> <string>my-app</string> </array> </dict> </array>
-
Open AppDelegate.swift to setup the
AGS
in yourAuthentication Manager App
. Import the ArcGIS library.Delegate AppDelegate.swiftUse dark colors for code blocks import UIKit import ArcGIS
-
Add a new method to setup the authentication manager in
App
. This code creates a configuration with the parameters you assigned to your app inDelegate.swift App
and then assigns that configuration to theConfiguration AGS
. The credentials are also saved in the device's keychain.Authentication Manager To construct the required
redirect
, combine theURL url
andScheme url
from yourAuth Path App
separated withConfiguration ://
.AppDelegate.swiftUse dark colors for code blocks // MARK: - OAuth extension AppDelegate { private func setupOAuthManager() { // Initialize OAuth configuration with Client ID and Redirect URL. let config = AGSOAuthConfiguration(portalURL: nil, clientID: .clientID, redirectURL: .redirectURL) // Add OAuth configuration to authentication manager. AGSAuthenticationManager.shared() .oAuthConfigurations .add(config) // Enable auto-sync to keychain on the auth manager's credential cache. AGSAuthenticationManager.shared() .credentialCache .enableAutoSyncToKeychain(withIdentifier: .keychainIdentifier, accessGroup: nil, acrossDevices: false) } }
-
Add a call to
setup
from the application launch.O Auth Manager() AppDelegate.swiftUse dark colors for code blocks func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { setupOAuthManager() return true }
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: