Learn how to display point, line, and polygon graphics in a map.
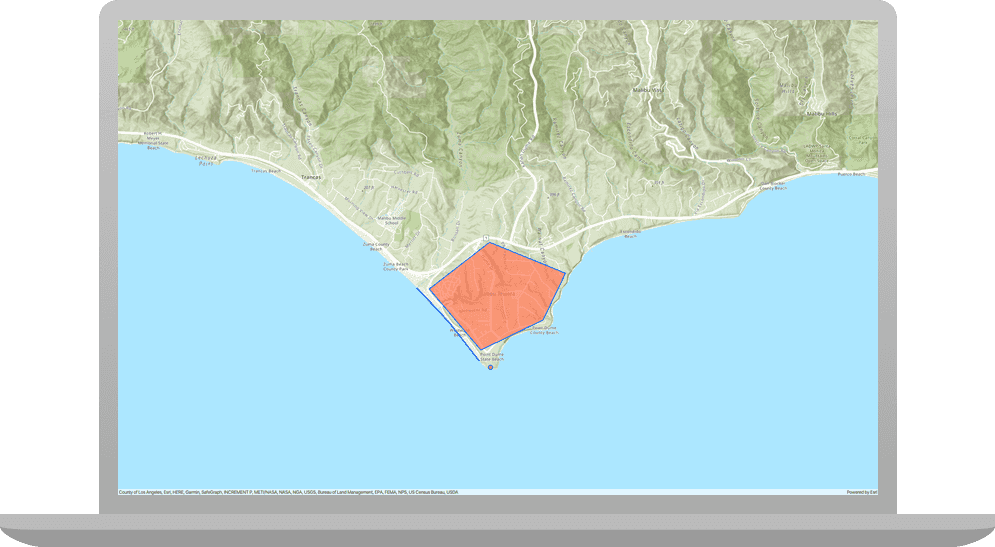
You typically use graphics to display geographic data that is not connected to a database and that is not persisted, like highlighting a route between two locations, displaying a search buffer around a point, or tracking the location of a vehicle in real-time. Graphics are composed of a geometry, symbol, and attributes.
In this tutorial, you display points, lines, and polygons on a map as graphics.
To learn how to display data from data sources, see the Add a feature layer tutorial.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the minimum system requirements.
-
An IDE for Java.
Steps
Open a Java project with Gradle
-
To start this tutorial, complete the Display a map tutorial, or download and unzip the Display a map solution into a new folder.
-
Open the build.gradle file as a project in IntelliJ IDEA.
-
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In the IntelliJ IDEA > Project tool window, open src/main/java/com.example.app and double-click App.
-
In the
start()
method, set the API key property on theArcGIS
with your access token. Replace YOUR_ACCESS_TOKEN with your copied access token. Be sure to surround your access token with double quotes as it is a string.Runtime Environment App.javaUse dark colors for code blocks ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN");
-
Add import statements
Add import statements to reference the ArcGIS Runtime API classes.
-
In the IntelliJ IDEA > Project tool window, open src/main/java/com.example.app and click App.
-
Add the following imports above the existing imports:
App.javaUse dark colors for code blocks 27 28 29 30 31Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. import com.esri.arcgisruntime.geometry.Point; import com.esri.arcgisruntime.geometry.PointCollection; import com.esri.arcgisruntime.geometry.Polygon; import com.esri.arcgisruntime.geometry.Polyline; import com.esri.arcgisruntime.geometry.SpatialReferences; import com.esri.arcgisruntime.mapping.Viewpoint; import com.esri.arcgisruntime.mapping.view.Graphic; import com.esri.arcgisruntime.mapping.view.GraphicsOverlay; import com.esri.arcgisruntime.symbology.SimpleFillSymbol; import com.esri.arcgisruntime.symbology.SimpleLineSymbol; import com.esri.arcgisruntime.symbology.SimpleMarkerSymbol; import com.esri.arcgisruntime.ArcGISRuntimeEnvironment; import com.esri.arcgisruntime.mapping.ArcGISMap; import com.esri.arcgisruntime.mapping.BasemapStyle; import com.esri.arcgisruntime.mapping.view.MapView;
Add a graphics overlay
A graphics overlay is a container for graphics. It is used with a map view to display graphics on a map. You can add more than one graphics overlay to a map view. Graphics overlays are displayed on top of all the other layers.
-
In the
start()
method, create a newGraphics
and add it to theOverlay map
.View App.javaUse dark colors for code blocks 68 69 70 71Add line. Add line. Add line. // set the map on the map view mapView.setMap(map); mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 72223.819286)); // create a graphics overlay and add it to the map view GraphicsOverlay graphicsOverlay = new GraphicsOverlay(); mapView.getGraphicsOverlays().add(graphicsOverlay);
Add a point graphic
A point graphic is created using a point and a marker symbol. A point is defined with x and y coordinates, and a spatial reference. For latitude and longitude coordinates, the spatial reference is WGS84.
-
Create a
Point
and aSimple
. To create theMarker Symbol Point
, provide longitude (x) and latitude (y) coordinates, and aSpatial
. Use theReference Spatial
convenience getter.References.get Wgs84() App.javaUse dark colors for code blocks 72 73 74 75Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // create a graphics overlay and add it to the map view GraphicsOverlay graphicsOverlay = new GraphicsOverlay(); mapView.getGraphicsOverlays().add(graphicsOverlay); // create a point geometry with a location and spatial reference Point point = new Point(-118.80657463861, 34.0005930608889, SpatialReferences.getWgs84()); // create an opaque orange (0xFFFF5733) point symbol with a blue (0xFF0063FF) outline symbol SimpleMarkerSymbol simpleMarkerSymbol = new SimpleMarkerSymbol(SimpleMarkerSymbol.Style.CIRCLE, 0xFFFF5733, 10); SimpleLineSymbol blueOutlineSymbol = new SimpleLineSymbol(SimpleLineSymbol.Style.SOLID, 0xFF0063FF, 2); simpleMarkerSymbol.setOutline(blueOutlineSymbol);
-
Create a
Graphic
with thepoint
andsimple
. Display theMarker Symbol Graphic
by adding it to thegraphics
's graphics collection withOverlay get
.Graphics() Point graphics support a number of symbol types such as
Simple
,Marker Symbol Picture
andMarker Symbol Text
. Learn more about the symbols in the API documentation.Symbol App.javaUse dark colors for code blocks 84 85Add line. Add line. Add line. Add line. Add line. simpleMarkerSymbol.setOutline(blueOutlineSymbol); // create a graphic with the point geometry and symbol Graphic pointGraphic = new Graphic(point, simpleMarkerSymbol); // add the point graphic to the graphics overlay graphicsOverlay.getGraphics().add(pointGraphic);
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, click run.
You should see a point graphic in Point Dume State Beach.
Add a line graphic
A line graphic is created using a polyline and a line symbol. A polyline is defined as a sequence of points.
Polylines have one or more distinct parts. Each part is a sequence of points. For a continuous line, you can use the Polyline
constructor to create a polyline with just one part. To create a polyline with more than one part, use a Polyline
.
-
Create a
Polyline
and aSimple
.Line Symbol To create the
Polyline
, create a newPoint
with aCollection Spatial
and add newReference Point
s to it.Polyline graphics support a number of symbol types such as
Simple
andLine Symbol Text
. Learn more about the symbols in the API documentation.Symbol App.javaUse dark colors for code blocks 89 90 91Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // add the point graphic to the graphics overlay graphicsOverlay.getGraphics().add(pointGraphic); // create a point collection with a spatial reference, and add three points to it PointCollection polylinePoints = new PointCollection(SpatialReferences.getWgs84()); polylinePoints.add(new Point(-118.821527826096, 34.0139576938577)); polylinePoints.add(new Point(-118.814893761649, 34.0080602407843)); polylinePoints.add(new Point(-118.808878330345, 34.0016642996246)); // create a polyline geometry from the point collection Polyline polyline = new Polyline(polylinePoints); // create a blue line symbol for the polyline SimpleLineSymbol polylineSymbol = new SimpleLineSymbol(SimpleLineSymbol.Style.SOLID, 0xFF0063FF, 3);
-
Create a
Graphic
with thepolyline
andpolyline
. Display theSymbol Graphic
by adding it to thegraphics
's graphics collection withOverlay get
.Graphics() App.javaUse dark colors for code blocks 100 101 102 103Add line. Add line. Add line. Add line. Add line. // create a blue line symbol for the polyline SimpleLineSymbol polylineSymbol = new SimpleLineSymbol(SimpleLineSymbol.Style.SOLID, 0xFF0063FF, 3); // create a polyline graphic with the polyline geometry and symbol Graphic polylineGraphic = new Graphic(polyline, polylineSymbol); // add the polyline graphic to the graphics overlay graphicsOverlay.getGraphics().add(polylineGraphic);
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, click run.
You should see a point and a line graphic along Westward Beach.
Add a polygon graphic
A polygon graphic is created using a polygon and a fill symbol. A polygon is defined as a sequence of points that describe a closed boundary.
Polygons have one or more distinct parts. Each part is a sequence of points describing a closed boundary. For a single area with no holes, you can use the Polygon
constructor to create a polygon with just one part. To create a polygon with more than one part, use a Polygon
.
-
Create a
Polygon
and aSimple
. To create theFill Symbol Polygon
, create a newPoint
with aCollection Spatial
and add newReference Point
s to it.Polygon graphics support a number of symbol types such as
Simple
,Fill Symbol Picture
,Fill Symbol Simple
, andMarker Symbol Text
. Learn more about symbols in the API documentation.Symbol App.javaUse dark colors for code blocks 107 108 109Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // add the polyline graphic to the graphics overlay graphicsOverlay.getGraphics().add(polylineGraphic); // create a point collection with a spatial reference, and add five points to it PointCollection polygonPoints = new PointCollection(SpatialReferences.getWgs84()); polygonPoints.add(new Point(-118.818984489994, 34.0137559967283)); polygonPoints.add(new Point(-118.806796597377, 34.0215816298725)); polygonPoints.add(new Point(-118.791432890735, 34.0163883241613)); polygonPoints.add(new Point(-118.795966865355, 34.0085648646355)); polygonPoints.add(new Point(-118.808558110679, 34.0035027131376)); // create a polygon geometry from the point collection Polygon polygon = new Polygon(polygonPoints); // create an orange fill symbol with 20% transparency and the blue simple line symbol SimpleFillSymbol polygonFillSymbol = new SimpleFillSymbol(SimpleFillSymbol.Style.SOLID, 0x80FF5733, blueOutlineSymbol);
-
Create a
Graphic
with thepolygon
andpolygon
. Display theSymbol Graphic
by adding it to thegraphics
's graphics collection withOverlay get
.Graphics() App.javaUse dark colors for code blocks 120 121 122 123Add line. Add line. Add line. Add line. // create an orange fill symbol with 20% transparency and the blue simple line symbol SimpleFillSymbol polygonFillSymbol = new SimpleFillSymbol(SimpleFillSymbol.Style.SOLID, 0x80FF5733, blueOutlineSymbol); // create a polygon graphic from the polygon geometry and symbol Graphic polygonGraphic = new Graphic(polygon, polygonFillSymbol); // add the polygon graphic to the graphics overlay graphicsOverlay.getGraphics().add(polygonGraphic);
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, click run.
You should see a point, line, and polygon graphic around Mahou Riviera in the Santa Monica Mountains.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: