Learn how to display and create charts using charts components and charts model.
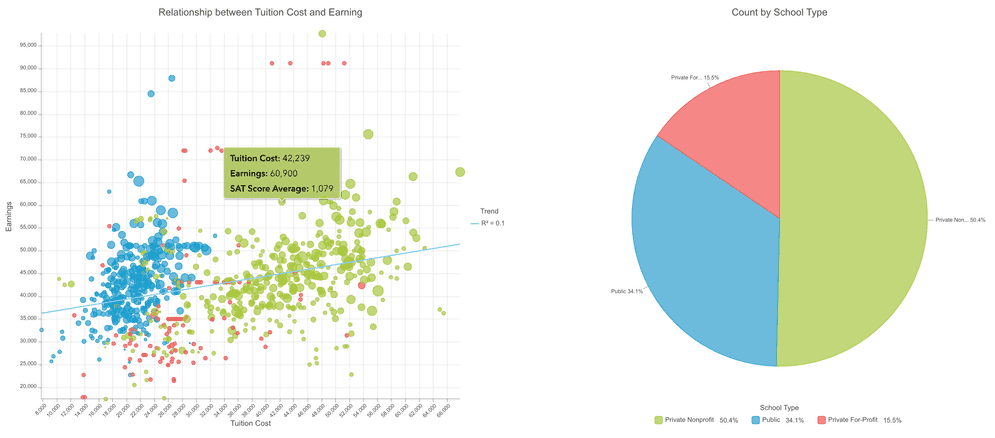
In this tutorial, you will:
- Load and display a chart (Scatterplot) using a webmap and feature layer.
- Create a new chart (Pie Chart) using Charts Model and display it in your app.
Steps
Create a new pen
- Go to CodePen to create a new pen for your application.
Add basic HTML
Define a basic HTML page.
- In CodePen > HTML, add HTML to create a basic page.
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no" />
<title>ArcGIS Maps SDK for JavaScript Tutorials: Display and create charts with charts-components & charts-model</title>
<style>
</style>
</head>
<body>
</body>
</html>
Display a chart with Charts Components
Add references
- In the
<head
tag, add references to the ArcGIS core library and CSS, and> @arcgis/charts-components
packages.
<!-- Load Calcite components from CDN -->
<link rel="stylesheet" type="text/css" href="https://js.arcgis.com/calcite-components/2.13.2/calcite.css" />
<script type="module" src="https://js.arcgis.com/calcite-components/2.13.2/calcite.esm.js"></script>
<!-- Load the ArcGIS Maps SDK for JavaScript from CDN -->
<link rel="stylesheet" href="https://js.arcgis.com/4.31/esri/themes/dark/main.css" />
<script src="https://js.arcgis.com/4.31"></script>
<!-- Load Charts components from CDN-->
<script type="module" src="https://js.arcgis.com/charts-components/4.31/arcgis-charts-components.esm.js"></script>
Add a chart component
- In the
<body
tag, create a> <div
element with a class set to> chart-container
. Add thearcgis-charts-scatter-plot
component inside the<div
with an id set to> scatterplot
.
<div class="chart-container">
<arcgis-charts-scatter-plot id="scatterplot"></arcgis-charts-scatter-plot>
</div>
Add styling
- In the
<style
tag, add CSS to style the chart container and the scatterplot.>
.chart-container {
display: flex;
height: 80vh;
}
#scatterplot {
flex: 1;
}
Add script logic for displaying a chart
- Add a
<script type="module"
section in the> <body
.> - Define a function named
load
for loading a feature layer from a webmap by passing in theFeature Layer() webmap
andId layer
.Title - Load the feature layer item by calling the asynchronous function
load
.Feature Layer(webmap Id, layer Title) - Use
document.query
to refer theSelector() arcgis-charts-scatter-plot
component. - Get the first chart's configuration from the feature layer.
- Assign the scatterplot configuration and the feature layer to the scatterplot element.
<!-- Step 1 -->
<script type="module">
// Step 2
async function loadFeatureLayer(webmapId, layerTitle) {
const WebMap = await $arcgis.import("esri/WebMap");
const webmap = new WebMap({
portalItem: {
id: webmapId
}
});
await webmap.loadAll();
const featureLayer = webmap.layers.find((layer) => layer.title === layerTitle);
return featureLayer;
}
// Step 3
const featureLayer = await loadFeatureLayer("96cb2d2825dc459abadcabc941958125", "College Scorecard");
// Step 4
const scatterplotElement = document.querySelector("#scatterplot");
// Step 5
const scatterplotConfig = featureLayer.charts[0];
// Step 6
scatterplotElement.config = scatterplotConfig;
scatterplotElement.layer = featureLayer;
</script>
Create a chart with Charts Model
Add references
- Within the head, add reference to the @arcgis/charts-model package.
<!-- Load Charts model from CDN-->
<script type="module" src="https://js.arcgis.com/charts-model/4.31/index.js"></script>
Add a chart component
- Add the
arcgis-charts-pie-chart
component inside the<div class="chart-container"
with an id set to> pie-chart
.
<div class="chart-container">
...
<arcgis-charts-pie-chart id="pie-chart"></arcgis-charts-pie-chart>
</div>
Add styling
- In the
<style
tag, add CSS to style the pie chart.>
#pie-chart,
#scatterplot {
flex: 1;
}
Add script logic for creating a chart
- Import
Pie
from the charts-model CDN. TheChart Model $arcgis
global is a new promise-based way of importing modules in ArcGIS Maps SDK for JavaScript AMD projects without the need forrequire
. - Use
document.query
to refer theSelector() arcgis-charts-pie-chart
component. - Create a new instance of pie chart model.
- Pass the feature layer to the pie chart model with
setup()
. - Modify properties of the pie chart model. Refer to the full list of available methods in the charts model references.
- Get the chart's configuration from the pie chart model with
get
.Config() - Assign the pie chart config and the feature layer to the pie chart element.
<script type="module">
// Step 1
const { PieChartModel } = await $arcgis.import("@arcgis/charts-model");
// Step 2
const pieChartElement = document.querySelector("#pie-chart");
// Step 3
const pieChartModel = new PieChartModel();
// Step 4
await pieChartModel.setup({ layer: featureLayer });
// Step 5
await pieChartModel.setCategory("Type");
pieChartModel.setDataLabelsVisibility(true);
pieChartModel.setTitleText("Count by School Type");
pieChartModel.setLegendTitleText("School Type");
pieChartModel.setLegendPosition("bottom");
// Step 6
const pieChartConfig = pieChartModel.getConfig();
// Step 7
pieChartElement.config = pieChartConfig;
pieChartElement.layer = featureLayer;
</script>
Run the app
In CodePen, run your code to display the charts.
The app should display a scatterplot showing the relationship between education cost and income earnings with a line displaying the linear trend. It should also display a pie chart showing the count of schools by type.
What's next?
- Go to the references for more detailed information about components.