What is thinning?
Thinning is a method of decluttering the view by removing features that overlap one another. This is helpful when many features overlap and you want to display uncluttered data, but don't necessarily need to communicate its density.
How thinning works
There are a couple of approaches to thinning:
- Feature reduction by selection only applies to point layers in 3D scenes. Feature reduction selection is configured on the featureReduction property of point layers. When set, overlapping features are randomly removed from the view and displayed at scales and camera angles where they no longer overlap nearby features.
- Scale-driven filter is the process of controlling which features are downloaded to the client based on the view scale. This is useful for reducing the download size of large layers and improving the visualization, particularly for mobile devices.
Examples
Feature reduction by selection (3D)
The following example demonstrates how to declutter the view by randomly removing overlapping features. This is controlled using the FeatureReductionSelection option on point layers in 3D scenes. Simply set the feature
type on the layer to selection
and features will be thinned automatically.
pointsLayer.featureReduction = {
type: "selection"
};
Scale-driven filter
This example demonstrates how to control which features are downloaded to the client based on the view scale. This is useful for reducing the download size of large layers, particularly for mobile devices.
For example, the OpenStreetMap Asia highways layer contains more than 46 million high resolution line features with more than 20 attributes. Since this layer requires several gigabytes of memory, it isn't feasible to load the entire dataset to a browser.
Thinning this data can significantly improve the initial download size, and the visualization.
Thinning | No thinning | |
---|---|---|
Initial download size | 141kB (27.8kB compressed) | 12.2MB (2.1MB compressed) |
Preview |
![]() |
![]() |
First, you should set scale visibility constraints to restrict layer visibility at scales best suited given the density of the data. This is controlled by the min
and max
properties of the layer.
const highwaysLayer = new FeatureLayer({
portalItem: {
id: "af989f50f24040e4890dce13ee1b1561"
},
minScale: 1000000,
maxScale: 0
});
While this will prevent users from loading unreasonable amounts of data to the browser, it still may not be aggressive enough, especially for mobile devices. You can further reduce download size by filtering features dynamically by updating the layer's definition
as the view's scale changes.
This snippet shows how you can define definition expressions (i.e. SQL where clauses) to apply to the layer when the user zooms to certain scales. Notice how this allows you to establish a priority for display. For example, only the largest motorways are displayed at the smallest scale. As the user progressively zooms in, more highways load in the view based on their size classification. This ensures only the minimum data required by users is downloaded to the view, effectively thinning it.
const levels = [
{
minScale: Infinity,
maxScale: 150000,
definitionExpression: "highway IN ('motorway')"
},
{
minScale: 150000,
maxScale: 60000,
definitionExpression: "highway IN ('motorway', 'trunk', 'trunk_link')"
},
{
minScale: 60000,
maxScale: 45000,
definitionExpression:
"highway IN ('motorway', 'motorway_link', 'trunk', 'trunk_link', 'primary', 'primary_link')"
},
{
minScale: 45000,
maxScale: 30000,
definitionExpression:
"highway IN ('motorway', 'motorway_link', 'trunk', 'trunk_link', 'primary', 'primary_link')"
},
{
minScale: 30000,
maxScale: 10000,
definitionExpression:
"highway IN ('motorway', 'motorway_link', 'trunk', 'trunk_link', 'primary', 'primary_link', 'secondary', 'secondary_link')"
}
];
// Update the definition expression based on view scale
reactiveUtils.watch(
() => view.scale,
() => {
let definitionExpression = null;
const level = levels.find((level) => view.scale < level.minScale && view.scale >= level.maxScale);
if (level) {
definitionExpression = level.definitionExpression;
}
if (definitionExpression !== highwaysLayer.definitionExpression) {
highwaysLayer.definitionExpression = definitionExpression;
}
}
);
Related samples and resources
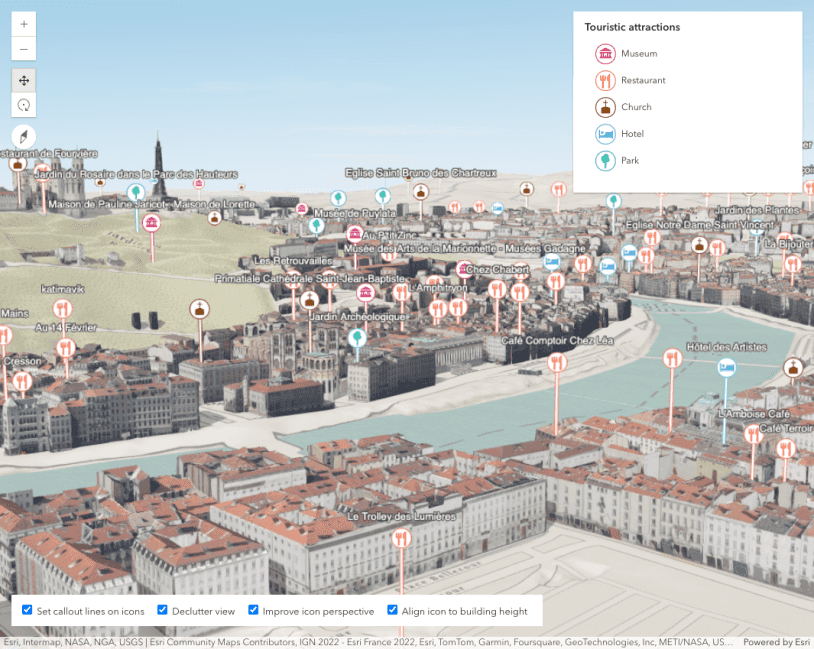
Point styles for cities
Point styles for cities
FeatureReductionSelection
Read the Core API Reference for more information.
API support
The following table describes the geometry and view types that are suited well for each visualization technique.
2D | 3D | Points | Lines | Polygons | Mesh | Client-side | Server-side | |
---|---|---|---|---|---|---|---|---|
Clustering | ||||||||
Binning | ||||||||
Heatmap | ||||||||
Opacity | ||||||||
Bloom | ||||||||
Aggregation | ||||||||
Thinning | 1 | 1 | 1 | 2 | 3 | |||
Visible scale range |
- 1. Feature reduction selection not supported
- 2. Only by feature reduction selection
- 3. Only by scale-driven filter