Learn how to use a URL to access and display a feature layer in a map.
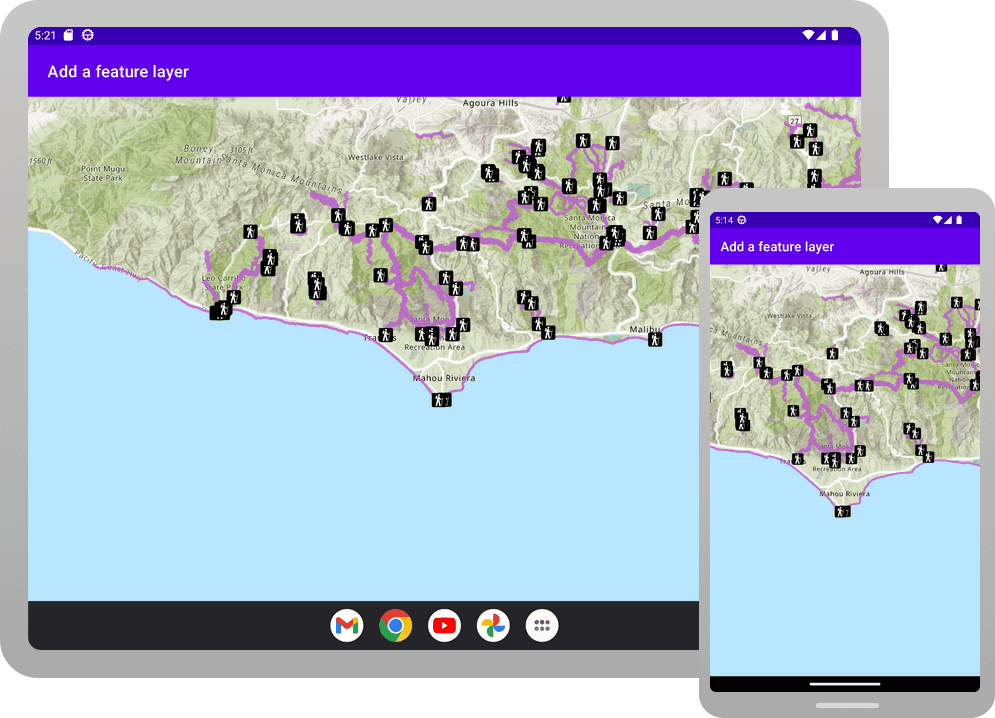
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. This tutorial shows you how to access and display a feature layer in a map. You access feature layers with an item ID or URL. You will use URLs to access the Trailheads, Trails, and Parks and Open Spaces feature layers and display them in a map.
A feature layer is a dataset in a feature service hosted in ArcGIS. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. You can use feature layers to store, access, and manage large amounts of geographic data for your applications.
In this tutorial, you use URLs to access and display three different feature layers hosted in ArcGIS Online:
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Add a feature layer._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Add a feature layer</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Add a feature layer".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } } } rootProject.name = "Add a feature layer" include(":app")
-
The UI theme composable in Display a map tutorial was
Display
. Rename the theme composable throughout the tutorial by refactoringA Map Theme Display
.A Map Theme In the Android tool window, open app > kotlin+java > com.exmple.app > ui.theme > Theme.kt.
Right-click the function name
Display
and select Refactor -> Rename. Replace the name withA Map Theme Add
.A Feature Layer Theme Theme.ktUse dark colors for code blocks Copy @Composable fun DisplayAMapTheme( darkTheme: Boolean = isSystemInDarkTheme(), // Dynamic color is available on Android 12+ dynamicColor: Boolean = true, content: @Composable () -> Unit ) { val colorScheme = when { dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> { val context = LocalContext.current if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context) } darkTheme -> DarkColorScheme else -> LightColorScheme }
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key in MainActivity.kt.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
function, find theApi Key() ApiKey.create()
call and paste your access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.MainActivity.ktUse dark colors for code blocks Copy private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") }
-
Add import statements
In MainScreen.kt, replace the import statements with the imports needed for this tutorial.
@file:OptIn(ExperimentalMaterial3Api::class)
package com.example.app.screens
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.stringResource
import com.arcgismaps.data.ServiceFeatureTable
import com.arcgismaps.mapping.ArcGISMap
import com.arcgismaps.mapping.BasemapStyle
import com.arcgismaps.mapping.Viewpoint
import com.arcgismaps.mapping.layers.FeatureLayer
import com.arcgismaps.toolkit.geoviewcompose.MapView
import com.example.app.R
Change the view point scale
In MainScreen.kt, inside the top-level function create
: Modify the Viewpoint
constructor call so it passes a scale
parameter more appropriate to tutorial.
fun createMap(): ArcGISMap {
return ArcGISMap(BasemapStyle.ArcGISTopographic).apply {
initialViewpoint = Viewpoint(
latitude = 34.0270,
longitude = -118.8050,
scale = 200000.0
)
}
}
Create service feature tables to reference feature service data
To display three new data layers (also known as operational layers) on top of the current basemap, you will create ServiceFeatureTable
s using URLs to reference datasets hosted in ArcGIS Online.
-
Open a browser and navigate to the URL for Parks and Open Spaces to view metadata about the layer. To display the layer in your app, you only need the URL.
The service page provides information such as the geometry type, the geographic extent, the minimum and maximum scale at which features are visible, and the attributes (fields) it contains. You can preview the layer by clicking on Map Viewer at the top of the page.
-
In
create
, before the code that creates theMap() ArcGIS
, do the following.Map -
Create strings that hold the URLs to the hosted layers. The URLs are: Trailheads (points), Trails (lines), and Parks and Open Spaces (polygons).
MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val parksUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" val trailsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" val trailHeadsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.0270, longitude = -118.8050, scale = 200000.0 ) } }
-
Create three
ServiceFeatureTable
objects, using a string URL to reference the datasets.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val parksUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" val trailsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" val trailHeadsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" val parksServiceFeatureTable = ServiceFeatureTable(parksUrl) val trailsServiceFeatureTable = ServiceFeatureTable(trailsUrl) val trailHeadsServiceFeatureTable = ServiceFeatureTable(trailHeadsUrl) return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.0270, longitude = -118.8050, scale = 200000.0 ) } }
-
You will use
FeatureLayer
s to display the hosted layers on top of the basemap.Create three new
FeatureLayer
s usingFeatureLayer.createWithFeatureTable()
, to which you pass a service feature table.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val parksUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" val trailsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" val trailHeadsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" val parksServiceFeatureTable = ServiceFeatureTable(parksUrl) val trailsServiceFeatureTable = ServiceFeatureTable(trailsUrl) val trailHeadsServiceFeatureTable = ServiceFeatureTable(trailHeadsUrl) val featureLayerPark = FeatureLayer.createWithFeatureTable(parksServiceFeatureTable) val featureLayerTrail = FeatureLayer.createWithFeatureTable(trailsServiceFeatureTable) val featureLayerTrailHead = FeatureLayer.createWithFeatureTable(trailHeadsServiceFeatureTable) return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.0270, longitude = -118.8050, scale = 200000.0 ) } }
-
-
In the
apply
block forArcGIS
, create a list of these threeMap FeatureLayer
s, and add them to the map'soperationalLayers
usingadd
.All() For more information on
apply {}
, see Kotlin scope functions.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val parksUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" val trailsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" val trailHeadsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" val parksServiceFeatureTable = ServiceFeatureTable(parksUrl) val trailsServiceFeatureTable = ServiceFeatureTable(trailsUrl) val trailHeadsServiceFeatureTable = ServiceFeatureTable(trailHeadsUrl) val featureLayerPark = FeatureLayer.createWithFeatureTable(parksServiceFeatureTable) val featureLayerTrail = FeatureLayer.createWithFeatureTable(trailsServiceFeatureTable) val featureLayerTrailHead = FeatureLayer.createWithFeatureTable(trailHeadsServiceFeatureTable) return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.0270, longitude = -118.8050, scale = 200000.0 ) operationalLayers.addAll( listOf( featureLayerPark, featureLayerTrail, featureLayerTrailHead ) ) } }
-
Click Run > Run > app to run the app.
You should see point features (representing trailheads) draw on the map for an area in the Santa Monica Mountains in southern California.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: