Learn how to display point, line, and polygon graphics in a map.
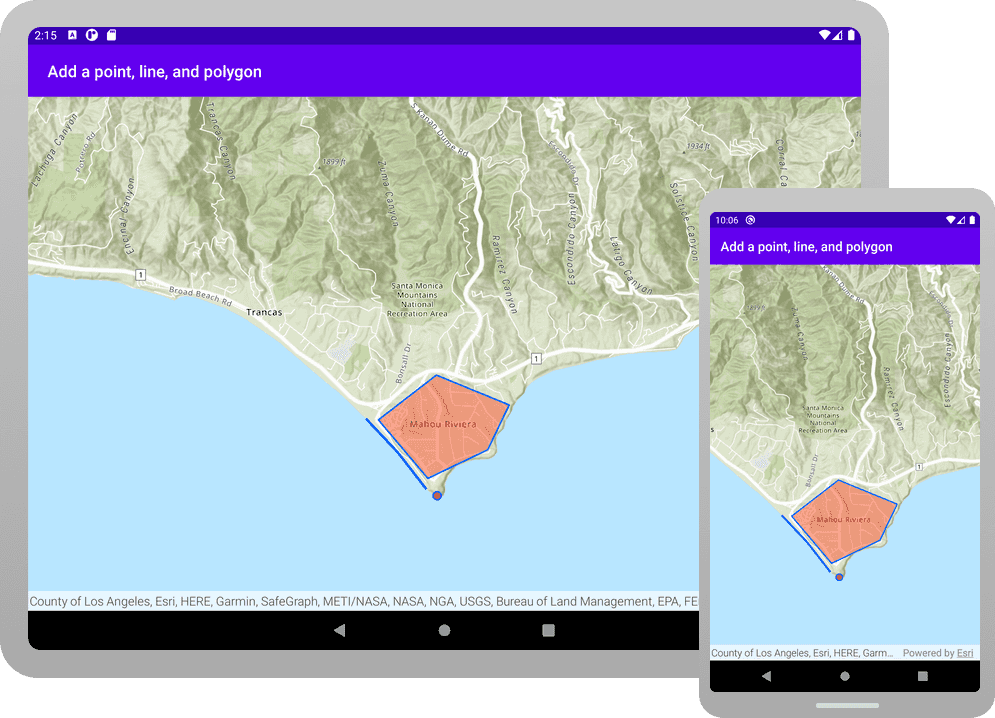
You typically use graphics to display geographic data that is not connected to a database and that is not persisted, like highlighting a route between two locations, displaying a search buffer around a point, or tracking the location of a vehicle in real-time. Graphics are composed of a geometry, symbol, and attributes.
In this tutorial, you display points, lines, and polygons on a map as graphics.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Add a point, line, and polygon._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Add a point, line, and polygon</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Add a point, line, and polygon".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } maven { url = uri("https://olympus.esri.com/artifactory/arcgisruntime-repo/") } } } rootProject.name = "Add a point, line, and polygon" include(":app")
-
The UI theme composable in Display a map tutorial was
Display
. Rename the theme composable throughout the tutorial by refactoringA Map Theme Display
.A Map Theme In the Android tool window, open app > kotlin+java > com.exmple.app > ui.theme > Theme.kt.
Right-click the function name
Display
and select Refactor -> Rename. Replace the name withA Map Theme Add
.A Point Line And Polygon Theme Theme.ktUse dark colors for code blocks Copy @Composable fun DisplayAMapTheme( darkTheme: Boolean = isSystemInDarkTheme(), // Dynamic color is available on Android 12+ dynamicColor: Boolean = true, content: @Composable () -> Unit ) { val colorScheme = when { dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> { val context = LocalContext.current if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context) } darkTheme -> DarkColorScheme else -> LightColorScheme }
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key in MainActivity.kt.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
function, find theApi Key() ApiKey.create()
call and paste your copied access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.MainActivity.ktUse dark colors for code blocks Copy private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") }
-
Add import statements
-
In the Android tool window, open app > kotlin+java > com.example.app > MainScreen.kt. Replace the import statements with the imports needed for this tutorial.
MainScreen.ktUse dark colors for code blocks @file:OptIn(ExperimentalMaterial3Api::class) package com.example.app.screens import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.foundation.layout.padding import androidx.compose.material3.ExperimentalMaterial3Api import androidx.compose.material3.Scaffold import androidx.compose.material3.Text import androidx.compose.material3.TopAppBar import androidx.compose.runtime.Composable import androidx.compose.runtime.remember import androidx.compose.ui.Modifier import androidx.compose.ui.res.stringResource import com.arcgismaps.Color import com.arcgismaps.geometry.Point import com.arcgismaps.geometry.PolygonBuilder import com.arcgismaps.geometry.PolylineBuilder import com.arcgismaps.geometry.SpatialReference import com.arcgismaps.mapping.ArcGISMap import com.arcgismaps.mapping.BasemapStyle import com.arcgismaps.mapping.Viewpoint import com.arcgismaps.mapping.symbology.SimpleFillSymbol import com.arcgismaps.mapping.symbology.SimpleFillSymbolStyle import com.arcgismaps.mapping.symbology.SimpleLineSymbol import com.arcgismaps.mapping.symbology.SimpleLineSymbolStyle import com.arcgismaps.mapping.symbology.SimpleMarkerSymbol import com.arcgismaps.mapping.symbology.SimpleMarkerSymbolStyle import com.arcgismaps.mapping.view.Graphic import com.arcgismaps.mapping.view.GraphicsOverlay import com.arcgismaps.toolkit.geoviewcompose.MapView import com.example.app.R // Create a blue outline symbol.
Add a graphics overlay and a list of graphics overlay
You will create a GraphicsOverlay
. Then add the graphics overlay to a list named graphics
.
For information about remembering state between recompositions in Android Jetpack Compose, see State and Jetpack Compose.
In subsequent sections of this tutorial, you will create a point, a line, and a polygon and add each of them to the GraphicsOverlay
.
-
In the
Main
composable, add aScreen remember
block and create aGraphicsOverlay
inside it. Then assignremember
to a local variable namedgraphics
.Overlay MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { // create an ArcGISMap val map = remember { createMap() } // Create a graphics overlay. val graphicsOverlay = remember { GraphicsOverlay() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { MapView( modifier = Modifier.fillMaxSize().padding(it), arcGISMap = map, ) }
-
Add a
remember
block. Inside it, create a list that contains thegraphics
you just created. Then assignOverlay remember
to a local variable namedgraphics
.Overlays MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { // create an ArcGISMap val map = remember { createMap() } // Create a graphics overlay. val graphicsOverlay = remember { GraphicsOverlay() } // Create a list of graphics overlays used by the MapView val graphicsOverlays = remember { listOf(graphicsOverlay) }
-
Pass
graphics
as theOverlays graphics
argument toOverlays MapView
.MainScreen.ktUse dark colors for code blocks Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { MapView( modifier = Modifier.fillMaxSize().padding(it), arcGISMap = map, graphicsOverlays = graphicsOverlays ) }
Add a point graphic
A point graphic is created using a point and a marker symbol. A point is defined with x and y coordinates, and a spatial reference. For latitude and longitude coordinates, the spatial reference is WGS84.
-
Create a lazy top-level property named
blue
. In theOutline Symbol lazy
block, create a solid, blue, 2px-wideSimpleLineSymbol
.MainScreen.ktUse dark colors for code blocks // Create a blue outline symbol. private val blueOutlineSymbol by lazy { SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.fromRgba(0, 0, 255), 2f) }
-
Create a lazy top-level property named
point
.Graphic MainScreen.ktUse dark colors for code blocks private val pointGraphic by lazy { }
-
In the
lazy
block of thepoint
property , create aGraphic Point
and aSimpleMarkerSymbol
. To create the point, provide x (longitude) and y (latitude) coordinates and aSpatialReference
(by callingwgs84()
onSpatialReference
).Point graphics support a number of symbol types such as
SimpleMarkerSymbol
,PictureMarkerSymbol
, andTextSymbol
. Learn more about symbols atSymbol
in the API Reference.Assign
blue
to theOutline Symbol outline
property ofsimple
.Marker Symbol MainScreen.ktUse dark colors for code blocks private val pointGraphic by lazy { // Create a point geometry with a location and spatial reference. // Point(latitude, longitude, spatial reference) val point = Point( x = -118.8065, y = 34.0005, spatialReference = SpatialReference.wgs84() ) // Create a point symbol that is an small red circle and assign the blue outline symbol to its outline property. val simpleMarkerSymbol = SimpleMarkerSymbol( style = SimpleMarkerSymbolStyle.Circle, color = Color.red, size = 10f ) simpleMarkerSymbol.outline = blueOutlineSymbol }
-
Create a
Graphic
withpoint
andsimple
.Marker Symbol MainScreen.ktUse dark colors for code blocks private val pointGraphic by lazy { // Create a point geometry with a location and spatial reference. // Point(latitude, longitude, spatial reference) val point = Point( x = -118.8065, y = 34.0005, spatialReference = SpatialReference.wgs84() ) // Create a point symbol that is an small red circle and assign the blue outline symbol to its outline property. val simpleMarkerSymbol = SimpleMarkerSymbol( style = SimpleMarkerSymbolStyle.Circle, color = Color.red, size = 10f ) simpleMarkerSymbol.outline = blueOutlineSymbol // Create a graphic with the point geometry and symbol. Graphic( geometry = point, symbol = simpleMarkerSymbol ) }
-
In
Main
, display the graphic by adding it to theScreen graphics
property ofgraphics
.Overlay MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { // create an ArcGISMap val map = remember { createMap() } // Create a graphics overlay. val graphicsOverlay = remember { GraphicsOverlay() } // Add the point graphic to the graphics overlay. graphicsOverlay.graphics.add(pointGraphic) // Create a list of graphics overlays used by the MapView val graphicsOverlays = remember { listOf(graphicsOverlay) }
Click Run > Run > app to run the app.
In Android Studio, you have two choices for running your app: an actual Android device or the Android Emulator.
Android device
Connect your computer to your Android device, using USB or Wi-Fi. For more details, see How to connect your Android device.
Android Emulator
Create an AVD (Android Virtual Device) to run in the Android Emulator. For details, see Run apps on the Android Emulator.
Selecting a device
When you build and run an app in Android Studio, you must first select a device. From the Android Studio toolbar, you can access the drop-down list of your currently available devices, both virtual and physical.
.
If you cannot access the list on the toolbar, click Tools > Device Manager.
You should see a point graphic in Point Dume State Beach.
Add a line graphic
A line graphic is created using a polyline and a line symbol. A polyline is defined as a sequence of points.
Polylines have one or more distinct parts. Each part is a sequence of points. For a continuous line, you can use the Polyline
constructor to create a polyline with just one part. To create a polyline with more than one part, use a PolylineBuilder
.
-
Create a lazy top-level property named
polyline
.Graphic MainScreen.ktUse dark colors for code blocks private val polylineGraphic by lazy { }
-
In the
lazy
block, create aPolyline
and aSimpleLineSymbol
. To create the polyline, create aPolylineBuilder
and then callto
on the polygon builder.Geometry() Line graphics support a number of symbol types such as
SimpleMarkerSymbol
andTextSymbol
. Learn more about symbols atSymbol
in the API Reference.MainScreen.ktUse dark colors for code blocks private val polylineGraphic by lazy { // Create a blue line symbol for the polyline. val polylineSymbol = SimpleLineSymbol( style = SimpleLineSymbolStyle.Solid, color = Color.fromRgba(0, 0, 255), width = 3f ) // Create a polylineBuilder with a spatial reference and add three points to it. val polylineBuilder = PolylineBuilder(SpatialReference.wgs84()) { addPoint(-118.8215, 34.0139) addPoint(-118.8148, 34.0080) addPoint(-118.8088, 34.0016) } // then get the polyline from the polyline builder val polyline = polylineBuilder.toGeometry() }
-
Create a
Graphic
withpolyline
andpolyline
.Symbol MainScreen.ktUse dark colors for code blocks private val polylineGraphic by lazy { // Create a blue line symbol for the polyline. val polylineSymbol = SimpleLineSymbol( style = SimpleLineSymbolStyle.Solid, color = Color.fromRgba(0, 0, 255), width = 3f ) // Create a polylineBuilder with a spatial reference and add three points to it. val polylineBuilder = PolylineBuilder(SpatialReference.wgs84()) { addPoint(-118.8215, 34.0139) addPoint(-118.8148, 34.0080) addPoint(-118.8088, 34.0016) } // then get the polyline from the polyline builder val polyline = polylineBuilder.toGeometry() // Create a polyline graphic with the polyline geometry and symbol. Graphic( geometry = polyline, symbol = polylineSymbol ) }
-
In
Main
, display the graphic by adding it to theScreen graphics
property ofgraphics
.Overlay MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { // create an ArcGISMap val map = remember { createMap() } // Create a graphics overlay. val graphicsOverlay = remember { GraphicsOverlay() } // Add the point graphic to the graphics overlay. graphicsOverlay.graphics.add(pointGraphic) // Add the polyline graphic to the graphics overlay. graphicsOverlay.graphics.add(polylineGraphic) // Create a list of graphics overlays used by the MapView val graphicsOverlays = remember { listOf(graphicsOverlay) }
Click Run > Run > app to run the app.
In Android Studio, you have two choices for running your app: an actual Android device or the Android Emulator.
Android device
Connect your computer to your Android device, using USB or Wi-Fi. For more details, see How to connect your Android device.
Android Emulator
Create an AVD (Android Virtual Device) to run in the Android Emulator. For details, see Run apps on the Android Emulator.
Selecting a device
When you build and run an app in Android Studio, you must first select a device. From the Android Studio toolbar, you can access the drop-down list of your currently available devices, both virtual and physical.
.
If you cannot access the list on the toolbar, click Tools > Device Manager.
You should see a point and line graphic along Westward Beach.
Add a polygon graphic
A polygon graphic is created using a polygon and a fill symbol. A polygon is defined as a sequence of points that describe a closed boundary.
Polygons have one or more distinct parts. Each part is a sequence of points describing a closed boundary. For a single area with no holes, you can use the Polygon
constructor to create a polygon with just one part. To create a polygon with more than one part, use a PolygonBuilder
.
-
Create a lazy top-level property named
polygon
.Graphic MainScreen.ktUse dark colors for code blocks private val polygonGraphic by lazy { }
-
In the
lazy
block, create aPolygon
and aSimpleFillSymbol
. To create the polygon, create aPolygonBuilder
and then callto
on the polygon builder.Geometry() Polygon graphics support a number of symbol types such as
SimpleFillSymbol
,PictureFillSymbol
,SimpleMarkerSymbol
andTextSymbol
. Learn more about symbols atSymbol
in the API Reference.Next, create a
SimpleFillSymbol
that has a solid red fill with an alpha channel of 128 and uses theblue
defined earlier.Outline Symbol MainScreen.ktUse dark colors for code blocks private val polygonGraphic by lazy { // Create a polygon builder with a spatial reference and add five vertices (points) to it. // Then get the polygon from the polygon builder. val polygonBuilder = PolygonBuilder(SpatialReference.wgs84()) { addPoint(-118.8189, 34.0137) addPoint(-118.8067, 34.0215) addPoint(-118.7914, 34.0163) addPoint(-118.7959, 34.0085) addPoint(-118.8085, 34.0035) } val polygon = polygonBuilder.toGeometry() // Create a red fill symbol with an alpha component of 128: values can run from 0 to 255). val polygonFillSymbol = SimpleFillSymbol( style = SimpleFillSymbolStyle.Solid, color = Color.fromRgba(255, 0, 0, 128), outline = blueOutlineSymbol ) }
-
Create a
Graphic
withpolygon
andpolygon
.Fill Symbol MainScreen.ktUse dark colors for code blocks private val polygonGraphic by lazy { // Create a polygon builder with a spatial reference and add five vertices (points) to it. // Then get the polygon from the polygon builder. val polygonBuilder = PolygonBuilder(SpatialReference.wgs84()) { addPoint(-118.8189, 34.0137) addPoint(-118.8067, 34.0215) addPoint(-118.7914, 34.0163) addPoint(-118.7959, 34.0085) addPoint(-118.8085, 34.0035) } val polygon = polygonBuilder.toGeometry() // Create a red fill symbol with an alpha component of 128: values can run from 0 to 255). val polygonFillSymbol = SimpleFillSymbol( style = SimpleFillSymbolStyle.Solid, color = Color.fromRgba(255, 0, 0, 128), outline = blueOutlineSymbol ) // Create a polygon graphic from the polygon geometry and symbol. Graphic( geometry = polygon, symbol = polygonFillSymbol ) }
-
In
Main
, display the graphic by adding it to theScreen graphics
property ofgraphics
.Overlay MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { // create an ArcGISMap val map = remember { createMap() } // Create a graphics overlay. val graphicsOverlay = remember { GraphicsOverlay() } // Add the point graphic to the graphics overlay. graphicsOverlay.graphics.add(pointGraphic) // Add the polyline graphic to the graphics overlay. graphicsOverlay.graphics.add(polylineGraphic) // Add the polygon graphic to the graphics overlay. graphicsOverlay.graphics.add(polygonGraphic) // Create a list of graphics overlays used by the MapView val graphicsOverlays = remember { listOf(graphicsOverlay) }
Click Run > Run > app to run the app.
In Android Studio, you have two choices for running your app: an actual Android device or the Android Emulator.
Android device
Connect your computer to your Android device, using USB or Wi-Fi. For more details, see How to connect your Android device.
Android Emulator
Create an AVD (Android Virtual Device) to run in the Android Emulator. For details, see Run apps on the Android Emulator.
Selecting a device
When you build and run an app in Android Studio, you must first select a device. From the Android Studio toolbar, you can access the drop-down list of your currently available devices, both virtual and physical.
.
If you cannot access the list on the toolbar, click Tools > Device Manager.
You should see a point, line, and polygon graphic around Mahou Riviera in the Santa Monica Mountains.
What's next
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: