Learn how to create and display a scene from a web scene stored in ArcGIS.
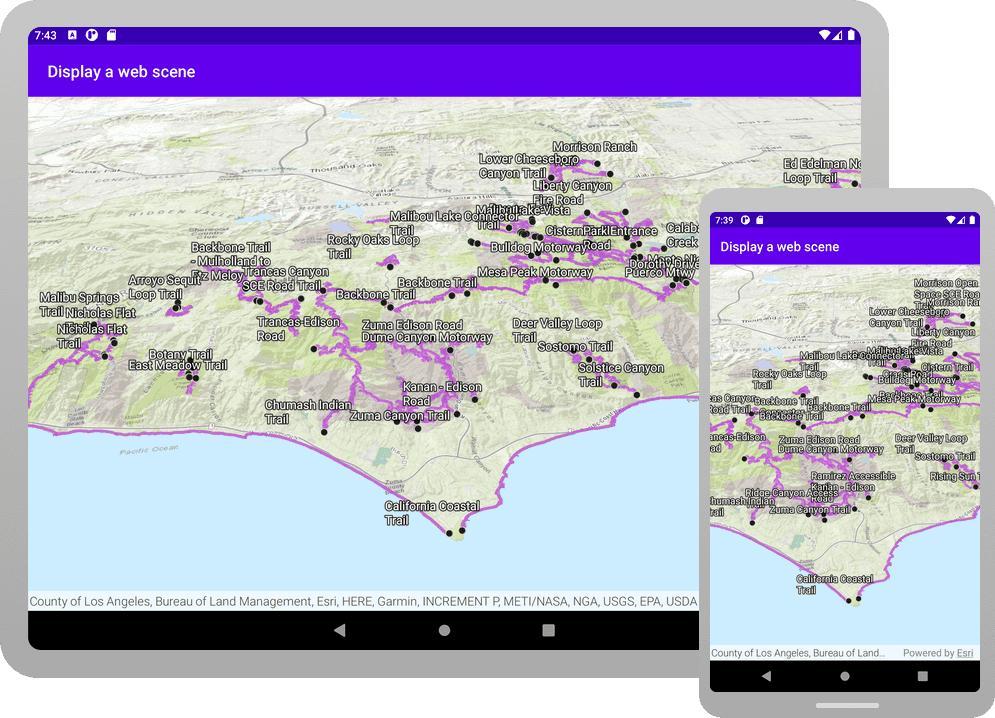
This tutorial shows you how to create and display a scene from a web scene. All web scenes are stored in ArcGIS with a unique item ID. You will access an existing web scene by item ID and display its layers. The web scene contains feature layers for the Santa Monica Mountains in California.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a scene tutorial. Or download and unzip the Display a scene solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Display a web scene._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display a web scene</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Display a web scene".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } } } rootProject.name = "Display a web scene" include(":app")
-
The UI theme composable in Display a scene tutorial was
Display
. Rename the theme composable throughout the tutorial by refactoringA Scene Theme Display
.A Web Scene Theme In the Android tool window, open app > kotlin+java > com.exmple.app > ui.theme > Theme.kt.
Right-click the function name
Display
and select Refactor -> Rename. Replace the name withA Scene Theme Display
.A Web Scene Theme Theme.ktUse dark colors for code blocks Copy @Composable fun DisplayASceneTheme( darkTheme: Boolean = isSystemInDarkTheme(), // Dynamic color is available on Android 12+ dynamicColor: Boolean = true, content: @Composable () -> Unit ) { val colorScheme = when { dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> { val context = LocalContext.current if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context) } darkTheme -> DarkColorScheme else -> LightColorScheme }
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key in MainActivity.kt.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
function, find theApi Key() ApiKey.create()
call and paste your access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.MainActivity.ktUse dark colors for code blocks Copy private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") }
-
Get the web scene item ID
You can use ArcGIS tools to create and view web scenes. Use the Scene Viewer to identify the web scene item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web scene in the Scene Viewer in ArcGIS Online. This web scene displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be 579f97b2f3b94d4a8e48a5f140a6639b.
Add import statements
In MainScreen.kt, replace the import statements with the imports needed for this tutorial.
@file:OptIn(ExperimentalMaterial3Api::class)
package com.example.app.screens
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.stringResource
import com.arcgismaps.mapping.ArcGISScene
import com.arcgismaps.mapping.PortalItem
import com.arcgismaps.portal.Portal
import com.arcgismaps.toolkit.geoviewcompose.SceneView
import com.example.app.R
Display the web scene
You can display a web scene using the web scene's item ID. Create a scene from the web scene portal item, and display it in your app.
-
Open the app > kotlin+java > com.example.app > MainActivity.kt. Delete the code inside
create
. These lines come from the Display a scene tutorial and do not apply here.Scene() MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } val cameraLocation = Point( x = -118.794, y = 33.909, z = 5330.0, spatialReference = SpatialReference.wgs84() ) val camera = Camera( locationPoint = cameraLocation, heading = 355.0, pitch = 72.0, roll = 0.0 ) return ArcGISScene(BasemapStyle.ArcGISImagery).apply { baseSurface = surface initialViewpoint = Viewpoint(cameraLocation, camera) } }
-
Inside the empty
create
, create a newScene() Portal
referencing ArcGIS Online as theurl
parameter andPortal.
for theConnection. Anonymous connection
parameter.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) }
-
Next, create a
Portal
by passing theportal
and the web scene's item ID as parameters.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) val portalItem = PortalItem( portal = portal, itemId = "579f97b2f3b94d4a8e48a5f140a6639b" ) }
-
Last, create an
ArcGISScene
usingportal
as the constructor parameter.Item MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) val portalItem = PortalItem( portal = portal, itemId = "579f97b2f3b94d4a8e48a5f140a6639b" ) return ArcGISScene(portalItem) }
-
Click Run > Run > app to run the app.
You should see a scene of trails, trailheads and parks in the Santa Monica Mountains. Drag, swipe, or pinch on the scene view to explore the scene.
What's Next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: