Learn how to localize place labels using the basemap styles service.
The basemap styles service provides a number of different styles that you can use in your MapLibre GL JS applications. Each style accepts a language
parameter, which allows you to localize place labels. There are currently over 30 different languages available.
In this tutorial, you use a <select
dropdown menu to toggle between a number of different place label languages.
Prerequisites
An ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, you can complete the Display a map tutorial or use the .
Get an access token
Create a new API key credential with the correct privileges to access the resources used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Privileges
- Copy the API key access token to your clipboard when prompted.
Add a language selector
Add a menu that allows users to change display language of your map. To accomplish this, use a standard html <select
element.
-
Create a
<div
tag to contain the> <select
dropdown menu. Add an> <option
for each language code available through the basemap styles service.> Use dark colors for code blocks </style> <script src=https://unpkg.com/maplibre-gl@4/dist/maplibre-gl.js></script> <link href=https://unpkg.com/maplibre-gl@4/dist/maplibre-gl.css rel="stylesheet" /> </head> <body> <div id="map"></div> <div id="languages-wrapper"> <select id="languages"> <option value="global">Global</option> <option value="ar">Arabic</option> <option value="bs">Bosnian</option> <option value="bg">Bulgarian</option> <option value="ca">Catalan</option> <option value="zh-CN">Chinese (Simplified)</option> <option value="zh-HK">Chinese (Hong Kong)</option> <option value="zh-TW">Chinese (Taiwan)</option> <option value="hr">Croatian</option> <option value="cs">Czech</option> <option value="da">Danish</option> <option value="nl">Dutch</option> <option value="en">English</option> <option value="et">Estonian</option> <option value="fi">Finnish</option> <option value="fr">French</option> <option value="de">German</option> <option value="el">Greek</option> <option value="he">Hebrew</option> <option value="hu">Hungarian</option> <option value="id">Indonesian</option> <option value="it">Italian</option> <option value="ja">Japanese</option> <option value="ko">Korean</option> <option value="lv">Latvian</option> <option value="lt">Lithuanian</option> <option value="nb">Norwegian</option> <option value="pl">Polish</option> <option value="pt-BR">Portugese (Brazil)</option> <option value="pt-PT">Portugese (Portugal)</option> <option value="ro">Romanian</option> <option value="ru">Russian</option> <option value="sr">Serbian</option> <option value="sk">Slovak</option> <option value="sl">Slovenian</option> <option value="es">Spanish</option> <option value="sv">Swedish</option> <option value="th">Thai</option> <option value="tr">Turkish</option> <option value="uk" selected>Ukrainian</option> <option value="vi">Vietnamese</option> </select> </div>
-
In the
<style
element, add CSS styling to the> languages-wrapper
andlanguages
.Use dark colors for code blocks <style> html, body, #map { padding: 0; margin: 0; height: 100%; width: 100%; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } #languages-wrapper { position: absolute; top: 20px; right: 20px; background: rgba(255, 255, 255, 0); } #languages { font-size: 16px; padding: 4px 8px; } </style> <script src=https://unpkg.com/maplibre-gl@4/dist/maplibre-gl.js></script> <link href=https://unpkg.com/maplibre-gl@4/dist/maplibre-gl.css rel="stylesheet" />
-
Run the code. The
<select
element should contain a list of different language enumerations.>
Set the basemap
Use a function to reference the basemap styles service and a language enumeration to update the map. This will be used when a selection is made.
-
Create a
base
and aUrl url
element. Theurl
function will use thebase
to create a reference to a basemap style in a specified language.Url Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/outdoor"; const map = new maplibregl.Map({ container: "map", // the id of the div element style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapEnum}?token=${accessToken}`, zoom: 2, // starting zoom center: [48.8790, 35.7088] // starting location [longitude, latitude] }) const baseUrl = "https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles"; const url = (language) => `${baseUrl}/arcgis/outdoor?token=${accessToken}&language=${language}`;
-
Set the style of the
map
using theurl
function and a language code.Use dark colors for code blocks const baseUrl = "https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles"; const url = (language) => `${baseUrl}/arcgis/outdoor?token=${accessToken}&language=${language}`; const setLanguage = (language) => { // Instantiate the given basemap layer. map.setStyle(url(language)); };
Add an event listener
Use an event listener to register a basemap change in the <select
element and to update the map.
-
Set the default language to Ukrainian (
uk
).Use dark colors for code blocks const setLanguage = (language) => { // Instantiate the given basemap layer. map.setStyle(url(language)); }; setLanguage("uk");
-
Access the language selector using its id.
Use dark colors for code blocks setLanguage("uk"); const languageSelectElement = document.querySelector("#languages");
-
Add an event listener to update the map when a new language is selected.
Use dark colors for code blocks const languageSelectElement = document.querySelector("#languages"); languageSelectElement.addEventListener("change", (e) => { setLanguage(e.target.value); console.log(e.target.value); });
Add RTL language support
MapLibre GL JS does not automatically display right-to-left languages such as Arabic and Hebrew correctly. Use the Mapbox GL RTL Text plugin to configure settings for RTL languages.
-
Call the
maplibregl.set
with the URL of the Mapbox GL RTL Text plugin to configure support for RTL languages.RTL Text Plugin Use dark colors for code blocks languageSelectElement.addEventListener("change", (e) => { setLanguage(e.target.value); console.log(e.target.value); }); maplibregl.setRTLTextPlugin('https://unpkg.com/@mapbox/mapbox-gl-rtl-text@0.2.3/mapbox-gl-rtl-text.js');
Run the app
Run the app.
You should be able to use the select element to switch between basemap layers.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:

Change the basemap style
Switch a basemap style in a map using the basemap styles service.
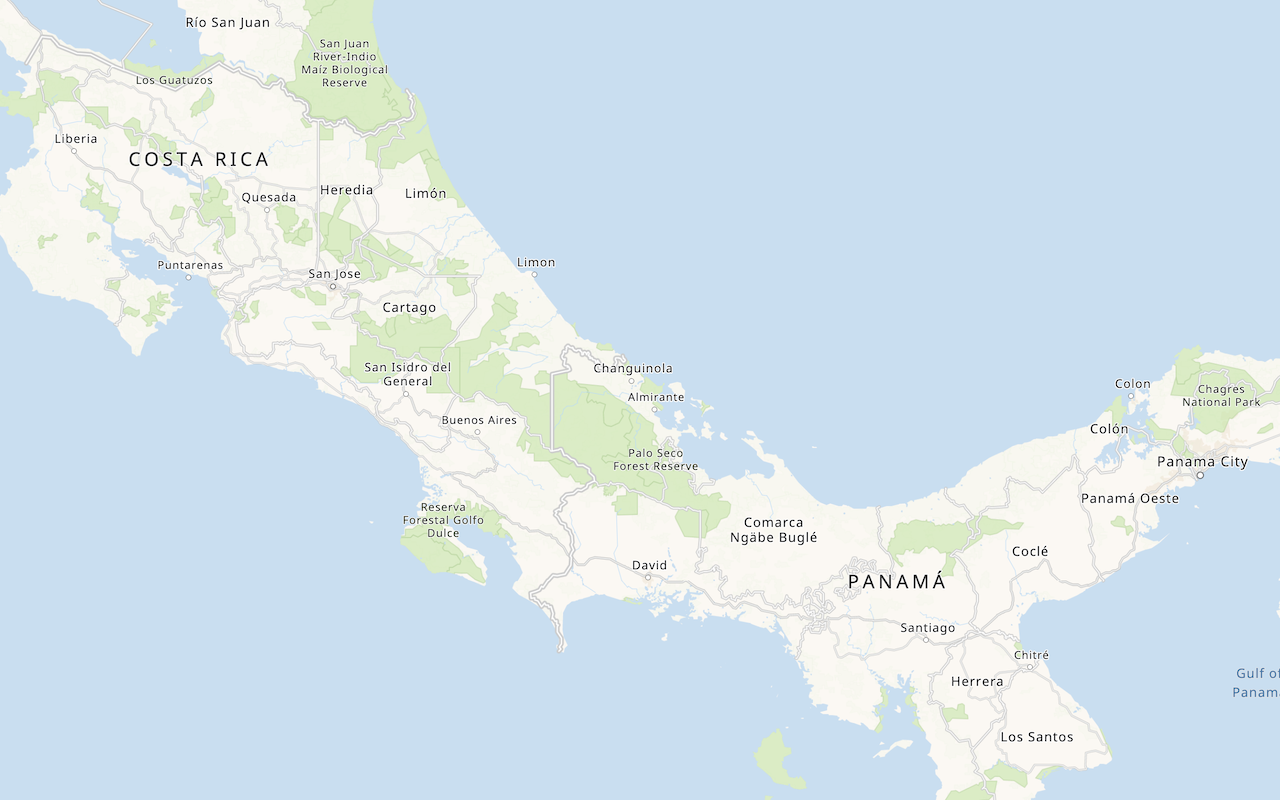
Display a custom basemap style
Add a styled vector basemap layer to a map.
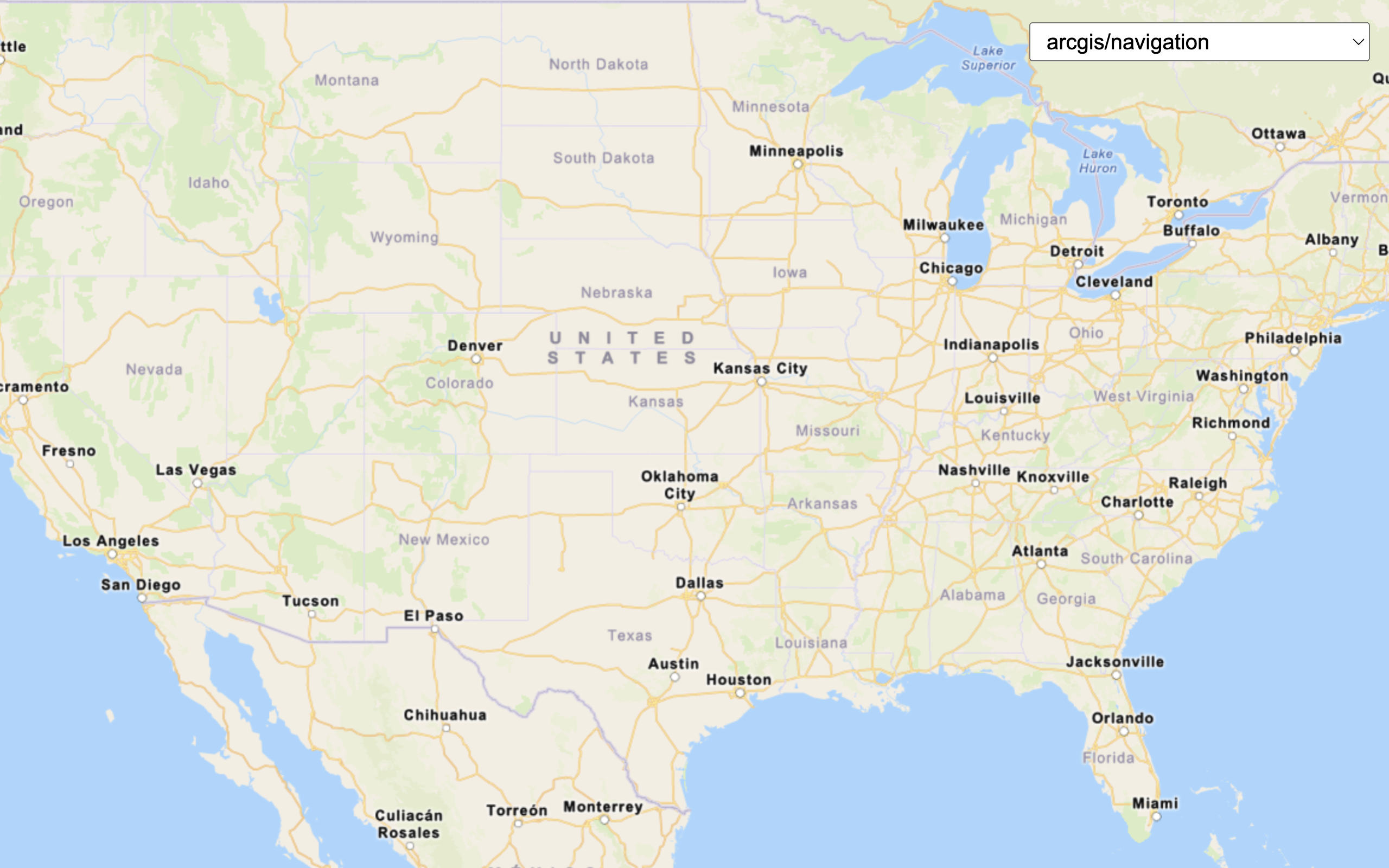
Change the static basemap tiles style
Change the basemap style in a map using the static basemap tiles service (beta).
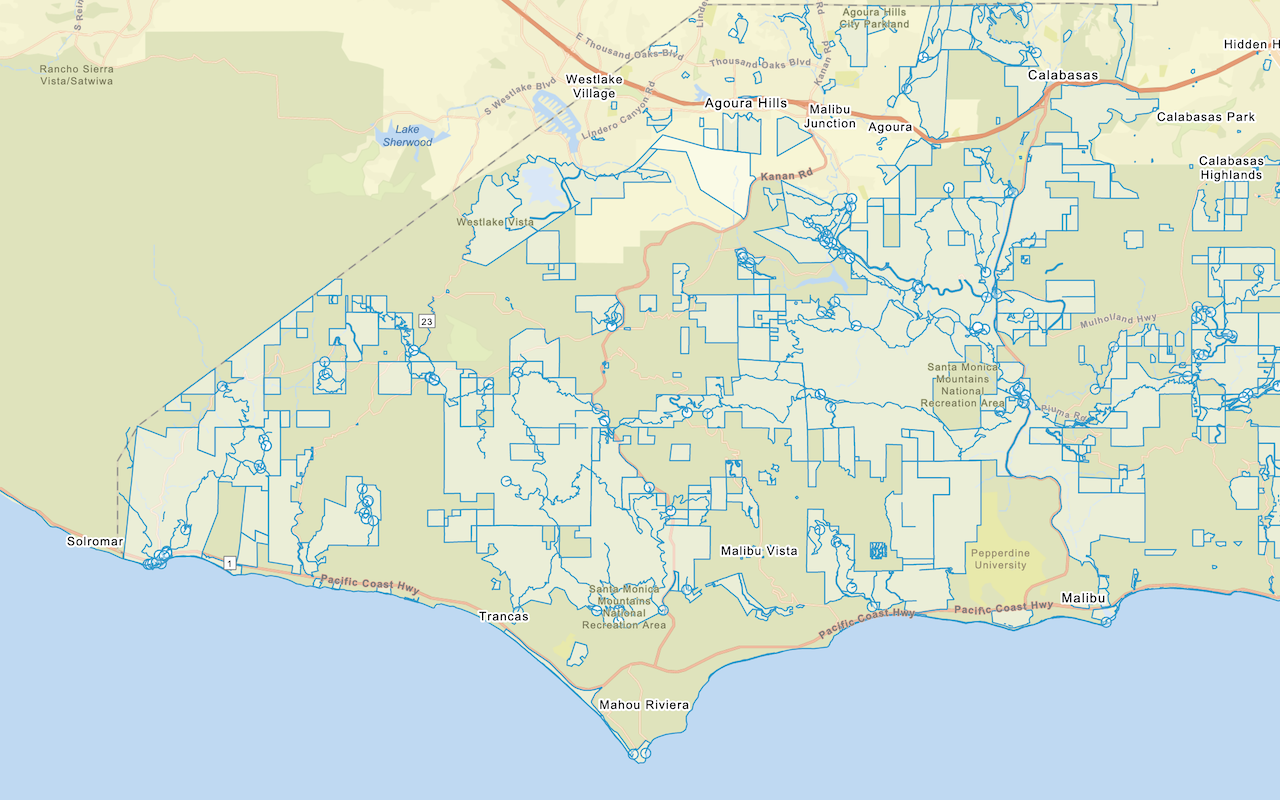
Add a feature layer
Add features from feature layers to a map.