Learn how to perform a hot spot feature analysis with the spatial analysis service.
A feature analysis is the process of using the spatial analysis service to perform server-side geometric and analytic operations on feature data. All feature analysis requests are job requests. The easiest way to programmatically run an analysis request to the spatial analysis service is to use ArcGIS REST JS, which provides a Job
class that handles long- running operations.
In this tutorial, you use ArcGIS REST JS to perform a hot spot analysis to find statistically significant clusters of parking violations.
Prerequisites
You need the following to access the spatial analysis service and perform feature analysis operations:
- An ArcGIS Online account.
- A registered application to obtain its Client ID.
- The redirect URL of the registered application to use for authentication in the format
"https
as in::// <server >[ :port]" https
.://localhost :8080
Steps
Download the starter code
Download the tutorial starter code zip file from the portal.
The zip file contains the following:
- feature-analysis.html
- authenticate.html
The feature-analysis.html file contains basic HTML scaffolding and the OAuth 2.0 code necessary to perform the analysis. The authenticate.html file is the callback page used as part of the authentication process.
Configure authentication
To access the spatial analysis service, you need an access token with privileges to perform spatial analysis. Use the ArcGIS
class from ArcGIS REST JS to configure user authentication.
Go to the item page of your OAuth credentials and copy the Client ID.
-
In the authenticate.html file, replace
YOUR
with your Client ID._CLIENT _ID Use dark colors for code blocks <script type="module"> import { ArcGISIdentityManager } from 'https://esm.run/@esri/arcgis-rest-request@4'; const clientId = "YOUR_CLIENT_ID"; const redirectUri = "authenticate.html"; ArcGISIdentityManager.completeOAuth2({ clientId, redirectUri }) </script>
-
In the feature-analysis.html file, replace
YOUR
with your Client ID._CLIENT _ID Use dark colors for code blocks const clientId = 'YOUR_CLIENT_ID'; const signInLabel = "Sign in"; const acctErrorMsg = "You can only access the spatial analysis service if you have an access token with privileges to perform spatial analysis. To learn more, go to the <a href='https://developers.arcgis.com/documentation/mapping-apis-and-services/security/'>Security and authentication guide</a>.";
-
Run the application and navigate to your localhost, for example
https
.://localhost :8080 You should be able to click the Sign in button and successfully log in to an ArcGIS Online account.
Display parking violations
To perform feature analysis, you need to provide feature data as input. In this tutorial, you use the SF parking violations hosted feature layer as input data for the hot spot analysis.
-
Add the parking violation feature layer and the corresponding vector tile layer to the
points
andpoints
variables.VTL Use dark colors for code blocks const acctErrorMsg = "You can only access the spatial analysis service if you have an access token with privileges to perform spatial analysis. To learn more, go to the <a href='https://developers.arcgis.com/documentation/mapping-apis-and-services/security/'>Security and authentication guide</a>."; const points = 'https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/sf_traffic_parking_violations_sa_osapi/FeatureServer/0'; const pointsVTL = 'https://vectortileservices3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/sf_traffic_parking_violations_sa_osapi/VectorTileServer'; // This layer contains the results of a hot spot analysis executes on the points layer. You can use it to verify that your program is working. const sampleResults = 'https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/sf_osapi_driveways_sidewalk_hot_spots/FeatureServer/0'; // You may need to modify this path to reflect your actual file structure
-
Create a
map
and set thesession.token
to display a basemap layer.Use dark colors for code blocks const initMap = (withToken) => { // create map and add basemap const basemapEnum = 'arcgis/human-geography'; map = new maplibregl.Map({ container: 'map', style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/arcgis/${basemapEnum}?token=${withToken}`, center: [-122.44571685791017, 37.76053707395286], zoom: 11, }); }; const updateSessionInfo = (session) => { let sessionInfo = document.getElementById('withPopupButton'); if (session) { sessionInfo.innerHTML = `Sign out : ${session.username}`; localStorage.setItem( '__ARCGIS_REST_USER_SESSION__', session.serialize() ); initMap(session.token); document.getElementById('signInModal').open = false; document.getElementById('runAnalysisBtn').disabled = false; } else { sessionInfo.innerHTML = signInLabel; if (map) { map.off(); map.remove(); } document.getElementById('signInModal').open = true; document.getElementById('runAnalysisBtn').disabled = true; } };
-
Create a style definition for the parking violations layer.
Use dark colors for code blocks let session = null; let map = null; let vtlLayer = { id: 'vtlLayer', type: 'circle', source: 'violations', 'source-layer': 'DeriveNewLocationsOutput', paint: { 'circle-color': 'rgb(0,0,0)', 'circle-radius': 1.5, }, };
-
Add the SF parking violations vector tile layer to the map.
Use dark colors for code blocks const initMap = (withToken) => { // create map and add basemap const basemapEnum = 'arcgis/human-geography'; map = new maplibregl.Map({ container: 'map', style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/arcgis/${basemapEnum}?token=${withToken}`, center: [-122.44571685791017, 37.76053707395286], zoom: 11, }); let lastResultUrl = null; map.once('load', () => { map.addSource('violations', { type: 'vector', tiles: [`${pointsVTL}/tile/{z}/{y}/{x}.pbf`], }); map.addLayer(vtlLayer); };
-
Run the application and navigate to your localhost, for example
https
. After you sign in, you will see the vector tile layer rendered on the map.://localhost :8080
Get the analysis URL
To make a request to the spatial analysis service, you need to get the URL first. The analysis service URL is unique to your organization.
-
Call the
get
operation from ArcGIS REST JS to obtain the analysis URL.Self Use dark colors for code blocks const getAnalysisUrl = async (withAuth) => { const portalSelf = await getSelf({ authentication: withAuth, }); return portalSelf.helperServices.analysis.url; }; document .getElementById('withPopupButton') .addEventListener('click', getAuth);
Make the request
Use the Job
class and set the operation
and params
required for the selected analysis.
-
Create a function that submits a
Job
request to the spatial analysis service using your organization's analysis URL. Set theparams
required for a hot spot analysis and authenticate using thesession
token.Use dark colors for code blocks const getAnalysisUrl = async (withAuth) => { const portalSelf = await getSelf({ authentication: withAuth, }); return portalSelf.helperServices.analysis.url; }; const runAnalysis = async () => { const analysisUrl = await getAnalysisUrl(session); const operationUrl = `${analysisUrl}/FindHotSpots/submitJob`; const params = { analysisLayer: { url: points }, shapeType: 'Hexagon', outputName: { serviceProperties: { name: `MapLibreGLJS_find_hot_spots_${new Date().getTime()}`, }, }, //Outputs results as a hosted feature serivce. }; const jobReq = await Job.submitJob({ url: operationUrl, params: params, authentication: session, }); // listen to the status event to get updates every time the job status is checked. jobReq.on(JOB_STATUSES.Status, (jobInfo) => { console.log(jobInfo.status); }); // get all the results, this will start monitoring and trigger events const jobResp = await jobReq.getAllResults(); // jobResp.aggregatedLayer.value.url return jobResp; };
-
Call the
run
function to run the operation.Analysis Use dark colors for code blocks const runAnalysisBtnWasClicked = (evt) => { document.getElementById('runAnalysisBtn').hidden = true; document.getElementById('progressBar').hidden = false; document.getElementById('withPopupButton').disabled = true; runAnalysis().then( (results) => { document.getElementById('runAnalysisBtn').hidden = false; document.getElementById('progressBar').hidden = true; document.getElementById('withPopupButton').disabled = false; }, (err) => { console.log(err); document.getElementById('progressBar').hidden = true; document.getElementById('runAnalysisBtn').hidden = false; document.getElementById('withPopupButton').disabled = false; showAlert(err); } ); };
Display results
The results of a feature analysis are returned as feature data.
-
Create a style definition for the analysis result layer.
Use dark colors for code blocks let session = null; let map = null; let lastResultLayer = { id: 'results', type: 'fill', source: 'analysisResults', paint: { 'fill-color': [ 'match', ['get', 'Gi_Text'], 'Hot Spot with 99% Confidence', '#d62f27', 'Hot Spot with 95% Confidence', '#ed7551', 'Hot Spot with 90% Confidence', '#fab984', 'Not Significant', '#f7f7f2', 'Cold Spot with 90% Confidence', '#c0ccbe', 'Cold Spot with 95% Confidence', '#849eba', 'Cold Spot with 99% Confidence', '#4575b5', 'blue', ], }, };
-
Add a new layer to your map to contain analysis results.
Use dark colors for code blocks let lastResultUrl = null; map.once('load', () => { map.addSource('violations', { type: 'vector', tiles: [`${pointsVTL}/tile/{z}/{y}/{x}.pbf`], }); map.addLayer(vtlLayer); map.addSource('analysisResults', { type: 'geojson', data: { type: "FeatureCollection", features: [] }, }); map.addLayer(lastResultLayer); });
-
Access the URL of the result layer when the analysis completes.
Use dark colors for code blocks runAnalysis().then( (results) => { document.getElementById('runAnalysisBtn').hidden = false; document.getElementById('progressBar').hidden = true; document.getElementById('withPopupButton').disabled = false; lastResultUrl = results.hotSpotsResultLayer.value.url; addResults(); }, (err) => { console.log(err); document.getElementById('progressBar').hidden = true; document.getElementById('runAnalysisBtn').hidden = false; document.getElementById('withPopupButton').disabled = false; showAlert(err); } );
-
Add the results to the map from the hosted feature layer URL as GeoJSON.
Use dark colors for code blocks // This layer contains the results of a hot spot analysis executes on the points layer. You can use it to verify that your program is working. const sampleResults = 'https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/sf_osapi_driveways_sidewalk_hot_spots/FeatureServer/0'; // You may need to modify this path to reflect your actual file structure const redirectUri = `${window.location.origin}/authenticate.html`; const addResults = () => { if (map.getSource('analysisResults')) { emptyLayerSource(); } map.getSource("analysisResults").setData(`${lastResultUrl}/query?f=geojson&where=Gi_Bin<>0&outFields=Gi_Text&token=${session.token}`); document.getElementById('clearResultsBtn').disabled = false; document.getElementById('runAnalysisBtn').disabled = true; }; const clearResults = () => { emptyLayerSource(); document.getElementById('clearResultsBtn').disabled = true; document.getElementById('runAnalysisBtn').disabled = false; document.getElementById('resultsCBox').checked = false; };
Run the app
Run the application and navigate to your localhost, for example https
.
When you click the Run analysis button, you submit a request to the spatial analysis service to perform a hot spot analysis. When the job is complete, the results of the analysis will display on the map.
What's next?
To learn how to perform other types of feature analysis, go to the related tutorials in the Spatial analysis guide:

Find and extract data
Find data with attribute and spatial queries using find analysis operations.

Combine data
Overlay, join, and dissolve features using combine analysis operations.

Summarize data
Aggregate and summarize features using summarize analysis operations.
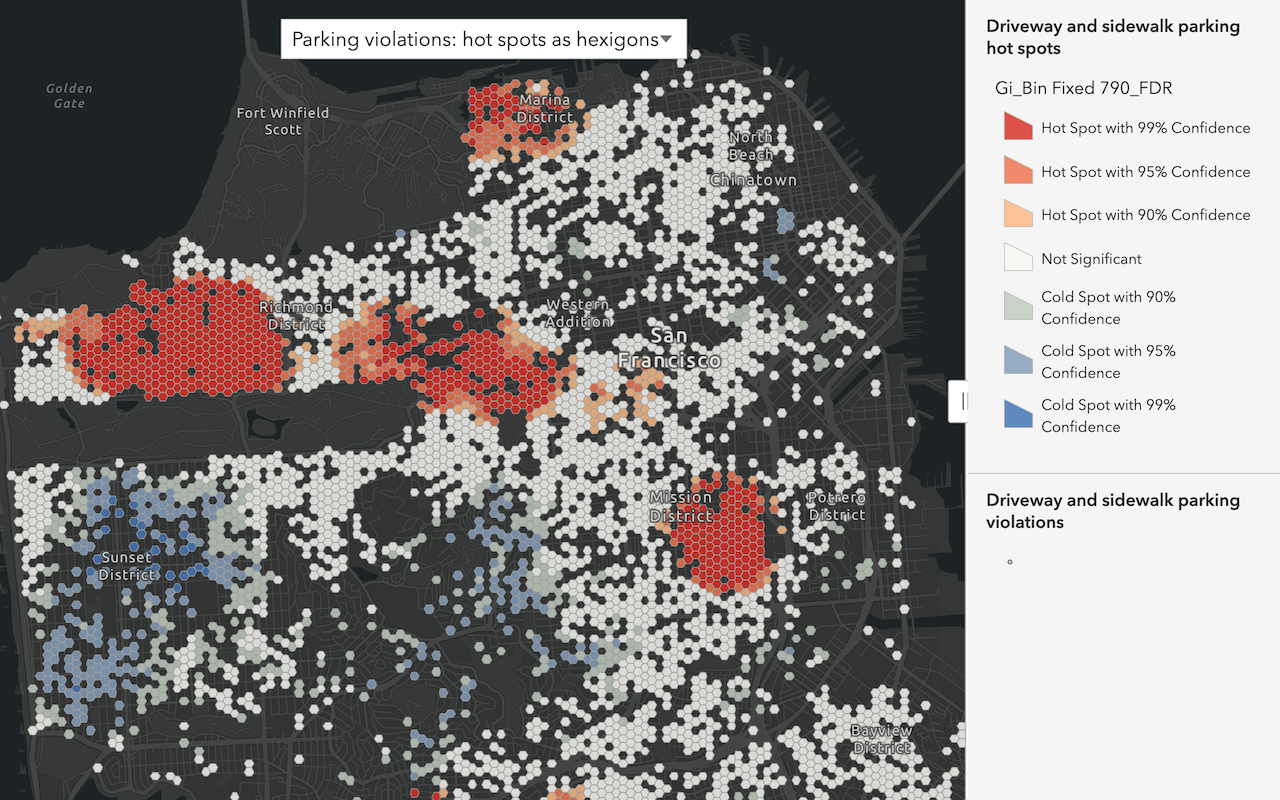
Discover patterns in data
Find patterns and trends in data using spatial analysis operations.