Learn how to display the current device location on a map or scene.
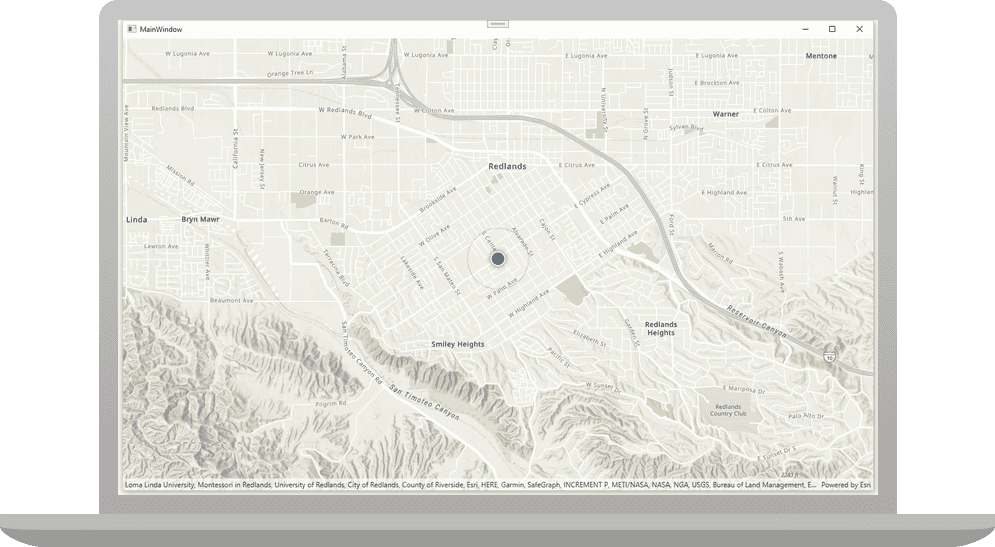
You can display the device location on a map or scene. This is important for workflows that require the user's current location, such as finding nearby businesses, navigating from the current location, or identifying and collecting geospatial information.
By default, location display uses the device's location provider. Your app can also process input from other location providers, such as an external GPS receiver or a provider that returns a simulated location. For more information, see the Show device location topic.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
On
function to set theStartup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Display
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Show the current location
Each map view has its own instance of a LocationDisplay
for showing the current location (point) of the device. The location is displayed as an overlay in the map view.
Instances of this class manage the display of device location on a map view: the symbols, animation, auto pan behavior, and so on. Location display is an overlay of the map view, and displays above everything else, including graphics overlays.
The location display does not retrieve location information, that is the job of the associated data source, which provides location updates on a regular basis. In addition to the default system location data source, you can use location providers based on external GPS devices or a simulated location source.
Each map view has its own instance of a location display and instances of location display and location data source are not shared by multiple map views. This allows you to start and stop location display independently on multiple map views without affecting each other.
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml.cs to open the file.
-
In the
Main
constructor, remove code that sets the map's initial viewpoint. The map will zoom to the extent of the current location, so this code is no longer needed.Window Use dark colors for code blocks public MainWindow() { InitializeComponent(); MapPoint mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); MainMapView.SetViewpoint(new Viewpoint(mapCenterPoint, 100000)); }
-
Add code to enable
LocationDisplay
for the map view and assign aLocationDisplayAutoPanMode
that centers the map at the device location.Use dark colors for code blocks public MainWindow() { InitializeComponent(); MainMapView.LocationDisplay.IsEnabled = true; MainMapView.LocationDisplay.AutoPanMode = Esri.ArcGISRuntime.UI.LocationDisplayAutoPanMode.Recenter; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see your current location displayed on the map. Different location symbols are used depending on the auto pan mode and whether a location is acquired. See LocationDisplayAutoPanMode
for details.
By default, a round blue symbol is used to display the device's location. The location data source tries to get the most accurate location available but depending upon signal strength, satellite positions, and other factors, the location reported could be an approximation. A semi-transparent circle around the location symbol indicates the range of accuracy. As the device moves and location updates are received, the location symbol will be repositioned on the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: