Learn how to create and display a scene from a web scene stored in ArcGIS.
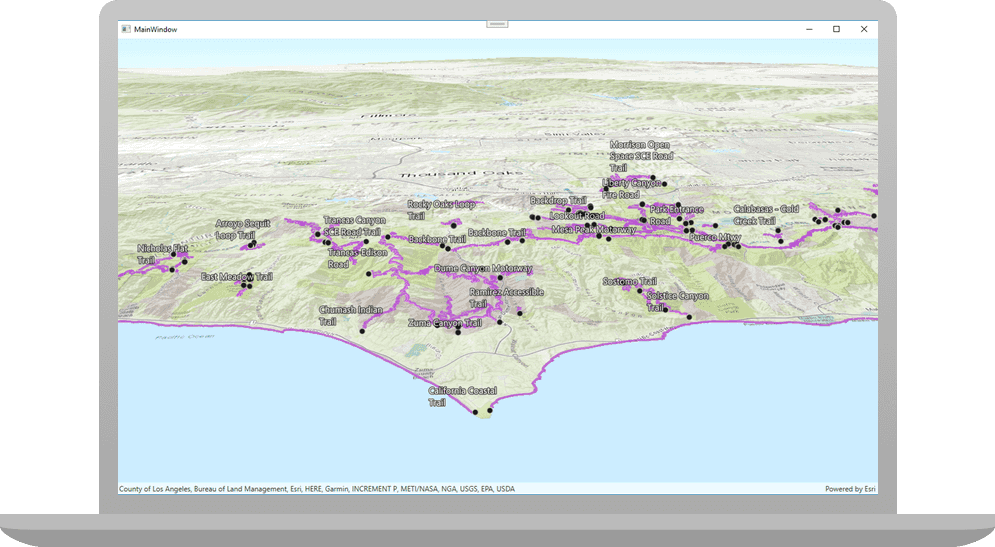
This tutorial shows you how to create and display a scene from a web scene. All web scenes are stored in ArcGIS with a unique item ID. You will access an existing web scene by item ID and display its layers. The web scene contains feature layers for the Santa Monica Mountains in California.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open the Visual Studio solution
-
To start the tutorial, complete the Display a scene tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
On
function to set theStartup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Display
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click SceneViewModel.cs in the Solution Explorer to open the file.
- In the
Scene
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Scene - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAScene window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Get the web scene item ID
You can use ArcGIS tools to create and view web scenes. Use the Scene Viewer to identify the web scene item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web scene in the Scene Viewer in ArcGIS Online. This web scene displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be 579f97b2f3b94d4a8e48a5f140a6639b.
Display the web scene
You can display a web scene using the web scene's item ID. Create a scene from the web scene portal item, and display it in your app.
-
In Visual Studio, in the Solution Explorer, double-click SceneViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Scene
contains the view model class for the application, calledView Model.cs Scene
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements at the top of the class.SceneViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using Esri.ArcGISRuntime.Portal; using System.Threading.Tasks;
-
In the SceneViewModel class, remove all the existing code in the
Setup
function.Scene() SceneViewModel.csUse dark colors for code blocks private void SetupScene() { // Create a new scene with an imagery basemap. Scene scene = new Scene(BasemapStyle.ArcGISImageryStandard); // Create an elevation source to show relief in the scene. string elevationServiceUrl = "http://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer"; ArcGISTiledElevationSource elevationSource = new ArcGISTiledElevationSource(new Uri(elevationServiceUrl)); // Create a Surface with the elevation data. Surface elevationSurface = new Surface(); elevationSurface.ElevationSources.Add(elevationSource); // Add an exaggeration factor to increase the 3D effect of the elevation. elevationSurface.ElevationExaggeration = 2.5; // Apply the surface to the scene. scene.BaseSurface = elevationSurface; // Create a point that defines the observer's (camera) initial location in the scene. // The point defines a longitude, latitude, and altitude of the initial camera location. MapPoint cameraLocation = new MapPoint(-118.804, 33.909, 5330.0, SpatialReferences.Wgs84); // Create a Camera using the point, the direction the camera should face (heading), and its pitch and roll (rotation and tilt). Camera sceneCamera = new Camera(locationPoint: cameraLocation, heading: 355.0, pitch: 72.0, roll: 0.0); // Create the initial point to center the camera on (the Santa Monica mountains in Southern California). // Longitude=118.805 degrees West, Latitude=34.027 degrees North MapPoint sceneCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); // Set an initial viewpoint for the scene using the camera and observation point. Viewpoint initialViewpoint = new Viewpoint(sceneCenterPoint, sceneCamera); scene.InitialViewpoint = initialViewpoint; // Set the view model "Scene" property. this.Scene = scene; }
A web scene can define all of the things that are created in this code, such as the basemap, elevation layer, and initial viewpoint. Loading a scene from a web scene usually requires less code, makes it easier to update a scene used by several apps, and can provide a more consistent experience for your user.
-
Modify the signature of the
Setup
function to include theScene() async
keyword and returnTask
rather thanvoid
.SceneViewModel.csUse dark colors for code blocks private async Task SetupScene() { }
When calling methods asynchronously inside a function (using the
await
keyword), theasync
keyword is required in the signature.Although a
void
return type would continue to work, this is not considered best practice. Exceptions thrown by anasync void
method cannot be caught outside of that method, are difficult to test, and can cause serious side effects if the caller is not expecting them to be asynchronous. The only circumstance whereasync void
is acceptable is when using an event handler, such as a button click.See the Microsoft documentation for more information about Asynchronous programming with async and await.
-
Modify the call to
Setup
(in theScene() Scene
constructor) to avoid a compilation warning. After changingView Model Setup
to an asynchronous method, the following warning appears in the Visual Studio Error List.Scene() Use dark colors for code blocks Copy Because this call is not awaited, execution of the current method continues before the call is completed. Consider applying the 'await' operator to the result of the call.
Because your code does not anticipate a return value from this call, the warning can be ignored. To be more specific about your intentions with this call and to address the warning, add the following code to store the return value in a discard.
SceneViewModel.csUse dark colors for code blocks public SceneViewModel() { _ = SetupScene(); }
From the Microsoft documentation:
"[Discards] are placeholder variables that are intentionally unused in application code. Discards are equivalent to unassigned variables; they don't have a value. A discard communicates intent to the compiler and others that read your code: You intended to ignore the result of an expression."
-
Add code to the
Setup
function to create aScene() PortalItem
for the web scene. To do this, provide the web scene's item ID and anArcGISPortal
referencing ArcGIS Online.SceneViewModel.csUse dark colors for code blocks private async Task SetupScene() { // Create a portal. If a URI is not specified, www.arcgis.com is used by default. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Get the portal item for a web scene using its unique item id. PortalItem sceneItem = await PortalItem.CreateAsync(portal, "579f97b2f3b94d4a8e48a5f140a6639b"); }
ArcGISPortal.CreateAsync()
is a static method that returns a new instance of theArcGISPortal
class. -
Create a
Scene
using thePortalItem
. To display the scene, set theScene
property to this newView Model. Scene Scene
.SceneViewModel.csUse dark colors for code blocks private async Task SetupScene() { // Create a portal. If a URI is not specified, www.arcgis.com is used by default. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Get the portal item for a web scene using its unique item id. PortalItem sceneItem = await PortalItem.CreateAsync(portal, "579f97b2f3b94d4a8e48a5f140a6639b"); // Create the scene from the item. Scene scene = new Scene(sceneItem); // To display the scene, set the SceneViewModel.Scene property, which is bound to the scene view. this.Scene = scene; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
Your app should display the scene that you viewed earlier in the Scene Viewer.
What's Next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: