Learn how to find an address or place with a search bar and the Geocoding service.
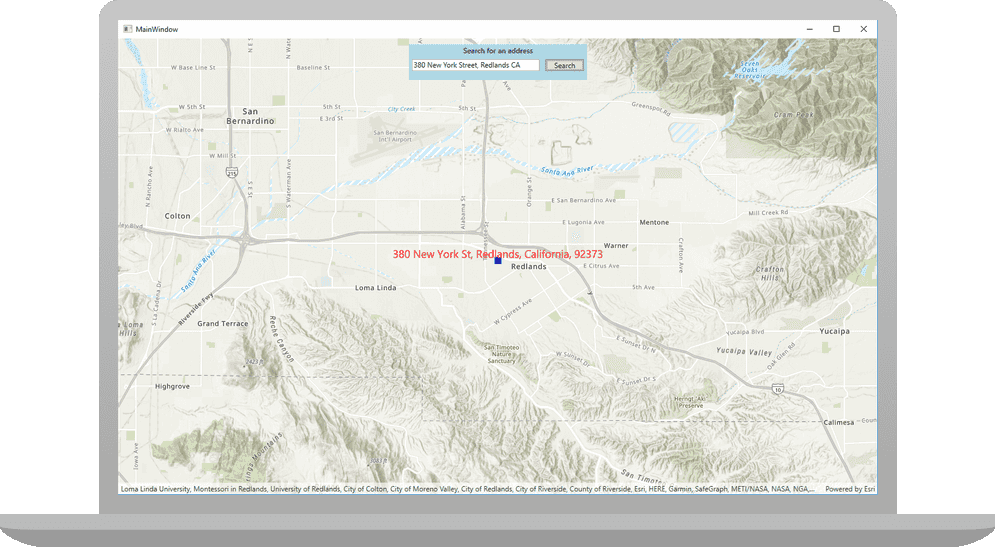
Geocoding is the process of converting address or place text into a location. The geocoding service can search for an address or a place and perform reverse geocoding.
In this tutorial, you use a search bar in the user interface to access the geocoding service and search for addresses and places.
To learn how to use the geocoding service to reverse geocode, visit the Reverse geocode tutorial.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Runtime SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Get an access token
You need an access token to use the location services used in this tutorial.
-
Go to the Create an API key tutorial to obtain an access token.
-
Ensure that the following privileges are enabled: Location services > Basemaps > Basemap styles service and Location services > Geocoding.
-
Copy the access token as it will be used in the next step.
To learn more about other ways to get an access token, go to Types of authentication.
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, set the
Api
property onKey ArcGIS
with the copied access token.Runtime Environment App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
If developing with Visual Studio for Windows, ArcGIS Runtime for .NET provides a set of project templates for each of the supported .NET platforms. These templates provide all of the code needed for a basic Model-View-ViewModel (MVVM) app. You need to install the ArcGIS Runtime for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Search
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Add a graphics overlay
A graphics overlay is a container for graphics. Graphics overlays are typically used to display temporary information, such as the results of an address search operation, and are displayed on top of all other layers. They do not persist when you save the map.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements to yourMap
class.View Model Esri.
contains classes used for geocoding (finding geographic locations from an address). TheArcGIS Runtime. Tasks. Geocoding Esri.
namespace contains theArcGIS Runtime. UI GraphicsOverlay
andGraphic
classes andEsri.
contains the classes that define the symbols for displaying them.ArcGIS Runtime. Symbology Use dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using Esri.ArcGISRuntime.Symbology; using Esri.ArcGISRuntime.Tasks.Geocoding; using Esri.ArcGISRuntime.UI; using System.Drawing; using System.Linq; using System.Threading.Tasks;
-
In the view model, create a new property named
Graphics
. This is a collection ofOverlays GraphicsOverlay
that will store geocode result graphics (address location and label).Use dark colors for code blocks private Map _map; public Map Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private GraphicsOverlayCollection _graphicsOverlayCollection; public GraphicsOverlayCollection GraphicsOverlays { get { return _graphicsOverlayCollection; } set { _graphicsOverlayCollection = value; OnPropertyChanged(); } }
-
At the end of the
Setup
function, add code to create a newMap GraphicsOverlay
, add it to a collection, and assign it to theGraphics
property.Overlays Use dark colors for code blocks // Set the view model "Map" property. this.Map = map; // Set the view model "GraphicsOverlays" property. GraphicsOverlay overlay = new GraphicsOverlay(); GraphicsOverlayCollection overlayCollection = new GraphicsOverlayCollection { overlay }; this.GraphicsOverlays = overlayCollection; }
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Use data binding to bind the
Graphics
property of theOverlays Map
to theView Model MapView
control.Data binding and the Model-View-ViewModel (MVVM) design pattern allow you to separate the logic in your app (the view model) from the presentation layer (the view). Graphics can be added and removed from the graphics overlays in the view model, and those changes appear in the map view through data binding.
Use dark colors for code blocks <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}"/>
Add a UI for user input
The user will type an address into a text box and then click a button to execute a search. The Map
class contains the logic to execute the search, but the controls are managed by the view.
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Add a
Border
above the map view that contains the address search controls.Use dark colors for code blocks <Grid> <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}"/> <Border Background="LightBlue" HorizontalAlignment="Center" VerticalAlignment="Top" Width="300" Height="60" Margin="0,10"> <Grid> <Grid.RowDefinitions> <RowDefinition Height="20"/> <RowDefinition Height="30" /> </Grid.RowDefinitions> <Grid.ColumnDefinitions> <ColumnDefinition Width="3*" /> <ColumnDefinition Width="*" /> </Grid.ColumnDefinitions> <TextBlock Text="Search for an address" Grid.Row="0" Grid.Column="0" Grid.ColumnSpan="2" TextAlignment="Center" VerticalAlignment="Center" FontWeight="SemiBold" /> <TextBox x:Name="AddressTextBox" Grid.Row="1" Grid.Column="0" Margin="5" Text="380 New York Street, Redlands CA"/> <Button x:Name="SearchAddressButton" Grid.Row="1" Grid.Column="1" Margin="5" Content="Search" Click="SearchAddressButton_Click" /> </Grid> </Border> </Grid>
Create a function to geocode an address
Geocoding is implemented with a locator, typically created by referencing a geocoding service such as the Geocoding service or, for offline geocoding, by referencing locator data contained in a mobile package. Geocoding parameters can be used to fine-tune the results, such as setting the maximum number of results or requesting additional attributes in the results.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
Keeping with the MVVM design pattern, the geocoding logic, along with any code unrelated to the user interface, is stored in the view model.
-
Add a new function to search for an address and return its geographic location. The address string and a spatial reference (for output locations) are passed to the function. The function is marked
async
since it will make asynchronous calls using theawait
keyword.Use dark colors for code blocks // Set the view model "Map" property. this.Map = map; // Set the view model "GraphicsOverlays" property. GraphicsOverlay overlay = new GraphicsOverlay(); GraphicsOverlayCollection overlayCollection = new GraphicsOverlayCollection { overlay }; this.GraphicsOverlays = overlayCollection; } public async Task<MapPoint> SearchAddress(string address, SpatialReference spatialReference) { MapPoint addressLocation = null; try { } catch (Exception ex) { System.Windows.MessageBox.Show("Couldn't find address: " + ex.Message); } // Return the location of the geocode result. return addressLocation; }
-
Get the
GraphicsOverlay
and clear all of itsGraphic
s.Use dark colors for code blocks public async Task<MapPoint> SearchAddress(string address, SpatialReference spatialReference) { MapPoint addressLocation = null; try { // Get the first graphics overlay from the GraphicsOverlays and remove any previous result graphics. GraphicsOverlay graphicsOverlay = this.GraphicsOverlays.FirstOrDefault(); graphicsOverlay.Graphics.Clear();
-
Create a
LocatorTask
based on the Geocoding service.A locator task is used to find the location of an address (geocode) or to interpolate an address for a location (reverse geocode). An address includes any type of information that distinguishes a place. A locator involves finding matching locations for a given address. Reverse-geocoding is the opposite and finds the closest address for a given location.
Use dark colors for code blocks // Get the first graphics overlay from the GraphicsOverlays and remove any previous result graphics. GraphicsOverlay graphicsOverlay = this.GraphicsOverlays.FirstOrDefault(); graphicsOverlay.Graphics.Clear(); // Create a locator task. LocatorTask locatorTask = new LocatorTask(new Uri("https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"));
-
Create a new
GeocodeParameters
and define some of its properties.- Add the names of attributes to return to the
Result
collection. An asterisk (Attribute Names *
) indicates all attributes. - Set the maximum number of results to be returned with
Max
. Results are ordered byResults score
, so that the first result has the best match score (ranging from 0 for no match to 100 for the best match). - Set the spatial reference for result locations with
Output
. By default, the output spatial reference is defined by the geocode service. For optimal performance when displaying the geocode result, you can ensure that returned coordinates match those of the map view by providing the map view's spatial reference.Spatial Reference
When geocoding an address, you can optionally provide
GeocodeParameters
to control certain aspects of the geocoding operation and specify the kinds of results to return from the locator task. Learn more about these parameters in the API documentation. For a list of attributes returned with geocode results, see Geocoding service output in the ArcGIS services reference.Use dark colors for code blocks // Create a locator task. LocatorTask locatorTask = new LocatorTask(new Uri("https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer")); // Define geocode parameters: limit the results to one and get all attributes. GeocodeParameters geocodeParameters = new GeocodeParameters(); geocodeParameters.ResultAttributeNames.Add("*"); geocodeParameters.MaxResults = 1; geocodeParameters.OutputSpatialReference = spatialReference;
- Add the names of attributes to return to the
-
To find the location for the provided address, call
Geocode
onAsync locator
, providing theTask address
string andgeocode
. The result is a read-only list ofParameters Geocode
objects. In this tutorial, either one or zero results will be returned, as the maximum results parameter was set to 1.Result Use dark colors for code blocks // Define geocode parameters: limit the results to one and get all attributes. GeocodeParameters geocodeParameters = new GeocodeParameters(); geocodeParameters.ResultAttributeNames.Add("*"); geocodeParameters.MaxResults = 1; geocodeParameters.OutputSpatialReference = spatialReference; // Geocode the address string and get the first (only) result. IReadOnlyList<GeocodeResult> results = await locatorTask.GeocodeAsync(address, geocodeParameters); GeocodeResult geocodeResult = results.FirstOrDefault(); if(geocodeResult == null) { throw new Exception("No matches found."); }
Display the result
The geocode result can be displayed by adding a graphic to the map view's graphics overlay.
-
If a result was found, create two
Graphic
s and add them to thegraphics
. Create a graphic to display the geocode result's location, and another to show the geocode result's label text (the located address).Overlay Use dark colors for code blocks // Geocode the address string and get the first (only) result. IReadOnlyList<GeocodeResult> results = await locatorTask.GeocodeAsync(address, geocodeParameters); GeocodeResult geocodeResult = results.FirstOrDefault(); if(geocodeResult == null) { throw new Exception("No matches found."); } // Create a graphic to display the result location. SimpleMarkerSymbol markerSymbol = new SimpleMarkerSymbol(SimpleMarkerSymbolStyle.Square, Color.Blue, 12); Graphic markerGraphic = new Graphic(geocodeResult.DisplayLocation, geocodeResult.Attributes, markerSymbol); // Create a graphic to display the result address label. TextSymbol textSymbol = new TextSymbol(geocodeResult.Label, Color.Red, 18, HorizontalAlignment.Center, VerticalAlignment.Bottom); Graphic textGraphic = new Graphic(geocodeResult.DisplayLocation, textSymbol); // Add the location and label graphics to the graphics overlay. graphicsOverlay.Graphics.Add(markerGraphic); graphicsOverlay.Graphics.Add(textGraphic);
-
Set
address
with the location of the result to return it from the function. The calling function will use thisLocation MapPoint
to pan the display to the result location.Use dark colors for code blocks // Add the location and label graphics to the graphics overlay. graphicsOverlay.Graphics.Add(markerGraphic); graphicsOverlay.Graphics.Add(textGraphic); // Set the address location to return from the function. addressLocation = geocodeResult.DisplayLocation;
Search for an address when the button is clicked
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml.cs to open the file.
A view defined with XAML, such as
Main
, is composed of two files in a Visual Studio project. The visual elements (such as map views, buttons, text boxes, and so on) are defined with XAML markup in aWindow .xaml
file. This file is often referred to as the "page". The code for the XAML elements is stored in an associated.xaml.cs
file that is known as the "code-behind", since it stores the code behind the controls. Keeping with the MVVM design pattern, user interface events (such as the code that responds to a button click) are handled in the code-behind for the view. -
Add a handler for the
Search
click event.Address Button Asynchronous functions should return
Task
orTask
. For event handlers, such as the<T > Button.
handler, the function must returnClick void
to conform to the delegate signature. See the Microsoft documentation regarding Async return types.Use dark colors for code blocks public MainWindow() { InitializeComponent(); // Create a point centered on the Santa Monica mountains in Southern California. // Longitude=118.805 degrees West, Latitude=34.027 degrees North var mapCenterPoint = new Esri.ArcGISRuntime.Geometry.MapPoint(-118.805, 34.027, Esri.ArcGISRuntime.Geometry.SpatialReferences.Wgs84); // Create a viewpoint for the initial display of the map. // Use the point defined above and a scale of 1:100,000 MainMapView.SetViewpoint(new Esri.ArcGISRuntime.Mapping.Viewpoint(mapCenterPoint, 100_000)); } private async void SearchAddressButton_Click(object sender, RoutedEventArgs e) { }
-
Find
Map
from the page resources.View Model Use dark colors for code blocks private async void SearchAddressButton_Click(object sender, RoutedEventArgs e) { // Get the MapViewModel from the page (defined as a static resource). MapViewModel viewModel = FindResource("MapViewModel") as MapViewModel; }
-
Call the
Search
function on theAddress Map
and store the returnedView Model MapPoint
.Use dark colors for code blocks private async void SearchAddressButton_Click(object sender, RoutedEventArgs e) { // Get the MapViewModel from the page (defined as a static resource). MapViewModel viewModel = FindResource("MapViewModel") as MapViewModel; // Call SearchAddress on the view model, pass the address text and the map view's spatial reference. Esri.ArcGISRuntime.Geometry.MapPoint addressPoint = await viewModel.SearchAddress(AddressTextBox.Text, MainMapView.SpatialReference); }
-
If a map point is returned, center the
MapView
on the address result.Use dark colors for code blocks private async void SearchAddressButton_Click(object sender, RoutedEventArgs e) { // Get the MapViewModel from the page (defined as a static resource). MapViewModel viewModel = FindResource("MapViewModel") as MapViewModel; // Call SearchAddress on the view model, pass the address text and the map view's spatial reference. Esri.ArcGISRuntime.Geometry.MapPoint addressPoint = await viewModel.SearchAddress(AddressTextBox.Text, MainMapView.SpatialReference); // If a result was found, center the display on it. if (addressPoint != null) { await MainMapView.SetViewpointCenterAsync(addressPoint); } }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see the address search controls at the top center of the map. To search for an address, enter an address and then click Search. If a result is found, the map will pan to the location and label a graphic for that address.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: