Learn how to change a basemap style in a map using the basemap styles service.
The basemap styles service provides a number basemap layer styles such as topography, streets, outdoor, navigation, and imagery that you can use in maps.
In this tutorial, you use a <select
dropdown menu with ol-mapbox-style
to toggle between a number of different basemap layer styles.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`; olms.apply(map, basemapURL);
To learn about the other types of authentication available, go to Types of authentication.
Remove basemap references
-
Remove the
basemap
,Id basemap
, and theURL olms
function.Use dark colors for code blocks <script> const map = new ol.Map({ target: "map" }); map.setView( new ol.View({ center: ol.proj.fromLonLat([-118.805, 34.027]), zoom: 12 }) ); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`; olms.apply(map, basemapURL); </script>
Add a basemap selector
OpenLayers does not have a basemap layer switching widget, so you will use the a plain HTML <select
element.
-
In the
<head
tag, add CSS that will position the> <select
menu wrapper element in the upper right corner and provide styling.> Use dark colors for code blocks <style> html, body, #map { padding: 0; margin: 0; height: 100%; width: 100%; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } #basemaps-wrapper { position: absolute; top: 20px; right: 20px; } #basemaps { font-size: 16px; padding: 4px 8px; } </style>
-
In the
<body
element, add the wrapper tag with an id of> basemaps-wrapper
.Use dark colors for code blocks <body> <div id="map"></div> <div id="basemaps-wrapper"> </div>
-
In the wrapper element, add a
<select
element with basemap layer enumerations and labels.> Use dark colors for code blocks <div id="basemaps-wrapper"> <select id="basemaps"> <option value="arcgis/outdoor">arcgis/outdoor</option> <option value="arcgis/community">arcgis/community</option> <option value="arcgis/navigation">arcgis/navigation</option> <option value="arcgis/streets">arcgis/streets</option> <option value="arcgis/streets-relief">arcgis/streets-relief</option> <option value="arcgis/imagery">arcgis/imagery</option> <option value="arcgis/oceans">arcgis/oceans</option> <option value="arcgis/topographic">arcgis/topographic</option> <option value="arcgis/light-gray">arcgis/light-gray</option> <option value="arcgis/dark-gray">arcgis/dark-gray</option> <option value="arcgis/human-geography">arcgis/human-geography</option> <option value="arcgis/charted-territory">arcgis/charted-territory</option> <option value="arcgis/nova">arcgis/nova</option> <option value="osm/standard">osm/standard</option> <option value="osm/navigation">osm/navigation</option> <option value="osm/streets">osm/streets</option> <option value="osm/blueprint">osm/blueprint</option> </select> </div>
Set the initial basemap layer
When the page loads, initialize the basemap layer to the first option in the menu.
-
Create a function called
url
that computes the style service URL for a basemap layer.Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const map = new ol.Map({ target: "map" }); map.setView( new ol.View({ center: ol.proj.fromLonLat([-118.805, 34.027]), zoom: 12 }) ); const baseUrl = "https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles"; const url = (style) => `${baseUrl}/${style}?token=${accessToken}`;
-
Create a function called
set
that will clear existing layers and useBasemap olms
to instantiate a basemap layer by name.Use dark colors for code blocks const baseUrl = "https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles"; const url = (style) => `${baseUrl}/${style}?token=${accessToken}`; const setBasemap = (style) => { // Clear out existing layers. map.setLayerGroup(new ol.layer.Group()); // Instantiate the given basemap layer. olms.apply(map, url(style)); };
-
Call
set
to initialize the basemap layer to theBasemap arcgis/outdoor
enumeration to match the first basemap in theselect
element.Use dark colors for code blocks const setBasemap = (style) => { // Clear out existing layers. map.setLayerGroup(new ol.layer.Group()); // Instantiate the given basemap layer. olms.apply(map, url(style)); }; setBasemap("arcgis/outdoor");
Set the selected basemap layer
Call set
when the user selects an option in the <select
menu.
-
Extract the
<select
DOM element using> document.query
.Selector Use dark colors for code blocks setBasemap("arcgis/outdoor"); const basemapsSelectElement = document.querySelector("#basemaps");
-
Call
set
in response toBasemap change
events on the<select
element.> Use dark colors for code blocks const basemapsSelectElement = document.querySelector("#basemaps"); basemapsSelectElement.addEventListener("change", (e) => { setBasemap(e.target.value); });
Run the app
In CodePen, run your code to display the map.
Use the layer switcher menu at the top right to select and explore different basemap layer styles.
What's Next?
Learn how to use additional ArcGIS location services in these tutorials:
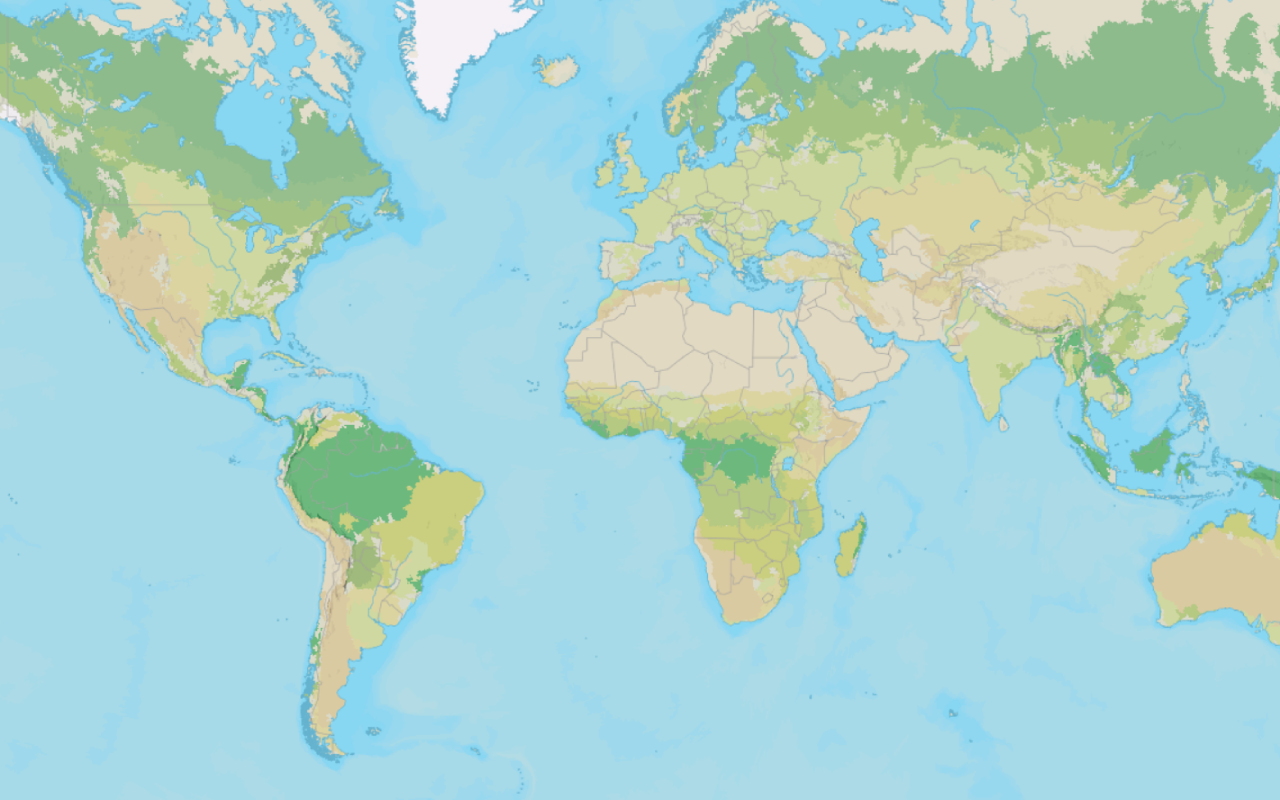
Change the basemap style
Switch a basemap style in a map using the basemap styles service.
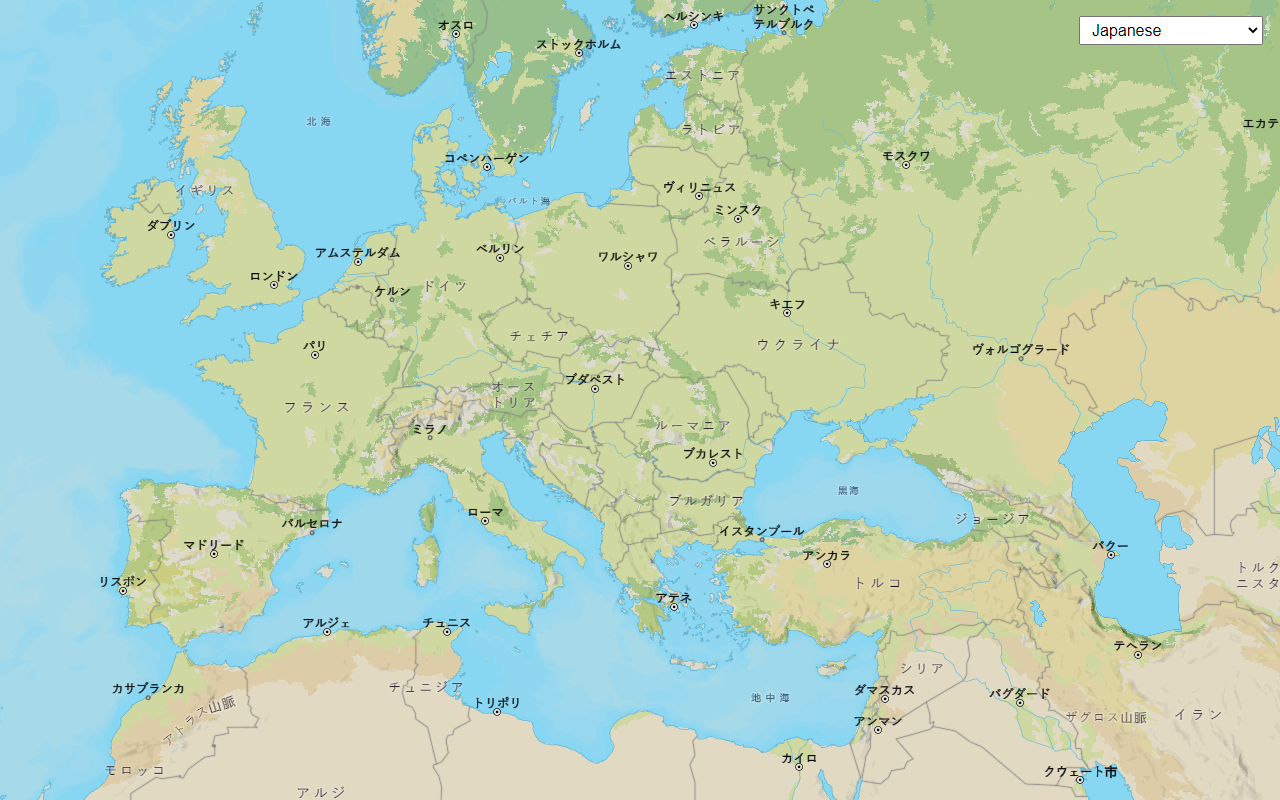
Change the place label language
Switch the language of place labels on a basemap.
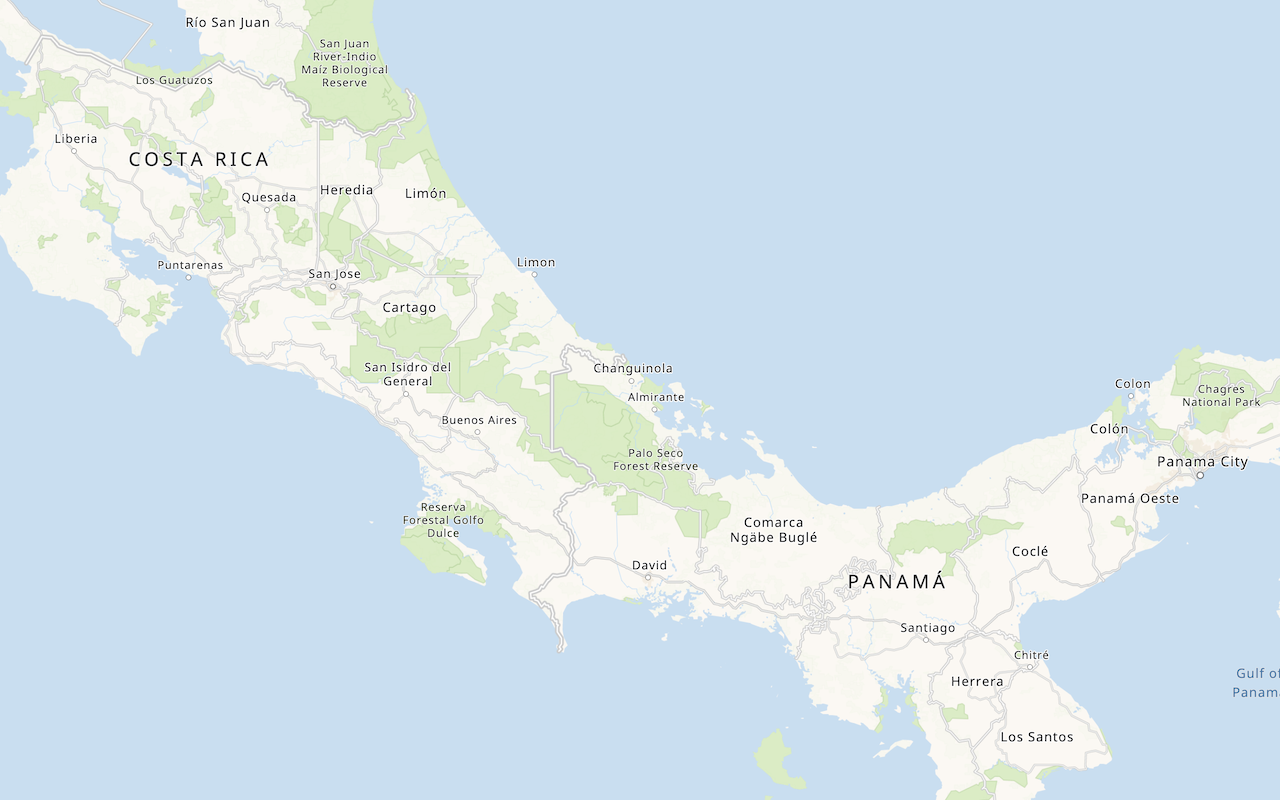
Display a custom basemap style
Add a styled vector basemap layer to a map.
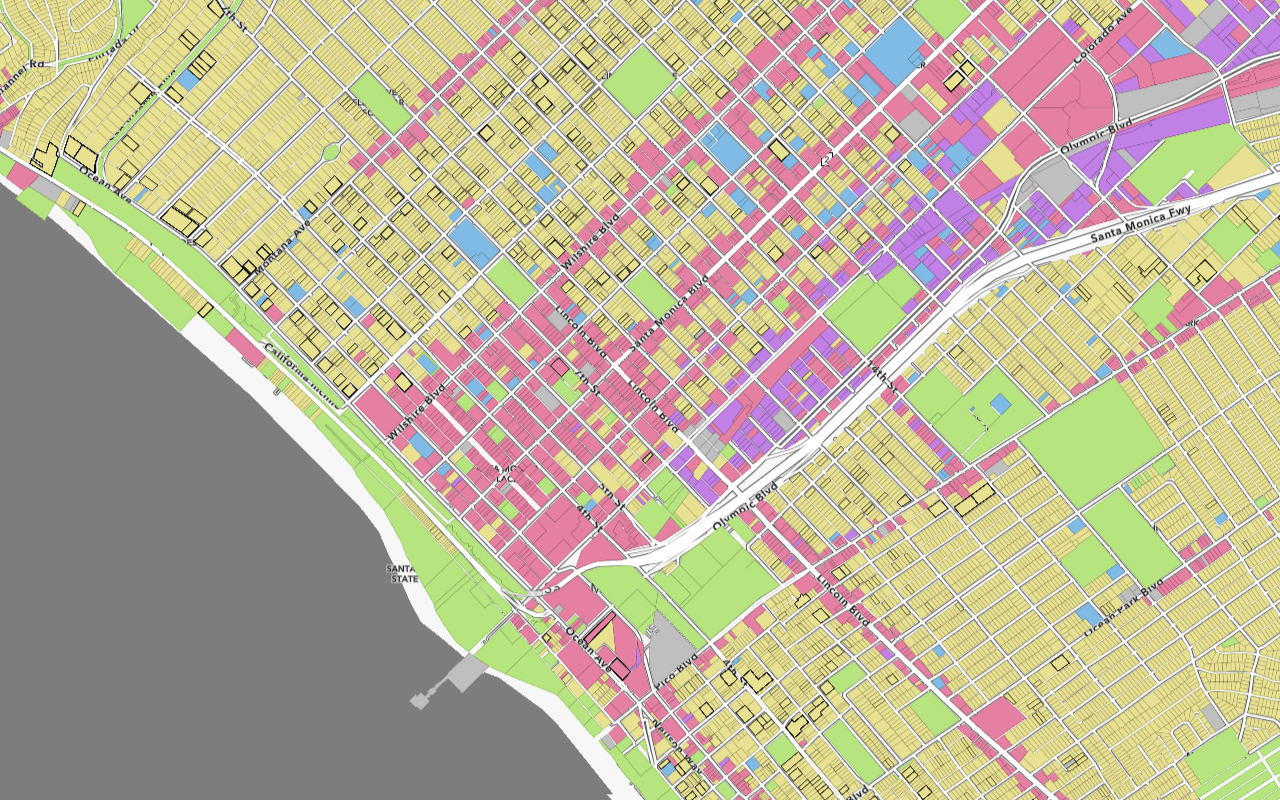
Style vector tiles
Change the fill and outline of vector tiles based on their attributes.