Learn how to perform a spatial query to a feature service.
A feature layer can contain a large number of features stored in ArcGIS. To access a subset of these features, you can execute an SQL or spatial query, either together or individually. The results can contain the attributes, geometry, or both for each record. SQL and spatial queries are useful when a feature layer is very large and you want to access only a subset of its data.
In this tutorial, you use the OpenLayers Draw
interaction to sketch a feature on the map and ArcGIS REST JS to perform a spatial query against the LA County Parcels hosted feature layer. The layer contains ±2.4 million features. The spatial query returns all of the parcels that intersect the sketched feature. A pop-up is also used to display feature attributes.
Prerequisites
An ArcGIS Location Platform or ArcGIS Online account.
Steps
Get the starter app
Select a type of authentication below and follow the steps to create a new application.
Set up authentication
Create developer credentials in your portal for the type of authentication you selected.
Set developer credentials
Use the API key or OAuth developer credentials so your application can access location services.
Add references
This tutorial uses ArcGIS REST JS to access a feature layer, and the ol-popup library to display pop-ups.
-
In the
<head
element, add references to the ArcGIS REST JS and ol-popup libraries.> Use dark colors for code blocks <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@v10.1.0/ol.css" type="text/css" /> <script src="https://cdn.jsdelivr.net/npm/ol@v10.1.0/dist/ol.js"></script> <script src="https://cdn.jsdelivr.net/npm/ol-mapbox-style@12.3.5/dist/olms.js" type="text/javascript"></script> <script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script> <script src="https://unpkg.com/@esri/arcgis-rest-feature-service@4.0.0/dist/bundled/feature-service.umd.js"></script> <script src="https://unpkg.com/ol-popup@5.1.1/dist/ol-popup.js"></script> <link rel="stylesheet" href="https://unpkg.com/ol-popup@5.1.1/src/ol-popup.css" />
Add layers
You will need two Vector
layers. interaction
will display the query polygon while the user is drawing it. Once the query returns, parcel
will display the individual property parcels in the default style. Each contains a Vector
source that initially contains no features.
In order to be able to draw shapes, you create a Draw
Interaction, connected to your interaction
. By setting its type to Polygon
, it only allows polygon features to be drawn.
-
Add a map load handler to the
olms
initialization. Inside, defineVector
layersparcel
andLayer interaction
. Provide a blackLayer Stroke
and blueFill
style forparcel
. Add each to the map withLayer map.add
.Layer Use dark colors for code blocks olms.apply(map, basemapURL).then(function (map) { const parcelLayer = new ol.layer.Vector({ source: new ol.source.Vector({ }), style: new ol.style.Style({ stroke: new ol.style.Stroke({ color: "black" }), fill: new ol.style.Fill({ color: "hsl(200,80%,50%)" }) }) }); const interactionLayer = new ol.layer.Vector({ source: new ol.source.Vector() }); map.addLayer(parcelLayer); map.addLayer(interactionLayer);
-
Add the data attribution for the feature layer source.
- Go to the LA County Parcels item.
- Scroll down to the Credits (Attribution) section and copy its value.
- Create an
attribution
property and paste the attribution value from the item.Use dark colors for code blocks olms.apply(map, basemapURL).then(function (map) { const parcelLayer = new ol.layer.Vector({ source: new ol.source.Vector({ // Attribution text retrieved from https://arcgis.com/home/item.html?id=a6fdf2ee0e454393a53ba32b9838b303 attributions: ['| County of Los Angeles Office of the Assessor'] }), style: new ol.style.Style({ stroke: new ol.style.Stroke({ color: "black" }), fill: new ol.style.Fill({ color: "hsl(200,80%,50%)" }) }) }); const interactionLayer = new ol.layer.Vector({ source: new ol.source.Vector() }); map.addLayer(parcelLayer); map.addLayer(interactionLayer);
-
Create a
Draw
interaction, linked tointeraction
, and with a type ofLayer Polygon
. Add it to the map withmap.add
.Interaction Use dark colors for code blocks map.addLayer(parcelLayer); map.addLayer(interactionLayer); const drawInteraction = new ol.interaction.Draw({ source: interactionLayer.getSource(), type: "Polygon", stopClick: true // don't fire "click" events (needed later) }); map.addInteraction(drawInteraction);
-
In the top right, click Run. You can now click to create multiple polygons.
Execute the query
Use the ArcGIS REST JS query
method to find features in the LA County Parcels feature layer that intersect the sketched feature. When the matching parcels are returned, use an Esri
feature format to read the features and add them to the parcel layer's source.
-
Create a function called
execute
with aQuery geometry
parameter and then callarcgis
.Rest.query Features -
Pass the
geometry
. Specify JSON as the return type and specifyreturn
. All of the features within the geometry will be returned.Geometry There are many other spatial relationships that you can specify with
spatial
. For example, you can useRel esri
to only return parcels within the sketched polygon. See the ArcGIS services reference for details.Spatial Rel Contains Use dark colors for code blocks map.addInteraction(drawInteraction); function executeQuery(geometry) { arcgisRest .queryFeatures({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0", geometry: geometry, geometryType: "esriGeometryPolygon", spatialRel: "esriSpatialRelIntersects", f: "json", returnGeometry: true }) }
-
Add a response handler. Inside, use an
Esri
feature format to set the returned parcels as the parcel layer's source.JSON Use dark colors for code blocks function executeQuery(geometry) { arcgisRest .queryFeatures({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0", geometry: geometry, geometryType: "esriGeometryPolygon", spatialRel: "esriSpatialRelIntersects", f: "json", returnGeometry: true }) .then((response) => { const features = new ol.format.EsriJSON().readFeatures(response); parcelLayer.getSource().addFeatures(features); }); }
Trigger the query
When the user completes a polygon, the drawend
event is fired, with an object containing the new polygon as a feature
attribute. You can use this feature to call execute
.
Use the Esri
feature format to convert the query polygon into the ArcGIS geometry format. It is not necessary to reproject the data.
-
Add a handler for the
draw
event. Inside, use anEnd Esri
feature format to convert the feature into an ArcGIS JSON format. Ensure the map's spatial reference information is included, by setting theJSON feature
property. CallProjection execute
with this feature's geometry.Query Use dark colors for code blocks map.addInteraction(drawInteraction); drawInteraction.on("drawend", (e) => { const feature = new ol.format.EsriJSON().writeFeatureObject(e.feature, { featureProjection: map.getView().getProjection() }); executeQuery(feature.geometry); });
-
At the top right, click Run. When you draw a polygon, a spatial query will run against the feature layer and display all land parcels within the boundary of the feature. Interaction polygons and parcels returned from previous queries remain visible. You will address this next.
Add a pop-up
The parcels returned from the query contain many attributes, such as land value and land use type. You can make the parcels interactive by showing a pop-up when the user clicks on a parcel. You create a Popup
to display parcel attributes. It is a type of Overlay
so you add it to the map with map.add
.
In order for clicking to trigger a pop-up, and not draw another polygon, you need to enable and disable the draw interaction using set
. Disable the interaction when the user has drawn a complete polygon. Enable it again when the user clicks somewhere other than a parcel, to reset the map. Clear the parcels and interaction layer at the same time.
-
Create a
Popup
and save it to apopup
variable. Add it to the map withmap.add
.Overlay Use dark colors for code blocks .then((response) => { const features = new ol.format.EsriJSON().readFeatures(response); parcelLayer.getSource().addFeatures(features); }); } const popup = new Popup(); map.addOverlay(popup);
-
Create a map
click
event handler. Inside, usemap.get
to get the clicked parcel, if any. If there is one, useFeatures At Pixel popup.show
to display a pop-up containing the parcel's APN, use type, land value and taxation city.Use dark colors for code blocks const popup = new Popup(); map.addOverlay(popup); map.on("click", (event) => { let parcel = map.getFeaturesAtPixel(event.pixel, { layerFilter: (l) => l === parcelLayer })[0]; if (parcel) { // user clicked on a parcel to see more information about it const message = `<b>Parcel ${parcel.get("APN")}</b>` + `<br>Type: ${parcel.get("UseType")} <br>` + `Land value: ${parcel.get("Roll_LandValue")} <br>` + `Tax Rate City: ${parcel.get("TaxRateCity")}`; popup.show(event.coordinate, message); drawInteraction.abortDrawing(); } else { } });
-
If the user did not click a parcel, hide the pop-up with
popup.hide
. Clear each layer by callingclear
on itsSource
. Useset
to enableActive draw
.Interaction Use dark colors for code blocks popup.show(event.coordinate, message); drawInteraction.abortDrawing(); } else { // user clicked elsewhere on the map to reset popup.hide(); parcelLayer.getSource().clear(); interactionLayer.getSource().clear(); drawInteraction.setActive(true);
-
In the
drawend
event handler, useset
to disableActive draw
.Interaction Use dark colors for code blocks drawInteraction.on("drawend", (e) => { const feature = new ol.format.EsriJSON().writeFeatureObject(e.feature, { featureProjection: map.getView().getProjection() }); executeQuery(feature.geometry); drawInteraction.setActive(false);
Run the app
Run the app.
When you click on the map to draw a polygon, a spatial query will run against the feature layer and display all land parcels that intersect the boundary of the feature. You can click on a parcel to see a pop-up with information about the parcel, or click somewhere else to reset the map.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
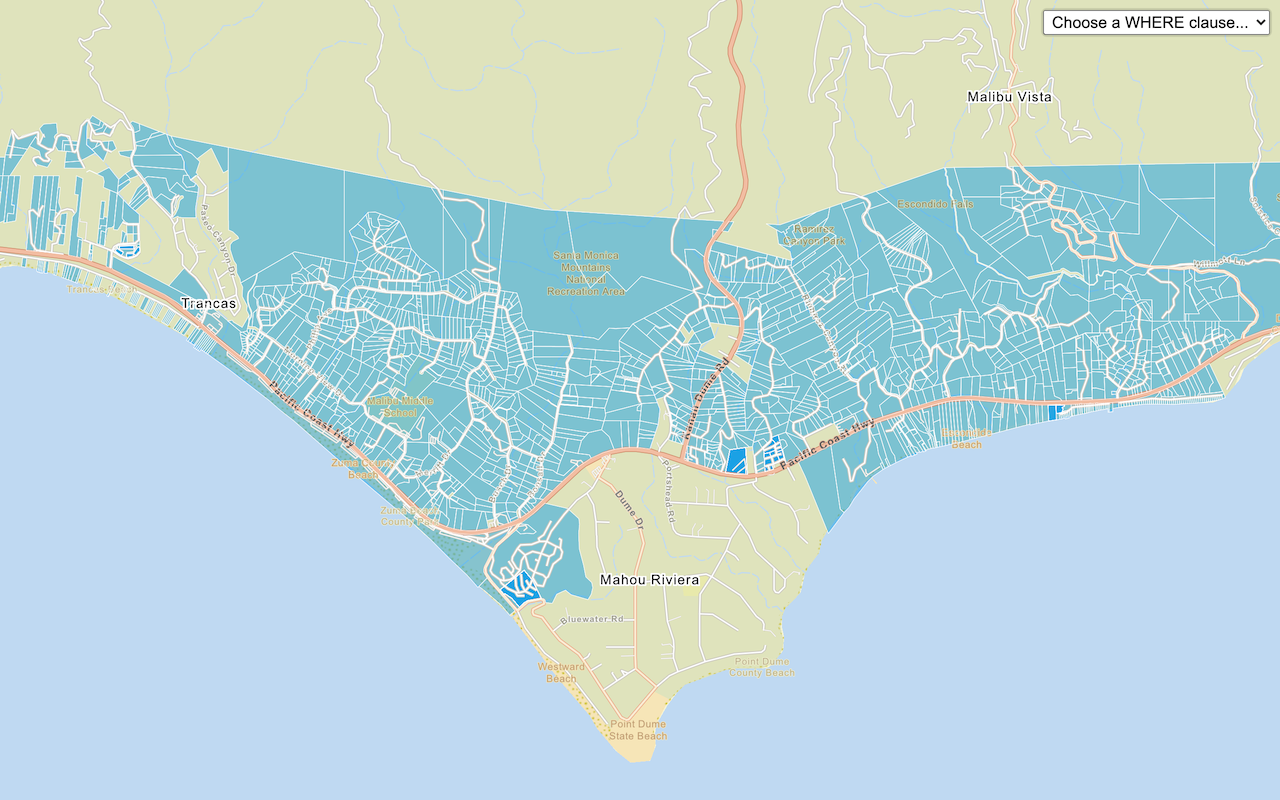
Query a feature layer (SQL)
Execute a SQL query to access polygon features from a feature layer.
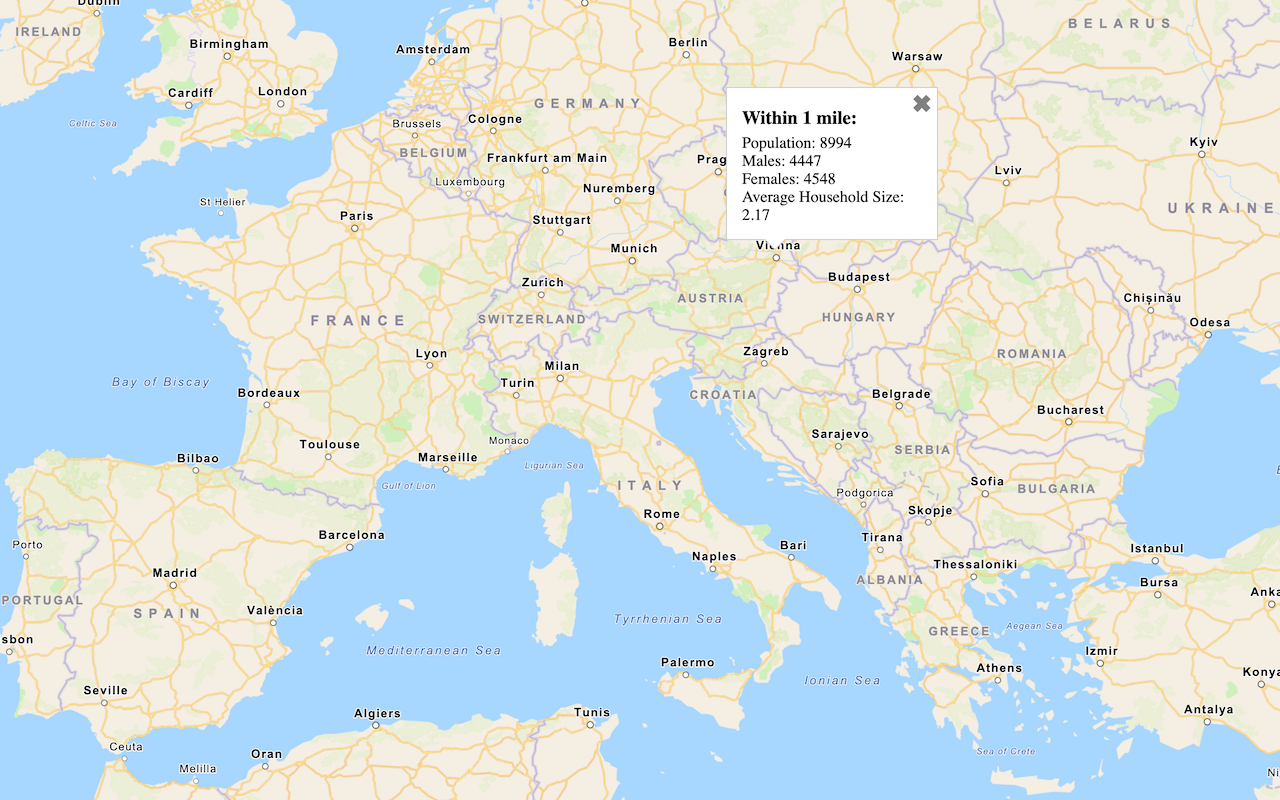
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
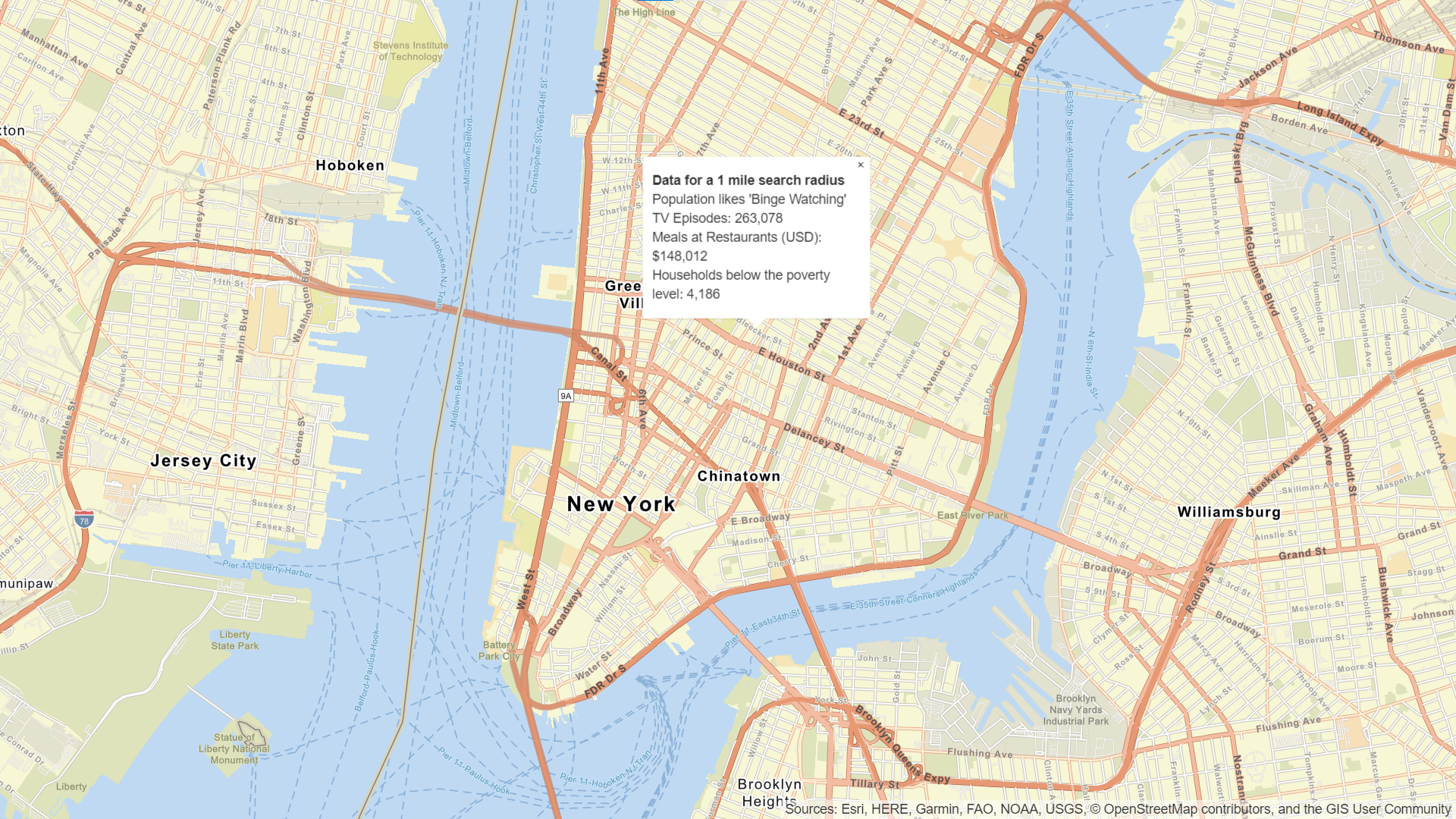
Get local data
Query regional facts, spending trends, and psychographics with the GeoEnrichment service.
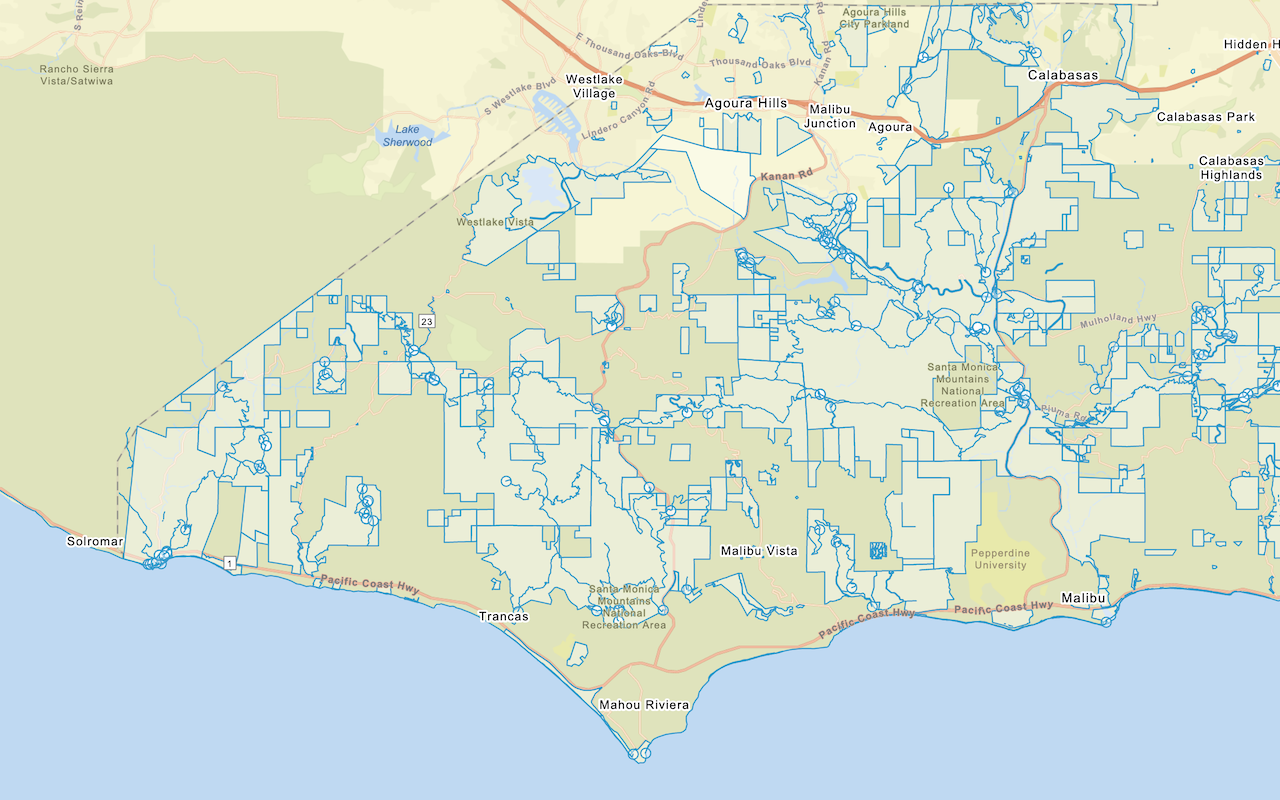
Add a feature layer
Add features from feature layers to a map.
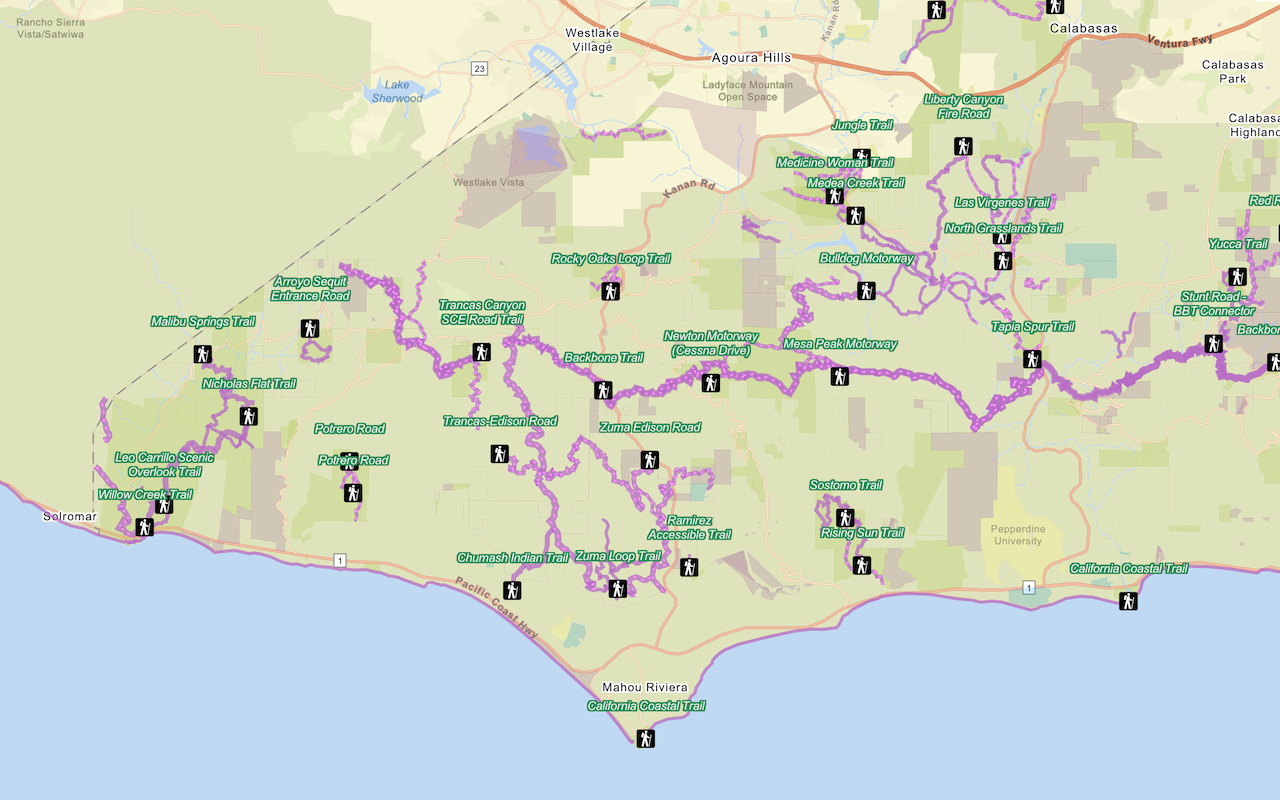
Style a feature layer
Use data-driven styling to apply symbol colors and styles to feature layers.