What is the concept of geocoding?
Geocoding
with ArcGIS, in short, is to convert your addresses and place names into co-ordinates and put them on a map.
A full explanation of the concept is also given as:
Geocoding is the process of transforming a description of a location — such as a pair of coordinates, an address, or a name of a place — to a location on the earth's surface. You can geocode by entering one location description at a time or by providing many of them at once in a table. The resulting locations are output as geographic features with attributes, which can be used for mapping or spatial analysis. You can quickly find various kinds of locations through geocoding. The types of locations that you can search for include points of interest or names from a gazetteer, like mountains, bridges, and stores; coordinates based on latitude and longitude or other reference systems, such as the Military Grid Reference System (MGRS) or the U.S. National Grid system; and addresses, which can come in a variety of styles and formats, including street intersections, house numbers with street names, and postal codes
The most popular use cases of geocoding include:
- Locate an address
- Search for places
- Search for intersections
- Find nearest address
- Batch geocoding
Ways to geocode
Using the geocode
module
For simple addresses, such as a street address, street name, or street intersection, you can perform the search using the geocode()
method. For best results, you should include as much location information as possible in the search in addition to the street address.
You can pass the address components as a single address string
or separated into multiple parameters using a dict object
. Examples of each are shown below. Note that in each case the response is the same for both the single and multiple parameter addresses.
To start with, import geocoding
and gis
modules, and initialize a GIS
connection to ArcGIS Online organization, or to ArcGIS Enterprise.
from arcgis.geocoding import geocode, Geocoder
from arcgis.gis import GIS
from arcgis.map.popups import PopupInfo
from arcgis.geometry import Point
Note: You can create the
GIS
with:
- username and password
- your profile name, e.g. `gis = GIS(profile='your_profile_name')
- with the
api_key
parameter, e.g. `gis = GIS(api_key='your api key')
- An
Application programming interface key
(API key
) is a permanent access token that defines the scope and permission for granting your public- facing application access to specific, ready-to-use services and private content. If you already have an ArcGIS account, you can sign in to view your default API key or to create a new API key. An API key is created for you when you sign up for an ArcGIS Developer account. For more, check out the API Keys Documentation.
gis = GIS(profile="your_online_profile")
single_line_address = "380 New York Street, Redlands, CA 92373"
# geocode the single line address
esrihq = geocode(single_line_address)[0]
esrihq['location'].update({"spatialReference" : {"wkid" : 4326}})
esri_pt = Point(esrihq['location'])
map = gis.map("Redlands, CA")
map
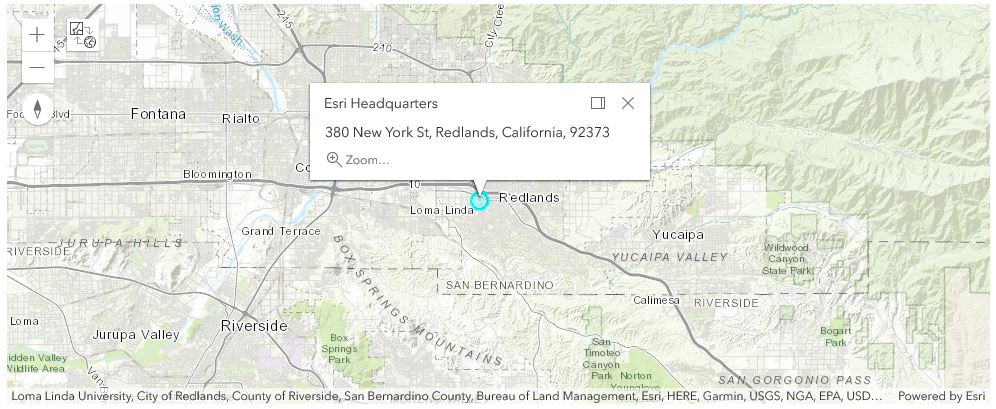
# plot the location of the first geocode result on the map
popup = PopupInfo(**{
"title" : "Esri Headquarters",
"description" : esrihq['address']
})
map.content.draw(esri_pt, popup)
Now, let's try inputing address in multiple parameters - the city component (Redlands
) as the value for the city parameter, the state component (CA
) as the region parameter, and the zip code (92373
) as the value for the postal parameter.
multi_field_address = {
"Address" : "380 New York Street",
"City" : "Redlands",
"Region" : "CA",
"Postal" : 92373
}
# geocode the multi_field_address
esrihq1 = geocode(multi_field_address)[0]
esrihq1['location'].update({"spatialReference" : {"wkid" : 4326}})
esri_pt1 = Point(esrihq1['location'])
map1 = gis.map("Redlands, CA")
map1
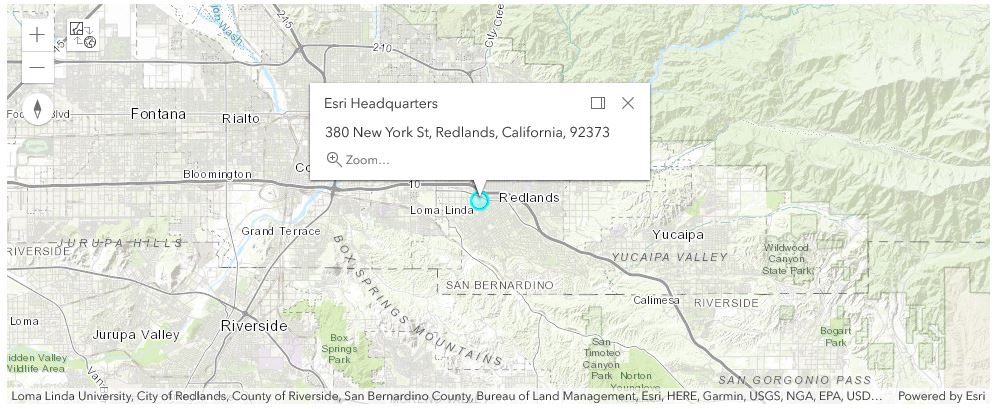
# plot the location of the first geocode result on the map
popup = PopupInfo(**{
"title" : "Esri Headquarters",
"description" : esrihq1['address']
})
map1.content.draw(esri_pt1, popup)
How geocoding works
What are geocoders and their types?
Geocoders
are tools that can find spatial coordinates of addresses, business names, places of interest and so on. The output points can be visualized on a map, inserted as stops for a route, or loaded as input for spatial analysis. They also used to generate batch results for a set of addresses, as well as for reverse geocoding, i.e. determining the address at a particular x/y location.
Geocoders
have numerous properties accessed through geocoder.properties
. These properties specify the types of information the geocode()
function accepts as input and the structure of the outputs when performing geocoding operations using a particular geocoder. A geocoder's properties also define any limits or requirements established by the underlying locator.
About ArcGIS World Geocoding Service
The ArcGIS World Geocoding Service
allows you to find addresses or places in the world, geocode a table of addresses, or reverse geocode
a location without the need to purchase the reference dataset for creating the locators. The service references rich and high-quality point and street address data, places, and gazetteers that cover much of the world. The World Geocoding Service is widely available. All you need to access it is a connection to an ArcGIS Online organization or Enterprise and the proper credentials, which may make it unnecessary for you to set up the service on your own instance of ArcGIS Server or enterprise. The service is intended to simplify the workflows of developers and GIS professionals.
A GIS
includes one or more geocoders
. The list of geocoders registered with the GIS can be queried using get_geocoders()
. This method returns a list of Geocoder instances. In the example below, there is one registered Geocoder with the GIS, that uses the Esri World Geocoding Service for geocoding:
from arcgis.geocoding import Geocoder, get_geocoders
my_geocoder = get_geocoders(gis)[0]
my_geocoder
<Geocoder url:"https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer">
The first available geocoder
in the active GIS is used by default, unless specified, and all geocoding functions have an optional parameter for specifying the geocoder to be used.
The newest GIS object being created will have its first available geocoder
as the default geocoder unless set_active=False
is passed in the GIS constructor. However, you can also use a different Geocoder
by specifying it explicitly as a method parameter.
Using the default geocoder
from arcgis.geocoding import geocode
results = geocode('New York St, Redlands, CA')
# query the first matched result
results[0]['location']
{'x': -117.195540331044, 'y': 34.064537763377}
Properties of the geocoder class
[prop_name for prop_name in my_geocoder.properties.keys()]
['currentVersion', 'serviceDescription', 'addressFields', 'categories', 'singleLineAddressField', 'candidateFields', 'spatialReference', 'locatorProperties', 'detailedCountries', 'countries', 'capabilities']
addressFields
property
The Geocoder's addressFields
property specifies the various address fields accepted by it when geocoding addresses. For instance, the address fields accepted by this geocoder, and their length, are the following:
for addr_fld in my_geocoder.properties.addressFields:
print(addr_fld['name'] + " (" + str(addr_fld['length']) +" chars)")
Address (100 chars) Address2 (100 chars) Address3 (100 chars) Neighborhood (50 chars) City (50 chars) Subregion (50 chars) Region (50 chars) Postal (20 chars) PostalExt (20 chars) CountryCode (100 chars)
singleLineAddressField
property
The geocoder may also support a single line address field. Single field input is easier because the address parsing is done for you; however, multi-field input may provide faster responses and more precise results. The field name can be found using the code below:
my_geocoder.properties.singleLineAddressField['name']
'SingleLine'
When using single line input for the address, it is unnecessary (though supported) to create a dict
with this key and the single line address as it's value. The address can be passed in directly as a text string.
One instance of when you might use a dict to include the SingleLine
parameter is when it is combined with the countryCode
parameter. The SingleLine
parameter cannot be used with any of the other multi-field parameters.
categories
property
The categories
property can be used to limit result to one or more categories. For example, "Populated Place" or "Scandinavian Food". It is only applicable to the World Geocoding Service. The following code lists the entire hierarchy of supported category values.
def list_categories(obj, depth = 0):
for category in obj['categories']:
print('\t'*depth + category['name'])
if 'categories' in category:
list_categories(category, depth + 1)
list_categories(my_geocoder.properties)
Address Subaddress Point Address Street Address Distance Marker Intersection Street Midblock Street Between Street Name Postal Primary Postal Postal Locality Postal Extension Coordinate System LatLong XY YX MGRS USNG Populated Place Block Sector Neighborhood District City Metro Area Subregion Region Territory Country Zone POI Arts and Entertainment Amusement Park Aquarium Art Gallery Art Museum Billiards Bowling Alley Casino Cinema Historical Monument History Museum Indoor Sports Jazz Club Landmark Library Live Music Museum Other Arts and Entertainment Performing Arts Ruin Science Museum Tourist Attraction Wild Animal Park Zoo Education College Fine Arts School Other Education School Vocational School Food African Food American Food Argentinean Food Australian Food Austrian Food Bakery Balkan Food BBQ and Southern Food Belgian Food Bistro Brazilian Food Breakfast Brewpub British Isles Food Burgers Cajun and Creole Food Californian Food Caribbean Food Chicken Restaurant Chilean Food Chinese Food Coffee Shop Continental Food Creperie East European Food Fast Food Filipino Food Fondue French Food Fusion Food German Food Greek Food Grill Hawaiian Food Ice Cream Shop Indian Food Indonesian Food International Food Irish Food Italian Food Japanese Food Korean Food Kosher Food Latin American Food Malaysian Food Mexican Food Middle Eastern Food Moroccan Food Other Restaurant Pastries Pizza Polish Food Portuguese Food Restaurant Russian Food Sandwich Shop Scandinavian Food Seafood Snacks South American Food Southeast Asian Food Southwestern Food Spanish Food Steak House Sushi Swiss Food Tapas Thai Food Turkish Food Vegetarian Food Vietnamese Food Winery Land Features Atoll Basin Butte Canyon Cape Cave Cliff Continent Desert Dune Flat Forest Glacier Grassland Hill Island Isthmus Lava Marsh Meadow Mesa Mountain Mountain Range Oasis Other Land Feature Peninsula Plain Plateau Point Ravine Ridge Rock Scrubland Swamp Valley Volcano Wetland Nightlife Spot Bar or Pub Dancing Karaoke Night Club Nightlife Parks and Outdoors Basketball Beach Campground Diving Center Fishing Garden Golf Course Golf Driving Range Hockey Ice Skating Rink Nature Reserve Other Parks and Outdoors Park Racetrack Scenic Overlook Shooting Range Ski Lift Ski Resort Soccer Sports Center Sports Field Swimming Pool Tennis Court Trail Wildlife Reserve Professional and Other Places Ashram Banquet Hall Border Crossing Building Business Facility Cemetery Church City Hall Civic Center Convention Center Court House Dentist Doctor Embassy Factory Farm Fire Station Government Office Gurdwara Hospital Industrial Zone Insurance Livestock Medical Clinic Military Base Mine Mosque Observatory Oil Facility Orchard Other Professional Place Other Religious Place Pagoda Place of Worship Plantation Police Station Post Office Power Station Prison Public Restroom Radio Station Ranch Recreation Facility Religious Center Scientific Research Shrine Storage Synagogue Telecom Temple Tower Veterinarian Vineyard Warehouse Water Tank Water Treatment Residence Estate House Nursing Home Residential Area Shops and Service ATM Auto Dealership Auto Maintenance Auto Parts Bank Beauty Salon Beauty Supplies Bookstore Butcher Candy Store Car Wash Childrens Apparel Clothing Store Consumer Electronics Store Convenience Store Delivery Service Department Store Electrical Fitness Center Flea Market Food and Beverage Shop Footwear Furniture Store Gas Station Grocery Home Improvement Store Jewelry Laundry Market Mens Apparel Mobile Phone Shop Motorcycle Shop Office Supplies Store Optical Other Shops and Service Pet Store Pharmacy Plumbing Repair Services Shopping Center Spa Specialty Store Sporting Goods Store Tire Store Toy Store Used Car Dealership Wholesale Warehouse Wine and Liquor Womens Apparel Yoga Studio Travel and Transport Airport Bed and Breakfast Bridge Bus Station Bus Stop Cargo Center Dock EV Charging Station Ferry Heliport Highway Exit Hostel Hotel Marina Metro Station Motel Other Travel Parking Pier Port Railyard Rental Cars Resort Rest Area Taxi Tollbooth Tourist Information Train Station Transportation Service Travel Agency Truck Stop Tunnel Weigh Station Water Features Abyssal Plain Bay Canal Channel Continental Rise Continental Shelf Continental Slope Cove Dam Delta Estuary Fjord Fracture Zone Gulf Harbor Hot Spring Irrigation Jetty Lagoon Lake Ocean Ocean Bank Oceanic Basin Oceanic Plateau Oceanic Ridge Other Water Feature Reef Reservoir Sea Seamount Shoal Sound Spring Strait Stream Submarine Canyon Submarine Cliff Submarine Fan Submarine Hill Submarine Terrace Submarine Valley Trench Undersea Feature Waterfall Well Wharf
About Custom Geocoding Service
Next, let's look at two ways to create a custom geocoder:
Creating a geocoder using a geocoding service item
Geocoding services can be published as items in the GIS. An instance of the geocoder can also be constructed by passing in a reference to these items from the GIS to the Geocoder's constructor:
from IPython.display import display
arcgis_online = GIS()
items = arcgis_online.content.search('Geocoder', 'geocoding service', max_items=3)
for item in items:
display(item)
# construct a geocoder using the first geocoding service item
worldgeocoder = Geocoder.fromitem(items[1])
worldgeocoder
<Geocoder url:"https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer">
Creating a geocoder from a geocoding service
Geocoders may also be created using the constructor by passing in their location, such as a url to a Geocoding Service. If the geocoding service is a secure service, pass in the GIS to which it is federated with as the gis parameter:
geocoder_url = worldgeocoder.url
esrinl_geocoder = Geocoder(geocoder_url, gis)
esrinl_geocoder
<Geocoder url:"https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer">
Next, let's look at an example below that shows calling the geocode() function to geocode an address. It specifies that the esrinl_geocoder created above should be used for geocoding by passing it in explicitly.
results = geocode(address='Raadhuisstraat 52, 1016 Amsterdam', geocoder=esrinl_geocoder)
results[0]['location']
{'x': 4.885574488382, 'y': 52.373987889092}
Features of geocoding services
What is needed to geocode?
Summarizing the requirements for performing geocode
that have been mentioned above, you need to meet either one of the two criteria:
- You need to have a subscription to the ArcGIS Online for organization. If not, then sign up for a developer account.
- You are using the ArcGIS Enterprise with custom geocoding service or Utility service configured. Refer to the Enterprise doc about this.
Geocoding capabilities
Generally the capabilities of geocoding include:
- Locate an address
- also known as
address search
, is the process of converting text for an address or place to a complete address with a location. For example, you can convert1600 Pennsylvania Ave NW, DC
to-77.03654 longitude and 38.89767 latitude
.
- also known as
- Search for places; User can use geocode() to
- Find the locations of geographic places around the world.
- Locate businesses near a location.
- Search for places: A user can use geocode() to find the locations of geographic places around the world, locate businesses near a location, search for places by category, such as restaurants, gas stations, or schools (The Geocoding Service allows you to search for different categories of places, such as businesses, restaurants, geographic features, and administrative areas), or find and display places on a map.
- Find and display places on a map.
- Search for intersections: In addition to addresses or street names, the Geocoding Service can search for and locate street intersections. An intersection is the point at which two streets cross each other. An intersection search consists of the intersecting street names plus the containing administrative division or postal code.
- Reverse geocode: The Geocoding Service can reverse geocode an x/y location and return the nearest address or place. Reverse geocoding is the process of converting a point to an address or place. For example, you can convert
-79.3871 longitude and 43.6426 latitude
toCN Tower, 301 Front St W, Toronto, Ontario, M5V, CAN
. Generally speaking, you can use reverse geocoding to: - Get the nearest address to your current location;
- Show an address or place name when you tap on a map;
- Find the address for a geographic location.
- Batch geocoding: Geocode place of interest or address queries with a single request using the Geocoding Service. An ArcGIS Developer or ArcGIS Online subscription is required to use the service. It is also known as bulk geocoding, the process of converting a list of addresses or place names to a set of complete addresses with locations. Batch geocoding is commonly used to: convert a number of addresses to complete addresses, find the locations for a list of addresses, perform large batches of geocoding, or geocode addresses that can be saved for future use.
- Convert a number of addresses to complete addresses. - Find the locations for a list of addresses. - Perform large batches of geocoding. - Geocode addresses that can be saved for future use.
Geocoding coverage
For countries covered by a geocoding service, e.g. the ArcGIS World Geocoding Service, the address coverage, the supported language, and relative geocoding quality would vary. The Geocoding Service Coverage
WebMap object displayed below illustrates the various levels.
The overall geocoding quality is a function of the degree of street-level address coverage in a country, knowledge of a country's address styles, and geocoding performance for addresses in that country. The quality levels are subject to change. Typically, the quality of geocoding for a given country improves over time and may be upgraded to a better level, but there may be instances where countries are downgraded to a lower level based on user feedback.
Countries for which there is no address coverage are classified as Level 4 and are white in the map. Even though there is no address or street-level coverage in these countries, there is still admin-level coverage available. This means that administrative divisions such as cities, counties, and provinces, as well as landmarks in some cases, can be found in them.
When multiple languages are listed in the Supported Languages column for a country, it does not necessarily mean that all addresses in the country are available in each language. In some cases, a language may only be supported within a particular region of a country or for a small subset of addresses. Generally, in the Level 1 and Level 2 tables, the first language listed is the primary language.
The information in the following tables only applies to street addresses and not to POIs (geocode candidates with Addr_type = POI).
geocode_coverage = gis.content.search("title:Geocoding Service Coverage type:Web Map", outside_org=True)[8]
geocode_coverage
from arcgis.map import Map
Map(geocode_coverage)
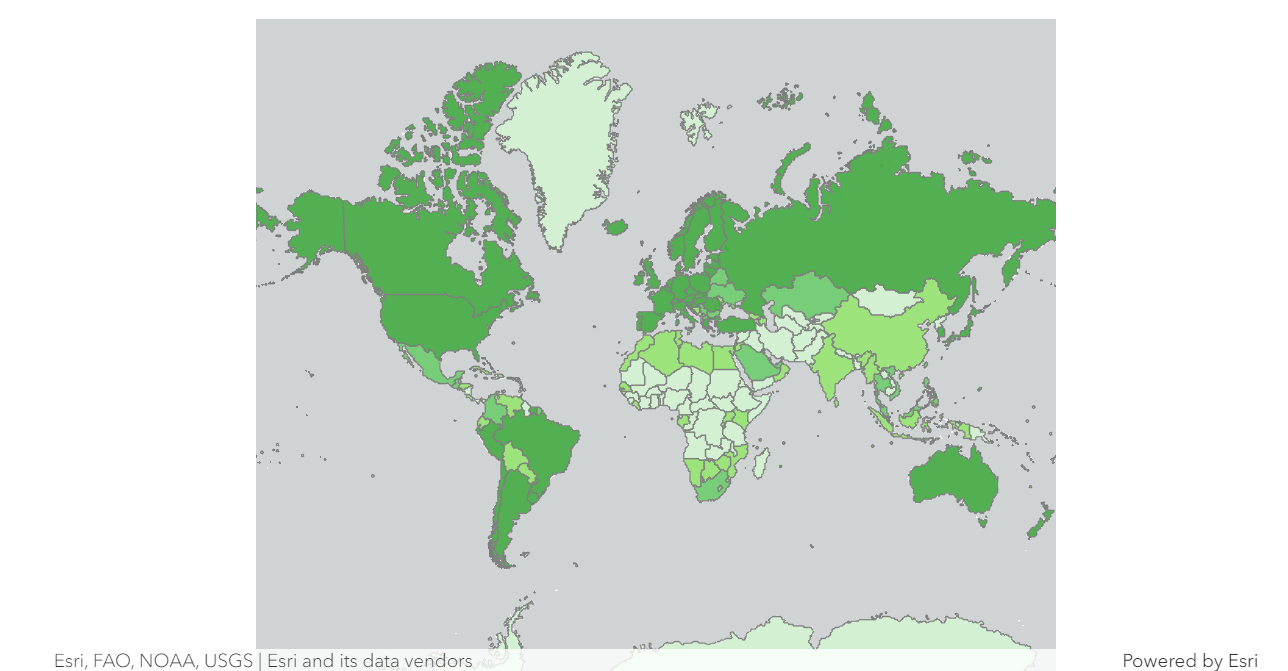
Level 1
The darkest-shaded countries in the map are Level 1. They provide the highest quality geocoding experience. Address searches are likely to result in accurate matches to PointAddress
and StreetAddress
levels.
Country Name | Supported Country Codes | Supported Languages | Supported Language Codes | Data Sources |
---|---|---|---|---|
Argentina | ARG, AR | Spanish | ES | HERE, Geonames |
Australia | AUS, AU | English | EN | PSMA Australia, HERE, Geonames |
Austria | AUT, AT | German, Croatian, Croatian Transliterated | DE, CR, EN | BEV, HERE, Geonames |
... | ... | ... | ... | ... |
Level 2
The medium-shaded countries in the map are Level 2. They provide a good geocoding experience. Address searches often result in PointAddress
and StreetAddress
level matches, but sometimes match to StreetName
and Admin
levels.
Country Name | Supported Country Codes | Supported Languages | Supported Language Codes | Data Sources |
---|---|---|---|---|
Andorra | AND, AD | Catalan | CA | HERE, Geonames |
Belarus | BLR, BY | Belarusian, Belarusian Transliterated, Russian, Russian Transliterated | BE, RU, EN | HERE, Geonames |
Bulgaria | BGR, BG | Bulgarian, Bulgarian Transliterated | BG, EN | HERE, Geonames |
... | ... | ... | ... | ... |
Level 3
The lightest-shaded countries in the map are Level 3. They provide a fair geocoding experience. Address searches will more likely match to StreetName
or Admin
levels.
Country Name | Supported Country Codes | Supported Languages | Supported Language Codes | Data Sources |
---|---|---|---|---|
Albania | ALB, AL | Albanian | SQ | HERE, Geonames |
Algeria | DZA, DZ | Arabic, French | AR, FR | HERE, Geonames |
American Samoa | ASM, AS, USA, US | English | EN | HERE, Geonames |
... | ... | ... | ... | ... |
Level 4
The unshaded countries in the map are Level 4. They only provide Admin-level
matches.
Cost of geocoding
Usage of ArcGIS World Geocoding Service operations can be paid-only (e.g., when performing geocode()
), or can be free (e.g., while using suggest()
). Other operations can be either free or paid, depending on whether you are using them for search capabilities only, such as temporarily displaying the results, or storing the results for later use (when using for_storage=True
). Please see the table below for more information, or see the Geocoding Pricing help doc..
Function | Description | Cost |
---|---|---|
geocode() | To match addresses | paid |
suggest() | To return a list of suggested matches for the input text | free |
reverse_geocode() | To find the address of a location (x and y coordinates) | free(view-only) / paid(to store results) |
To be more specific about paid services, for instance, for geocoding
a table of addresses, rematching
a geocoded feature class, and reverse geocoding
a feature class, the service operates under a credit-based usage model
that allows you to pay only for what you use. If you don't already have a subscription to an ArcGIS Online for organizations
, you can purchase one or request a free trial. For more information on the credit-based usage, see service credits for geocoding.
Function | Description | Cost |
---|---|---|
geocode() | To match addresses using ArcGIS World Geocoding Service or a view of this locator | 40 credits per 1,000 geocodes |
Layout of the arcgis.geocoding
module
The functions and the Geocoder
class defined in the geocoding
module can be seen in the Object Model Diagram drawn below:
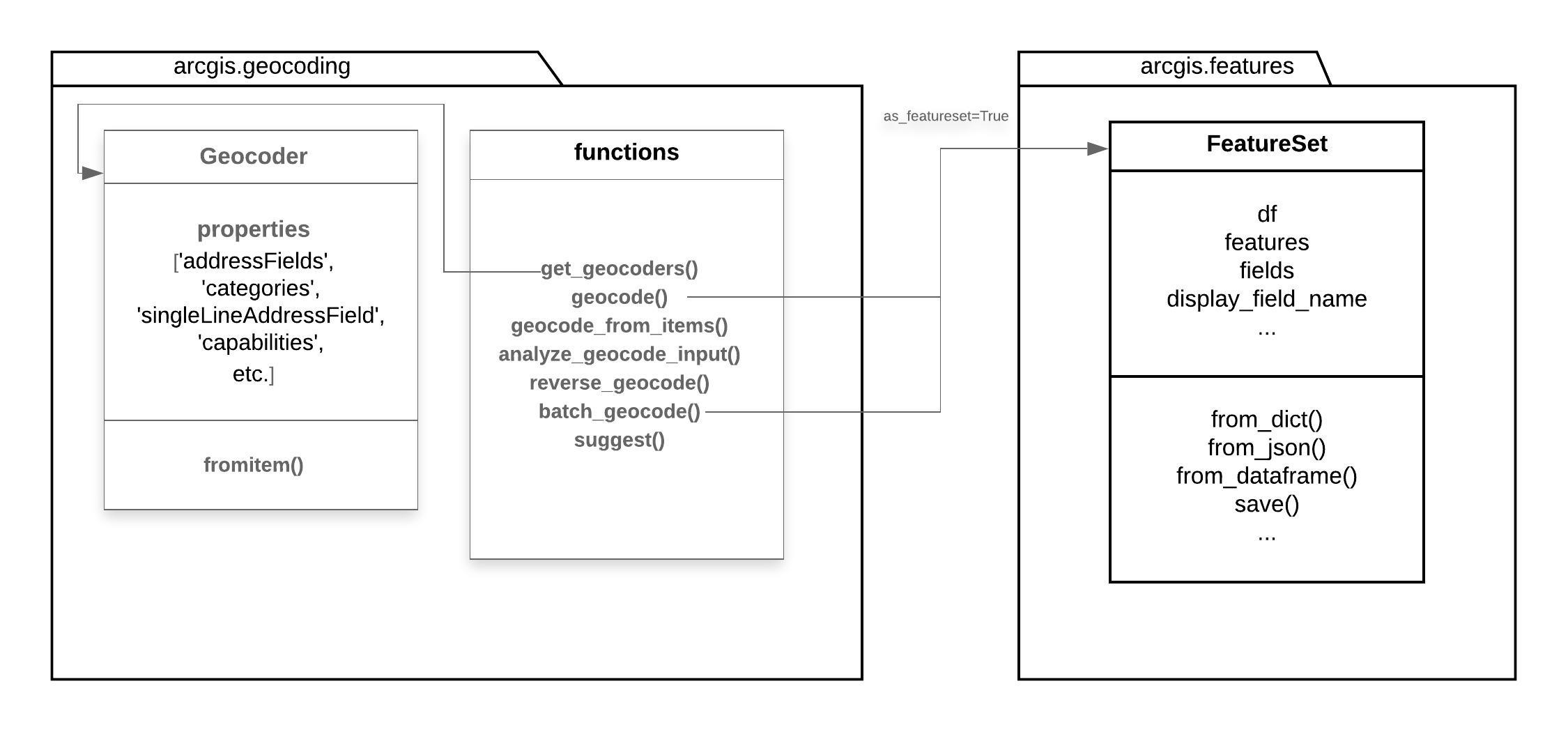
Conclusions
In Part 1 of the geocoding guides, we have discussed what is geocoding, two ways to perform geocoding, how geocoding works, and the requirements and costs needed to perform geocoding. For advanced topics of geocoding, please go to Part 2 of the sequential guides.