Learn how to execute a SQL query to access polygon features in a feature layer.
A feature layer can contain a large number of features. To access a subset of these features, you can execute an SQL or spatial query, either together or individually. The results can contain the attributes, geometry, or both for each record. SQL and spatial queries are useful when a feature layer is very large and you want to access only a subset of its data.
In this tutorial, you perform server-side SQL queries to return a subset of the features from the LA County Parcels feature service. The feature layer contains over 2.4 million features.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Steps
Import modules and log in to portal
-
Import the
arcgis.gis
module.Use dark colors for code blocks from arcgis.gis import GIS
-
Create an anonymous connection to ArcGIS Online to access public data. Since this dataset is public you do not need credentials to access it. If it were a private dataset, you would be required to log in
Use dark colors for code blocks from arcgis.gis import GIS portal = GIS()
Access the feature layer by itemId
-
Use the
Content
class to access the dataset by Item ID.Manager Use dark colors for code blocks portal = GIS() parcel_layer_item = portal.content.get("a6fdf2ee0e454393a53ba32b9838b303") parcel_layer = parcel_layer_item.layers[0]
Run the query
-
Create a string variable containing the SQL statement. This will query only the features of this feature layer where the
Use
attribute is set toType Residential
. Pass this in thewhere
parmeter to thequery()
method of the FeatureLayer object. Theas
parameter is set to_df False
so that the results will be returned asFeature
. TheSet return
and_all _records result
parameters are used to only return the first 100 records satisfying the query. Set the_record _cout out
as an array of field names to return in the results._fields Use dark colors for code blocks parcel_layer_item = portal.content.get("a6fdf2ee0e454393a53ba32b9838b303") parcel_layer = parcel_layer_item.layers[0] where_clause = "UseType = 'Residential'" results = parcel_layer.query( where = where_clause, as_df = False, return_all_records = False, result_record_count = 100, out_fields = "APN, UseType" )
Display the results
-
Use the
map
method to create a map widget. Use theadd
method to add theFeature
results to the map contents and theSet zoom
method to set the maps extent so the query results are visible. Import the_to _layer() arcgis.map.popups
module and use thePopup
class to define and enable the popups. The curly braces in the popup content are templates that will use the field's value at run time.Info Use dark colors for code blocks where_clause = "UseType = 'Residential'" results = parcel_layer.query( where = where_clause, as_df = False, return_all_records = False, result_record_count = 100, out_fields = "APN, UseType" ) map = portal.map() from arcgis.map.popups import PopupInfo map.content.add(results, popup_info=PopupInfo(title= "{UseType} Parcel", description = "Parcel number: {APN}")) map map.zoom_to_layer(results)
-
Optional: Use the
export
method to export the current state of the map widget to a static HTML file which can be viewed in any web browser._to _html Use dark colors for code blocks map = portal.map() from arcgis.map.popups import PopupInfo map.content.add(results, popup_info=PopupInfo(title= "{UseType} Parcel", description = "Parcel number: {APN}")) map map.zoom_to_layer(results) import os from os import path, getcwd export_dir = path.join(getcwd(), "home") if not path.isdir(export_dir): os.mkdir(export_dir) export_path = path.join(export_dir, "query-a-feature-layer-sql.html") map.export_to_html(export_path, title="Query a feature layer (SQL))
When the map displays, you should see the results records displayed in the center of the map. Click on a parcel to show a pop-up with the features attributes.
What's next?
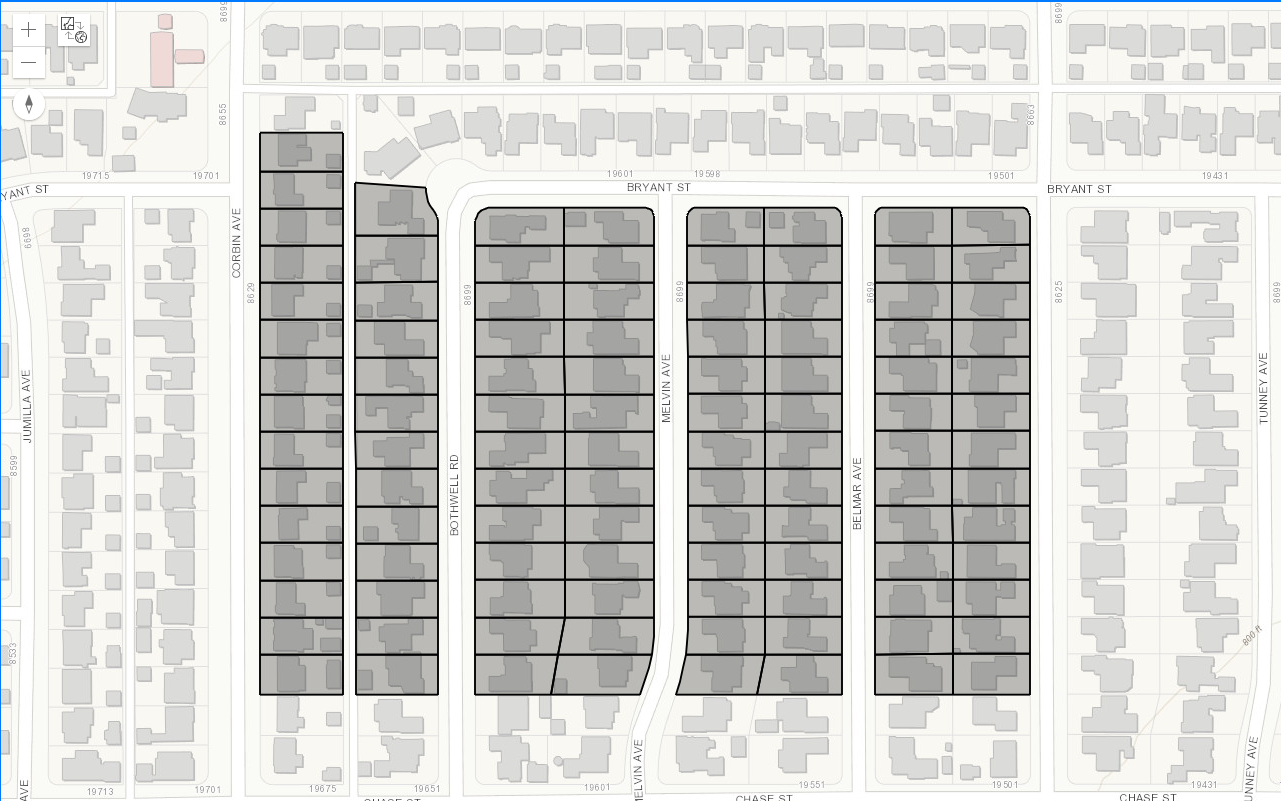
Query a feature layer (spatial)
Learn how to execute a spatial query to access polygon features from feature services.
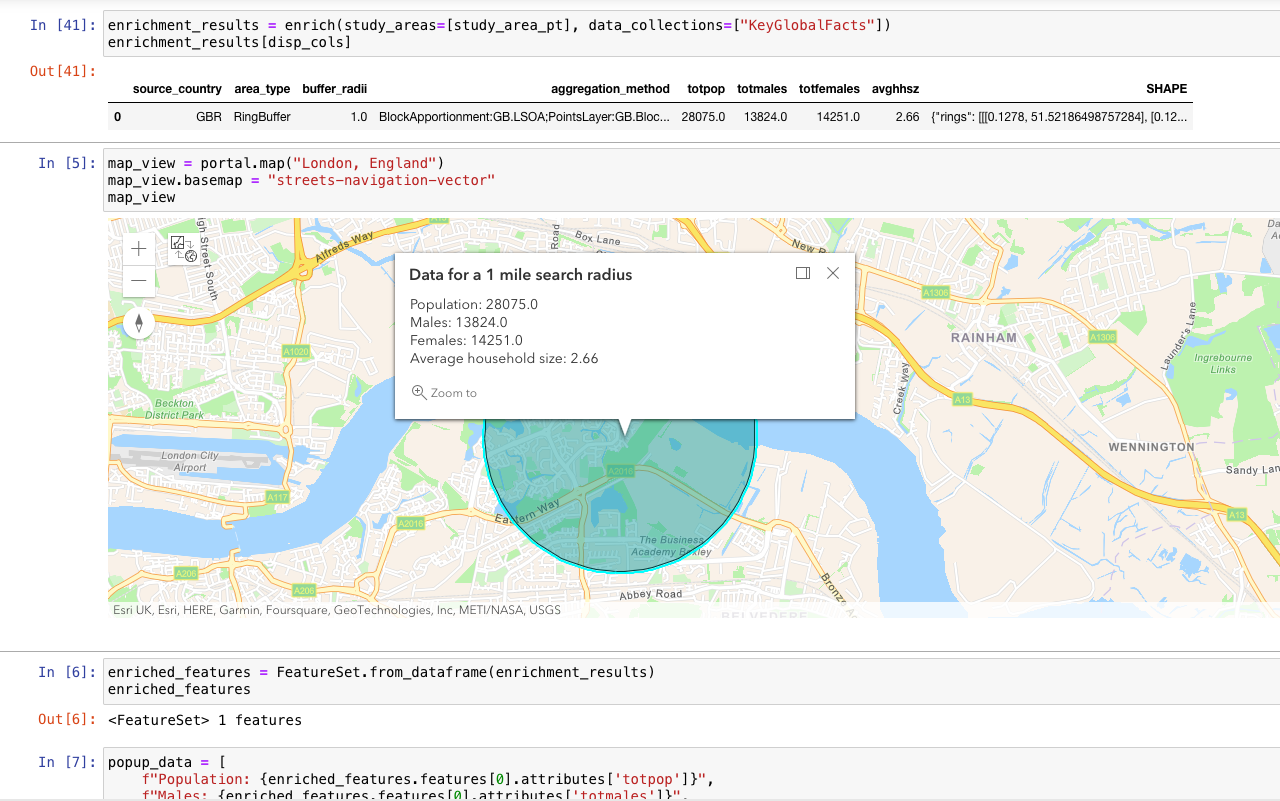
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
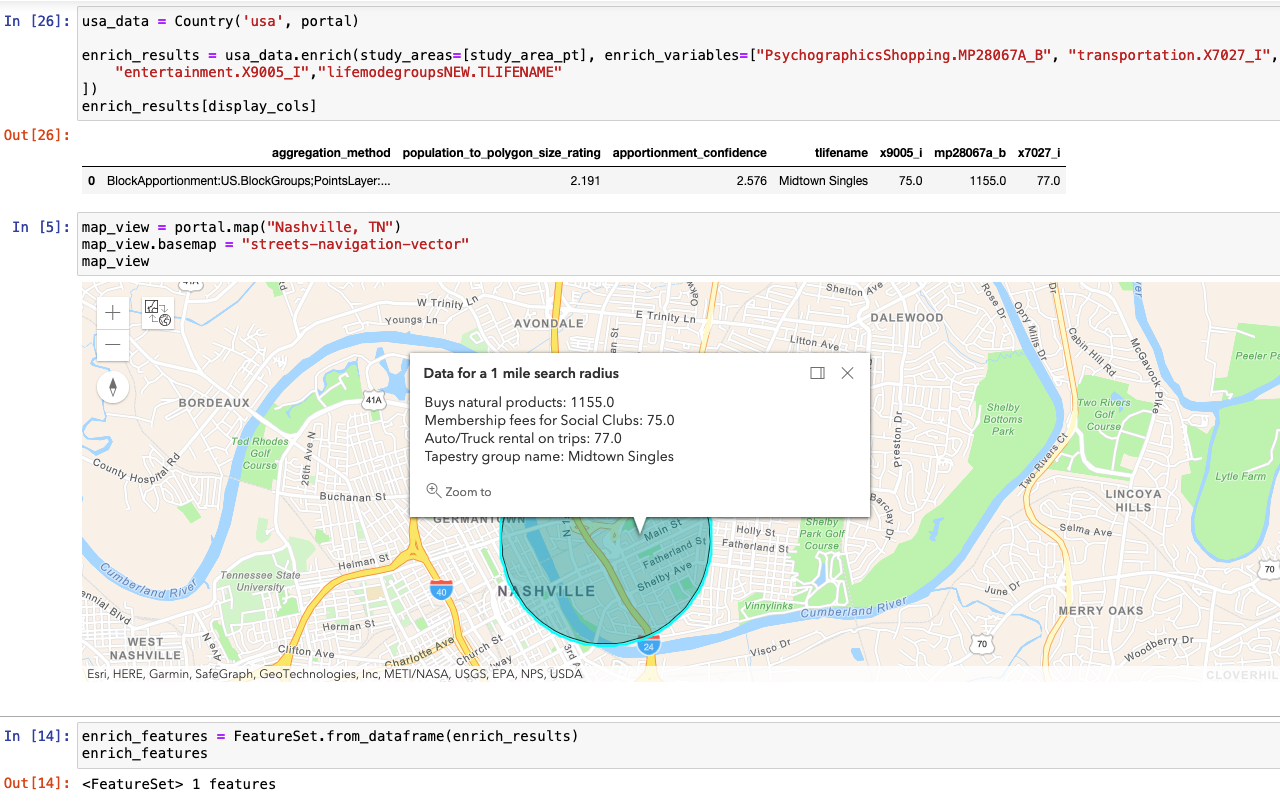
Get local data
Query local analysis variables in select countries around the world with the GeoEnrichment service.
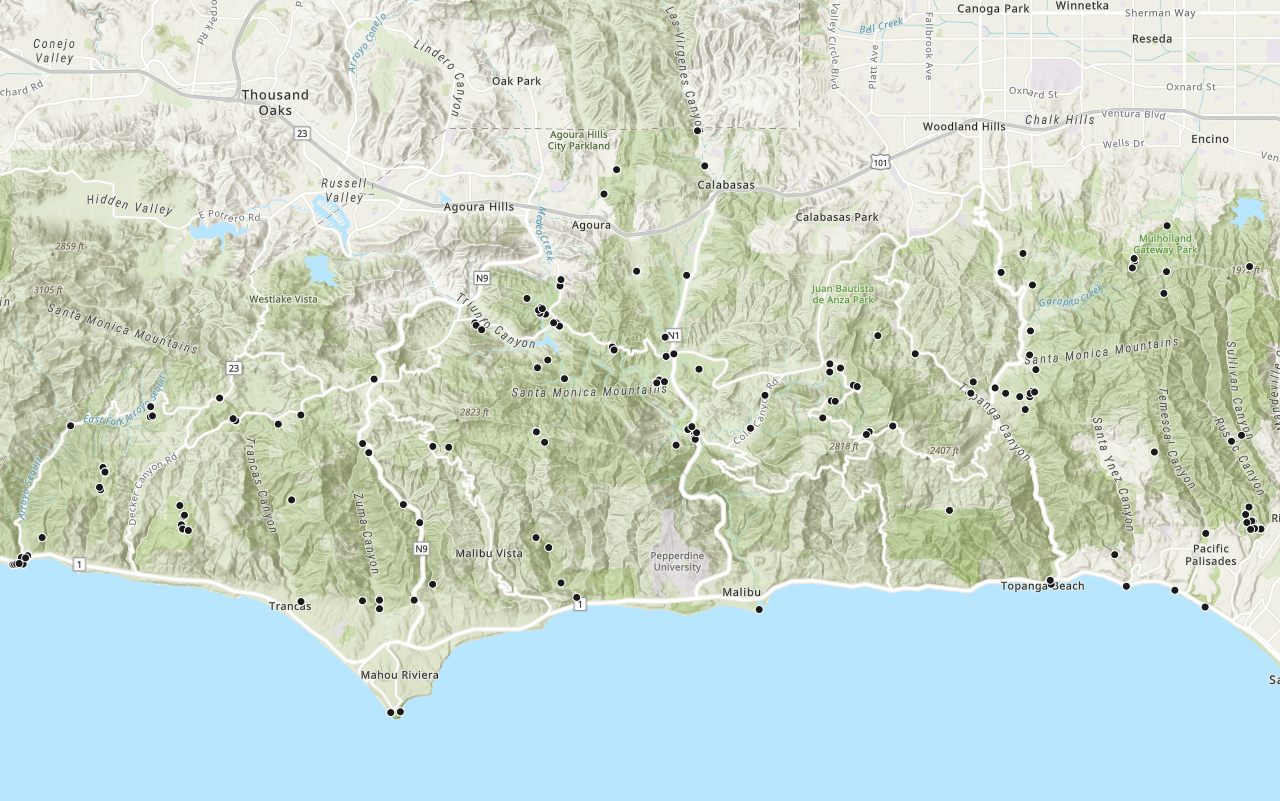
Add a layer from a portal item
Learn how to use a portal item to access and display point features from a feature service.