Learn how to use a URL to access and display a feature layer in a map.
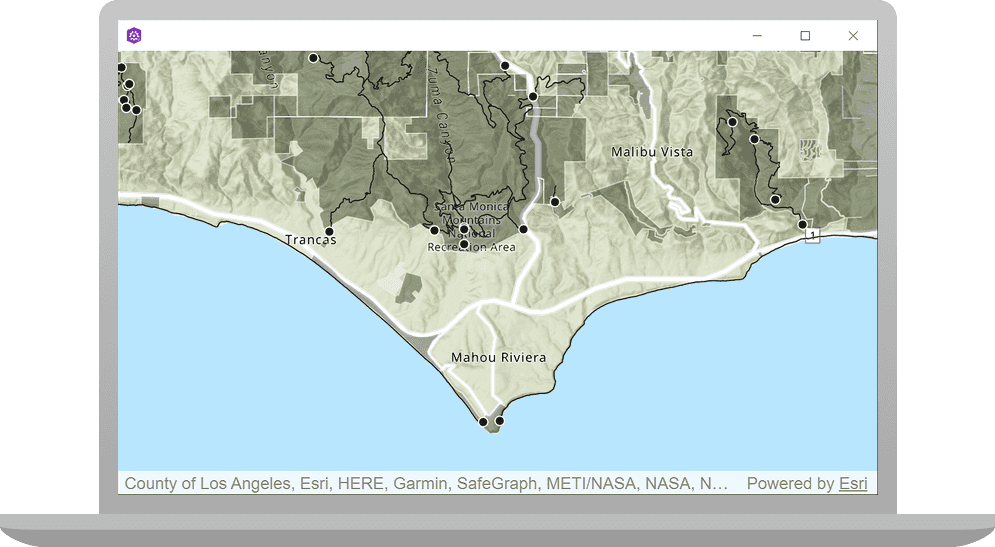
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. This tutorial shows you how to access and display a feature layer in a map. You access feature layers with an item ID or URL. You will use URLs to access the Trailheads, Trails, and Parks and Open Spaces feature layers and display them in a map.
A feature layer is a dataset in a feature service hosted in ArcGIS. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. You can use feature layers to store, access, and manage large amounts of geographic data for your applications.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Runtime API for Qt is installed.
Choose your API
You can do this tutorial in C++ or QML. Make your selection below:
Steps for C++
Open the project in Qt Creator
-
To start this tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the display_a_map project in Qt Creator.
-
If you downloaded the solution, get an access token and set your API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In the Projects window, in the Sources folder, open the main.cpp file.
-
Modify the code to set the API key to the access token. Save and close the file.
main.cppUse dark colors for code blocks 43 44 45 46Change line // 2. API key authentication: Get a long-lived access token that gives your application access to // ArcGIS location services. Go to the tutorial at https://links.esri.com/create-an-api-key. // Copy the API Key access token. const QString accessToken = QString("");
-
Include header files
-
Double click on Sources > Display_a_map.cpp to open the file. Include these header files to be able to create
Service
andFeature Table Feature
instances.Layer Display-a-map.cppUse dark colors for code blocks 15 16 17 18 19 20 21 22Add line. Add line. #include "Display_a_map.h" #include "ArcGISRuntimeEnvironment.h" #include "Basemap.h" #include "Map.h" #include "MapQuickView.h" #include <QUrl> #include "ServiceFeatureTable.h" #include "FeatureLayer.h"
Add a polygon feature layer
This tutorial will draw the map in following order:
- ArcGISTopographic basemap style layer
- Parks and Open Spaces (polygons)
- Trails (lines)
- Trailheads (points)
It is important to add feature layers in the correct order so features are displayed correctly. Polygon feature layers are typically created before lines or points, so that those features are not obscured by the polygons.
Use the Feature
class to reference the Parks and Open Spaces URL and add features to the map.
-
Go to the Parks and Open Spaces URL and browse the properties of the layer. You may want to explore the Name, Type, Drawing Info, and Fields properties.
-
Within the
setup
method, add the following code. Create aViewpoint Q
instance namedUrl polygon
using an ArcGIS feature server as the feature data source. Then create aFeature Url Service
namedFeature Table polygon
from that_feature _table Q
.Url Display-a-map.cppUse dark colors for code blocks 43 44 45 46 47 48Add line. Add line. void Display_a_map::setupViewpoint() { const Point center(-118.80543, 34.02700, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 100000.0); m_mapView->setViewpoint(viewpoint); const QUrl polygonFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Parks_and_Open_Space/FeatureServer/0"); ServiceFeatureTable* polygon_feature_table = new ServiceFeatureTable(polygonFeatureUrl, this);
-
Create a
Feature
instance namedLayer polygon
, passing in_feature _layer polygon
as the argument. Then append your new_feature _table polygon
to the_feature _layer Map
instance namedm
._map Display-a-map.cppUse dark colors for code blocks 49 50 51Add line. Add line. const QUrl polygonFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Parks_and_Open_Space/FeatureServer/0"); ServiceFeatureTable* polygon_feature_table = new ServiceFeatureTable(polygonFeatureUrl, this); FeatureLayer* polygon_feature_layer = new FeatureLayer(polygon_feature_table, this); m_map->operationalLayers()->append(polygon_feature_layer);
Press Ctrl + R to run the app.
You should see a map view centered in the Santa Monica Mountains, with your feature layer showing the Parks and Open Spaces layer in the map.
Add a line feature layer
Line features are typically displayed in a feature layer before points. Use the Feature
class to reference the Trails URL and add features to the map, as described next.
-
Add the following code to create a
Q
instance namedUrl line
using the ArcGIS feature server as the feature data source, in this case,Feature Url Trails
. Then create a_Styled Service
namedFeature Table line
from that_feature _table Q
.Url Display-a-map.cppUse dark colors for code blocks 52 53 54Add line. Add line. FeatureLayer* polygon_feature_layer = new FeatureLayer(polygon_feature_table, this); m_map->operationalLayers()->append(polygon_feature_layer); const QUrl lineFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Trails/FeatureServer/0"); ServiceFeatureTable* line_feature_table = new ServiceFeatureTable(lineFeatureUrl, this);
-
Create a
Feature
instance namedLayer line
, passing in_feature _layer line
as the argument. Then append your new_feature _table line
to the_feature _layer Map
instance namedm
._map Display-a-map.cppUse dark colors for code blocks 55 56 57Add line. Add line. const QUrl lineFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Trails/FeatureServer/0"); ServiceFeatureTable* line_feature_table = new ServiceFeatureTable(lineFeatureUrl, this); FeatureLayer* line_feature_layer = new FeatureLayer(line_feature_table, this); m_map->operationalLayers()->append(line_feature_layer);
Press Ctrl + R to run the app.
The map view should display the Parks and Open Spaces layer (polygons) and the Trails (lines) layer.
Add a point feature layer
-
Add the following code to create a
Q
instance namedUrl point
using the ArcGIS feature server as the feature data source, in this case,Feature Url Trailheads
. Then create aService
namedFeature Table point
from that_feature _table Q
.Url Display-a-map.cppUse dark colors for code blocks 58 59 60Add line. Add line. FeatureLayer* line_feature_layer = new FeatureLayer(line_feature_table, this); m_map->operationalLayers()->append(line_feature_layer); const QUrl pointFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0"); ServiceFeatureTable* point_feature_table= new ServiceFeatureTable(pointFeatureUrl, this);
-
Add the following two lines of code to create a
Feature
, passing inLayer point
as the argument. Then append your_feature _table Feature
to theLayer Map
instance namedm
._map Display-a-map.cppUse dark colors for code blocks 61 62 63Add line. Add line. const QUrl pointFeatureUrl("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0"); ServiceFeatureTable* point_feature_table= new ServiceFeatureTable(pointFeatureUrl, this); FeatureLayer* point_feature_layer = new FeatureLayer(point_feature_table, this); m_map->operationalLayers()->append(point_feature_layer);
Press Ctrl + R to run the app.
The map view should display all three feature layers in the map. Double-click, drag, and scroll the mouse wheel over the map view to explore the map.
To explore other tutorials, see What's next.
Steps for QML
Open the project in Qt Creator
-
To start this tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the display_a_map project in Qt Creator.
-
If you downloaded the solution, get an access token and set your API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In the Projects window, in the Sources folder, open the main.cpp file.
-
Modify the code to set the API key to the access token. Save and close the file.
main.cppUse dark colors for code blocks 43 44 45 46Change line // 2. API key authentication: Get a long-lived access token that gives your application access to // ArcGIS location services. Go to the tutorial at https://links.esri.com/create-an-api-key. // Copy the API Key access token. const QString accessToken = QString("");
-
This tutorial will draw the map in following order:
- ArcGISTopographic basemap style layer
- Parks and Open Spaces (polygons)
- Trails (lines)
- Trailheads (points)
To display each of three new feature layers (also known as operational layers) on top of the current basemap, you will create a Service
using a URL to reference datasets hosted in ArcGIS Online. Each service feature table will become an argument to a Feature
which will be displayed on the map.
Add a polygon feature layer
It is important to add feature layers in the correct order so that features are displayed correctly. Polygon feature layers are typically created before lines or points, so that those features are not obscured by the polygons.
-
Open a web browser and go to the feature service URL for Parks and Open Spaces. The service page provides information such as the geometry type, the geographic extent, the minimum and maximum scale at which features are visible, and the attributes (fields) it contains. You can preview the layer by clicking on ArcGIS.com Map in the View In: list at the top of the page.
-
A
Polygon
geometry represents the shape and location of an area, for example, a country, island, or a lake. A polygon can be used as the geometry of features and graphics, or as input or output of tasks or geoprocessing operations. In this example, our polygons are delivered by a feature service. Use theFeature
class andLayer Service
class to display the Parks and Open Spaces polygons. Open the main.qml file and add the following code where shown.Feature Table main.qmlUse dark colors for code blocks 31 32 33 34 35 36Add line. Add line. Add line. Add line. Add line. Add line. // add a map to the mapview Map { // add the ArcGISTopographic basemap to the map initBasemapStyle: Enums.BasemapStyleArcGISTopographic initialViewpoint: viewpoint // Add the polygon feature layer FeatureLayer { ServiceFeatureTable { url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Parks_and_Open_Space/FeatureServer/0" } }
Press Ctrl + R to run the app.
You should see a map view centered in the Santa Monica Mountains, with your feature layer showing the Parks and Open Spaces layer as gray polygons in the map.
Add a line feature layer
Line features are typically displayed in a feature layer before points.
-
Use the
Feature
class andLayer Service
class to display the Trails with the following code.Feature Table main.qmlUse dark colors for code blocks 37 38 39 40 41 42 43Add line. Add line. Add line. Add line. Add line. Add line. // Add the polygon feature layer FeatureLayer { ServiceFeatureTable { url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Parks_and_Open_Space/FeatureServer/0" } } // Add the line feature layer FeatureLayer { ServiceFeatureTable { url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Trails/FeatureServer/0" } }
Press Ctrl + R to run the app.
The map view should display the Parks and Open Spaces (polygons) feature layer and the Trails (lines) feature layer.
Add a point feature layer
Point
geometries represent discrete locations or entities, such as a geocoded house address, the location of a water meter in a water utility network, a moving vehicle, and so on. In this example, our pre-defined points are being delivered by a feature service.
-
Use the
Feature
class andLayer Service
class to display the Trailheads with the following code.Feature Table main.qmlUse dark colors for code blocks 44 45 46 47 48 49 50Add line. Add line. Add line. Add line. Add line. Add line. // Add the line feature layer FeatureLayer { ServiceFeatureTable { url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Trails/FeatureServer/0" } } // Add the points feature layer FeatureLayer { ServiceFeatureTable { url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0" } }
Press Ctrl + R to run the app.
The map view should display all three feature layers in the map. Double-click, drag, and scroll the mouse wheel over the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials:
Not all tutorials listed have instructions for QML.