Learn how to display a map from a mobile map package (MMPK).
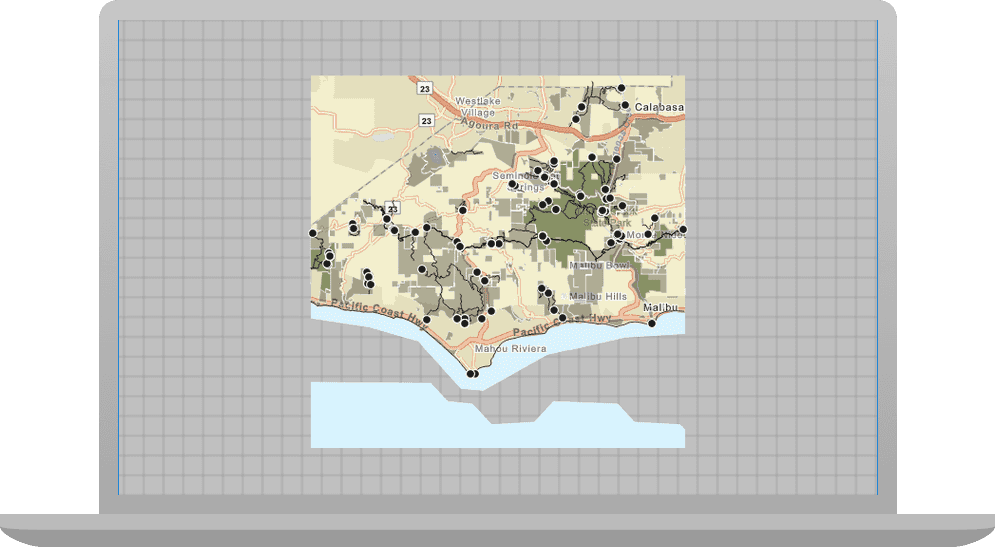
In this tutorial you will display a fully interactive map from a mobile map package (MMPK). The map contains a basemap layer and data layers and does not require a network connection.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Runtime API for Qt is installed.
Steps
Add a mobile map package
-
Download or create the MahouRivieraTrails.mmpk mobile map package. You can download the mobile map package from here: MahouRivieraTrails.mmpk. Otherwise, complete the steps in the Create a mobile map package tutorial, using ArcGIS Pro to create the package.
-
Copy the file MahouRivieraTrails.mmpk to the following local path:
<userhome
/ArcGIS/Runtime/Data/mmpk/, where> <userhome
is your user home location (for example, C:\users\username on Windows, or $HOME on Linux).>
Create a new ArcGIS Runtime Qt Creator Project
-
Launch Qt Creator and create a new project. Under Choose a Template, select Qt Quick C++ app project for the latest version of ArcGIS Runtime installed.
-
Name your project display_an_mmpk.
-
Accept all defaults. At the Define Project Details window, leave the ArcGIS Online Basemap selection as is. Complete the project creation.
Modify the project to use a mobile map package
The project you created from the template includes classes for the
Basemap
,
Map
, and QUrl. Because the mobile map package contains the map, basemap, and all the data layers it requires, in the following steps you will remove the unneeded code from your project and add/modify other code as needed.
-
In Projects, double-click Headers > Display_an_mmpk.h to open the file. Remove the code that forward declares the
Map
class.Display_an_mmpk.hUse dark colors for code blocks 18 19 20 21 22Remove line namespace Esri { namespace ArcGISRuntime { class Map;
-
Remove the code that creates the
Map
pointer variablem
._map This code was generated by the template, but in this tutorial the
Map
class is not needed because it is part of the MMPK.Display_an_mmpk.hUse dark colors for code blocks 44 45 46 47 48 49 50Remove line private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); void setupViewpoint(); Esri::ArcGISRuntime::Map* m_map = nullptr;
-
Under
private
, declare the new function you will implement to load the mobile map package. Then save and close the file.Display_an_mmpk.hUse dark colors for code blocks 40 41 42 43Add line. private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); void setupMapFromMmpk();
-
In Projects, double-click Sources > Display_an_mmpk.cpp to open the file. Remove the lines
#include "
andBasemap.h" #include "
. Also removeMap.h" #include <Q
because it is not needed.Url > This code was generated by the template, but map and basemap are not needed for this tutorial because they are part of the MMPK.
Display_an_mmpk.cppUse dark colors for code blocks 15 16 17 18 21 22 23Remove line Remove line Remove line #include "Display_a_map.h" #include "ArcGISRuntimeEnvironment.h" #include "Basemap.h" #include "Map.h" #include "MapQuickView.h" #include <QUrl>
-
Add
include
statements for theMobileMapPackage
class, the Error class, and the Qt Qdir class.Display_an_mmpk.cppUse dark colors for code blocks 13 14 15 16Add line. Add line. Add line. #include "Display_an_mmpk.h" #include "MapQuickView.h" #include "MobileMapPackage.h" #include "Error.h" #include <QDir>
-
Remove the comma after
Q
and then modify the constructor to remove initialization withObject(parent) Basemap
and theStyle Map
. (Note that theBasemap
configured for your project may be different than that shown here.)Style Display_an_mmpk.cppUse dark colors for code blocks 30 31 33Change line Remove line Display_a_map::Display_a_map(QObject* parent /* = nullptr */): QObject(parent), m_map(new Map(BasemapStyle::ArcGISTopographic, this))
-
Remove the line of code that assigns the
Map
(m
) to_map m
._map View This code was generated by the template, but is not needed because the map is provided by the MMPK.
Display_an_mmpk.cppUse dark colors for code blocks 58 59 60 61 62 63 64 65 66 67Remove line // Set the view (created in QML) void Display_a_map::setMapView(MapQuickView* mapView) { if (!mapView || mapView == m_mapView) { return; } m_mapView = mapView; m_mapView->setMap(m_map);
-
Add a call to a function that reads the map from the mobile map package. You will implement this function in the next step.
Display_an_mmpk.cppUse dark colors for code blocks 37 38 39 40 41 42 43 44 45 46 48 49Add line. // Set the view (created in QML) void Display_an_mmpk::setMapView(MapQuickView* mapView) { if (!mapView || mapView == m_mapView) { return; } m_mapView = mapView; setupMapFromMmpk(); emit mapViewChanged();
-
Add code to implement
setup
. This function defines the path to the MMPK file, instantiates the mobile map package using theMap From Mmpk() MobileMapPackage
constructor, loads the mobile map package, and once loaded, sets the first map in the mobile map package to theMapView
. This also checks that the MMPK file loaded correctly.Display_an_mmpk.cppUse dark colors for code blocks 47 48 49 50 51 52Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. setupMapFromMmpk(); emit mapViewChanged(); } void Display_an_mmpk::setupMapFromMmpk() { // Instantiate a MobileMapPackage object and establish the path to the MMPK file. MobileMapPackage* m_mobileMapPackage = new MobileMapPackage(QDir::homePath() + "/ArcGIS/Runtime/Data/mmpk/MahouRivieraTrails.mmpk", this); // Use connect to signal when the package is done loading so that m_mapView can be set to the first map (0). // Check that the mmpk file has loaded correctly. connect(m_mobileMapPackage, &MobileMapPackage::doneLoading, this, [m_mobileMapPackage, this](Error error) { // Check that the mmpk file has loaded correctly if (!error.isEmpty()) { qDebug() << "Error:" << error.message()<< error.additionalMessage(); return; } // Get the first map in the list of maps, and set the map on the map view to display. // This could be set to any map in the list. m_mapView->setMap(m_mobileMapPackage->maps().at(0)); }); m_mobileMapPackage->load(); }
A
MobileMapPackage
can contain many maps in aMaps
list. Loading the mobile map package is an asynchronous process, and ArcGIS Runtime and Qt take care of reading the file on a thread that will not block the UI. -
Press Ctrl + R to run the app.
You see a map of trailheads, trails, and parks for the area south of the Santa Monica mountains. Drag, zoom in, and zoom out to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: