Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials.
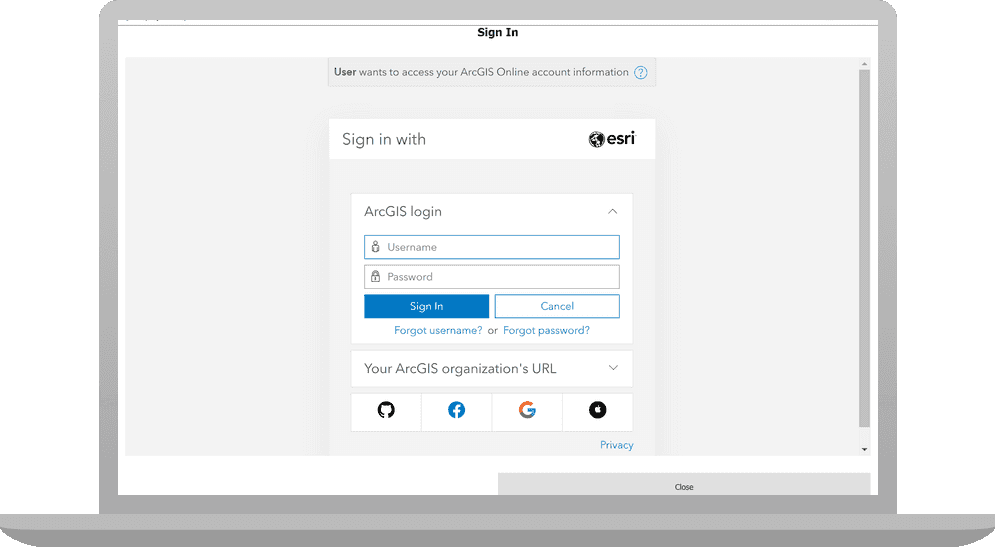
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Runtime API for Qt is installed.
Steps
Download the ArcGIS Runtime Toolkit for Qt
The open-source ArcGIS Runtime Toolkit for Qt contains UI components and utilities to help simplify your Qt app development. For this OAuth tutorial app we will use the toolkit that provides the
AuthenticationView
class, which has a dialog that automatically displays the proper authentication view for any of the supported authentication types (OAuth, Token, HTTP Basic, HTTP Digest, SAML, PKI).
-
To configure the toolkit for use in this tutorial, you need to copy the ArcGIS Runtime Toolkit for Qt repository in GitHub onto your development machine.
-
You can do this by cloning the ArcGIS Runtime Toolkit for Qt repo (using the the URL: https://github.com/Esri/arcgis-runtime-toolkit-qt.git) or downloading the .zip version of the repo and unzipping it to your preferred location on your development machine.
IMPORTANT: Make a particular note for the path to the toolkitcpp.pri
file that is in the directory structure of the toolkit on your development machine. You will need to reference the path to this file later in the tutorial (for example: C
).
Create OAuth credentials for user authentication
OAuth credentials are required to implement user authentication. These credentials are created as an Application item in your organization's portal.
-
Go to the Create OAuth credentials for user authentication tutorial and create OAuth credentials using your ArcGIS Location Platform or ArcGIS Online account.
-
Copy the
Client ID
andRedirect URL
as you will use them to implement user authentication in the next step. TheClient ID
is found on the Application item's Overview page, while theRedirect URL
is found on the Settings page.
The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth sign in. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
IMPORTANT: Make a particular note for the Client ID
string that is generated. You will need to use this string later in the tutorial (for example: XY
).
Open the project in Qt Creator
- To start this tutorial, complete the Display a map tutorial or download and unzip the solution.
- Open the display_a_map project in Qt Creator.
Implement user authentication using OAuth credentials
The OAuth sign in web page is implemented using Qt WebEngine and the
AuthenticationView
class, which is part of the ArcGIS Toolkit.
-
Open
display
and add_a _map.pro webengine
to the list of included modules. WebEngine is a Qt module that can display an OAuth sign in web page in your app.display_a_map.proUse dark colors for code blocks 16 17 18 19 20 21Change line TEMPLATE = app CONFIG += c++14 # additional modules are pulled in via arcgisruntime.pri QT += opengl qml quick webengine
-
To utilize
AuthenticationView
, add the path to where thetoolkitcpp.pri
file is located on your development system (for example:C
). Then save and close:/arcgis-runtime-toolkit-qt/toolkit/uitools/toolkitcpp.pri display
._a _map.pro display_a_map.proUse dark colors for code blocks 35 36 37 38 39 40 41Add line. Add line. equals(QT_MAJOR_VERSION, 6) { error("This version of the ArcGIS Runtime SDK for Qt is incompatible with Qt 6") } ARCGIS_RUNTIME_VERSION = 100.15.0 include($$PWD/arcgisruntime.pri) # Set the path to the toolkit, absolute or relative to this app. include(<path_to_toolkit_repo>/toolkit/uitools/toolkitcpp.pri) # example: C:/arcgis-runtime-toolkit-qt/toolkit/uitools/toolkitcpp.pri
-
In the project Sources folder, open main.cpp. Remove these
#include
statements. These Qt types are not needed for this tutorial.main.cppUse dark colors for code blocks 17 18 19Remove line Remove line Remove line #include "ArcGISRuntimeEnvironment.h" #include "MapQuickView.h" #include <QDir> #include <QGuiApplication> #include <QQmlApplicationEngine>
-
Include
Qt
, andWeb Engine register.h
for the ArcGIS Runtime Toolkit.main.cppUse dark colors for code blocks 17 18 19Add line. Add line. #include "ArcGISRuntimeEnvironment.h" #include "MapQuickView.h" #include <QtWebEngine> #include "Esri/ArcGISRuntime/Toolkit/register.h"
-
Add code to initialize
Qt
.Web Engine main.cppUse dark colors for code blocks 28 29 30 31 32Add line. int main(int argc, char *argv[]) { QGuiApplication::setAttribute(Qt::AA_EnableHighDpiScaling); QGuiApplication app(argc, argv); QtWebEngine::initialize();
-
Register the ArcGIS Runtime Toolkit. Then save and close main.cpp.
main.cppUse dark colors for code blocks 70 71 72 73 74 75Add line. Add line. // Register the Display_a_map (QQuickItem) for QML qmlRegisterType<Display_a_map>("Esri.display_a_map", 1, 0, "Display_a_map"); // Initialize application view QQmlApplicationEngine engine; // Register the Toolkit Esri::ArcGISRuntime::Toolkit::registerComponents(engine);
-
Navigate to Resources > qml\qml.qrc > qml and open Display_a_mapForm.qml. Import the ArcGIS Runtime Toolkit. Be sure set the version to your installed ArcGIS Runtime version.
Display_a_mapForm.qmlUse dark colors for code blocks 15 16 17 18Add line. import QtQuick 2.6 import QtQuick.Controls 2.2 import Esri.display_a_map 1.0 import Esri.ArcGISRuntime.Toolkit 100.13
-
Now that the toolkit is available, declare an
AuthenticationView
component. Then save and close Display_a_mapForm.qml.AuthenticationView
is an ArcGIS Runtime Toolkit component that simplifies the authentication workflow by usingAuthenticationManager
to automatically display the correct login user interface for each security method (Token, OAuth, PKI, and so on). For more information, seeAuthenticationView
.Display_a_mapForm.qmlUse dark colors for code blocks 31 32 33 34 35 36Add line. Add line. Add line. Add line. Add line. // Declare the C++ instance that creates the map etc., and supply the view. Display_a_map { id: model mapView: view } // Declare an AuthenticationView to support login. AuthenticationView { id: authView anchors.fill: parent }
Add required class header files
Several additional classes are required to support the functionality your app needs. Specifically, for managing OAuth authentication, creating a credential, creating an image layer, and accessing the secured traffic layer portal item.
-
In the project Sources folder, open Display_a_map.cpp. Include the six required header files as shown.
Display_a_map.cppUse dark colors for code blocks 19 20 21 22 23 24Add line. Add line. Add line. Add line. Add line. #include "Basemap.h" #include "Map.h" #include "MapQuickView.h" #include <QUrl> #include "Credential.h" #include "OAuthClientInfo.h" #include "Portal.h" #include "PortalItem.h" #include "ArcGISMapImageLayer.h"
Create a credential and portal to access secured services
You can use an instance of the
Portal
class to access secure services on your portal. In the constructor, you can either provide the portal URL or leave it out to use ArcGIS Online by default. You can also provide a
Credential
in the constructor to use when accessing secured services.
-
In the
setup
function, add the following code to createViewpoint Credential
. Paste your Client ID (created earlier with Create OAuth credentials) to replace the "CLIENT_ID" placeholder. Also be sure thatOAuthMode
is set toUser
.Display_a_map.cppUse dark colors for code blocks 49 50 51 52 53 54 55Add line. Add line. void Display_a_map::setupViewpoint() { const Point center(-118.80543, 34.02700, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 100000.0); m_mapView->setViewpoint(viewpoint); // Create a credential for this app and set the authentication mode. Credential* credential = new Credential(OAuthClientInfo("CLIENT_ID", OAuthMode::User), this); // Change the CLIENT_ID to your string, example "XYZ123"
-
Create a
Portal
that will usecredential
to gain access to the secured ArcGIS World Traffice service.Display_a_map.cppUse dark colors for code blocks 52 53 54 55 56 57 58 59Add line. const Point center(-118.80543, 34.02700, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 100000.0); m_mapView->setViewpoint(viewpoint); // Create a credential for this app and set the authentication mode. Credential* credential = new Credential(OAuthClientInfo("CLIENT_ID", OAuthMode::User), this); // Change the CLIENT_ID to your string, example "XYZ123" Portal* portal = new Portal(credential, this);
Add the secured traffic layer
The ArcGIS World Traffic service is a dynamic map service that presents historical and near real-time traffic information for different regions of the world. This service requires an ArcGIS Online organizational subscription. You will add a portal item referencing this service and use the item to create a traffic layer.
ArcGIS World Traffic service data is updated every five minutes to provide traffic speed and traffic incident visualization and identification. Traffic speeds are displayed as a percentage of free-flow speeds, which is frequently the speed limit or how fast cars tend to travel when unencumbered by other vehicles. The streets are color coded as follows:
- Green (fast): 85 - 100% of free flow speeds
- Yellow (moderate): 65 - 85%
- Orange (slow); 45 - 65%
- Red (stop and go): 0 - 45%
-
Create a
PortalItem
using the traffic service's item ID. Then create anArcGISMapImageLayer
to display the traffic service. Finally, append the traffic layer to the map's collection of data layers (operational layers)Display_a_map.cppUse dark colors for code blocks 56 57 58 59 60 61 62Add line. Add line. Add line. Add line. Add line. Add line. // Create a credential for this app and set the authentication mode. Credential* credential = new Credential(OAuthClientInfo("CLIENT_ID", OAuthMode::User), this); // Change the CLIENT_ID to your string, example "XYZ123" Portal* portal = new Portal(credential, this); // Create a layer to display the ArcGIS World Traffic service. // traffic layer https://www.arcgis.com/home/item.html?id=ff11eb5b930b4fabba15c47feb130de4 PortalItem* item = new PortalItem(portal, "ff11eb5b930b4fabba15c47feb130de4", this); ArcGISMapImageLayer* imageLayer = new ArcGISMapImageLayer(item, this); // Append the traffic layer to the map's data layer collection. m_map->operationalLayers()->append(imageLayer);
-
Press Ctrl + R to run the app.
You should briefly see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. The AuthenticationView will then prompt you with an OAuth sign in page to enter your ArcGIS Online username and password. After authenticating successfully with ArcGIS Online, the map will appear with the traffic layer also displayed.
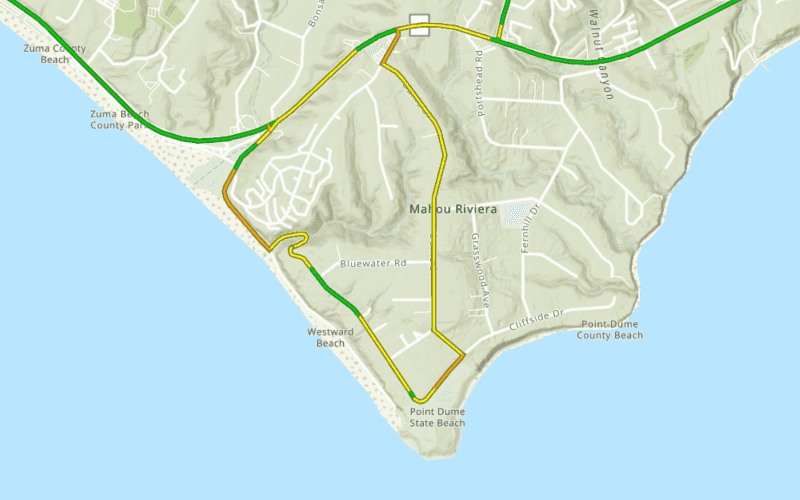
Other tutorials
Some of the following also include instructions for QML.