Associations enable the modeling of connectivity, containment, and structure attachment between non-spatial and non-coincident network features. The following table describes the types of relationships between two utility network elements:
Association | Description | Geometry supported |
---|---|---|
Connectivity | Models the connectivity between two junctions that don't have geometric coincidence (are not in the same x, y and z location). A transformer may be connected to a fuse, for example. | Yes |
Structural attachment | Models equipment attached to structures. A transformer bank may be attached to a pole, for example. | Yes |
Containment | Models assets that contain other assets. A vault may contain valves and pipes, for example. | No |
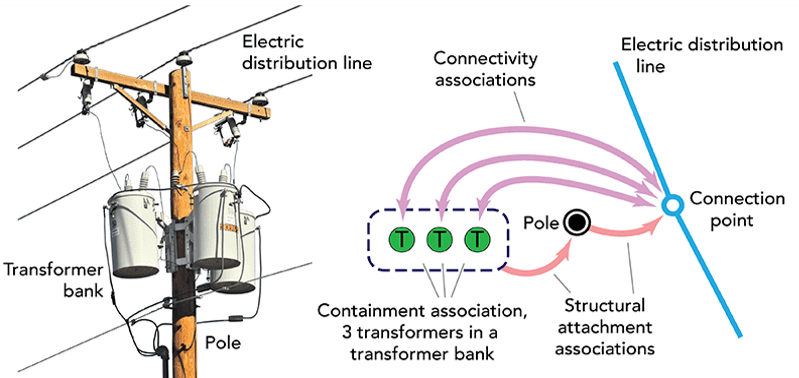
// Get the elements associated with a single utility element (using containment).
do {
let associations = try await utilityNetwork.associations(for: utilityElement, ofKind: .containment)
for association in associations {
let fromElement = association.fromElement
let toElement = association.toElement
}
} catch {
print("Error getting associations.")
}
You can also provide an envelope to find all valid associations within the specified extent.
The following shows a graphics overlay to display all associations within the map extent using a unique symbol for each association type.
// Create a graphics overlay for associations.
let associationsOverlay = GraphicsOverlay()
// Create symbols for the attachment and connectivity associations.
let attachmentSymbol = SimpleLineSymbol(style: .dot, color: .green, width: 5)
let connectivitySymbol = SimpleLineSymbol(style: .dot, color: .red, width: 5)
// Create a unique value renderer for the associations and apply it to the graphics overlay.
let attachmentValue = UniqueValue(description: "Attachment", symbol: attachmentSymbol, values: [UtilityAssociation.Kind.attachment])
let connectivityValue = UniqueValue(description: "Connectivity", symbol: connectivitySymbol, values: [UtilityAssociation.Kind.connectivity])
let renderer = UniqueValueRenderer(fieldNames: ["AssociationType"], uniqueValues: [attachmentValue, connectivityValue])
associationsOverlay.renderer = renderer
let currentExtent = currentViewpoint.targetGeometry.extent
do {
// Get all of the associations for the current extent.
let associations = try await utilityNetwork.associations(forExtent: currentExtent)
for association in associations where association.kind != .containment {
let graphic = Graphic()
graphic.setAttributeValue(association.globalID, forKey: "GlobalId")
graphic.setAttributeValue(association.globalID, forKey: "AssociationType")
associationsOverlay.addGraphic(graphic)
}
} catch {
print("Error getting associations.")
}
Specifying a utility element will return all its associations unless the utility element's terminal has been set, which limits the connectivity associations returned.
Specifying an extent will return all its connectivity or structural attachment associations with geometry. The geometry value (polyline) represents the connection relationship between a from
element and a to
element. You can use the geometry to visualize the association as a graphic in the map.