Learn how to display point, line, and polygon graphics in a map.
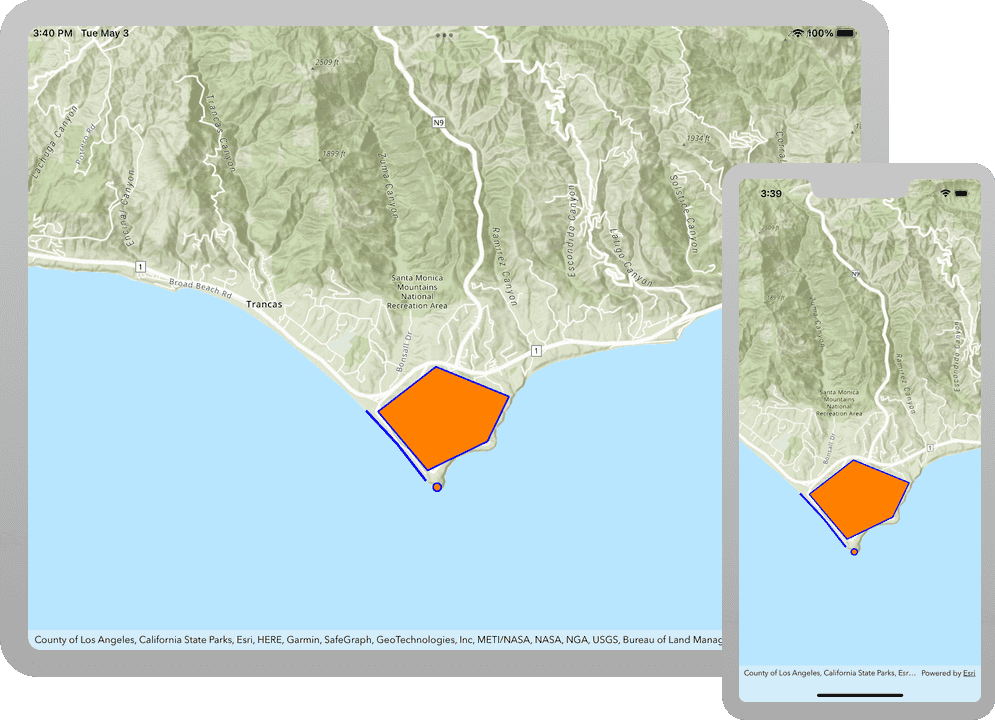
You typically use graphics to display geographic data that is not connected to a database and that is not persisted, like highlighting a route between two locations, displaying a search buffer around a point, or tracking the location of a vehicle in real-time. Graphics are composed of a geometry, symbol, and attributes.
In this tutorial, you display points, lines, and polygons on a map as graphics.
To learn how to display data from data sources, see the Add a feature layer tutorial.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
Steps
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Xcode, in the Project Navigator, click AppDelegate.swift.
-
In the editor, set the
API
property on theKey AGS
with your access token.ArcGIS Runtime Environment AppDelegate.swiftUse dark colors for code blocks func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { AGSArcGISRuntimeEnvironment.apiKey = "YOUR_ACCESS_TOKEN" return true }
-
Add a graphics overlay
A graphics overlay is a container for graphics. It is used with a map view to display graphics on a map. You can add more than one graphics overlay to a map view. Graphics overlays are displayed on top of all the other layers.
-
In Xcode, in the Project navigator, click ViewController.swift.
-
Create a new method named
add
. Create anGraphics AGS
to display point, line, and polygon graphics, and add it to theGraphics Overlay map
's collection of graphics overlays. Call theView add
method from theGraphics() View
'sController view
method.Did Load() ViewController.swiftUse dark colors for code blocks 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 46 47 48 49 50 51 53 54 55 56Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. import UIKit import ArcGIS class ViewController: UIViewController { @IBOutlet weak var mapView: AGSMapView! private func setupMap() { let map = AGSMap( basemapStyle: .arcGISTopographic ) mapView.map = map mapView.setViewpoint( AGSViewpoint( latitude: 34.02700, longitude: -118.80500, scale: 72_000 ) ) } private func addGraphics() { let graphicsOverlay = AGSGraphicsOverlay() mapView.graphicsOverlays.add(graphicsOverlay) } override func viewDidLoad() { super.viewDidLoad() setupMap() addGraphics() } }
Add a point graphic
A point graphic is created using a point and a marker symbol. A point is defined with x and y coordinates, and a spatial reference. For latitude and longitude coordinates, the spatial reference is WGS84.
-
Create an
AGS
and anPoint AGS
. To create theSimple Marker Symbol AGS
, provide longitude (x) and latitude (y) coordinates, and anPoint AGS
. Use theSpatial Reference AGS
convenience method.Spatial Reference.wgs84() Point graphics support a number of symbol types such as
AGS
,Simple Marker Symbol Picture
andMarker Symbol AGS
. Learn more about symbols in the API documentation.Text Symbol Use dark colors for code blocks 37 38 39 40 41 42 48 49 50Add line. Add line. Add line. Add line. Add line. private func addGraphics() { let graphicsOverlay = AGSGraphicsOverlay() mapView.graphicsOverlays.add(graphicsOverlay) let point = AGSPoint(x: -118.80657463861, y: 34.0005930608889, spatialReference: .wgs84()) let pointSymbol = AGSSimpleMarkerSymbol(style: .circle, color: .orange, size: 10.0) pointSymbol.outline = AGSSimpleLineSymbol(style: .solid, color: .blue, width: 2.0) }
You can also create
AGS
s with latitude and longitude using thePoint AGS
convenience function. Notice that the order of the coordinates passed into this function is y,x (latitude,longitude):Point Make WG S84() Use dark colors for code blocks Copy AGSPointMakeWGS84(34.0005930608889, -118.80657463861)
-
Create an
AGS
with theGraphic point
andpoint
. Display theSymbol AGS
by adding it to theGraphic graphics
'sOverlay graphics
collection.Use dark colors for code blocks 46 47Add line. Add line. Add line. pointSymbol.outline = AGSSimpleLineSymbol(style: .solid, color: .blue, width: 2.0) let pointGraphic = AGSGraphic(geometry: point, symbol: pointSymbol) graphicsOverlay.graphics.add(pointGraphic)
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
You should see a point graphic in Point Dume State Beach.
Add a line graphic
A line graphic is created using a polyline and a line symbol. A polyline is defined as a sequence of points.
Polylines have one or more distinct parts. Each part is a sequence of points. For a continuous line, you can use the AGS
constructor to create a polyline with just one part. To create a polyline with more than one part, use an AGS
.
-
Create an
AGS
and anPolyline AGS
. To create theSimple Line Symbol AGS
, provide an array ofPolyline AGS
s.Point Line graphics support a number of symbol types such as
AGS
andSimple Line Symbol AGS
. Learn more about symbols in the API documentation.Text Symbol Use dark colors for code blocks 50 51 52Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. graphicsOverlay.graphics.add(pointGraphic) let polyline = AGSPolyline( points: [ AGSPoint(x: -118.821527826096, y: 34.0139576938577, spatialReference: .wgs84()), AGSPoint(x: -118.814893761649, y: 34.0080602407843, spatialReference: .wgs84()), AGSPoint(x: -118.808878330345, y: 34.0016642996246, spatialReference: .wgs84()) ] ) let polylineSymbol = AGSSimpleLineSymbol(style: .solid, color: .blue, width: 3.0)
-
Create an
AGS
with theGraphic polyline
andpolyline
. Display theSymbol AGS
by adding it to theGraphic graphics
'sOverlay graphics
collection.Use dark colors for code blocks 61 62Add line. Add line. Add line. let polylineSymbol = AGSSimpleLineSymbol(style: .solid, color: .blue, width: 3.0) let polylineGraphic = AGSGraphic(geometry: polyline, symbol: polylineSymbol) graphicsOverlay.graphics.add(polylineGraphic)
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
You should see a point and line graphic along Westward Beach.
Add a polygon graphic
A polygon graphic is created using a polygon and a fill symbol. A polygon is defined as a sequence of points that describe a closed boundary.
Polygons have one or more distinct parts. Each part is a sequence of points describing a closed boundary. For a single area with no holes, you can use the AGS
constructor to create a polygon with just one part. To create a polygon with more than one part, use an AGS
.
-
Create an
AGS
and anPolygon AGS
. To create theSimple Fill Symbol AGS
, provide an array ofPolygon AGS
s.Point Polygon graphics support a number of symbol types such as
AGS
,Simple Fill Symbol AGS
,Picture Fill Symbol AGS
andSimple Marker Symbol AGS
. Learn more about symbols in the API documentation.Text Symbol Use dark colors for code blocks 65 66 67Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. graphicsOverlay.graphics.add(polylineGraphic) let polygon = AGSPolygon( points: [ AGSPoint(x: -118.818984489994, y: 34.0137559967283, spatialReference: .wgs84()), AGSPoint(x: -118.806796597377, y: 34.0215816298725, spatialReference: .wgs84()), AGSPoint(x: -118.791432890735, y: 34.0163883241613, spatialReference: .wgs84()), AGSPoint(x: -118.79596686535, y: 34.008564864635, spatialReference: .wgs84()), AGSPoint(x: -118.808558110679, y: 34.0035027131376, spatialReference: .wgs84()) ] ) let polygonSymbol = AGSSimpleFillSymbol(style: .solid, color: .orange, outline: AGSSimpleLineSymbol(style: .solid, color: .blue, width: 2.0))
-
Create an
AGS
with theGraphic polygon
andpolygon
. Display theSymbol AGS
by adding it to theGraphic graphics
'sOverlay graphics
collection.Use dark colors for code blocks 78 79Add line. Add line. Add line. let polygonSymbol = AGSSimpleFillSymbol(style: .solid, color: .orange, outline: AGSSimpleLineSymbol(style: .solid, color: .blue, width: 2.0)) let polygonGraphic = AGSGraphic(geometry: polygon, symbol: polygonSymbol) graphicsOverlay.graphics.add(polygonGraphic)
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
You should see a point, line, and polygon graphic around Mahou Riviera in the Santa Monica Mountains.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: