Autocasting
Autocasting casts JavaScript objects as ArcGIS Maps SDK for JavaScript class types without the need for these classes to be explicitly imported by the app developer.
In the following code sample, five API classes are needed to create a SimpleRenderer for a FeatureLayer.
require([
"esri/Color",
"esri/symbols/SimpleLineSymbol",
"esri/symbols/SimpleMarkerSymbol",
"esri/renderers/SimpleRenderer",
"esri/layers/FeatureLayer",
], (
Color, SimpleLineSymbol, SimpleMarkerSymbol, SimpleRenderer, FeatureLayer
) => {
const layer = new FeatureLayer({
url: "https://services.arcgis.com/V6ZHFr6zdgNZuVG0/arcgis/rest/services/WorldCities/FeatureServer/0",
renderer: new SimpleRenderer({
symbol: new SimpleMarkerSymbol({
style: "diamond",
color: new Color([255, 128, 45]),
outline: new SimpleLineSymbol({
style: "dash-dot",
color: new Color([0, 0, 0])
})
})
})
});
});
With autocasting, you don't have to import renderer and symbol classes; the only module you need to import is esri/layers/
.
require([ "esri/layers/FeatureLayer" ], (FeatureLayer) => {
const layer = new FeatureLayer({
url: "https://services.arcgis.com/V6ZHFr6zdgNZuVG0/arcgis/rest/services/WorldCities/FeatureServer/0",
renderer: { // autocasts as new SimpleRenderer()
symbol: { // autocasts as new SimpleMarkerSymbol()
type: "simple-marker",
style: "diamond",
color: [ 255, 128, 45 ], // autocasts as new Color()
outline: { // autocasts as new SimpleLineSymbol()
style: "dash-dot",
color: [ 0, 0, 0 ] // autocasts as new Color()
}
}
}
});
});
To know whether a class can be autocasted, look at the ArcGIS Maps SDK for JavaScript reference for each class. If a property can be autocasted, the following image will appear:
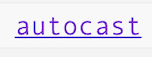
For example, the documentation for the property renderer
of the Feature
class has an autocast
tag.
Notice the code using autocasting is simpler and is functionally identical to the above code snippet where all the modules are explicitly imported. The ArcGIS Maps SDK for JavaScript will take the values passed to the properties in the constructor and instantiate the typed objects internally.
Keep in mind there is no need to specify the type
on properties where the module type is known, or fixed. For example, look at the outline
property in the SimpleMarkerSymbol class from the snippet above. It doesn't have a type
property because the only Symbol
subclass with an outline
property is Simple
.
const diamondSymbol = {
type: "simple-marker",
outline: {
type: "simple-line", // Not needed, as type `simple-line` is implied
style: "dash-dot",
color: [ 255, 128, 45 ]
}
};
In cases where the type
is more generic, such as FeatureLayer.renderer, then type
must always be specified for autocasting to work properly.