By default, the arcgis-layer-list component allows users to toggle layer visibility on and off in the map. List items represent a layer in the component. You can augment the layer list to allow users to perform custom actions for each layer by adding action buttons or toggles to each list item or add additional content to a list item's panel.
In this sample, we add custom actions to zoom to the layer's full extent, view the layer's REST endpoint, and modify the opacity of individual layers. We also add a slider to adjust the layer's opacity in the list item's panel. The actions and panel content are created by defining a listItemCreatedFunction property on the layer list. This function runs when each list item is created and returns an event object with the ListItem for that individual layer.
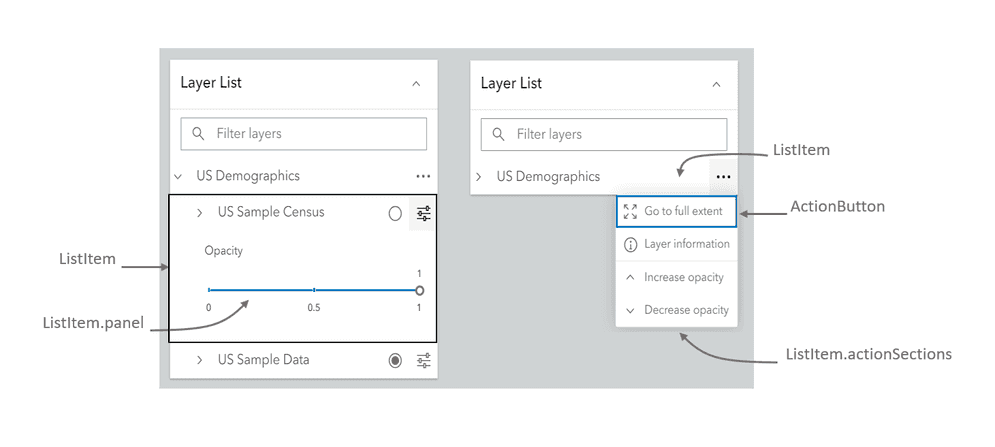
Add a layer list to the top right corner of the map. Set attributes to show the heading, collapse button and the filter input.
<arcgis-layer-list
position="top-right"
show-collapse-button
show-heading
show-filter
filter-placeholder="Filter layers"
></arcgis-layer-list>
Define the actions for each ListItem in the listItemCreatedFunction function. This function is called for each ListItem when it's created for a layer.
// Get a reference to the arcgis-layer-list component.
const arcgisLayerList = document.querySelector("arcgis-layer-list");
// A function that executes each time a ListItem is created for a layer.
arcgisLayerList.listItemCreatedFunction = (event) => {
// The event object contains an item property.
// It is a ListItem referencing the associated layer
// and other properties. You can control the visibility of the
// item, its title, and actions using this object.
const { item } = event;
// If the title of the item is "US Demographics"
if (item.title === "US Demographics") {
// A collection of ActionButtons to place in the LayerList.
// By making this collection two-dimensional, you can separate similar
// actions into separate groups with a breaking line.
item.actionsSections = new Collection([
new Collection([
new ActionButton({
title: "Go to full extent",
icon: "zoom-out-fixed",
id: "full-extent"
}),
new ActionButton({
title: "Layer information",
icon: "information",
id: "information"
})
]),
new Collection([
new ActionButton({
title: "Increase opacity",
icon: "chevron-up",
id: "increase-opacity"
}),
new ActionButton({
title: "Decrease opacity",
icon: "chevron-down",
id: "decrease-opacity"
})
])
]);
}
};
Use the arcgisTriggerAction event to define the behavior of each action returned by the function.
// Event listener that fires each time an action is triggered
arcgisLayerList.addEventListener("arcgisTriggerAction", (event) => {
// The layer visible in the view at the time of the trigger.
const visibleLayer = USALayer.visible ? USALayer : censusLayer;
// Get a reference to the action id.
const { id } = event.detail.action;
// If the full-extent action is triggered then navigate
// to the full extent of the visible layer
if (id === "full-extent") {
arcgisMap?.goTo(visibleLayer.fullExtent).catch((error) => {
if (error.name != "AbortError") {
console.error(error);
}
});
}
// If the information action is triggered, then
// open the item details page of the service layer
if (id === "information") {
window.open(visibleLayer.url);
}
// If the increase-opacity action is triggered, then
// increase the opacity of the GroupLayer by 0.25
if (id === "increase-opacity") {
if (demographicGroupLayer.opacity < 1) {
demographicGroupLayer.opacity += 0.25;
}
}
// If the decrease-opacity action is triggered, then
// decrease the opacity of the GroupLayer by 0.25
if (id === "decrease-opacity") {
if (demographicGroupLayer.opacity > 0) {
demographicGroupLayer.opacity -= 0.25;
}
}
});
You can also specify custom content in the ListItem's panel. This can be any text, HTML node, or widget. For example, this app adds sliders to the panels of the two map image layers. Each slider updates the opacity of the respective layer.
// Add a calcite slider for updating opacity on group layers.
if (item.layer.type === "map-image") {
const label = document.createElement("calcite-label");
label.innerText = "Opacity";
label.scale = "s";
const slider = document.createElement("calcite-slider");
slider.labelHandles = true;
slider.labelTicks = true;
slider.min = 0;
slider.minLabel = "0";
slider.max = 1;
slider.maxLabel = "1";
slider.scale = "s";
slider.step = 0.01;
slider.value = 1;
slider.ticks = 0.5;
slider.addEventListener("calciteSliderChange", () => {
item.layer.opacity = slider.value;
});
label.appendChild(slider);
item.panel = {
content: label,
icon: "sliders-horizontal",
title: "Change layer opacity"
};
}