This sample shows how to use the visibleArea property in a 3D SceneView. The visible
is
the visible portion of a map within the view as an instance of a Polygon, as shown in the top-right secondary Scene
.
This Polygon
is thus a 2D approximation of the 3D frustum projected onto the ground surface. The sample covers two scenarios:
Query a FeatureLayerView within the visibleArea
The following code snippet demonstrates how to use the visible
geometry to query a FeatureLayerView and retrieve features within the current Scene
using the queryFeatures method.
// Query the treeLayerView to get all the trees inside the visibleArea
const featureSet = await treeLayerView.queryFeatures({
geometry: view.visibleArea,
returnGeometry: false,
outFields: ["commonname"]
});
This ensures that only features currently in the visible
are considered and the results of the Feature
query
are further processed
to count the number of trees belonging to different categories within the visible area. This information is then used to update indicators at the bottom
of the application, providing real-time feedback about the distribution of tree types in their current view
.
Visualize the visibleArea
The sample provides also a visual representation of the visible
polygon by utilizing a secondary Scene
.
In fact, it renders the visible
polygon on the ground, offering a clear visual indication of the area currently visible to the user.
Additionally, when the 3D mode is toggled,the mini-view shows the lines connecting the camera
position to the vertices of the visible
polygon, effectively outlining the 3D frustum.
let { visibleArea } = view;
const visibleAreaGraphics = getVisibleAreaGraphics(visibleArea);
visibleAreaGraphicsLayer.addMany(visibleAreaGraphics);
// Create the Polygon3D Symbol for the visibleArea polygon
const visibleAreaSymbol = {
type: "polygon-3d",
symbolLayers: [
{
type: "fill",
material: { color: "white" },
outline: { color: "white" },
pattern: {
type: "style",
style: "forward-diagonal"
}
}
]
};
// Create the graphics for the visibleArea vertices
function getVisibleAreaGraphics(visibleArea) {
const parts = visibleArea.rings.map(
(ring) => new Polygon({ rings: [ring], spatialReference: visibleArea.spatialReference })
);
return parts.map((part) => new Graphic({ geometry: part, symbol: visibleAreaSymbol }));
}
Similar to the feature query above-mentioned, in response to changes in the main view's visible
, we use reactiveUtils when
to dynamically update the visualization: we recreate the frustum with the new visible
polygon in the supportView and we also update the trees indicators at the bottom.
// Every time the visibleArea of the view change, we update both the supportView and the trees indicators
reactiveUtils.when(
() => view.visibleArea,
() => {
// Debounce the queries to the tree featureLayerView to avoid multiple requests
debounceQueryTrees().catch((error) => {
if (error.name === "AbortError") {
return;
}
console.error(error);
});
// Update the supportView
updateSceneView();
},
{
initial: true
}
);
Related samples and resources
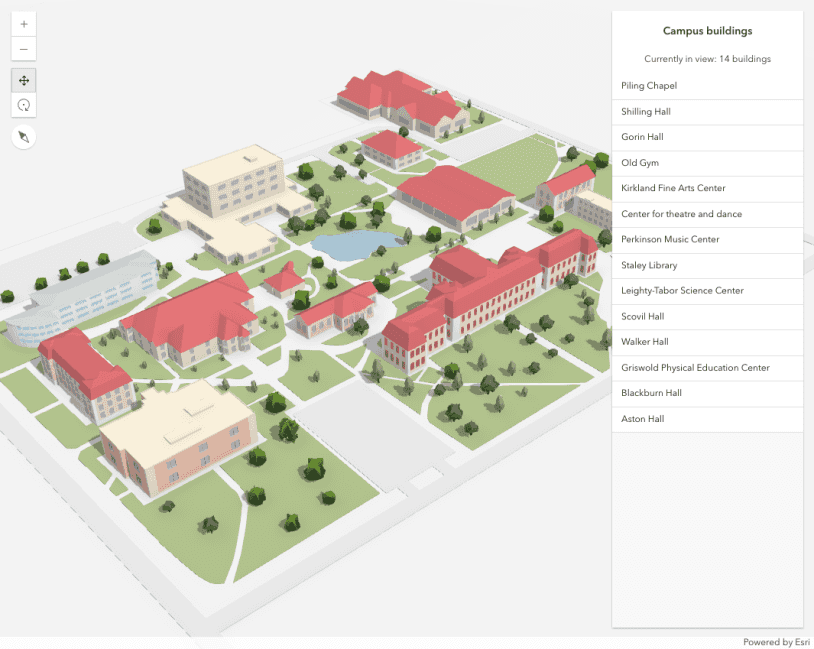
Highlight SceneLayer
Highlight SceneLayer
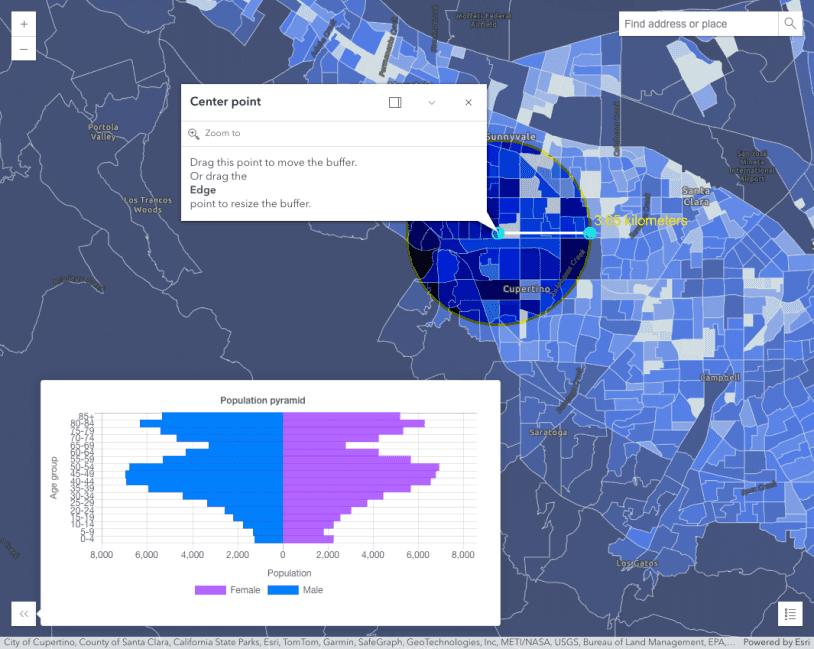
FeatureLayerView - query statistics by geometry
FeatureLayerView - query statistics by geometry
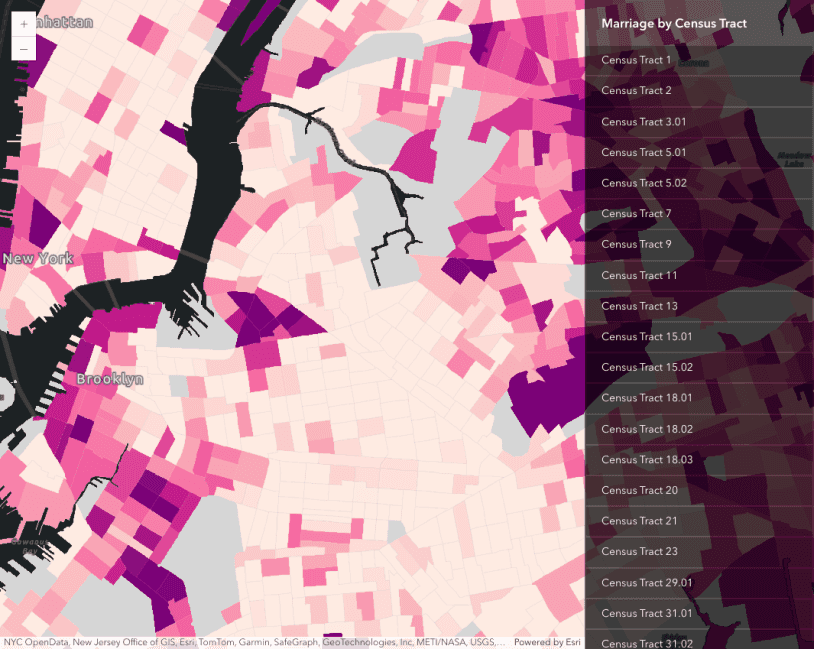
Query features from a FeatureLayerView
Query features from a FeatureLayerView
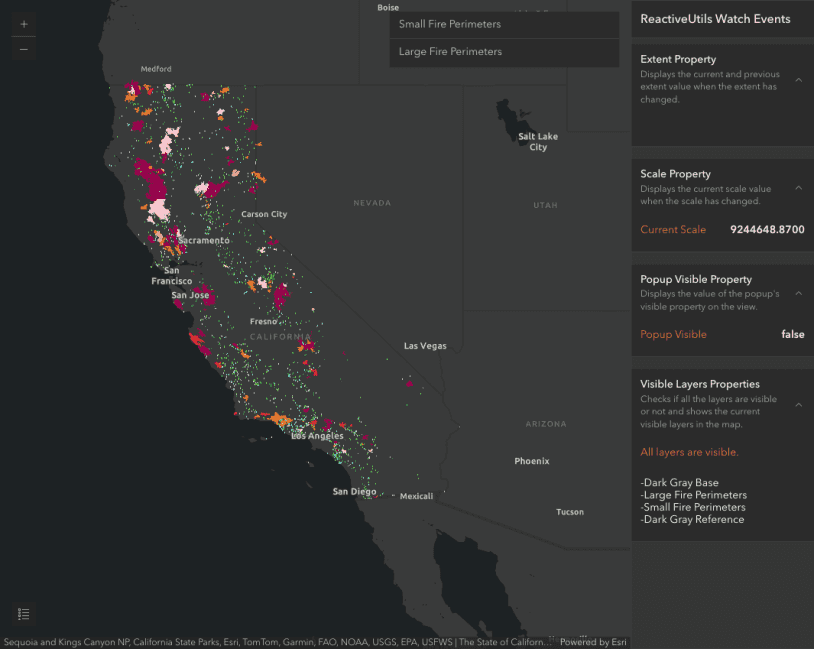
Property Changes with ReactiveUtils
Property Changes with ReactiveUtils
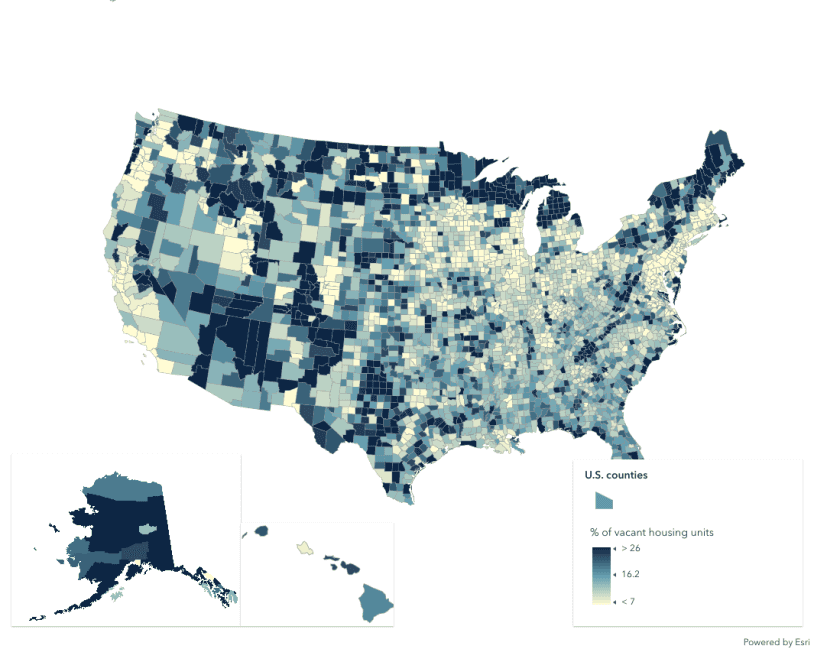
Create an app with composite views
Create an app with composite views