Learn how to search for businesses, POIs, and geographic locations with the geocoding service.
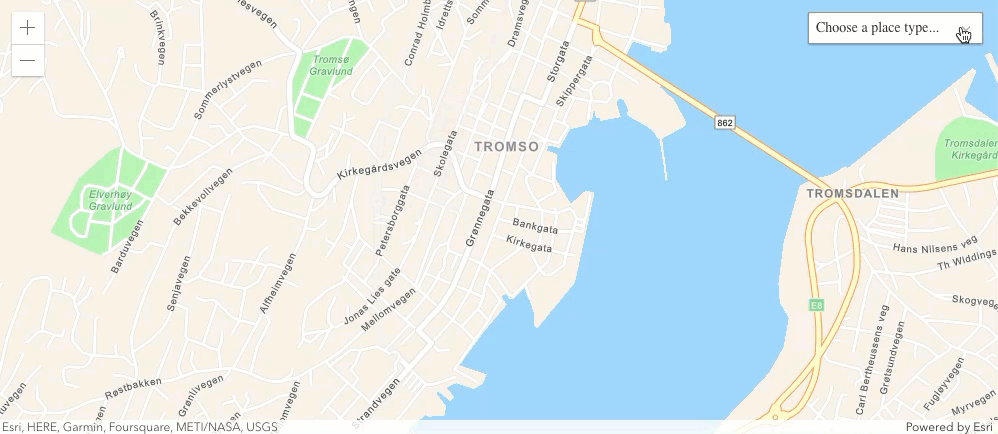
Place finding is the process of searching for a place name or POI to find its address and location. You can use the Geocoding service to find places such as coffee shops, gas stations, or restaurants for any geographic location around the world. You can search for places by name or by using categories. You can search near a location or you can search globally.
In this tutorial, you will use a locator
to access the Geocoding service and find places by place category. Pop-ups are used to display the place name and address.
Prerequisites
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the location services used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Location services > Places
- Privileges
- In CodePen, set
esri
to your access token.Config.api Key Use dark colors for code blocks var esriConfig = { apiKey: "YOUR_ACCESS_TOKEN" };
To learn about other ways to get an access token, go to Types of authentication.
Add modules
-
In the
require
statement, add thelocator
andGraphic
modules.The ArcGIS Maps SDK for JavaScript is available as AMD modules and ES modules, but this tutorial is based on AMD. The AMD
require
function uses references to determine which modules will be loaded – for example, you can specify"esri/layers/
for loading the FeatureLayer module. After the modules are loaded, they are passed as parameters (e.g.Feature Layer" Feature
) to the callback function where they can be used in your application. It is important to keep the module references and callback parameters in the same order. To learn more about the API's different modules visit the Overview Guide page.Layer Use dark colors for code blocks require([ "esri/config", "esri/Map", "esri/views/MapView", "esri/rest/locator", "esri/Graphic", "esri/core/reactiveUtils" ], (esriConfig, Map, MapView, locator, Graphic, reactiveUtils) => {
Update the map
A streets basemap layer is typically used in geocoding applications. Update the basemap
property to use the arcgis/navigation
basemap layer and change the position of the map to center on Tromso.
-
Update the
basemap
property fromarcgis/topographic
toarcgis/navigation
.Use dark colors for code blocks esriConfig.apiKey = "YOUR API KEY"; const map = new Map({ basemap: "arcgis/navigation" });
-
Update the
center
property to[18.9553, 69.6492]
.Use dark colors for code blocks const view = new MapView({ container: "viewDiv", map: map, center: [18.9553, 69.6492], //Longitude, latitude zoom: 13 });
Create a place category selector
You filter place search results by providing a location and category. Places can be filtered by categories such as coffee shop, gas station, and hotels. Use an HTML select
element to provide a list of several categories from which to choose.
-
Create an array of
places
for the categories that will be used to make a selection.Use dark colors for code blocks const view = new MapView({ container: "viewDiv", map: map, center: [18.9553, 69.6492], //Longitude, latitude zoom: 13 }); const places = ["Choose a place type...", "Parks and Outdoors", "Coffee shop", "Gas station", "Food", "Hotel"];
-
Create a parent
select
element for the search categories and assign some styles.Use dark colors for code blocks const places = ["Choose a place type...", "Parks and Outdoors", "Coffee shop", "Gas station", "Food", "Hotel"]; const select = document.createElement("select"); select.setAttribute("class", "esri-widget esri-select"); select.setAttribute("style", "width: 175px; font-family: 'Avenir Next W00'; font-size: 1em");
-
Create an
option
element for each category and add it to theselect
element.Use dark colors for code blocks const select = document.createElement("select"); select.setAttribute("class", "esri-widget esri-select"); select.setAttribute("style", "width: 175px; font-family: 'Avenir Next W00'; font-size: 1em"); places.forEach((p) => { const option = document.createElement("option"); option.value = p; option.innerHTML = p; select.appendChild(option); });
-
Add the
select
element to thetop-right
corner of the view.Use dark colors for code blocks places.forEach((p) => { const option = document.createElement("option"); option.value = p; option.innerHTML = p; select.appendChild(option); }); view.ui.add(select, "top-right");
Define the service url
You can use a locator
to access the Geocoding service.
- Define a variable,
locator
, to the URL for the Geocoding service.Url Use dark colors for code blocks view.ui.add(select, "top-right"); const locatorUrl = "http://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer";
Search for places
To find places, use the locator
address
function. Performing a local search based on a category requires a location from which to search and a category name. The function sends a request to the Geocoding service and the service returns place candidates with a name, address and location information. Use the function to perform a search and add the results to the map as graphics.
-
Create a
find
function and callPlaces address
. Set theTo Locations location
,categories
andout
properties.Fields Use dark colors for code blocks const locatorUrl = "http://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"; // Find places and add them to the map function findPlaces(category, pt) { locator .addressToLocations(locatorUrl, { location: pt, categories: [category], maxLocations: 25, outFields: ["Place_addr", "PlaceName"] }) }
-
Clear the view of existing pop-ups and graphics.
Use dark colors for code blocks // Find places and add them to the map function findPlaces(category, pt) { locator .addressToLocations(locatorUrl, { location: pt, categories: [category], maxLocations: 25, outFields: ["Place_addr", "PlaceName"] }) .then((results) => { view.closePopup(); view.graphics.removeAll(); }); }
-
Create a
Graphic
for each result returned. Set theattributes
,geometry
,symbol
andpopup
properties for each. Add each graphic to theTemplate view
.Use dark colors for code blocks .then((results) => { view.closePopup(); view.graphics.removeAll(); results.forEach((result) => { view.graphics.add( new Graphic({ attributes: result.attributes, // Data attributes returned geometry: result.location, // Point returned symbol: { type: "simple-marker", color: "#000000", size: "12px", outline: { color: "#ffffff", width: "2px" } }, popupTemplate: { title: "{PlaceName}", // Data attribute names content: "{Place_addr}" } }) ); }); });
-
Call the
find
function when the view loads and each time the view changes and becomes stationary.Places Use dark colors for code blocks popupTemplate: { title: "{PlaceName}", // Data attribute names content: "{Place_addr}" } }) ); }); }); } // Search for places in center of map reactiveUtils.when( () => view.stationary, () => { findPlaces(select.value, view.center); } );
-
Places for parks should be displayed on the map. By dragging the map, you will see new places populate the view.
Add a handler to select a category
Use an event handler to call the find
function when the category is changed.
- Add an event listener to listen for category changes.
Use dark colors for code blocks // Search for places in center of map reactiveUtils.when( () => view.stationary, () => { findPlaces(select.value, view.center); } ); // Listen for category changes and find places select.addEventListener("change", (event) => { findPlaces(event.target.value, view.center); });
Run the app
In CodePen, run your code to display the map.
When you select a category, you should see places displayed in the center of the map for the category you selected. The search occurs when the map loads and when the map view position changes by zooming or panning. You can also click on the graphics to display pop-ups with the name and address information for each place.
What's next?
Learn how to use additional API features and ArcGIS services in these tutorials: